Count Number of Unique Elements in Array in C Language
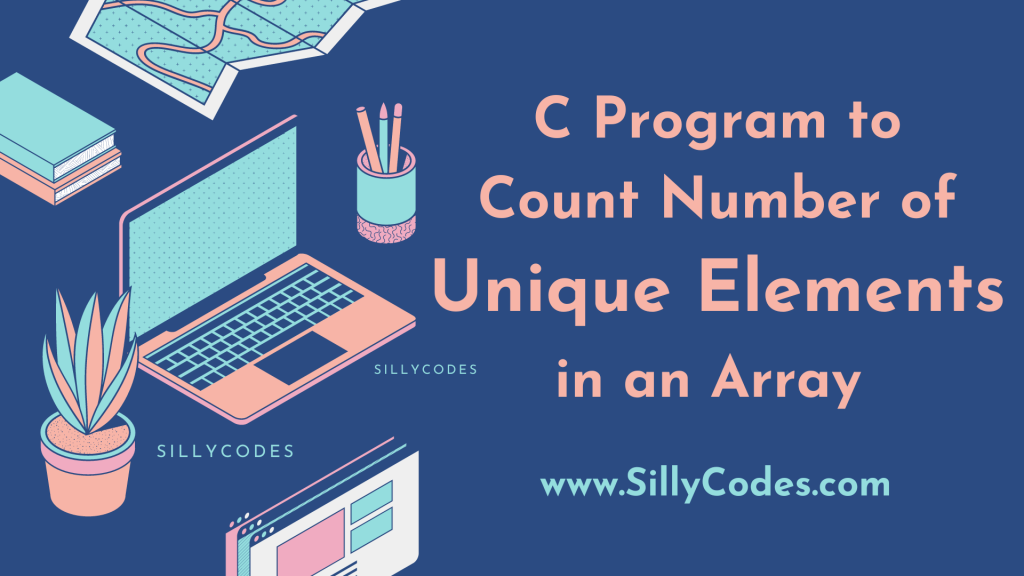
Program Description:
We have looked at the Program to Reverse Array Elements using Recursion in our earlier article, In today’s article, We will look the program to Count the Number of Unique Elements in Array in C programming language. We should not use extra array.
Prerequisites:
We need to know the C Arrays and Functions. Please go through the below posts to learn about them.
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- How to pass arrays to functions in C
- Functions in C Language
Let’s examine what the program’s input and output should look like.
Example Input and Output:
Input:
Enter desired array size(1-1000): 5
Please enter array elements(5) : 1 2 3 3 2
Output:
Original Array: 1 2 3 3 2
Total number of unique elements in array is : 3
From the above output, The input array contains 5 elements but only 3 are unique elements or Distinct elements.
📢 This Program is Part of the Array Practice Programs Series.
Count Number of Unique Elements in Array in C Explanation/Algorithm:
- Start the program by declaring an array, i.e numbers[1000] array. Also, declare other supporting variables.
- Initialize the nDistinct variable with zero(0). This nDistinct will hold the number of unique elements in the array.
- Prompt the user for the desired size of the array and store it in size variable.
- Check for array bounds and display an error message if the size is out of bounds. – if(size >= 1000 || size <= 0)
- Request input from the user for the array’s elements, and update the numbers array. Use a For Loop to iterate over the elements and update them.
- Display the original array on the screen using a For loop.
- Now, To Count the Number of Unique Elements in an Array, We need to iterate over the numbers array and increment the nDistinct variable if there is no duplicate element found in the array. So we need to use two For Loops.
- Create a For Loop (Outer Loop) to iterate over the
numbers array. Start from
i=0 and go till i>size
- Create another for loop (Inner Loop), The inner loop will go from the j=0 to j<i
- At Each Iteration, Check if the numbers[i] == numbers[j]. If this condition is true, Then we found a Duplicate, So break the inner loop.
- Inside of the Outer Loop, Check if the i == j, If it is true, Which means we reached the end of the array but didn’t find the duplicate element for the current element ( numbers[i]). So we can increase the nDistinct variable. So increment the nDistinct variable using Increment Operator.
