3-Digit / N-Digit Armstrong Number Program in C Language
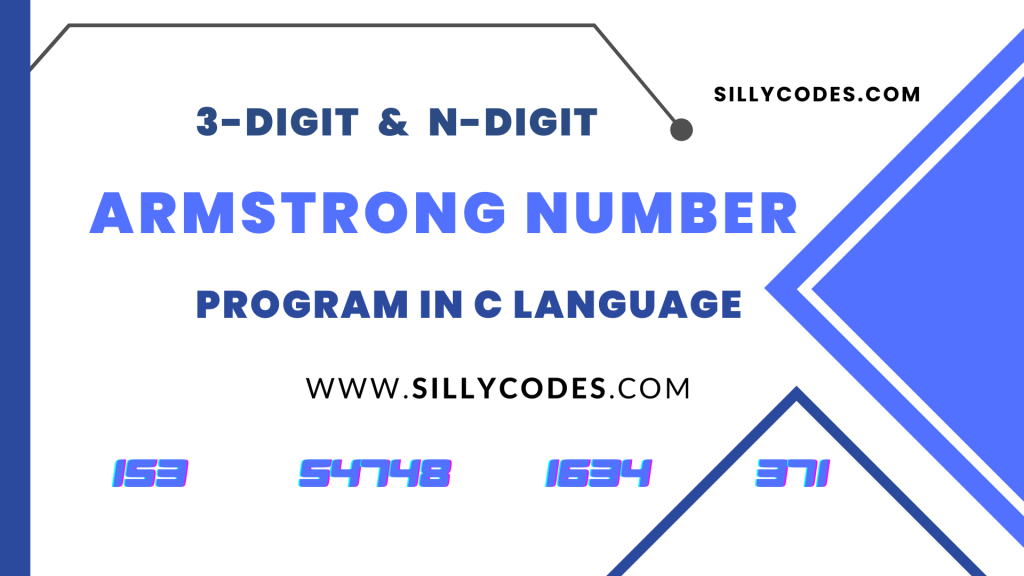
Program Description:
Write a Program to check if the given number is Armstrong Number in C Language. We are going to check the 3-Digit Armstrong number program and also the N-Digit Armstrong number program as well. The program should accept a positive number from the user and display if the number is an Armstrong number or not.
What is an Armstrong Number?
An Armstrong number of an integer such that the sum of the cubes of its digits is equal to the number itself. Which is a 3-digit Armstrong Number.
Let’s look at a couple of 3-digit Armstrong number examples
Example 1:
Number 371 is an Armstrong number
since 3^3 + 7^3 + 1^3 = 371
Example 2:
Number 153 is also an Armstrong number
since 1^3 + 5^3 + 3^3 = 153
The above numbers are Three digits Armstrong numbers.
What is an N-Digit Armstrong Number?
A positive number of n digits is said to be an Armstrong number of order n, If the sum of the power of n of each digit in the number is equal to the original number itself.
If the number is qwer is Armstrong number, Then it should satisfy the below equation.
qwer = pow(q, n) + pow(w, n) + pow(e, n) + pow(r, n)
Here n is the number of digits in the number qwer which is 4
For Four digit Armstrong numbers, We need to calculate the digit ^ 4 for each digit.
Similarly, for Five digit Armstrong numbers, We need to calculate the digit ^ 5 for each digit.
Here is an example, The number 54748 is an Armstrong number, So ( n = 5 i.e number of digits in the 54748)
54748 = pow(5, 5) + pow(4, 5) + pow(7, 5) + pow(4, 5) + pow(8, 5)
54748 = 3125 + 1024 + 16807 + 1024 + 32768
54748 = 54748
As the powers of n of each digit in the number is equal to the number itself. So the number 54748 is an Armstrong number.
Another example, The number 1634 is a Four digit Armstrong number as 1634 = 1^4 + 6^4 + 3^4 + 4^4.
