Reverse Number Program in C language
- Program Description:
- Reverse Number Program in C Language Explanation:
- Reverse Number Program Algorithm:
- Method 1: Reverse Number program in C using While loop:
- Method 2: Reverse Number program in C using For loop:
- Method 3: Reverse Number program in C using do while loop:
- Method 4: Reverse Number program in C using goto statement:
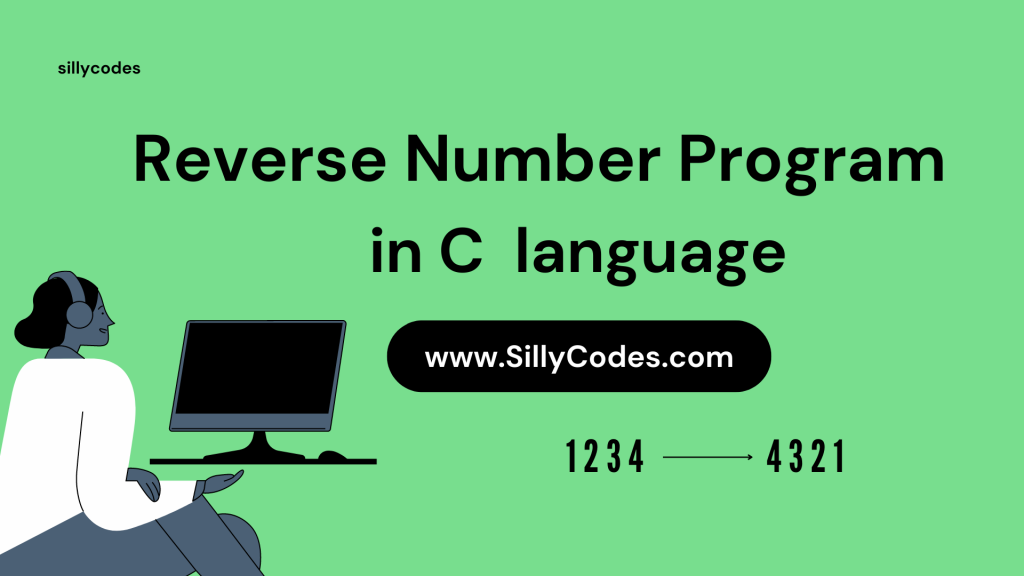
Program Description:
In this article, We are going to look at Reverse Number Program in C programming language. The program should accept a number from the user and display the Reverse of the given number. The program only accepts positive values, If the user enters a negative value, It should display the error message. Here is an example Input and Output of the program.
Example Input and Output:
Example 1:
Input:
Enter a Positive Number : 12345
Output:
Original Number is : 12345
Reversed Number is : 54321
Pre Requisites:
It is good to have familiarity with the Modulus Operator, Division Operator, and Loops (while, for, and do while). Please read the material below to gain the needed understanding.
- Division Operator behavior based on datatypes
- Modulus Operator
- While Loop in C Language
- For Loop in C Language with Example Programs
- do while loop in Language with example programs
Reverse Number Program in C Language Explanation:
We can use any of the C Loops to write this program. So we are going to show the program with all loops.
- Method 1: Reverse Number using while loop
- Method 2: Reverse Number using for loop
- Method 3: Reverse Number using do while loop
- Method 4: Reverse Number using goto statement [Not Recommended – only for demonstration purposes]
Reverse Number Program Algorithm:
- We start the program by asking the user for Input. The user needs to provide the a Positive Number. Once we got the Input we save the input value in the num variable.
- Firstly, we check if the num is positive using the if(num <= 0) condition. If the number is Negative or Zero, We are going to display the error message and stop the program using return statement.
- If the num is Positive Number, We proceed to next steps.
- We use two extra variables, temp and rev_num, The temp variable holds the given num. and we perform all our operations on temp. The rev_num initialized with zero and updated during the program execution and once we finished the program, The rev_num contains the Reversed Number
- Now, It is time to start the loop. The main logic is to loop until the
temp value becomes less than
( Loop Condition
temp > 0). At Each Iteration,
- We are going to take the remainder of the temp using the modulus operator – rem = temp % 10;
- Then updating the rev_num using the rev_num = (rev_num * 10) + rem ;, Which is going to reverse the number.
- Then finally, We are dividing the temp to get to the Quotient which is assigned to temp i.e temp = temp / 10; and used in the next iteration.
- Here is an example Walkthrough, If you’re trying to reverse the number 123,
- Then at the first Iteration,
- The rem will be 3 ( i.e 123 % 10 = 3),
- And rev_num will be ( 0 * 10) + 3 which is 3,
- The temp changed to temp = temp / 10 which is temp = 123/10 i.e temp = 12.
