Calculate Sum of N Natural Numbers in C using Recursion
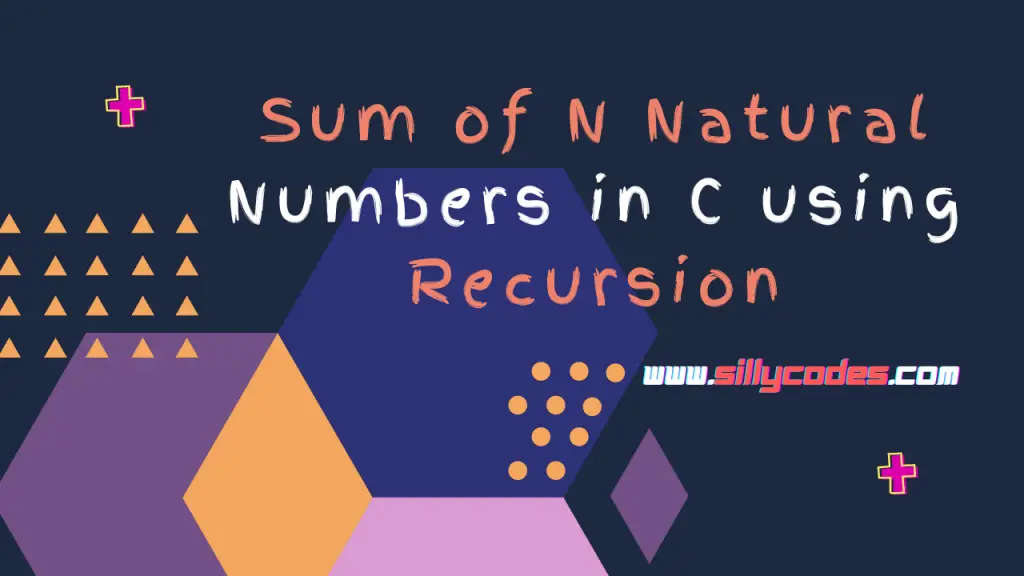
Sum of N Natural Numbers in C using Recursion Program Description:
Write a Program to Calculate the Sum of N Natural Numbers in C using Recursion. The program accepts a number from the user and calculates the sum of the first n natural numbers. We need to use the recursion and should not use the C Loops ( like for, while, and do-while) to calculate the sum.
Example Input and Output:
Input:
Enter a Number: 10
Output:
Sum of first 10 numbers is : 55
📢Related Program: Sum of N Natural Numbers using Loops
What is Recursion:
Function calling itself is called recursion. Any function which calls itself is called a recursive function.
Here is the pseudo-code of recursion.
1 2 3 4 5 6 7 8 |
int func(int num) { Â Â Â Â .... Â Â Â Â .... Â Â Â Â func() Â Â Â Â .... Â Â Â Â .... } |
Pre-Requisites:
Sum of N Natural Numbers in C using Recursion Algorithm / Explanation:
- Take the input from the user and store it in the variable named n.
- Check if the
n is a negative number, If so display an error message (
ERROR! Enter a Valid Number). Ask for the user input again. Take the program control back to the start of the program using the Goto Statement in C
- Use goto INPUT; and INPUT: labels to jump to the start of the program.
- Once we got the positive number, Proceed to the next step.
- Create a function called
sumOfNumbers. The
sumOfNumbers function takes an integer as input and calculates the sum of the first n natural numbers.
- The sumOfNumbers uses recursion to calculate the sum of n numbers and returns it.
- The base condition for the recursion is n == 0. So our recursive calls will stop once the formal argument n reaches the value .
- If the formal argument n value is not equal to zero. Then make a recursive call to the sumOfNumbers function with n-1 as an argument. Also, add the value of the formal argument to the return value of the function call ( n + sumOfNumbers(n-1))
- The above recursive calls continue until we reach the base condition.
- Call the sumOfNumbers function from the main function, Which returns the sum of first n natural numbers. Store the return value in result variable and display the result onto the console using the printf
Sum of N Natural Numbers in C using Recursion Program:
Here is the code for the above algorithm
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
/*     Program to calculate the sum of first N Numbers using recursion in C */ #include <stdio.h>  /** * @brief Calculate sum of first n natural numbers using recursion and return result * * @param n - numbers * @return int - sum of first n numbers */  int sumOfNumbers(int n) {     // base condition     if(n == 0)         return 0;         // recursive function call     return(n + sumOfNumbers(n-1)); }   int main() {     // User Input     INPUT:          // goto label     int n, result = 0;     printf("Enter a Number: ");     scanf("%d", &n);      if(n <= 0)     {         printf("ERROR! Enter a Valid Number \n");         goto INPUT;     }      // call the sumOfNumbers function     result = sumOfNumbers(n);      printf("Sum of first %d numbers is : %d\n", n, result);     return 0; } |
Here are the prototype details of the sumOfNumbers function
- int sumOfNumbers(int);
- function_name: sumOfNumbers
- argument_list: int
- return_value : int
Program output:
Compile and run the program.
