Program to calculate the Sum of Digits in C
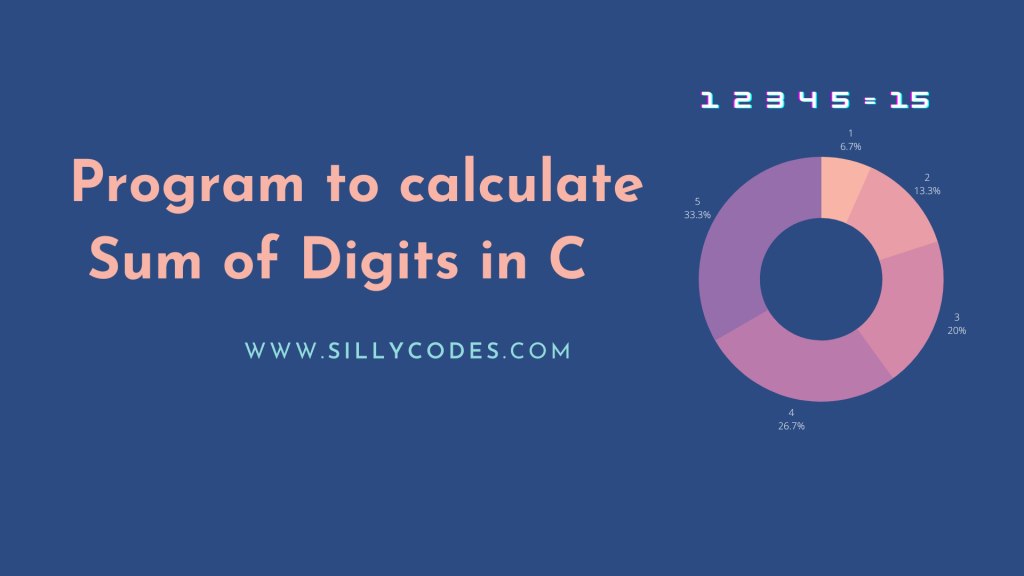
Program Description:
Write a program to calculate the Sum of Digits in C language. The program should accept a number from the user and calculate the sum of all the individual digits. We are going to use the C Loops ( while, for, etc) along with the division and modulus operators to calculate the sum of digits.
The program should show display the error message if the user enters an Invalid input ( i.e Negative numbers, etc). Here is an example expected inputs and outputs of the program.
Expected Input and Output:
Example 1:
Input:
Enter a Positive Number: 1234
Output:
Sum of Digits of 1234 is: 10
Example 2:
Input:
Enter a Positive Number: -9
Output:
ERROR: Please enter valid number
Program Pre-Requisites:
We are going to use the while, for loops, modulus, and division operators to calculate the sum of digits. Please go through the following articles to learn more
- While Loop in C Language
- For Loop in C Language with Example Programs
- Division Operator
- Modulus Operator
Sum of digits in C Program Algorithm:
- The idea is to get the individual digits of the given number ( num) and Add them to get the sum of the digits.
- We will maintain one variable sum to hold the sum of the digits. This sum variable will be initialized to zero. We need a way to go through all digits one by one and add them to “sum” variable.
- Then, We will use the modulus operator and division operators to get
each digit.
- The modulus operator is useful to get the last digit of any number. If we do the modulus of any number with 10, We will get the last digit.
- For example 123 % 10 = 3
- Similarly, We can remove the last digit from the number by dividing the given number by 10.
- For example: 123 / 10 = 12
- So we are going to use the above two operators to get the last digit and remove the last digit.
- The modulus operator is useful to get the last digit of any number. If we do the modulus of any number with 10, We will get the last digit.
- Our loop will start from the last digit of the number and continue till we reach the first digit.
- At each iteration,
- Get the last digit using the modulus operator and then Add the last digit to the sum variable.
- Remove the last digit from the number ( num or temp) by using the division operator.
- Repeat the above steps until our number( temp) becomes less than 0. Which is the stopping condition for our loop. i.e temp > 0
- Once all iterations are complete, The sum variable contains the sum of all digits in a given number.
Sum of Digits in C Program using while loop:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/* Program: Sum of digits of number using while loop */ #include<stdio.h> int main() { // User Input int num, temp; printf("Enter a Positive Number: "); scanf("%d", &num); // sum will be zero at the start int sum = 0; if(num > 0) { // take temparory number temp = num; // Iterate over each digit using modulus and division operator. while(temp > 0) { sum = sum + temp%10; temp = temp/10; } } else { // Negative Number, Display error message and return. printf("ERROR: Please enter valid number\n"); return 0; } // print the results on console. printf("Sum of Digits of %d is: %d \n", num, sum); return 0; } |
Program Output:
1 2 3 4 5 |
$ gcc sum-of-digits.c $ ./a.out Enter a Positive Number: 1234 Sum of Digits of 1234 is: 10 $ |
Example Walk-Through – Calculate Sum of digits in C Program:
Let’s take an example number, num = 1234. and here is the step-by-step walkthrough of the program.