- Create another for loop (Inner Loop), The inner loop will go from the j=0 to j<i
- Once the above two For Loops are completed, The nDistinct variable contains the total number of Unique elements in the array.
- Display the results on the standard Output using printf function.
Program to Count Number of Unique Elements in Array in C Language:
Let’s convert the above algorithm into the C program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
/* Program to count number of distinct elements in an array Author: SillyCodes.com */ #include <stdio.h> int main() { // declare the 'numbers' array int numbers[1000]; int i, j, size, nDistinct = 0; printf("Enter desired array size(1-1000): "); scanf("%d", &size); // check for array bounds if(size >= 1000 || size <= 0) { printf("Invalid Size, Max Array size reached, Try again \n"); return 0; } // User input for array elements printf("Please enter array elements(%d) : ", size); for(i = 0; i < size; i++) { // printf("numbers[%d] : ", i); scanf("%d", &numbers[i]); } // Print the original array printf("Original Array: "); for(i = 0; i < size; i++) { printf("%d ", numbers[i]); } printf("\n"); // Iterate over the original array 'numbers' // and increment 'nDistinct' if no duplicate is found for(i=0; i<size; i++) { for(j=0; j<i; j++) { if(numbers[i] == numbers[j] ) { // found duplicate break; } } if(i == j) { // we reached end, so not found the duplicate nDistinct++; } } // Print the result printf("Total number of unique elements in array is : %d \n", nDistinct); return 0; } |
Program Output:
Compile and Run the Program.
1 2 3 4 5 6 7 |
$ gcc n-distinct-num-arr.c $ ./a.out Enter desired array size(1-1000): 10 Please enter array elements(10) : 1 2 1 2 1 2 3 4 5 6 Original Array: 1 2 1 2 1 2 3 4 5 6 Total number of unique elements in array is : 6 $ |
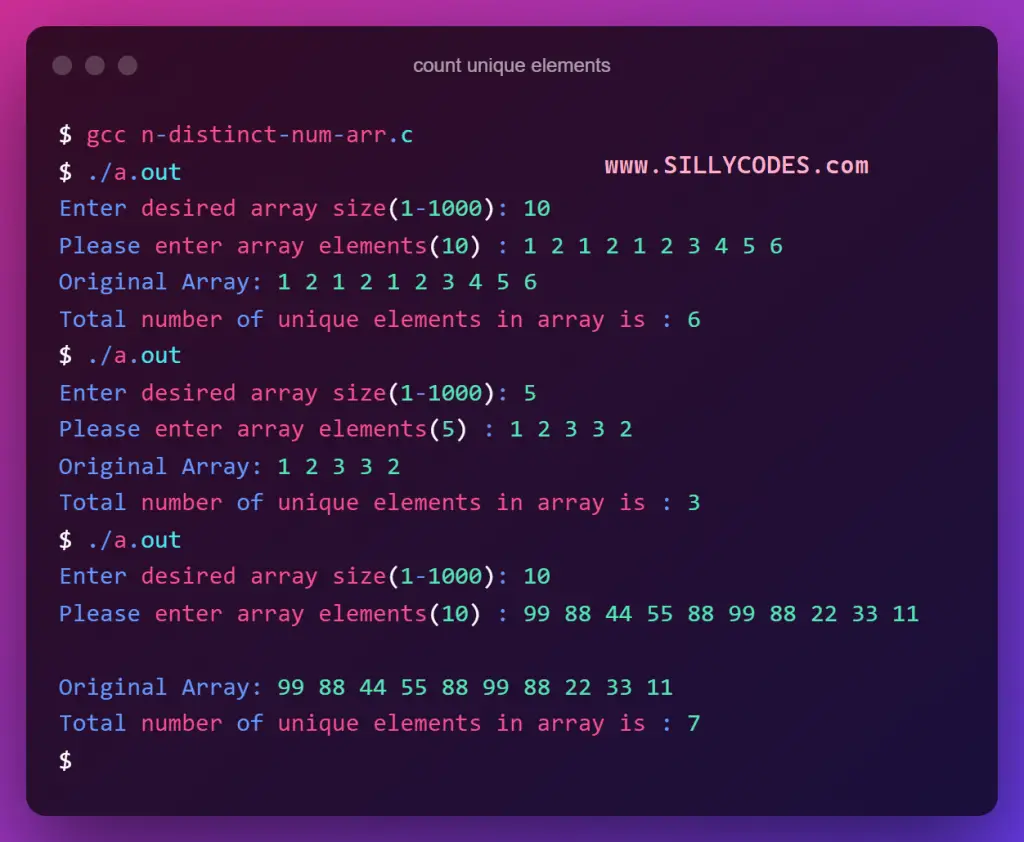
Let’s try few more examples
1 2 3 4 5 6 7 8 9 10 11 |
$ ./a.out Enter desired array size(1-1000): 5 Please enter array elements(5) : 1 2 3 3 2 Original Array: 1 2 3 3 2 Total number of unique elements in array is : 3 $ ./a.out Enter desired array size(1-1000): 10 Please enter array elements(10) : 99 88 44 55 88 99 88 22 33 11 Original Array: 99 88 44 55 88 99 88 22 33 11 Total number of unique elements in array is : 7 $ |
As you can see from the above output, The program is properly generating the count of unique or distinct numbers.
For example, When we entered 99 88 44 55 88 99 88 22 33 11 as the input array, The program generated output as 7, which is the number of unique elements in the array.
Let’s check few corner cases
1 2 3 4 5 6 7 |
$ ./a.out Enter desired array size(1-1000): 0 Invalid Size, Max Array size reached, Try again $ ./a.out Enter desired array size(1-1000): 1099 Invalid Size, Max Array size reached, Try again $ |
