Reverse a number using Recursion in C Language
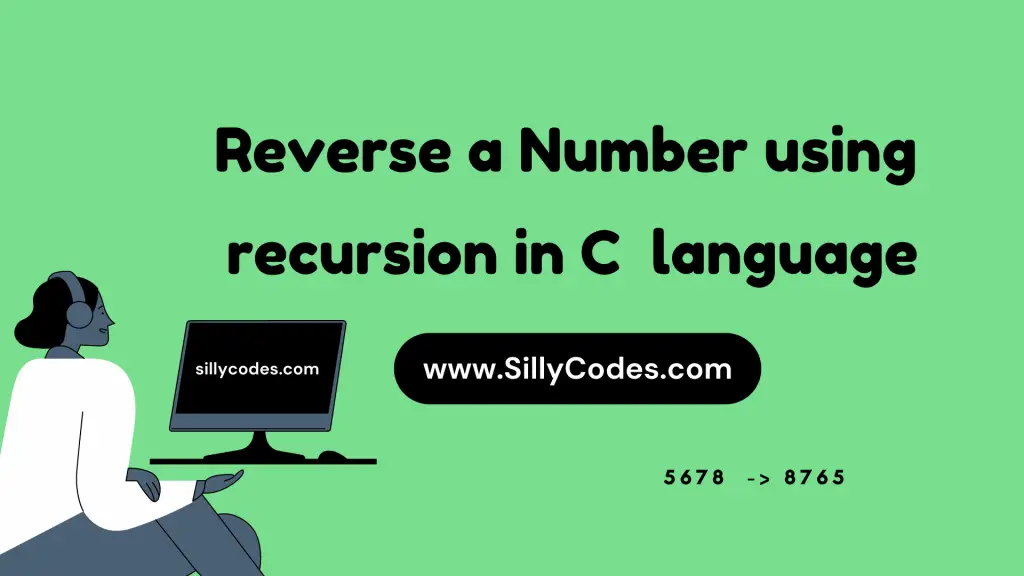
Reverse a number using Recursion in C Program Description:
Write a Program to Reverse a number using Recursion in C Programming Language. The Program will accept an input number from the user and reverses it using recursion. We have already looked at the Iterative method to reverse a number in our earlier articles.
Excepted Program Input and Output:
Input:
Enter a Number to Reverse: 5738
Output:
Reversed Number is : 8375
Pre-Requisites:
We are going to use the functions and recursion in the following program. If you need to refresh your knowledge please go through the following articles, where we covered the above topics.
- Introduction to Functions in C Langauge ( Declare, Define, and Call Functions)
- Recursion in C Language with Step by step call flow
- Types of Functions in C
Reverse a number using Recursion in C Algorithm/Explanation:
- Take the user input and store it in the variable named num.
- We are not going to allow negative numbers, So display the error message if the user enters a negative number. Check if the number is a negative number using num <= 0 condition.
- Define a function named
reverseNumber. The
reverseNumber function, Takes two values as input, they are
num and
reverse number. Pass the input number
num as the first argument and zero as the second argument –
reverseNumber(num, 0);
- The reverseNumber function generates the Reverse value of the provided number using recursion and returns it to the caller.
- The base condition for the recursion is if(num == 0). So the recursion will continue until the num reaches zero.
- At each function call, update the rev_num to create the reverse value – rev_num = (rev_num * 10) + (num % 10);
- Then make a recursive call to reverseNumber and pass the num/10 and rev_num. – reverseNumber(num/10, rev_num);
- Note: We can also use the Static rev_num variable instead of passing it to every call like above. ( We will write the static number method program as well in the next section).
- The recursion will continue until the num reaches the base condition.
- Call the reverseNumber function from the main function. Pass the num and zero(0) as the parameters – reverseNumber(num, 0);. The reverseNumber function returns reversed number, Then Store the return value in reversedNum variable
- Finally, Display the results to the user.
