Armstrong numbers between two intervals in C Program
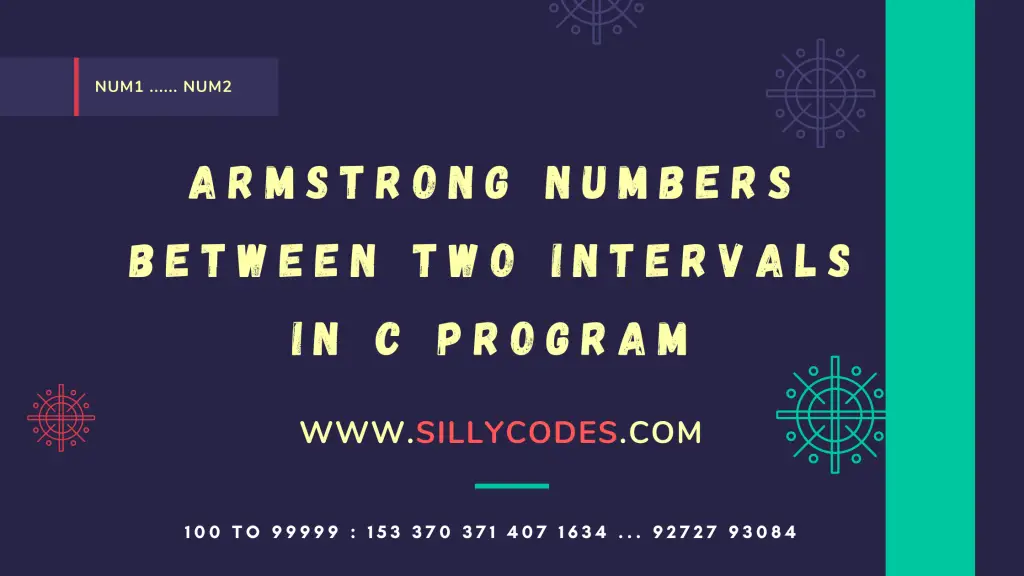
Armstrong numbers between two intervals in C Program Description:
Write a Program to generate Armstrong numbers between two intervals in C programming language using loops. The program should accept two positive integers from the user and display all Armstrong numbers between the given numbers (given interval).
📢 The Program should generate all digits of Armstrong numbers i.e 3-digit and N-digit Armstrong numbers
This program is useful to generate the Armstrong numbers between the numbers. Like you may want to know all Armstrong numbers from 1 to 1000, or You may want to know all Armstrong numbers from 100 to 10000, etc. So by using this program you can easily get all Armstrong numbers between two intervals.
Here are the expected input and output of the program:
Expected Input and Output:
Input:
Enter Two Number: 1 1000
Output:
Armstrong numbers between 1 and 1000 are : 1 2 3 4 5 6 7 8 9 153 370 371 407
What is an N-Digit Armstrong Number?
A positive number of n digits is said to be an Armstrong number of order n, If the sum of the power of n of each digit in the number is equal to the original number itself.
If the number is qwer is Armstrong number, Then it should satisfy the below equation.
qwer = pow(q, n) + pow(w, n) + pow(e, n) + pow(r, n)
Here n is the number of digits in the number qwer which is 4
Few Examples of Armstrong Numbers.
Digit | Armstrong Numbers |
---|---|
1 | 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 |
3 | 153, 370, 371, 407 |
4 | 1634, 8208, 9474 |
5 | 54748, 92727, 93084 |
6 | 548834 |
7 | 1741725, 4210818, 9800817, 9926315 |
Related Armstrong Numbers Programs:
This program is part of the Armstrong numbers programs series. You can find the other programs below.
- Program Check 3-digit / N-digit Armstrong Numbers in C language
- Program to Generate Armstrong Numbers up to n (given number)
Pre-Requisites (Knowledge Required):
It is recommended to know the basics of the C Loops like the While Loop, For loop, And Arithmetic operators like Modulus operators, and Division Operators.
Armstrong numbers between two intervals in C Algorithm:
- Accept two numbers from the user and store them in variables num1 and num2 respectively.
- We don’t allow the negative number for intervals, So Check if any of the numbers ( num1 or num2 or both) is a Negative number, If so display the error message( Error: Please enter positive numbers) and stop the program. Otherwise, proceed to the next step.
- As specified earlier, We need to calculate the pow(digit, nDigits) to check the Armstrong number.