- At the Second Iteration,
- The rem = 12 % 10 which is rem = 2.
- rev_num will be (3 * 10) + 2, which is 32
- The temp will change to temp = 12 / 10, which is equal to temp = 1
- Third Iteration,
- The rem = 1 % 10 which is rem = 1.
- rev_num will be (32 * 10) + 1, which is 321
- The temp will change to temp = 1 / 10, which is equal to temp = 0
- As the temp reached , temp > 0 so We stop the loop. and our Reverse Number is rev_num which is equal to 321.
- Finally, display the results on the console.
📢 This algorithm is going to be the same for all the loops, Only the loop syntax will change.
Method 1: Reverse Number program in C using While loop:
Here is the program using the while loop. We are going to implement the above algorithm and to iterate over the number, we are going to use the while loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/*     Program: C Program to Reverse a Number using while loop */  #include<stdio.h> int main() {     int num,rem,temp,rev_num;     printf("Enter a Positive Number : ");     scanf("%d",&num);      if(num <= 0) {         // Number is Negative Number         // Display error and return.         printf("Please enter Positive Number \n");         return 0;     }      // Number is Positive Number.     temp = num;     rev_num = 0;      // Use while loop to Iterate over the number.     // We are going to go through each digit by using the modulus operator     // and division operator.     while(temp > 0)     {         rem = temp % 10;         rev_num = (rev_num * 10) + rem ;         temp = temp / 10;     }      // Display the results     printf("Original Number is : %d \n", num);     printf("Reversed Number is : %d \n", rev_num);      return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
$ gcc reverse-number.c $ ./a.out Enter a Positive Number : 12345 Original Number is : 12345 Reversed Number is : 54321 $ ./a.out Enter a Positive Number : 987654321 Original Number is : 987654321 Reversed Number is : 123456789 $ $ ./a.out Enter a Positive Number : 5678 Original Number is : 5678 Reversed Number is : 8765 $ |
If the user enter’s Negative Number
1 2 3 4 |
$ ./a.out Enter a Positive Number : -2983 Please enter Positive Number $ |
Method 2: Reverse Number program in C using For loop:
The program logic is very much the same as the while loop, but as it is for loop, we can take advantage of the for loop’s initialization and update options to Init and update our loop control variables.
Here we are going to initialize the temp ( temp = num )at the for loops Init step and update the temp ( temp = temp / 10 ) in for loops update step.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
/*     Program: C Program to Reverse a Number using for loop */  #include<stdio.h> int main() {     int num,rem,temp,rev_num;     printf("Enter a Positive Number : ");     scanf("%d",&num);      if(num <= 0) {         // Number is Negative Number         // Display error and return.         printf("Please enter Positive Number \n");         return 0;     }      // Number is Positive Number.     rev_num = 0;      // Using for loop to calculate the reverse number     // We are going to go through each digit by using the modulus operator     // and division operator to reduce the number.     // Loop runs until temp becomes lessthan zero.      for(temp = num; temp > 0; temp = temp / 10) {         rem = temp % 10;         rev_num = (rev_num * 10) + rem;     }      // Display the results     printf("Original Number is : %d \n", num);     printf("Reversed Number is : %d \n", rev_num);      return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ gcc reverse-number.c $ ./a.out Enter a Positive Number : 6574 Original Number is : 6574 Reversed Number is : 4756 $ ./a.out Enter a Positive Number : 2323Â Â Original Number is : 2323 Reversed Number is : 3232 $ ./a.out Enter a Positive Number : -2093 Please enter Positive Number $ |
As you can see from the above output, We got the desired output and the program is displaying the error message on Invalid inputs.
Method 3: Reverse Number program in C using do while loop:
The Do while loop executes the loop at least once. We are performing division and modulo division inside the do-while loop body.