From the above output, We can see the program is generating the an error message if the array size is not valid.
Count Number of Unique Elements in Array using functions in C:
It is always good idea to divide the program into the functions, Functions help to increase the debugging, readability and encourages modularity, So let’s modify the above program and divide it in to the few functions, Where each function does a specific task. Then call the defined functions from the main() function.
Here is the modified version of the above program, We have defined three functions(except main() function) in the following program. Look at the main() function and how clean it became.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 |
/* Program to count number of distinct elements in an array Author: SillyCodes.com */ #include <stdio.h> /** * @brief - Read the user input and update the 'numbers' array * * @param numbers - 'numbers' array * @param size - size of the 'numbers' array */ void read(int numbers[], int size) { int i; // User input for array elements printf("Please enter array elements(%d) : ", size); for(i = 0; i < size; i++) { // printf("numbers[%d] : ", i); scanf("%d", &numbers[i]); } } /** * @brief - Display the 'numbers' array * * @param numbers - Array * @param size - Array size */ void display(int numbers[], int size) { int i; for(i = 0; i < size; i++) { printf("%d ", numbers[i]); } printf("\n"); } /** * @brief - Get the number of distinct elements in 'numbers' array * * @param numbers - Array * @param size - size of 'numbers' array * @return int - Returns total number of distinct numbers. */ int countDistinct(int numbers[], int size) { int i, j, nDistinct=0; // Iterate over the original array 'numbers' // and increment 'nDistinct' if no duplicate is found for(i=0; i<size; i++) { for(j=0; j<i; j++) { if(numbers[i] == numbers[j] ) { // found duplicate break; } } if(i == j) { // we reached end, so not found the duplicate nDistinct++; } } return nDistinct; } int main() { // declare the 'numbers' array int numbers[1000]; int size, nDistinct = 0; printf("Enter desired array size(1-1000): "); scanf("%d", &size); // check for array bounds if(size >= 1000 || size <= 0) { printf("Invalid Size, Max Array size reached, Try again \n"); return 0; } // User input for array elements read(numbers, size); // Print the original array printf("Original Array: "); display(numbers, size); // call the 'countnDistinct' to get the number of distinct numbers nDistinct = countDistinct(numbers, size); // Print the result printf("Total number of unique elements in array is : %d \n", nDistinct); return 0; } |
In the above program, We have defined three extra functions, which are
- The
read() function –
void read(int numbers[], int size)
- This function takes two formal arguments. The numbers[] array and the array size
- The read() takes the user input and updates the array elements.
