Print numbers upto n in C (user-provided number) using loops
- Program Description:
- Pre Requisites:
- Print Numbers upto n in C – Program Algorithm:
- Print Numbers up to n in C using the While loop:
- Print Numbers up to in n in C using For loop:
- Print Numbers up to in n in C using do while loop:
- Program Output:
- Pretty Print Output – print numbers upto n in C Language :
- Related Programs:
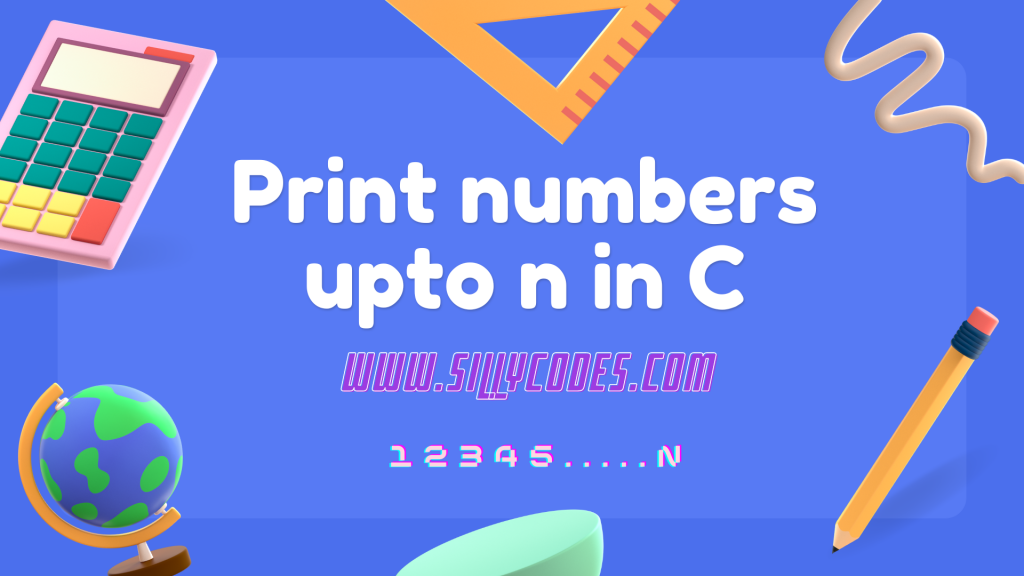
Program Description:
Write a C Program to print numbers upto n in C Language. Here n is the user-provided number. So the program should accept a number from the user and print all the numbers from 1 to the given number n. if the user enters 10, Program should print numbers from 1 to 10. Here is an example of input and output.
Example Input and Output:
Input:
Enter a Positive Number: 10
Output:
1 2 3 4 5 6 7 8 9 10
Pre Requisites:
We will write the program using the while, for, and do while loop. So it is good to have knowledge of the C loops. Please follow the below articles to learn more about the above concepts.
- While Loop in C Language
- For Loop in C Language with Example Programs
- do while loop in Language with example programs
Print Numbers upto n in C – Program Algorithm:
- The Program starts by asking the user for Input, User needs to provide a Positive Number. We are storing the number in a variable num. If the user provides a negative number or zero. We are going to show the error message.
- Once we got the number, Then we will create a loop, which is going to iterate from the number 1 to number num ( user provided number)
- We iterate with the help of a loop control variable or counter. here it is count. which starts from 1 and incremented by 1 at every iteration.
- The loop will stop once the count reaches the num , Here is our loop condition – count <= num.
- At Each Iteration, we print the value of counter and increment the count variable by 1. This way we will be able to print the numbers from 1 to num.
Let’s convert the above logic into the program.
Print Numbers up to n in C using the While loop:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
/*     Program: print numbers up to the user-provided number using while loop */  #include<stdio.h> int main() {     int num;     printf("Enter a Positive Number: ");     scanf("%d", &num);      if(num > 0) {         // Number is zero or positive          // loop control variable         int count = 1;          // star the loop         while(count <= num) {             // print number.             printf("%d ", count);             // don't forgot to increase the counter.             count++;         }      }     else {         printf("Error: Please provide a Valid Number.");     }      printf("\n");     return 0; } |
Program Output:
We are using the GCC compiler to run the program. Learn more about the program compilation on Linux in the following article.