Here is the list of Armstrong numbers ( based on the number of digits).
Digit | Armstrong Numbers |
---|---|
1 | 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 |
3 | 153, 370, 371, 407 |
4 | 1634, 8208, 9474 |
5 | 54748, 92727, 93084 |
6 | 548834 |
7 | 1741725, 4210818, 9800817, 9926315 |
Program Pre-Requisites:
We need to have knowledge of C Loops and Arithmetic Operators. Please read the following articles to learn more.
We are going to write two programs to calculate the Armstrong number.
- Armstrong number Program for Three digits Number in C
- Armstrong number Program for N-Digits number in C.
Armstrong Number Program in C Language Algorithm [For 3 digit Number]:
In Nutshell, We need to get each digit of the number and then calculate the cubes of each digit. Then add all cubes which should be equal to the given number. If the cubes are equal to the given number then it’s an Armstrong number. Otherwise, it is not an Armstrong number.
Here is the Algorithm.
- Take the input from the user and store it in variable n.
- Check if the given number n is positive or negative. Display an error message if the number n is negative.
- Let’s use an extra variable temp to hold the input number n. As we need 'n' to verify our result.
- Start the Loop, The loop will continue as long as the variable temp is greater than Zero(0). – temp > 0.
- At Each Iteration
- We are going to use the Modulus operator to get the last digit of the number, We can get the last digit of any number by performing the
modulus with
10.
- For example, 8798 % 10 = 8
- Once we get the last digit, Calculate the cube of the digit. Then add to arm variable ( arm variable holds the Armstrong number so far). The arm variable is initialized with zero at the start. and We will add the Cubes of each digit to the arm variable.
- As we processed the last digit, We can remove the last digit of the number
temp by dividing the number by using the number
10.
- For example, 8798 / 10 = 879
- We are going to use the Modulus operator to get the last digit of the number, We can get the last digit of any number by performing the
modulus with
10.
- The above step-5 continues until temp > 0 becomes
False.
- So at each iteration, we are going to get the last digit and then calculate the cube of that digit, Then Add the cube to the arm variable. Finally, We removed the last digit from the number. So once we pass through the all digits of the temp. The temp will become less than zero. And our loop will be terminated.
- Check if the
arm is equal to the original number
n.
- If both arm number and variable n are equal, Then the given number n is an Armstrong number.
- If numbers arm and n are not equal, Then n is not an Armstrong number.
Check or Detect an Armstrong Number program in C Language [For 3-Digit Number]:
Here is the Armstrong number program implementation in C language. We are using the while loop to iterate over the number ( temp).
The program also performs the error check and displays an error message on Invalid inputs like negative numbers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
/* Program: Armstrong Number program in C Langauge Author: Sillycodes.com */ #include<stdio.h> int main() { int n,temp,rem,arm; arm = 0; printf("Enter a positive number : "); scanf("%d",&n); // check if the 'n' is negative. Display the error message. if(n <= 0) { printf("Error: Please enter positive number\n"); return 0; } // Take backup of number 'n' temp = n; // Go through the each digit and calculate the cube of each digit // add the cubes to 'arm' variable. which will be holding sum of all cubes while(temp>0) { rem = temp %10; arm = arm + (rem*rem*rem); temp = temp/10; } // After all iterations, The 'arm' variable contains the sum of cubes of each digit. // if 'arm' is equal to the input number 'n', Then the 'n' is Armstrong number. if(arm == n) printf("Number %d is an Armstrong Number \n", n); else printf("Number %d Not an Armstrong Number\n", n); return 0; } |
Armstrong Number Program in C Program Output:
Compile and run the program. We are using the GCC compiler under the Linux Operating system to Run and compile the program.
gcc armstrong.c
Above command, generate the executable file named a.out. Run the program using the ./a.out command.