- So we need to count the number of digits in the given number num. To do that, We use a function called countDigits(), Which takes a number as input and returns the number of digits.- int nDigits = countDigits(temp);
- We are going to use two loops, Outer-Loop and Inner-Loop.
- The Outer-Loop: This starts from the number
num1 and goes all the way up to the number
num2. We use the variable
i to control Outer-Loop. – for(i=num1; i<=num2; i++)
- Take the backup of the variable i and store it in variable temp. As we need i for later comparisons.
- The Inner-Loop: Inner-Loop will help us to check if the outer loop variable
i is an Armstrong number or not. If the
i is Armstrong number, Then it will print it onto the console.
- At Each Iteration,
- Get the last digit from the number ( temp) i.e rem = temp %10;
- Calculate the power of rem with nDigits(Number of digits in temp) – i.e power(rem, nDigits);. We are using the Power of Number Program in C to calculate the power instead of the pow built-in function. Then Update the arm variable.
- Remove the last digit using the temp/10 operation.
- Check if the variable arm is equal to i. If both are equal, Then i is an Armstrong number. Print it onto the console.
- The above step-6 will continue until i reaches the second number num2. ( Outer Loop Condition – i<=num2 )
- Once the above two loops are terminated, We will get all Armstrong numbers between the interval of num1 and num2. ( user-provided interval )
Armstrong numbers between two intervals in C Program:
In this program, We are using the custom power function ( double power(double base, double exponent) ) to calculate the power of numbers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 |
/*     Program: Armstrong Number program in C Langauge     Author: Sillycodes.com */  #include<stdio.h>  /* * count number of digits of given number 'num' * Lean more at - https://sillycodes.com/program-to-count-number-of-digits-in-c/ */ int countDigits(int num) {     int digitCnt = 0;     // Number is positive     // take backup of 'num', we can also directly use 'num'     int temp = num;     // Iterate over each digit using division operator.     while(temp > 0) {         // increment the number of digits count (digitCnt)         digitCnt++;         // remove the last element using division operator.         temp = temp / 10;     }      return digitCnt; }  /* * Calculate the power of number * Learn more at - https://sillycodes.com/program-to-find-power-of-number-in-c */ double power(double base, double exponent) {     long double res = 1.0;     // The power of base and exponent is equal to ( base multiplied exponent times)     // So loop until the exponent became zero.     while(exponent > 0) {         res = res * base;  // res *= base;         exponent--;     }     return res; }   int main() {     int num1, num2, temp, rem, arm, i;      // Take the input from the user.     printf("Enter Two Number: ");     scanf("%d%d",&num1, &num2);      // check if either of 'num1' or 'num2' negative. Display the error message.     if(num1 <= 0 || num2 <= 0)     {         printf("Error: Please enter positive numbers\n");         return 0;     }      printf("Armstrong numbers between %d and %d are : ", num1, num2);      // Start the loop from 'num1' go till 'num2'     for(i=num1; i<=num2; i++)     {         // Take backup of number 'n'         temp = i;          // reset 'rem', 'arm' variables         rem = 0;         arm = 0;          // count number of digits in Number         // Use - https://sillycodes.com/program-to-count-number-of-digits-in-c/         int nDigits = countDigits(temp);          // Go through the each digit and calculate the cube of each digit         // add the cubes to 'arm' variable. which will be holding sum of all cubes         while(temp>0)         {             // get the last digit of number or current digit using modulus             rem = temp %10;                         // Using custom power function. Pass 'base' and 'exponent'             // Source - https://sillycodes.com/program-to-find-power-of-number-in-c/             // calculate the power of 'rem' with 'nDigits'             // You can also use `math.h` in-built 'pow' function             arm = arm + power(rem, nDigits);                         // Remove the last digit by dividing the 'temp' by '10'             temp = temp/10;         }          // After all iterations, The 'arm' variable contains the sum of cubes of each digit.         // if 'arm' is equal to the 'i', Then the 'i' is Armstrong number.         if(arm == i)             printf("%d ", i);     }         printf("\n");     return 0; } |
We are using two user-defined functions in this program, They are
The countDigits function:
Prototype:
int countDigits(int num)
The countDigits function is used to count the number of digits in the given number. This function takes num as the input and returns an integer which is the number of digits in num
Learn more about it at Count digits in a Number Program
The power function:
Prototype:
double power(double base, double exponent)
The power function is used to calculate the power of a number. It accepts two numbers base and exponent and calculates the power and returns it (as double).