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc print-numbers.c $ ./a.out Enter a Positive Number: 10 1 2 3 4 5 6 7 8 9 10 $ ./a.out Enter a Positive Number: 100 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 $ ./a.out Enter a Positive Number: 50 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 $ |
If the user enters Invalid Input
1 2 3 4 |
$ ./a.out Enter a Positive Number: -10 Error: Please provide a Valid Number. $ |
As you can see from the above output, The program is displaying the error message and suggesting the user to provide the positive number.
Print Numbers up to in n in C using For loop:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
/*     Program: print numbers up to the user-provided number using for loop */  #include<stdio.h> int main() {     int num;     printf("Enter a Positive Number: ");     scanf("%d", &num);      if(num > 0) {         // Number is zero or positive         // loop control variable         int count;          for(count = 1; count <= num; count++) {             // print number.             printf("%d ", count);         }      }     else {         printf("Error: Please provide a Valid Number.");     }      printf("\n");     return 0; } |
Program Output:
Compile and run the program.
$ gcc print-numbers.c
Run the program – Print numbers up to 5
1 2 3 4 |
$ ./a.out Enter a Positive Number: 5 1 2 3 4 5 $ |
Print numbers up to 20
1 2 3 4 |
$ ./a.out Enter a Positive Number: 20 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 $ |
Print Numbers up to in n in C using do while loop:
To print numbers using the do-while loop, The program logic is going to be the same as the above two methods.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
/*     Program: print numbers up to the user-provided number using do while loop */  #include<stdio.h> int main() {     int num;     printf("Enter a Positive Number: ");     scanf("%d", &num);      if(num > 0) {         // Number is zero or positive         // loop control variable         int count = 1;          do {             // print numbers             printf("%d ", count);              // increment the counter.             count++;         } while(count <= num);      }     else {         // number is negative or zero. display error.         printf("Error: Please provide a Valid Number.");     }      printf("\n");     return 0; } |
Program Output:
Here is the do-while program output.
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc print-numbers.c $ ./a.out Enter a Positive Number: 7 1 2 3 4 5 6 7 $ ./a.out Enter a Positive Number: 4 1 2 3 4 $ ./a.out Enter a Positive Number: -82 Error: Please provide a Valid Number. $ |
Pretty Print Output – print numbers upto n in C Language :
We can also introduce a new line after every 10 numbers So that the output loops like a Table. Let’s re-write the above program to print the new line after every 10 numbers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
/*     Program: print numbers up to the user-provided number using do while loop */  #include<stdio.h> int main() {     int num;     printf("Enter a Positive Number: ");     scanf("%d", &num);      if(num > 0) {         // Number is zero or positive         // loop control variable         int count;          for(count = 1; count <= num; count++) {             // print number.             printf("%d ", count);              // see if 'count' is perfectly modulo divisible.             if (count % 10 == 0) {                 // print new line                 printf("\n");             }         }      }     else {         // number is negative or zero. display error.         printf("Error: Please provide a Valid Number.");     }      printf("\n");     return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
$ gcc print-numbers.c $ ./a.out        Enter a Positive Number: 100 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100  $ |
As you can see from the above output, We are printing the new line after every 10 numbers. We accomplished it using the following code
1 2 3 4 5 |
// see if 'count' is perfectly modulo divisible. if (count % 10 == 0) {     // print new line     printf("\n"); } |
We can change the count % 10 as your need to change the table size. If you want to print new line after every 20 numbers, Then perform modulo division with 20, Like this – count % 20 == 0.
Exercise Program:
- Print the numbers up to n in C using the goto statement.
You can refer to Reverse a Number Program in C where we have used a goto statement to create a loop. ( Check the Method 4).
3 Responses
[…] C Program to Print Numbers up to n ( user-provided number) […]
[…] 📢 Related Program: We have already looked at the program to print n natural numbers using Loops. […]
[…] C Program to Print Numbers up to n ( user-provided number) […]