Test Case 1: Positive Numbers
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ ./a.out Enter a positive number : 153 Number 153 is an Armstrong Number $ $ ./a.out Enter a positive number : 475 Number 475 Not an Armstrong Number $ ./a.out Enter a positive number : 928 Number 928 Not an Armstrong Number $ ./a.out Enter a positive number : 371 Number 371 is an Armstrong Number $ |
As you can see from the above output, We are getting the expected results.
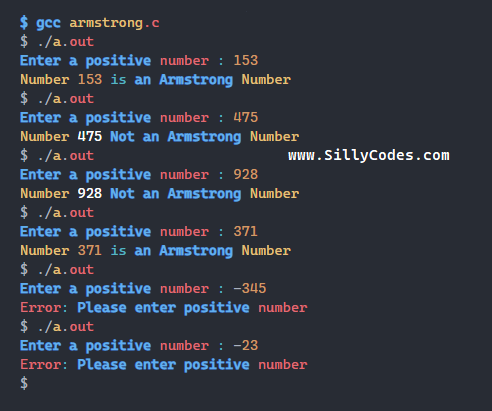
Let’s also verify the output for the negative numbers.
1 2 3 4 5 6 7 |
$ ./a.out Enter a positive number : -345 Error: Please enter positive number $ ./a.out Enter a positive number : -23 Error: Please enter positive number $ |
The program is displaying the error message when the user enters a Negative number – Error: Please enter positive number
Armstrong Number Program in C for N-Digits Number Algorithm:
For the three-digit Armstrong number, We calculated the Cubes of each digit. Similarly, for N-Digits Armstrong numbers we need to calculate the power of nDigits i.e pow(digit, nDigits).
Here is the algorithm to check the N-Digit Armstrong number
- Take the input from the user and store it in a variable n. Display error if the n is a Negative number.
- First of all, We need to Calculate the Number of digits in the number. Which is used to calculate the power. We used a function countDigits and passed the input number n to calculate the number of digits and countDigits function returns the number of digits in the number( n).
- Then we need to go through each digit of the given number
n using the Loop.
- At Each Iteration,
- Get the digit from the number i.e n . rem = temp %10;
- Calculate the power of rem with nDigits(Number of digits in n). We are using the Power function from the math.h header file – pow(rem, nDigits);. Update the arm variable.
- Remove the digit using the temp/10 operation.
- At Each Iteration,
- Once the above loop is terminated, If the arm variable is equal to n, Then given the number n is N-digit Armstrong Number.
N-Digit Armstrong Number Program in C Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 |
/* Program: N-Digit Armstrong Number program in C Langauge Author: Sillycodes.com */ #include<stdio.h> #include<math.h> /* * count number of digits of given number 'num' */ int countDigits(int num) { int digitCnt = 0; // Number is positive // take backup of 'num', we can also directly use 'num' int temp = num; // Iterate over each digit using division operator. while(temp > 0) { // increment the number of digits count (digitCnt) digitCnt++; // remove the last element using division operator. temp = temp / 10; } return digitCnt; } int main() { int n,temp,rem,arm; arm = 0; printf("Enter a positive number : "); scanf("%d",&n); // check if the 'n' is negative. Display the error message. if(n <= 0) { printf("Error: Please enter positive number\n"); return 0; } // Take backup of number 'n' temp = n; // count number of digits in Number // Use - https://sillycodes.com/program-to-count-number-of-digits-in-c/ int nDigits = countDigits(n); // Go through the each digit and calculate the power(digit, nDigit) // add the powers to 'arm' variable. which will be holding sum of all powers while(temp>0) { // get the last digit of number or current digit using modulus rem = temp %10; // calculate the power of 'rem' with 'nDigits' // Use - https://sillycodes.com/program-to-find-power-of-number-in-c/ arm = arm + pow(rem, nDigits); // Remove the last digit by dividing the 'temp' by '10' temp = temp/10; } // After all iterations, The 'arm' variable contains the sum of power of each digit. // if 'arm' is equal to the input number 'n', Then the 'n' is Armstrong number. if(arm == n) printf("Number %d is an Armstrong Number \n", n); else printf("Number %d Not an Armstrong Number\n", n); return 0; } |
As we have used the pow function, We need to include the math.h header file.
