realloc function in C language – Reallocate / Resize Dynamic memory
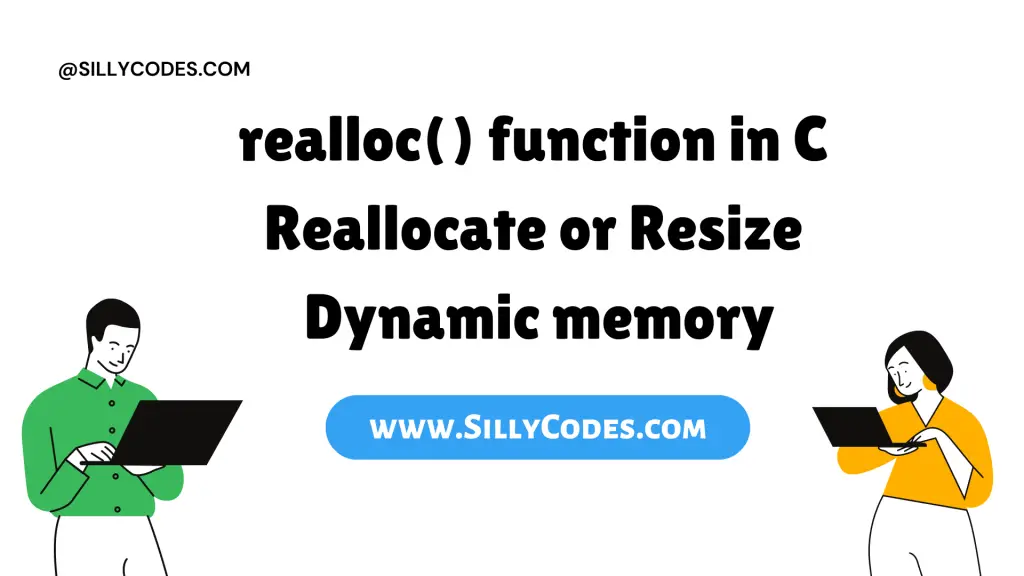
Introduction:
In earlier posts, we looked at static vs dynamic memory allocation, Memory allocation using the malloc() function and calloc() function. In today’s article, We will look at the Reallocation of dynamically allocated memory using the realloc function in c programming language.
Prerequisites:
Please go through the following pointers and functions programs to better understand this tutorial.
The realloc() function in C – Re-allocate Dynamically allocated memory:
As previously mentioned, The malloc() and calloc() functions are used to allocate memory, but what if you wish to modify the size of the dynamically allocated memory? This is where we use the realloc() function in the c language.
The realloc() function is used to reallocate the memory allocated by the malloc() or calloc() functions. We can decrease or increase the size of the dynamically allocated memory using the realloc() function in c. The realloc() function resizes the memory block while retaining the previous data.
Syntax of realloc() function in C language:
1 |
void *realloc(void *ptr, size_t size); |
Here
- ptr – Pointer to dynamically allocated memory( malloc() or calloc() allocated memory).
- size – New size of the memory block. It can see less than or greater than the previous size.
- void * – The realloc() function also returns the void pointer.
The realloc() function returns the pointer to the newly allocated memory block on successful reallocation.
If the realloc() fails, It will return the NULL.
Example usage of realloc() function in C Programming:
Let’s say we want to dynamically allocate memory for 5 integer numbers.
1 2 3 |
int rPtr; Â rPtr = (int *) malloc ( 5 * sizeof(int) ); |
Here we used the malloc() function to allocate the memory for 5 integer variables. The rPtr pointer variable is storing the address of the first memory byte.
Now, our requirement is changed and we need to increase the dynamically allocated memory to store the 10 integers variables.
We can use the realloc() function to resize the dynamically allocated memory.
1 |
rPtr = (int *) realloc( rPtr, 10 * sizeof(int) ); |
We passed the new size 10 * sizeof(int) to the realloc() function along with the pointer variable rPtr
The realloc() function resizes the memory and returns a pointer to the newly allocated memory block, The returned pointer is a void pointer, So we need to typecast it to the appropriate pointer type.
