Modulus Operator in C Programming with Example programs
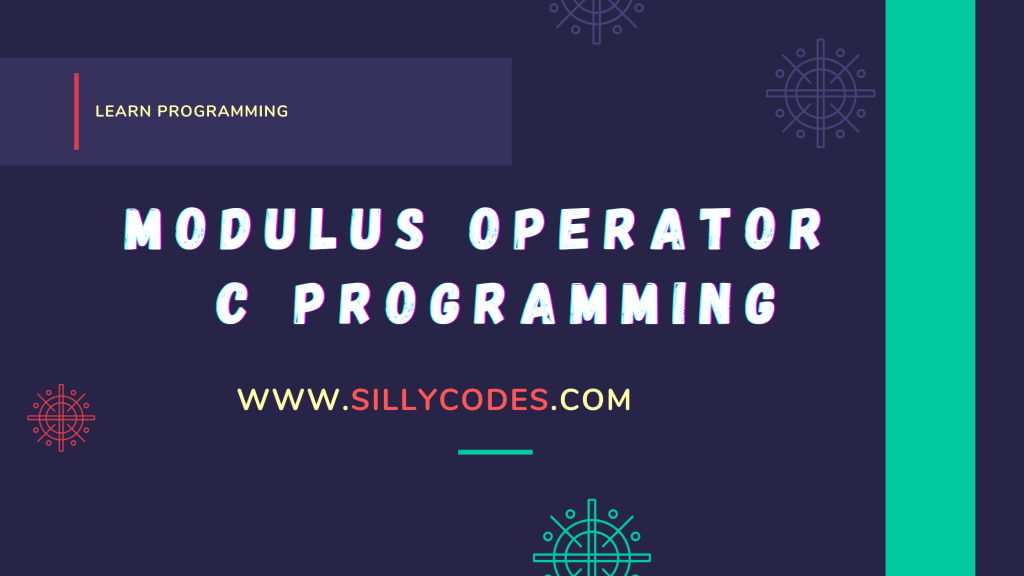
Introduction:
In our previous article, We have learned about the Division Operator in C Programming. In today’s article, We will continue with arithmetic operators and discuss the Modulus Operator in C Language.
What is Modulus Operator:
Modulus Operator gives the Remainder of the division of one number by another.Â
C Programming Language supports the modulus operator. Which is used to calculate the remainder of two values.
We denote the modulus operator using the Percentage ( % ) Symbol in C programming.
Modulus Operator Examples:
Let’s say we have two numbers 11 and 5,
The Modulus of 11 and 5 is 11 % 5 which is equal to 1.
As we discussed earlier, the Modulus operator returns the remainder value ( after division) as the result.
Here, The statement 11 % 5 will give us the result as 1, Because 11 divided by 5 gives us the quotient as 2 and remainder as 1.
11 % 5 = 1
Modulus Operator example Program:
Let’s write a simple program to demonstrate the modulus operator.
1 2 3 4 5 6 7 8 9 |
#include <stdio.h> int main() { Â Â Â Â int a; Â Â Â Â // '%' is the modulus Operator. Â Â Â Â a = 11 % 2; Â Â Â Â printf("Result is : %d \n", a); Â Â Â Â return 0; } |
Program Output:
1 |
Result is : 1 |
The output of the above program is 1. The remainder of the 11 % 5 Operation.
Modulus Operator results Sign:
Modulus Operator output sign always depends upon the Numerator sign only.
So the resulting remainder sign will be based on the Numerator sign.
Program – Modulus operator Sign:
1 2 3 4 5 6 7 8 9 10 |
#include<stdio.h> int main() {     int a;     printf("a = %d \n",  11 % 2);     printf("a = %d \n", -11 % 2);     printf("a = %d \n",  11 % -2);     printf("a = %d \n", -11 % -2);     return 0; } |
Program Output:
1 2 3 4 |
a = 1 a = -1 a = 1 a = -1 |
Form Above Example that is clear Modulus Operator Sign is always Depends on Numerator Sign Only.
Modulus Operator when Numerator less than Denominator:
When the Numerator Value is less than the denominator Value, then the modulus operator result will be Numerator Value.
For example,
44 % 100
Here, The 44 is less than the 100, So the modulus operator result will be 44.
Example Program :
1 2 3 4 5 6 7 |
#include <stdio.h> int main() { Â Â Â Â printf(" Result = %d \n", 1 % 5); Â Â Â Â printf(" Result = %d \n", 5 % 10); Â Â Â Â return 0; } |
Output:
1 2 |
Result = 1 Result = 5 |
No Modulus Operator for Float Data:
The C Language modulus operator is not allowed on floating-point data.
Modulus Operators both arguments i.e numerator and denominator must be integer values.
Using floating-point values with The modulus operator will result in the Compilation error.
Here is an example program,
Program with Float data:
In this program, We are going to calculate the modulus of the integer variable and float variable.
1 2 3 4 5 6 7 8 |
#include <stdio.h> int main()     {     int a = 10;     float b = 3.14;     printf("Result is %d \n", a % b );     return 0; } |
Program Output:
As the modulus operator won’t accept the float values, the above program will generate a compilation error.
1 2 3 4 |
modulus1.c:6:34: error: invalid operands to binary expression ('int' and 'float') Â Â Â Â printf("Result is %d \n", a % b ); Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â ~ ^ ~ 1 error generated. |
How to calculate the Modulus of Float Data in C:
If you want to calculate the modulus of the floating-point data in C language, Then we need to use the fmod() and fmodl() library functions.
The fmod() and fmodl() functions are available as part of the math.h library.
fmod function:
Syntax of fmod function.
1 |
double fmod(double x, double y); |
The fmod() function can be used to calculate the Modulus or Remainder of the float datatype and Double datatype values.
fmodl function:
Syntax of fmodl function
1 |
long double fmodl(long double x, long double y); |
Similarly, The fmodl() function can calculate the Modulus of Long Double data values.
Program to calculate the modulus of Float data:
In this program, We are calculating the modulus of the float data using math.h fmod function.
1 2 3 4 5 6 7 8 9 |
#include <stdio.h> #include <math.h> int main(void) {     float f = 5.5;     int a = 3;     printf("Result is %f \n", fmod(f, a) );     return 0; } |
Output:
1 |
Result is 2.500000 |
As you can from the output, We got the desired result of 2.500000
So by using the fmod and fmodl functions we can calculate the modulus of the floating-point data.
Related Articles:
- Start Here – Step by step tutorials to Learn C programming Online with Example Programs – SillyCodes
- Come Together – The Collection of all Pattern Programs in C – SillyCodes
8 Responses
[…] Modulus Operator […]
[…] Modulus Operator […]
[…] Modulus Operator in C Programming with Example programs – SillyCodes […]
[…] Modulus Operator in C […]
[…] Modulus Operator in C […]
[…] to know the basics of the C Loops like the While Loop, For loop, And Arithmetic operators like Modulus operators, and Division […]
[…] Modulus Operator in C language […]
[…] Modulus Operator in C […]