Swap Two Numbers using Pointers in C Language
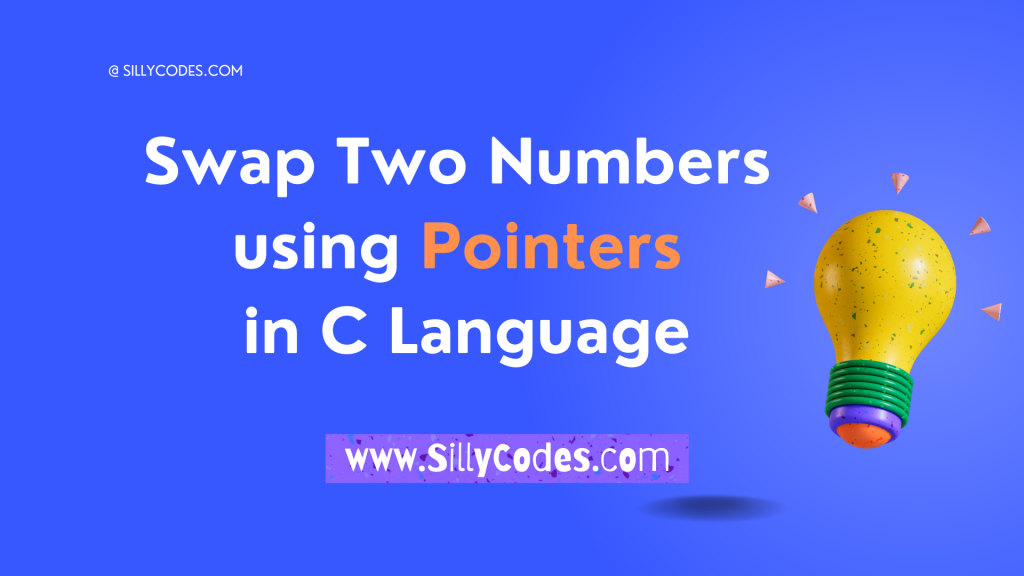
Program Description:
Write a Program to Swap Two Numbers using Pointers in C programming language. The program will accept two integer numbers from the user and swaps the values of the given number using the pointers.
📢 This Program is part of the Pointers Practice Programs series
let’s look at the example input and output of the program.
Example Input and Output:
Input:
Enter the first number(number1): 6666
Enter the second number(number2): 9999
Output:
Numbers before Swapping - number1:6666, number2:9999
Numbers after Swapping - number1:9999, number2:6666
If you notice, The values of the number1 and number2 are swapped.
Prerequisites:
It is recommended to go through the following pointers and functions tutorials to understand the program better.
- Pointers in C Programming Language – Declare, Initialize, and use pointers
- Functions in C Language with Example Programs
- Operators in C Language
Swap Two Numbers using Pointers in C Program Explanation:
Let’s look at the step-by-step explanation of Swapping two numbers using pointers in C programming language.
- Start the program by declaring two integer variables, Let’s say they are number1 and number2.
- Prompt the user to provide the two numbers and update the input in the number1 and number2 variables respectively. Use the scanf() function to read the data from the user.
- Define a function called
swap(). The
swap() function will take two integer pointers as formal arguments. let’s say they are
*number1 and
*number2. This function swaps the values pointed by the
number1 and
number2.
- Prototype of the swap() function – void swap(int *number1, int *number2)
- We use the temporary variable to swap the numbers. If you want to swap numbers without using a temporary variable. Please check this article – Swap two integers without using third variable
- Get the value of the first number by dereferencing the number1 and store it in temp variable – temp = *number1;
- Copy the number2 value to number1 variable. Again use dereference operator or indirection operator on the pointers to get and update the values. – i.e *number1 = *number2;
- Finally, Copay the value of the temp variable to number2 variable. – i.e *number2 = temp;
- From the main() function, Call the swap() function and pass the
number1 and
number2 variables with their addresses. use the address of operator to pass the address of the
number1and
number2.
- swap(&number1, &number2);
- As we are passing the variables as the references, All the changes made in the swap() function will reflect on the main() function.
- Finally, Display results on the console.