Learn more about custom power function at Power of Number Program
We can also use the C languages library power function. We will look at in the next section.
Program Output:
Compile the Program. We are using the GCC compiler here.
$ gcc armstrong-between-numbers.c
Run the executable. Let’s generate all Armstrong numbers from 1 to 1000 numbers.
1 2 3 4 |
$ ./a.out Enter Two Number: 1 1000 Armstrong numbers between 1 and 1000 are : 1 2 3 4 5 6 7 8 9 153 370 371 407 $ |
As you can see from the above output, The numbers 1, 2, 3, 4, 5, 6, 7, 8, 9, 153, 370, 371, 407 are the Armstrong numbers between the interval 1 and 1000.
Let’s try a few more examples.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter Two Number: 1000 9999 Armstrong numbers between 1000 and 9999 are : 1634 8208 9474 $ ./a.out Enter Two Number: 10001 99000 Armstrong numbers between 10001 and 99000 are : 54748 92727 93084 $ ./a.out Enter Two Number: 100 500 Armstrong numbers between 100 and 500 are : 153 370 371 407 $ ./a.out Enter Two Number: 1 100000 Armstrong numbers between 1 and 100000 are : 1 2 3 4 5 6 7 8 9 153 370 371 407 1634 8208 9474 54748 92727 93084 $ |
We are getting the expected results, The Armstrong numbers between the number 1 and 100000 are 1, 2, 3, 4, 5, 6, 7, 8, 9, 153, 370, 371, 407, 1634, 8208, 9474, 54748, 92727, 93084.
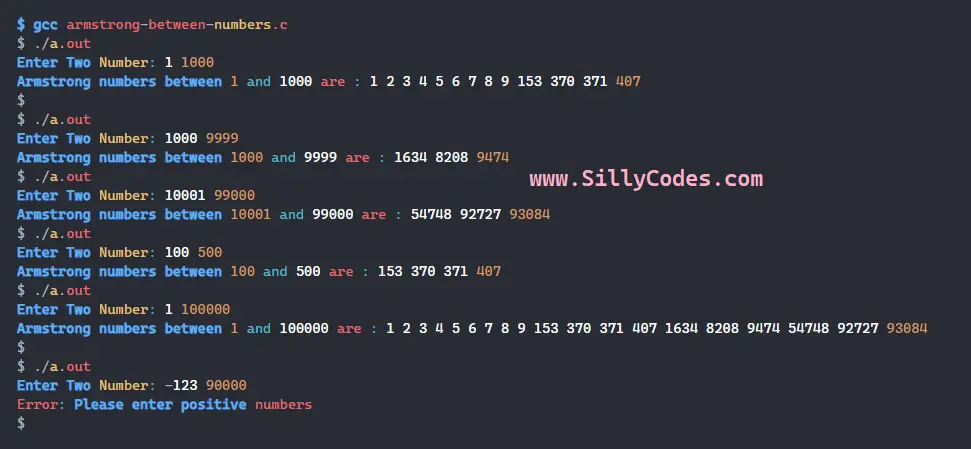
Here is the example with Negative numbers
1 2 3 4 |
$ ./a.out Enter Two Number: -123 90000 Error: Please enter positive numbers $ |
As expected, The program is displaying the error message if the user enters a negative number.
Generate Armstrong numbers between two intervals in C using pow library function:
We have used our custom power function in the above example, But as we already know the C language has an in-built pow function, which is available as part of math.h header files and libm.so library.
Let’s remove our custom power function from the above program and use the in-built pow function.