Test Case 1: Positive Numbers:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc sum-nNumbers-recursive.c $ ./a.out Enter a Number: 10 Sum of first 10 numbers is : 55 $ ./a.out Enter a Number: 01 Sum of first 1 numbers is : 1 $ ./a.out Enter a Number: 18273 Sum of first 18273 numbers is : 166960401 $ ./a.out Enter a Number: 30 Sum of first 30 numbers is : 465 $ |
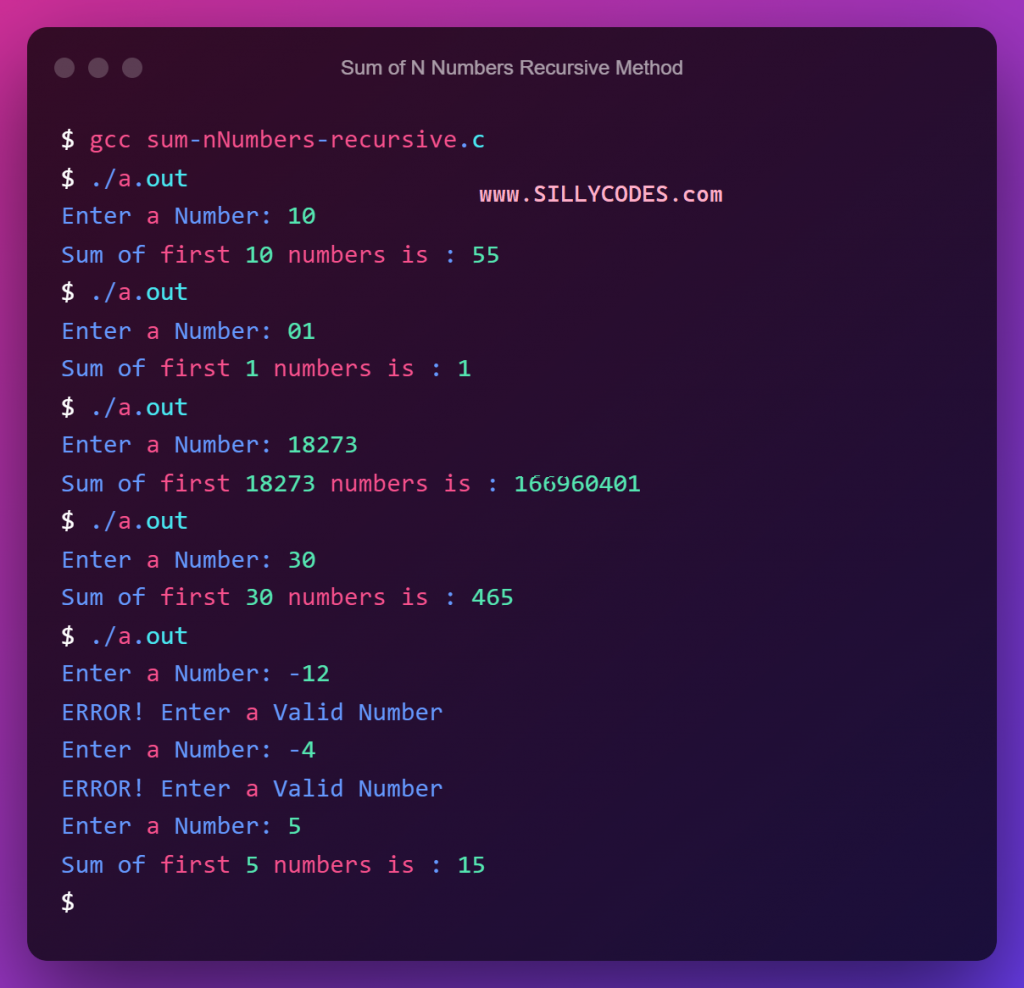
Test Case 2: Negative Numbers:
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Number: -12 ERROR! Enter a Valid Number Enter a Number: -4 ERROR! Enter a Valid Number Enter a Number: 5 Sum of first 5 numbers is : 15 $ |
As you can see from the above output, We are getting the desired results.
3 Responses
[…] Calculate Sum of N Natural Numbers in C using Recursion […]
[…] C Program to find sum of Fist N Natural Number using Recursion […]
[…] C Program to calculate the sum of N Natural Numbers using Recursion […]