Reverse a number using Recursion in C Program:
Let’s convert the above algorithm into the C code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
/*     Program to Reverse a Number using Recursion in C Language     Author: sillycodes.com */  #include<stdio.h>  /** * @brief Reverse the number 'num' * * @param num * @param rev_num * @return int reversed number */  int reverseNumber(int num, int rev_num) {     // base condition     if(num == 0)     {         return rev_num;     }         // get remender and update 'rev_num'     rev_num = (rev_num * 10) + (num % 10);      // make a recursive call to 'reverseNumber'     return reverseNumber(num/10, rev_num); }   int main() {     // User Input     int num;     printf("Enter a Number to Reverse: ");     scanf("%d", &num);      // Negative number check     if(num <= 0)     {         printf("Error: Please enter a positive Number \n");         return 0;     }      // call the 'reverseNumber' Function     int reversedNum = reverseNumber(num, 0);      // Display the results     printf("Reversed Number is : %d \n", reversedNum);      return 0;  } |
The prototype details of the reverseNumber function.
- int reverseNumber(int num, int rev_num)
- Function_name: reverseNumber
- Arguments_list: (int, int)
- Return_value: int
📢 Here we are passing the rev_num to all recursive calls, We can also use the Static variable for the rev_num parameter so that it will live through the function calls.
Program Output:
Compile and Run the program using your IDE. We are using the GCC compiler here. Let’s try few test cases and see if our program is working fine.
Test Case 1: When the user provides the Positive Numbers:
1 2 3 4 5 6 7 8 |
$ gcc reverse-number-recursive.c $ ./a.out Enter a Number to Reverse: 123456 Reversed Number is : 654321 $ ./a.out Enter a Number to Reverse: 5738 Reversed Number is : 8375 $ |
Test Case 2: Positive Numbers with Zeros:
1 2 3 4 5 6 7 |
$ ./a.out Enter a Number to Reverse: 10023 Reversed Number is : 32001 $ ./a.out Enter a Number to Reverse: 0012002302 Reversed Number is : 20320021 $ |
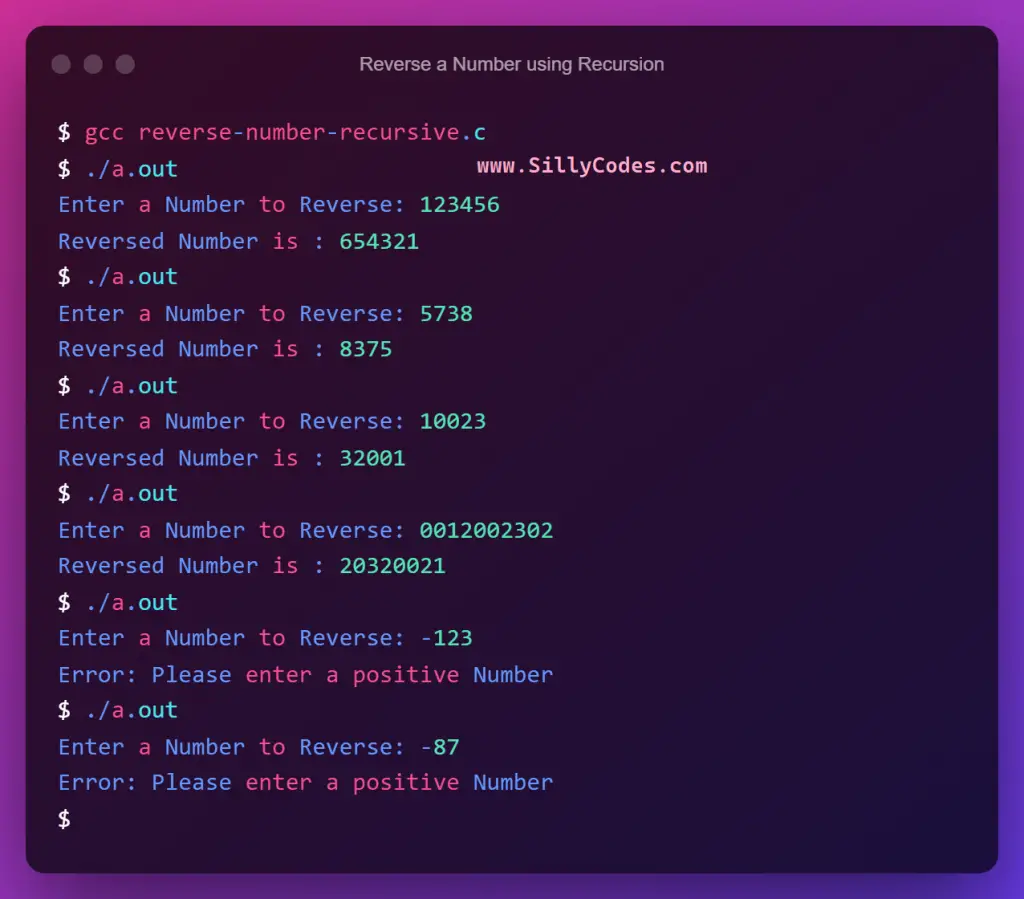
As we can see from the above output, We are getting the appropriate results from the program.
