Program to find Factors of a number in C Language
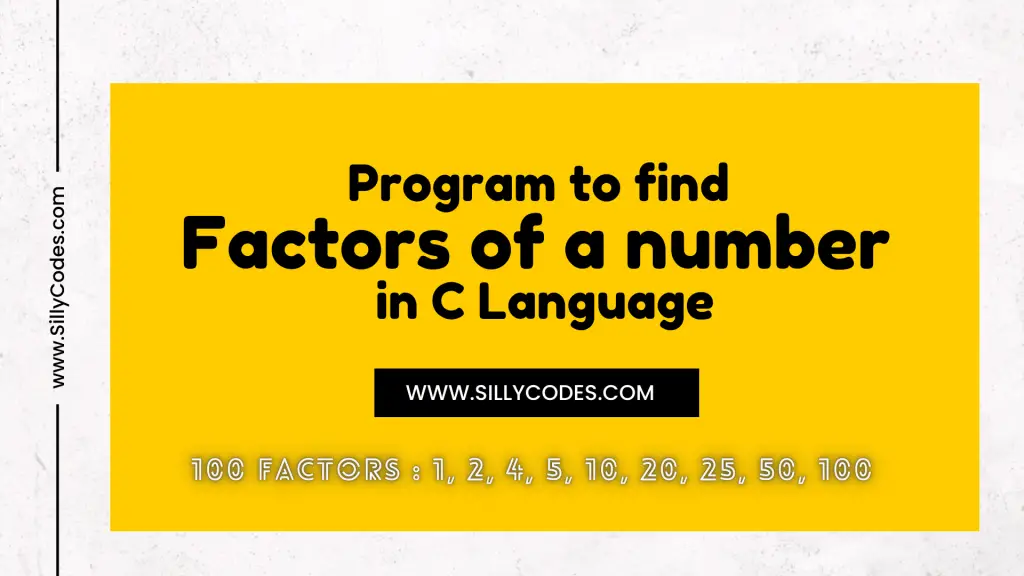
Program Description:
Write a Program to find all Factors of a number in C programming language. The program should accept number from the user and find all Factors of the number. Make sure program should display error on Invalid inputs ( like negative numbers)
What are the Factors of a Number?
The factors of a number are All numbers that evenly divide the given number without any remainder.
Let’s take number 20 , The factors of number 20 are 1, 2, 4, 5, 10, 20. Since 20 is evenly divisible by all the above numbers. So we can say 1, 2, 4, 5, 10, 20 as factors of number 20.
Here is a few Numbers and Their Factors.
Number | Factors |
---|---|
15 | 1, 3, 5, 15 |
40 | 1, 2, 4, 5, 8, 10, 20, 40 |
30 | 1, 2, 3, 5, 6, 10, 15, 30 |
36 | 1, 2, 3, 4, 6, 9, 12, 18, 36 |
Expected Input and Output of the program:
Input:
Enter a Number to calculate factors: 10
Output:
Factors of 10 are : 1 2 5 10
Program Pre-Requisites:
It is recommended to have the knowledge of the C Loops and modulus operator. Please go through following articles to learn more about these topics.
- Modulus Operator in C
- While Loop in C Language
- For Loop in C Language with Example Programs
- do while loop in Language with example programs
Factors of a number in C Program Algorithm:
- Take the input from the user and store it in a variable, Let’s say we are using variable num
- Check if num is positive or negative. If num is negative number, Display an error message and exit the program.
- If the num is positive continue further.
- To be factor of a number num, The factor must evenly divide the num. So all the numbers from 1 to num, which evenly divide the num are the Factors of the give number num.
- Start the loop, The loop goes from
1 to
num. At each iteration
- check if the num is evenly divided by i using Modulus operator – num % i == 0, If this condition is True, Then i is Factor of num, So display the i
- Increment the value of i by 1 and go to next iteration.
- The Step-5 continues until the loop condition i <= num becomes False. And we will get All Factors of the number
Factors of a number in C using while loop:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
/*     Program: Factors of a Number in C     Author: sillyCodes.com */ #include<stdio.h> int main() {     int num, i=1;      // Take the input from the user     printf("Enter a Number to calculate factors: ");     scanf("%d", &num);      if(num <= 0) {         printf("Invalid Input, Please try again \n");         return 0;     }      printf("Factors of %d are : ", num);      // Loop upto 'num'     while(i<=num)     {         // Factor of number is which evenly divide the 'num'         if(num % i == 0)         {             printf("%d ", i);         }          // increment counter 'i'         i++;     }      printf("\n");     return 0; } |
Program Output:
Compile and run the above program. We are using the GCC compiler.