Program to Swap Two Numbers using Pointers in C Language:
Let’s convert the above program into the c program. Here is the C program to swap two numbers using pointers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
/* Program to Swap two numbers using pointers sillycodes.com */ #include <stdio.h> /** * @brief - swap two numbers pointed by number1 and number2 * * @param number1 - first number * @param number2 - second number */ void swap(int *number1, int *number2) { // dereference the numbers to get values int temp = *number1; *number1 = *number2; *number2 = temp; } int main() { // declare two variables int number1, number2; // Take two numbers from user printf("Enter the first number(number1): "); scanf("%d", &number1); printf("Enter the second number(number2): "); scanf("%d", &number2); // original numbers printf("Numbers before Swapping - number1:%d, number2:%d\n", number1, number2); // Call the 'swap()' function with two numbers // pass numbers as addresses swap(&number1, &number2); // print results printf("Numbers after Swapping - number1:%d, number2:%d\n", number1, number2); return 0; } |
📢 As we are passing the number1 and number2 with the addresses/references, All the changes made to number1 and number2 will be visible in the main() function. That is the reason the number1 and number2 values are swapped.
Swap Two Numbers using Pointers in C – Program Output:
Let’s compile and run the program using GCC compiler (Any compiler).
Test 1:
1 2 3 4 5 6 7 |
$ gcc swap-pointers.c $ ./a.out Enter the first number(number1): 10 Enter the second number(number2): 20 Numbers before Swapping - number1:10, number2:20 Numbers after Swapping - number1:20, number2:10 $ |
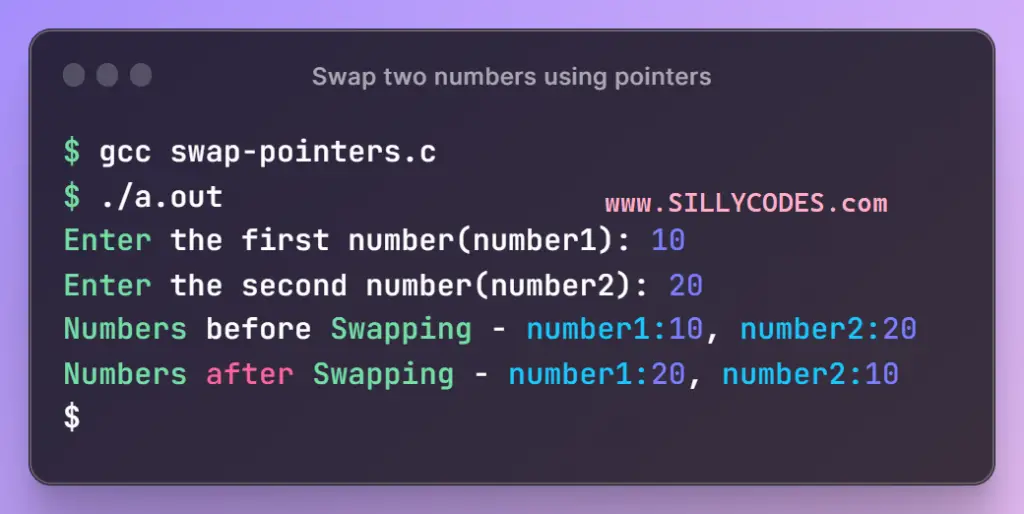
In the above example, The input numbers are 10 and 20. The program swapped the numbers and printed the result on the console.
Test 2:
1 2 3 4 5 6 7 |
$ gcc swap-pointers.c $ ./a.out Enter the first number(number1): -399 Enter the second number(number2): -9000 Numbers before Swapping - number1:-399, number2:-9000 Numbers after Swapping - number1:-9000, number2:-399 $ |
In this example, The number1 is -399 and number2 is -9000. After swapping the number1 value is -9000 and number2 value is -399.
Related Programs:
- C Tutorials Index
- C Programs Index – 300+ Programs
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
- C Program to Count Frequencies of each character in a string
- C Program to Find Highest Frequency character in a String
- C Program to Find Lowest Frequency Character in a String
- C Program to Remove Vowels from a String
- C Program to Remove Consonants from a String
- C Program to Sort String in Ascending order
- C Program to Sort String in Descending Order
1 Response
[…] Swap Two Numbers using Pointers in C Language […]