Swap Without Third Variable or Temporary Variable
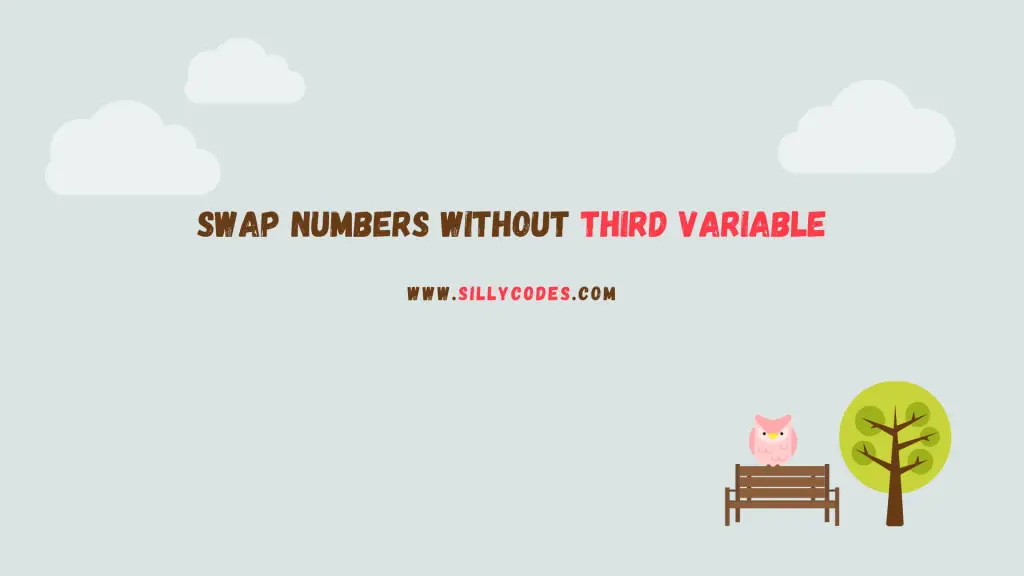
Program Description:
Write a C program to swap two numbers without using any third or temporary variable. We are not allowed to use the extra variable. So lets write a program to swap without third variable.
Example 1:
Input:
Enter two numbers.
1 |
444 333 |
Output:
Output after swapping the numbers
1 |
333 444 |
Swap Two Numbers without using Temporary or Extra Variable:
In this method, We are going to use a small math trick to swap the numbers. Here is the algorithm.
1 |
b = (a*b)/(a=b); |
Algorithm to Swap without Third variable:
- Let’s take two variables 'a'and 'b' and We are going to swap these two variables.
- First of all, We are going to multiply both variables 'a'and variable 'b'.
- And The result would be 'ab'. We will use this product as the Numerator for our division.
- Then we are going to use (a=b) Operation. This means we are copying the value of ‘b’ into the variable ‘a’.
- Now the variable ‘a’ have the desired value of ‘b’.
- Then in the denominator, We got the final value as ‘b’.
- Now we will divide our numerator ‘ab’ with the denominator ‘b’, The result would be the value of ‘a’
- Then we are assigning this result value i.e ‘a’ to variable ‘b’. Now the variable ‘b’ have the desired value of the variable 'a'
- Finally, Two numbers are swapped without using any extra variable.
So by using above algorithm, We swapped the two integers without using temporary variable. And it is a very useful and elegant method to swap numbers.
There are other ways to swap the numbers, We have covered all those methods in the following article. Program to Swap Two Numbers | Swapping Program in C language – SillyCodes
Let’s write the code for the above logic.
Program: Swap two numbers without third variable:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
#include<stdio.h> int main() { // Take Two variable, Two variable 'a' and 'b' for input int a, b; printf("Enter Two Numbers : "); scanf("%d%d", &a, &b); // Let's print the values of 'a' and 'b' before swapping printf("Before Swapping :: Values of a : %d \t b : %d \n", a, b); // We are going to use small math trick to swap numbers. // Here in the Numerator we are multiplying variable 'a' and variable 'b', // So it will become 'ab' // then we are dividing it by (a = b ) means b will be copied to a, // then Numerator ab will be divided by b, // After dividing ab with b, we get a. // Then we are assigning a to b. // So at the end a have the value of b and b have the value of a b = (a*b)/(a=b); // Now the numbers are swapped. // Let's print the values of 'a' and 'b' After swapping printf("After Swapping :: Values of a : %d \t b : %d \n", a, b); return 0; } |
Swap without third variable Program Output:
We are using GCC compiler to compile and run the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// We are Compiling the program using the GCC compiler venkey$ gcc swap2.c // Executable file generated and it's name is 'a.out'. // Let's Run it venkey$ ./a.out Enter Two Numbers : 44 33 Before Swapping :: Values of a : 44 b : 33 After Swapping :: Values of a : 33 b : 44 // Trial 2 venkey$ venkey$ ./a.out Enter Two Numbers : 567 908 Before Swapping :: Values of a : 567 b : 908 After Swapping :: Values of a : 908 b : 567 // Trail 3 venkey$ ./a.out Enter Two Numbers : 31 44 Before Swapping :: Values of a : 31 b : 44 After Swapping :: Values of a : 44 b : 31 venkey$ |
Conclusion:
In this article, We have discussed about the swapping the numbers without using the third or extra variable. This method is one way to swap the numbers but there are other methods as well.
3 Responses
[…] our previous articles, We have discussed different ways to swap the two numbers. In this article, We are going to discuss the swap two numbers without using third variable here we […]
[…] We are using a temporary variable temp to swap the elements. We can also use other methods like Swapping without using temporary variables […]
[…] We use the temporary variable to swap the numbers. If you want to swap numbers without using a temporary variable. Please check this article – Swap two integers without using third variable […]