To compile the program using the GCC compiler use the following command.
gcc factors.c
The above command creates the executable file named a.out. So run it using the following command.
./a.out
1 2 3 4 |
$ ./a.out Enter a Number to calculate factors: 10 Factors of 10 are : 1 2 5 10 $ |
Let’s try few more examples.
1 2 3 4 5 6 7 |
$ ./a.out Enter a Number to calculate factors: 50 Factors of 50 are : 1 2 5 10 25 50 $ ./a.out Enter a Number to calculate factors: 1000 Factors of 1000 are : 1 2 4 5 8 10 20 25 40 50 100 125 200 250 500 1000 $ |
What if the user enters a Negative number.
1 2 3 4 |
$ ./a.out Enter a Number to calculate factors: -20 Invalid Input, Please try again $ |
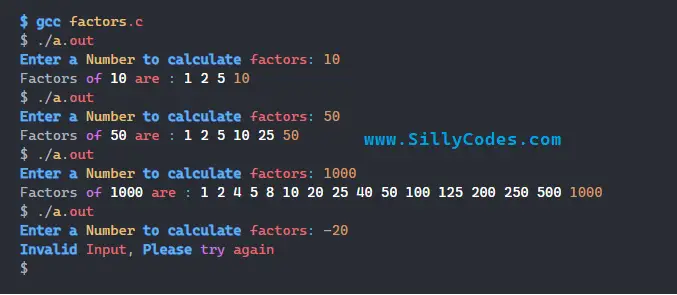
As you can see from the above outputs, The program is giving the All factors of the number and Also doing the error check and displaying the error notice on Invalid input.
Factors of a number in C using for loop and goto statement:
Let’s try to write the above program using the for loop and we also add the functionality to allow the user to provide the input again incase of the invalid input.
To do that, We are going to use the Goto Statement, Which is a Jump statement used to jump from one statement to another statement in the C program.
Please look at the following program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
/*     Program: Factors of a Number in C using for loop     Author: sillyCodes.com */ #include<stdio.h> int main() {     int num, i;     // goto statement label;     INPUT:      // Take the input from the user     printf("Enter a Number to calculate factors: ");     scanf("%d", &num);      if(num <= 0) {         printf("Invalid Input, Please try again \n");         // goto statement         goto INPUT;     }      printf("Factors of %d are : ", num);      // Loop from '1' to 'num'     for(i = 1; i <= num; i++)     {         // Factor of number is which evenly divide the 'num'         if(num % i == 0)         {             printf("%d ", i);         }     }      printf("\n");     return 0; } |
Program Output:
Save the above program to file with the extension .c and compile and run the program.
Positve number test cases.
1 2 3 4 5 6 7 8 |
$ gcc factors.c $ ./a.out Enter a Number to calculate factors: 60 Factors of 60 are : 1 2 3 4 5 6 10 12 15 20 30 60 $ ./a.out Enter a Number to calculate factors: 70 Factors of 70 are : 1 2 5 7 10 14 35 70 $ |
Program is providing the desired output.
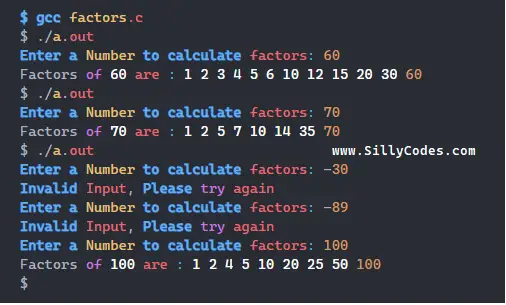
Let’s test the negative number test case.
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Number to calculate factors: -30 Invalid Input, Please try again Enter a Number to calculate factors: -89 Invalid Input, Please try again Enter a Number to calculate factors: 100 Factors of 100 are : 1 2 4 5 10 20 25 50 100 $ |
As you can see from the above output, The program is displaying the error message Invalid Input, Please try again on Negative number (-30). And program also displaying the instruction to provide the number again. So that user can provide new number. The above process continues until user enters a valid number.
Related Programs:
- If else practice Programs
- Switch Case Practise Programs
- C program to test Leap yearÂ
- C program to Find Area and Perimeter of Rectangle.
- Calculate the Grade of Student.
- Type Conversion Operators on Assignment operator.
- Prime Numbers up to n
- Calculate Sum of Digits Program in C
- Octal to Decimal Number Conversion
4 Responses
[…] have already discussed about the Program to find all Factors of a number in earlier […]
[…] C Program to Find all Factors of a Number […]
[…] have looked at the Factors of number program, and GCD Program in earlier posts, In today’s post we are going to write a program to […]
[…] C Program to Find all Factors of a Number […]