Here is the pow function syntax:
double pow(double x, double y)
📢 Don’t forget to include the math.h header file
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 |
/*     Program: Armstrong Number program in C Langauge     Author: Sillycodes.com */  #include<stdio.h> #include<math.h>  /* * count number of digits of given number 'num' * Lean more at - https://sillycodes.com/program-to-count-number-of-digits-in-c/ */ int countDigits(int num) {     int digitCnt = 0;     // Number is positive     // take backup of 'num', we can also directly use 'num'     int temp = num;     // Iterate over each digit using division operator.     while(temp > 0) {         // increment the number of digits count (digitCnt)         digitCnt++;         // remove the last element using division operator.         temp = temp / 10;     }      return digitCnt; }  int main() {     int num1, num2, temp, rem, arm, i;      // Take the input from the user.     printf("Enter Two Number: ");     scanf("%d%d",&num1, &num2);      // check if either of 'num1' or 'num2' negative. Display the error message.     if(num1 <= 0 || num2 <= 0)     {         printf("Error: Please enter positive numbers\n");         return 0;     }      printf("Armstrong numbers between %d and %d are : ", num1, num2);      // Start the loop from 'num1' go till 'num2'     for(i=num1; i<=num2; i++)     {         // Take backup of number 'n'         temp = i;          // reset 'rem', 'arm' variables         rem = 0;         arm = 0;          // count number of digits in Number         // Use - https://sillycodes.com/program-to-count-number-of-digits-in-c/         int nDigits = countDigits(temp);          // Go through the each digit and calculate the cube of each digit         // add the cubes to 'arm' variable. which will be holding sum of all cubes         while(temp>0)         {             // get the last digit of number or current digit using modulus             rem = temp %10;                         // using in-built pow function.             // calculate the power of 'rem' with 'nDigits'             arm = arm + pow(rem, nDigits);                         // Remove the last digit by dividing the 'temp' by '10'             temp = temp/10;         }          // After all iterations, The 'arm' variable contains the sum of cubes of each digit.         // if 'arm' is equal to the 'i', Then the 'i' is Armstrong number.         if(arm == i)             printf("%d ", i);     }         printf("\n");     return 0; } |
Program Output:
As we are using the pow function from the math.h headerfile, We need to pass the -lm compilation option. Otherwise, we will get a Linking error like below.
1 2 3 4 5 |
$ gcc armstrong-between-interval.c /usr/bin/ld: /tmp/cc816tVA.o: in function `main': armstrong-between-interval.c:(.text+0x165): undefined reference to `pow' collect2: error: ld returned 1 exit status $ |
So let’s add the -lm option as well to compile the program.
$ gcc armstrong-between-interval.c -lm
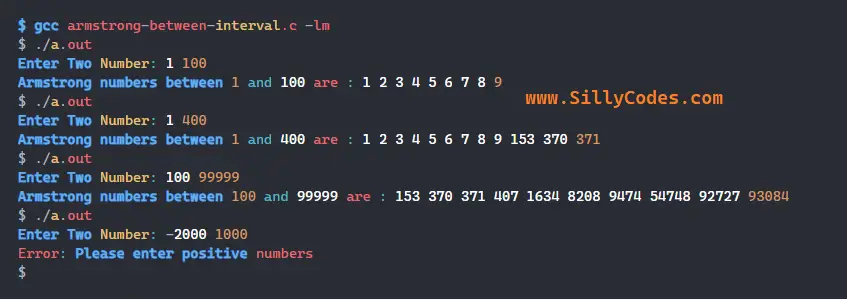
Run the program
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter Two Number: 1 100 Armstrong numbers between 1 and 100 are : 1 2 3 4 5 6 7 8 9 $ ./a.out Enter Two Number: 1 400 Armstrong numbers between 1 and 400 are : 1 2 3 4 5 6 7 8 9 153 370 371 $ ./a.out Enter Two Number: 100 99999 Armstrong numbers between 100 and 99999 are : 153 370 371 407 1634 8208 9474 54748 92727 93084 $ ./a.out Enter Two Number: -2000 1000 Error: Please enter positive numbers $ |
Related Programs:
- Collection of C Loops Programs [ List of Loop Practice Programs ]
- C Program to Check number is Prime Number or not?
- C Program to Check Prime Number using Square Root (Sqrt) Method
- C Program to Print Prime Numbers between Two numbers
- C Program to print First N Prime numbers
- C Program to generate Prime numbers up to N
- C Program to Calculate Nth Prime Number
- C Program Check Prime Number [Mutliple Methods]
- C Program to Convert Decimal Number to Binary Number
- C Program to Convert Binary Number to Decimal Number
- C Program to Convert Octal Number to Decimal Number
4 Responses
[…] C Program to Generate Armstrong Numbers between two Intervals […]
[…] C Program to Generate Armstrong Numbers between two Intervals […]
[…] C Program to Generate Armstrong Numbers between two Intervals […]
[…] C Program to Generate Armstrong Numbers between two Intervals […]