Quick Notes on realloc() function in C Language:
- On successful resize of memory, The realloc() function returns the pointer to the newly allocated memory block. If realloc() function fails, It will return the NULL value.
- The address of the pointer variable rPtr(Returned by the realloc() function) might change if there is not sufficient memory at the previous address. In such cases, The realloc() function moves the data to a new block of memory and returns the pointer to the newly allocated memory block. So we might get a different pointer address.
- The newly allocated memory bytes are uninitialized, So they might contain garbage values.
Let’s look at a program to reallocate dynamically allocated memory using realloc() function in c programming language.
Resizing the dynamically allocated memory using realloc() function in C programming language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 |
/*     Program to resize memory using realloc() function in c programming     sillycodes.com */  #include <stdio.h> #include <stdlib.h>  int main() {     int size, i;      printf("Enter the number of integers you want to store dynamically: ");     scanf("%d", &size);      int *rPtr;      rPtr = (int *) malloc(size * sizeof(int));      // check rPtr     if(rPtr == NULL)     {         printf("ERROR! malloc() - Failed to Allocate Memory\n");         return 0;     }      printf("SUCCESS! Memory allocated successfully\n");     printf("Please provide integers to store\n");      // Take the use input and update the integers     for(i = 0; i<size; i++)     {         printf("Enter an Integer: ");         scanf("%d", rPtr+i);     }      // print the values     printf("Entered Integers are : ");     for(i = 0; i<size; i++)     {         printf("%d ", rPtr[i]);    // we can also use *(rPtr+i)     }     // new line     printf("\n");      // resize     int newSize;     printf("RESIZE: Please provide new size : ");     scanf("%d", &newSize);      // call 'realloc' to resize     rPtr = (int *) realloc( rPtr, newSize * sizeof(int) );      // update the resized array     if(newSize > size)     {         printf("Provide values for remaining integers\n");             for(i = size; i<newSize; i++)         {             printf("Enter an Integer: ");             scanf("%d", rPtr+i);         }     }      // print the resized numbers     // print the values     printf("Entered Integers are : ");     for(i = 0; i<newSize; i++)     {         printf("%d ", rPtr[i]);    // we can also use *(rPtr+i)     }     // new line     printf("\n");      // free the memory before returning.     free(rPtr);      return 0; } |
We started the above program by dynamically allocating memory for a user-provided number of integer variables using the malloc() function. If the malloc() failed to allocate the memory, Then we terminated the program using a return statement. Otherwise, it will continue and prompt the user to provide the values for each integer variable using a for loop and store the values in the allocated memory. We then printed the integer variable on the console using another for loop.
Next, We prompted the user to provide the new size and reallocated the dynamically allocated memory using the realloc() function. To reallocate memory we need to pass the pointer variable and the new size to the realloc() function. like below
1 |
rPtr = (int *) realloc( rPtr, newSize * sizeof(int) ); |
If the new size is greater than the old size. Then we prompted the user to provide the remaining values and displayed them on the screen.
Finally, We freed the allocated memory using the free() function.
Program Output:
Let’s compile and run the program.