Test Case 3: Negative Numbers:
1 2 3 4 5 6 7 |
$ ./a.out Enter a Number to Reverse: -123 Error: Please enter a positive Number $ ./a.out Enter a Number to Reverse: -87 Error: Please enter a positive Number $ |
The program generates the error message Error: Please enter a positive Number when the negative number is provided by the user.
Method 2: Reverse a number using Recursion in C using Static Variable:
Here is the program to Reverse a number using recursion using a static variable in C programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
/*     Method 2: Program to Reverse a Number using Recursion in C Language     sillycodes.com */  #include<stdio.h>  /** * @brief Reverse the number 'num' * * @param num * @return int reversed number */  int reverseNumber(int num) {     // use static variable for rev_num     static int rev_num = 0;         // base condition     if(num == 0)     {         return rev_num;     }         // get remender and update 'rev_num'     rev_num = (rev_num * 10) + (num % 10);      // make a recursive call to 'reverseNumber'     return (reverseNumber(num/10)); }   int main() {     // User Input     int num;     printf("Enter a Number to Reverse: ");     scanf("%d", &num);      // Negative number check     if(num <= 0)     {         printf("Error: Please enter a positive Number \n");         return 0;     }      // call the 'reverseNumber' Function     int reversedNum = reverseNumber(num);      // Display the results     printf("Reversed Number is : %d \n", reversedNum);      return 0;  } |
The main difference between the above two methods is, Instead of passing the rev_num to reverseNumber function, We are using the static variable.
📢 The static variables will be created at the start of the program and will be destroyed when the program terminates. They will live through the function calls. This means unlike the local variables(auto), The static variables will not be freed once the function terminates.
We will dive deep into the variable Life and Scopes in the Storage Classes in the C language section.
Program Output:
Let’s compile and run the program.
$ gcc reverse-number-recursive-method-2.c
The above command generates the a.out file. Run the executable file.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter a Number to Reverse: 123956 Reversed Number is : 659321 $ ./a.out Enter a Number to Reverse: 987312 Reversed Number is : 213789 $ ./a.out Enter a Number to Reverse: 10098002 Reversed Number is : 20089001Â Â $ ./a.out Enter a Number to Reverse: -854 Error: Please enter a positive Number $ |
The program is giving the desired results.
Recursion Practice Programs:
- C Program to Find Factorial of Number using Recursion
- C Program to Generate Fibonacci Number using Recursion.
- C Program to calculate the sum of Digits of Number using Recursion
- C Program to Print First N Natural Numbers using Recursion
- C Program to find sum of Fist N Natural Number using Recursion
- Product of N natural Number using Recursion C Program
- Program to Count number of Digits in a Number using Recursion
Functions Practice Programs:
- Arithmetic Operations using functions
- Fibonacci Series using function
- Factorial of a Number using functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C
- 100+ C Practice Programs
- C Tutorials Index