As we are checking if the number is positive at line 12, which ensures us the num is going to be a valid positive number. And we are also not allowing the zero. So we will be good.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/*     Program: C Program to Reverse a Number using do while loop */  #include<stdio.h> int main() {     int num,rem,temp,rev_num;     printf("Enter a Positive Number : ");     scanf("%d",&num);      if(num <= 0) {         // Number is Negative Number         // Display error and return.         printf("Please enter Positive Number \n");         return 0;     }      // Number is Positive Number.     rev_num = 0;     temp = num;      // Using do while loop to calculate the reverse number     // We are going to go through each digit by using the modulus operator     // and division operator to reduce the number.     // Loop runs until temp becomes lessthan zero.      do {         // as we checked the 'num' for 'zero' at the start(line 12),         // So 'num' should be above zero.         rem = temp % 10;          rev_num = (rev_num * 10) + rem;         temp = temp / 10;     } while(temp > 0);      // Display the results     printf("Original Number is : %d \n", num);     printf("Reversed Number is : %d \n", rev_num);      return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
$ gcc reverse-number.c $ ./a.out Enter a Positive Number : 987654 Original Number is : 987654 Reversed Number is : 456789 $ ./a.out Enter a Positive Number : 1 Original Number is : 1 Reversed Number is : 1 $ ./a.out Enter a Positive Number : 58 Original Number is : 58 Reversed Number is : 85 $ ./a.out Enter a Positive Number : -1 Please enter Positive Number $ ./a.out Enter a Positive Number : 0 Please enter Positive Number $ ./a.out Enter a Positive Number : 123456 Original Number is : 123456 Reversed Number is : 654321 $ |
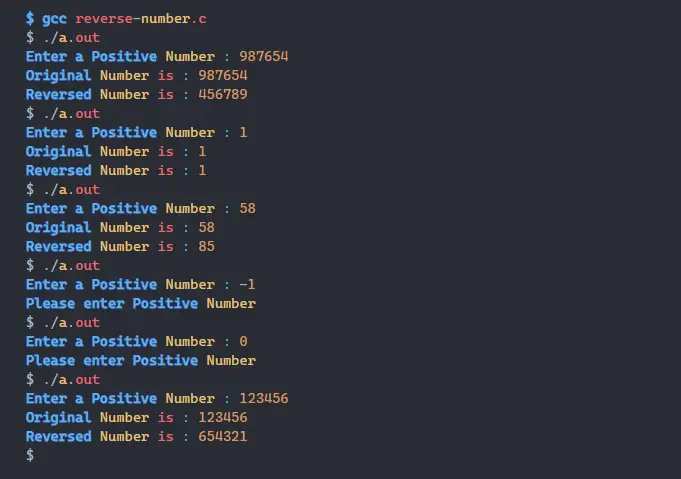
Method 4: Reverse Number program in C using goto statement:
We can also repeat the block of code using the goto statement, So we are going to use the goto statement to iterate over our temp and create the Reverse Number.
📢 It is only for demonstration purposes, It is always better and easy for us to use the Loops to iterate over the block of code. So use the loops instead of the goto statement to iterate over the code block.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
/*     Program: C Program to Reverse a Number using  Goto statement */  #include<stdio.h> int main() {     int num,rem,temp,rev_num;     printf("Enter a Positive Number : ");     scanf("%d",&num);      if(num <= 0) {         // Number is Negative Number         // Display error and return.         printf("Please enter Positive Number \n");         return 0;     }      // Number is Positive Number.     rev_num = 0;     temp = num;      // Using goto statement to calculate the reverse number     // We are going to go through each digit by using the modulus operator     // and division operator to reduce the number.      // We are creating loop using goto statement here.     // This will continue until the 'temp' becomes less than 0.     LOOP:         // as we checked the 'num' for 'zero' at the start(line 12),         // So 'num' should be above zero.         rem = temp % 10;          rev_num = (rev_num * 10) + rem;         temp = temp / 10;     if (temp > 0)         goto LOOP;      // Display the results     printf("Original Number is : %d \n", num);     printf("Reversed Number is : %d \n", rev_num);      return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
$ gcc reverse-number.c $ ./a.out Enter a Positive Number : 1234567 Original Number is : 1234567 Reversed Number is : 7654321 $ ./a.out Enter a Positive Number : 0 Please enter Positive Number $ ./a.out Enter a Positive Number : 1 Original Number is : 1 Reversed Number is : 1 $ ./a.out Enter a Positive Number : 9876 Original Number is : 9876 Reversed Number is : 6789 $ $ ./a.out Enter a Positive Number : 12300 Original Number is : 12300 Reversed Number is : 321 $ |
Related Programs:
- C program to calculate Sum of first N Natural Numbers.
- Sum of Digits of Given Number.
- Calculate area of Circle.
- C Program to calculate area and perimeter of Rectangle.
- C Program to calculate area of Traingle.
- C program to generate Prime Numbers between n1 and n2.
- C Program to Generate Fibonacci series using Recursion.
- More Mathematical C Programs.
- 200+ C Language programs.
4 Responses
[…] C Program to Reverse a Number […]
[…] C Program to Reverse a Number […]
[…] C Program to Reverse a Number […]
[…] accept an input number from the user and reverses it using recursion. We have already looked at the Iterative method to reverse a number in our earlier […]