- The
display() function –
void display(int numbers[], int size)
- This function also takes two formal arguments, which are numbers[] array and its size
- The display() function is used to display the array on the standard output (console).
- The
countDistinct() Function –
int countDistinct(int numbers[], int size)
- The countDistinct() function also takes two arguments similar to the read() and display() functions.
- This function goes through the array elements using two for loops and calculates the Distinct Elements in the array.
- It returns the total number of distinct elements back to the caller.
We Called the above functions from the main() function and displayed the results on the console.
Program Output:
Let’s compile and run the program using GCC compiler (Any compiler)
1 2 3 4 5 6 7 8 9 10 11 12 |
$ gcc n-distinct-num-arr-func.c $ ./a.out Enter desired array size(1-1000): 8 Please enter array elements(8) : 1 3 7 2 3 8 19 3 Original Array: 1 3 7 2 3 8 19 3 Total number of unique elements in array is : 6 $ ./a.out Enter desired array size(1-1000): 10 Please enter array elements(10) : 1 1 1 1 1 4 3 3 2 1 Original Array: 1 1 1 1 1 4 3 3 2 1 Total number of unique elements in array is : 4 $ |
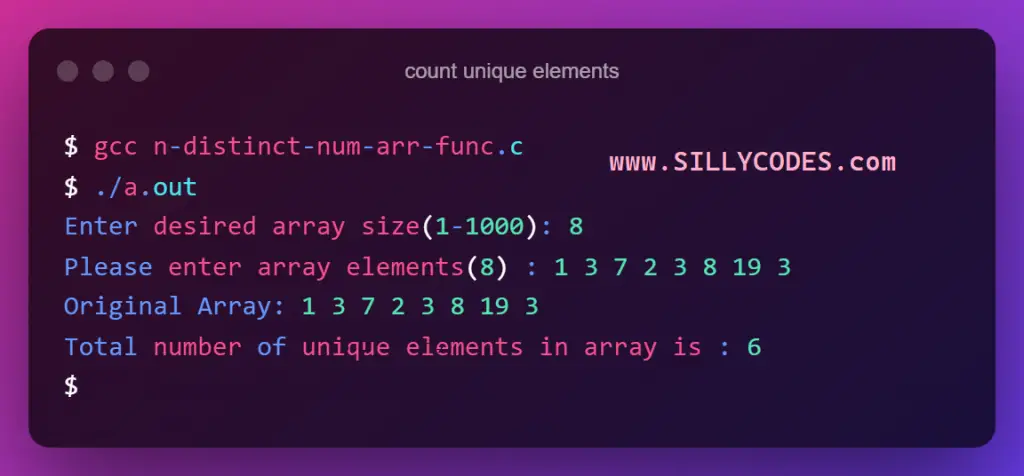
Check negative cases
1 2 3 4 5 6 7 8 9 10 11 12 |
$ ./a.out Enter desired array size(1-1000): 5000 Invalid Size, Max Array size reached, Try again $ ./a.out Enter desired array size(1-1000): 0 Invalid Size, Max Array size reached, Try again $ ./a.out Enter desired array size(1-1000): 5 Please enter array elements(5) : 2 2 2 2 2 Original Array: 2 2 2 2 2 Total number of unique elements in array is : 1 $ |
As we can from the above output, The program is generating the excepted results.
Related Array Programs:
- C Program to find minimum element in Array
- C Program to Find Maximum Element in Array
- C Program to Find Second Largest Element in Array
- C Program to Insert an element in Array
- C Program to Delete an Element in Array
- C Program to Find Unique Elements in an Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Reverse the Array Elements
2 Responses
[…] C Program to Count Number of Unique Elements in Array […]
[…] C Program to Count Number of Unique Elements in Array […]