Test 1:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
$ gcc realloc.c $ ./a.out Enter the number of integers you want to store dynamically: 5 SUCCESS! Memory allocated successfully Please provide integers to store Enter an Integer: 10 Enter an Integer: 20Â Â Enter an Integer: 30 Enter an Integer: 40 Enter an Integer: 50 Entered Integers are : 10 20 30 40 50 RESIZE: Please provide new size : 9 Provide values for remaining integers Enter an Integer: 60 Enter an Integer: 70 Enter an Integer: 80 Enter an Integer: 90 Entered Integers are : 10 20 30 40 50 60 70 80 90 $ |
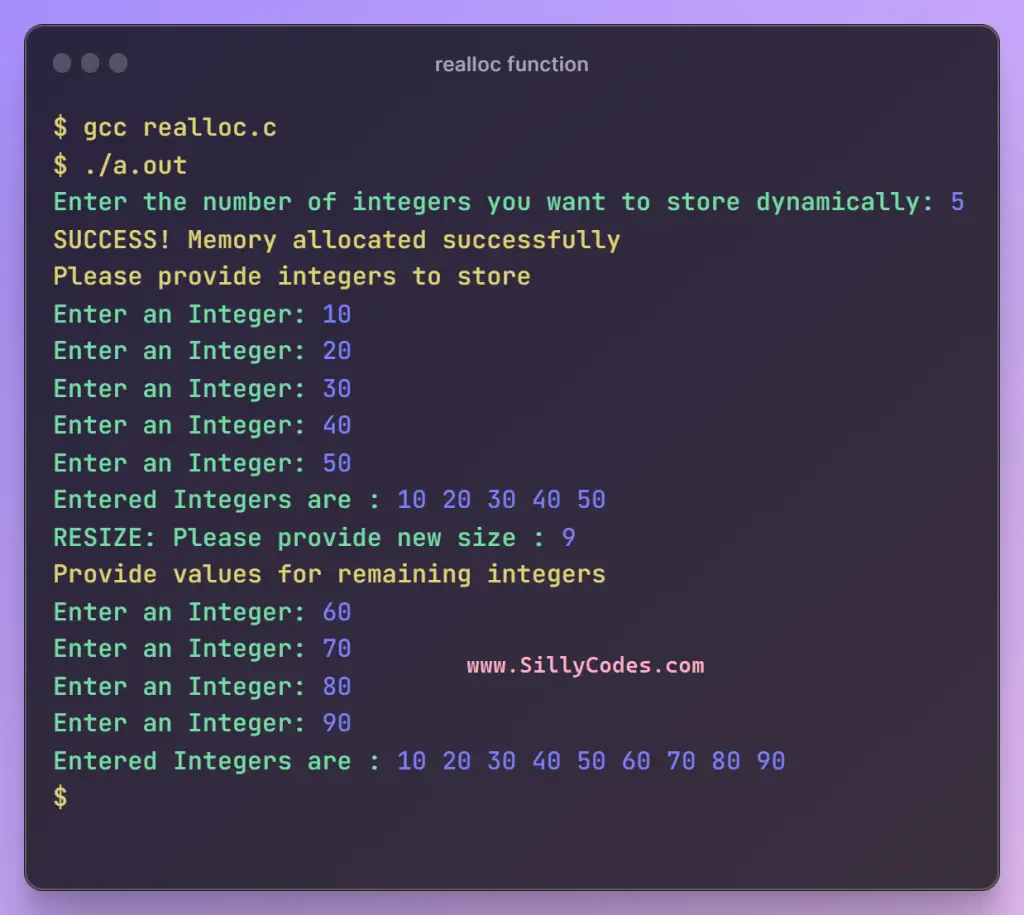
As we can see from the above output, Initially we allocated memory for 5 Integersusing the malloc() function, Then we resized the array to store the 9 Integers using the realloc() function.
Test 2:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
$ ./a.out Enter the number of integers you want to store dynamically: 7 SUCCESS! Memory allocated successfully Please provide integers to store Enter an Integer: 1 Enter an Integer: 2 Enter an Integer: 3 Enter an Integer: 4 Enter an Integer: 5 Enter an Integer: 6 Enter an Integer: 7 Entered Integers are : 1 2 3 4 5 6 7 RESIZE: Please provide new size : 4 Entered Integers are : 1 2 3 4 $ |
In this example, We have decreased the size of the dynamically allocated memory using realloc(). The initial memory stores 7 Integers and resized memory stores only 4 Integers. ( As we reallocated memory using realloc() function, Only the 4 * sizeof(int) memory is allocated to our program.