Program Output:
The pow function available as part of the libm.so. So We need to pass the library function to the GCC using the -lm option.
Here is the command to compile the C Program which using the functions from the math.h header file.
gcc armstrong-n-digits.c -lm
The above command generates the output file named a.out. Run the executable.
Let’s give the 4 digit Armstrong numbers to the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter a positive number : 8208 Number 8208 is an Armstrong Number $ ./a.out Enter a positive number : 9474 Number 9474 is an Armstrong Number $ ./a.out Enter a positive number : 1634 Number 1634 is an Armstrong Number $ ./a.out Enter a positive number : 2002 Number 2002 Not an Armstrong Number $ |
As expected, The program is able to detect the 4-digit Armstrong numbers.
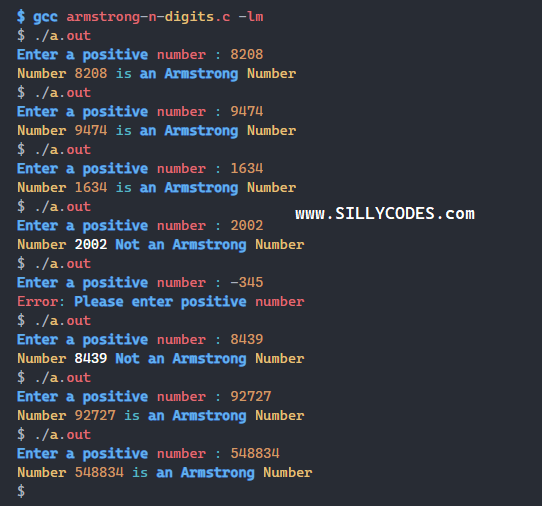
Let’s try 5-digit Armstrong number.
1 2 3 4 |
$ ./a.out Enter a positive number : 92727 Number 92727 is an Armstrong Number $ |
How about 6-Digit Armstrong Number?
1 2 3 4 |
$ ./a.out Enter a positive number : 548834 Number 548834 is an Armstrong Number $ |
The program is working as expected. If you plan to use the very large numbers, Then make sure to use the appropriate datatypes like (long int, long long int, etc).
N Digit Armstron Number without using Inbuilt Functions:
In the above program, We used the in-built pow function to calculate the power of the number. Let’s use our custom pow function instead of the pow library function.
We are using the Power of Number Program in C to calculate the power instead of pow built-in function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 |
/* Program: N-Digit Armstrong Number program in C Langauge without Built-in Functions Author: Sillycodes.com */ #include<stdio.h> /* * count number of digits of given number 'num' * Lean more at - https://sillycodes.com/program-to-count-number-of-digits-in-c/ */ int countDigits(int num) { int digitCnt = 0; // Number is positive // take backup of 'num', we can also directly use 'num' int temp = num; // Iterate over each digit using division operator. while(temp > 0) { // increment the number of digits count (digitCnt) digitCnt++; // remove the last element using division operator. temp = temp / 10; } return digitCnt; } /* * Calculate the power of number * Learn more at - https://sillycodes.com/program-to-find-power-of-number-in-c/#5-method-11-calculate-the-power-of-number-in-c-language-using-while-loop- */ double power(double base, double exponent) { long double res = 1.0; // The power of base and exponent is equal to ( base multiplied exponent times) // So loop until the exponent became zero. while(exponent > 0) { res = res * base; // res *= base; exponent--; } return res; } int main() { int n,temp,rem,arm; arm = 0; printf("Enter a positive number : "); scanf("%d",&n); // check if the 'n' is negative. Display the error message. if(n <= 0) { printf("Error: Please enter positive number\n"); return 0; } // Take backup of number 'n' temp = n; // count number of digits in Number // Use - https://sillycodes.com/program-to-count-number-of-digits-in-c/ int nDigits = countDigits(n); // Go through the each digit and calculate the cube of each digit // add the cubes to 'arm' variable. which will be holding sum of all cubes while(temp>0) { // get the last digit of number or current digit using modulus rem = temp %10; // calculate the power of 'rem' with 'nDigits' // Use - https://sillycodes.com/program-to-find-power-of-number-in-c/ // Using custom power function. Pass 'base' and 'exponent' arm = arm + power(rem, nDigits); // Remove the last digit by dividing the 'temp' by '10' temp = temp/10; } // After all iterations, The 'arm' variable contains the sum of cubes of each digit. // if 'arm' is equal to the input number 'n', Then the 'n' is Armstrong number. if(arm == n) printf("Number %d is an Armstrong Number \n", n); else printf("Number %d Not an Armstrong Number\n", n); return 0; } |
Please note that, We haven’t used the math.h headerfile, As we no longer using the pow function.