At the first iteration:
- Initially the sum variable will be zero and we used temp variable, which holds the value of num.
- Get the last digit using the modulus operator, Present
temp value is
1234
- temp % 10 which is 1234 % 10 = 4
- Add the last digit to
sum variable. The present value of
sum = 0
- sum = 0 + 4 the sum becomes 4 (sum = 4)
- Remove the last digit from the number using the division operator.
- temp = temp / 10 which is equal to temp = 1234 / 10 and the temp becomes 123 ( i.e temp = 123)
- After the first iteration, The sum is 4, and the temp is 123.
Second Iteration:
- Get the last digit using the modulus operator, Present
temp value is
123
- temp % 10 which is 123 % 10 = 3
- Add the last digit to
sum variable. The present value of
sum = 4
- sum = 4 + 3 the sum becomes 7 (sum = 7)
- Remove the last digit from the number using the division operator.
- temp = temp / 10 which is equal to temp = 123 / 10 and the temp becomes 12( i.e temp = 12)
- After the second iteration, The sum is 7, and the temp is 12.
Third Iteration:
- Get the last digit using the modulus operator, Present
temp value is
12
- temp % 10 which is 12 % 10 = 2
- Add the last digit to
sum variable. The present value of
sum = 7
- sum = 7 + 2the sum becomes 9 (sum = 9)
- Remove the last digit from the number using the division operator.
- temp = temp / 10 which is equal to temp = 12 / 10 and the temp becomes 1 ( i.e temp = 1)
- After the Third iteration, The sum is 9, and the temp is 1.
Fourth Iteration:
- Get the last digit using the modulus operator, Present
temp value is
1
- temp % 10 which is 1 % 10 = 1
- Add the last digit to
sum variable. The present value of
sum = 9
- sum = 9 + 1the sum becomes 10 (i.e sum = 10)
- Remove the last digit from the number using the division operator.
- temp = temp / 10 which is equal to temp = 1 / 10 and the temp becomes ( i.e temp = 0)
- After the fourth iteration, The sum is 10, and the temp is .
Fifth Iteration:
- The loop condition num > 0 will become False and The loop will terminate.
- The final value of the Sum of all digits is sum = 10.
Program to calculate Sum of digits in C using for loop:
Let’s rewrite the program using the for loop. The program logic will remain the same but this will differ in syntax.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
/* Program: Sum of digits of number using for loop */ #include<stdio.h> int main() { // User Input int num, temp; printf("Enter a Positive Number: "); scanf("%d", &num); // sum will be zero at the start int sum = 0; if(num > 0) { // Iterate over each digit using modulus and division operator. // We are using the for loop. // we can take advantage of the for loops // 'Init' and 'update' options. So we are assinging and updating the temp in these for loop options. for (temp = num; temp > 0; temp = temp / 10) { sum = sum + temp%10; } } else { // Negative Number, Display error message and return. printf("ERROR: Please enter valid number\n"); return 0; } // print the results on console. printf("Sum of Digits of %d is: %d \n", num, sum); return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc sum-of-digits.c $ ./a.out Enter a Positive Number: 12345 Sum of Digits of 12345 is: 15 $ ./a.out Enter a Positive Number: 9483 Sum of Digits of 9483 is: 24 $ ./a.out Enter a Positive Number: 0987 Sum of Digits of 987 is: 24 $ ./a.out Enter a Positive Number: 3746334 Sum of Digits of 3746334 is: 30 $ |
If the user enters a Negative number.
1 2 3 4 |
$ ./a.out Enter a Positive Number: -345 ERROR: Please enter valid number $ |
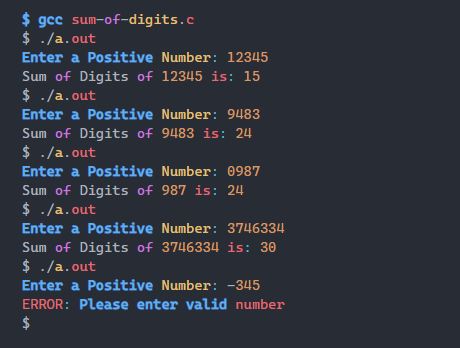
Exercise Programs:
- Rewrite the above sum of digits program using the do while loop.
9 Responses
[…] C Program to find sum of digits of a Number | Sum of Digits program in C – SillyCodes […]
[…] C Program to find sum of digits of a Number | Sum of Digits program in C – SillyCodes […]
[…] C Program to find sum of digits of a Number | Sum of Digits program in C – SillyCodes […]
[…] C Program to find sum of digits of a Number | Sum of Digits program in C – SillyCodes […]
[…] C Program to find sum of digits of a Number | Sum of Digits program in C – SillyCodes […]
[…] C Program to find sum of digits of a Number | Sum of Digits program in C – SillyCodes […]
[…] C Program to Calculate Sum of Digits of a Number […]
[…] C Program to Calculate Sum of Digits of a Number […]
[…] 📢 Related Program: Program to Calculate Sum of Digits of Number using Iterative Method […]