Program Output:
Compile and run the program.
gcc armstrong-n-digits-without-pow.c
📢 Note that We haven’t passed -lm Compilation Option to GCC. As we are not using the pow function.
Here is the program output form different digit numbers like 3-digits, 4-digits, 5-digits, and 6-digits Armstrong numbers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
$ ./a.out Enter a positive number : 92727 Number 92727 is an Armstrong Number $ ./a.out Enter a positive number : 548834 Number 548834 is an Armstrong Number $ ./a.out Enter a positive number : 371 Number 371 is an Armstrong Number $ ./a.out Enter a positive number : 1634 Number 1634 is an Armstrong Number $ ./a.out Enter a positive number : 3847 Number 3847 Not an Armstrong Number $ ./a.out Enter a positive number : 2345 Number 2345 Not an Armstrong Number $ ./a.out Enter a positive number : 93084 Number 93084 is an Armstrong Number $ |
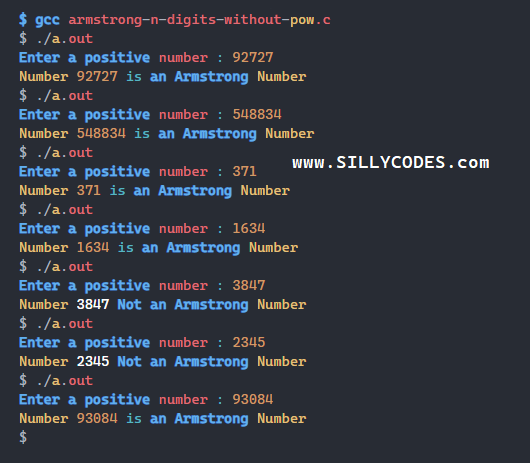
As we can see from the above output, The program is able to calculate the N-Digit Armstrong numbers correctly.
Related Programs:
- Sum of Digits of Number in C
- Program to calculate Product of Digits of a Number in C
- Program to count number of digits of a Number in C
- C program to print all ASCII characters and ASCII values/numbers – SillyCodes
9 Responses
[…] Armstrong Number Program in C Language – SillyCodes […]
[…] Armstrong Number Program in C Language – SillyCodes […]
[…] Armstrong Number Program in C Language – SillyCodes […]
[…] Armstrong Number Program in C Language – SillyCodes […]
[…] Armstrong Number Program in C Language – SillyCodes […]
[…] C Program to Check Armstrong Number […]
[…] our previous article, We have looked at the 3-Digit and N-digit Armstrong number program. In today’s article, We are going to extend that program and will write a program to generate […]
[…] Program Check 3-digit / N-digit Armstrong Numbers in C language […]
[…] C Program to Check 3-Digit and N-Digit Armstrong Number […]