Fibonacci Series in C using Function
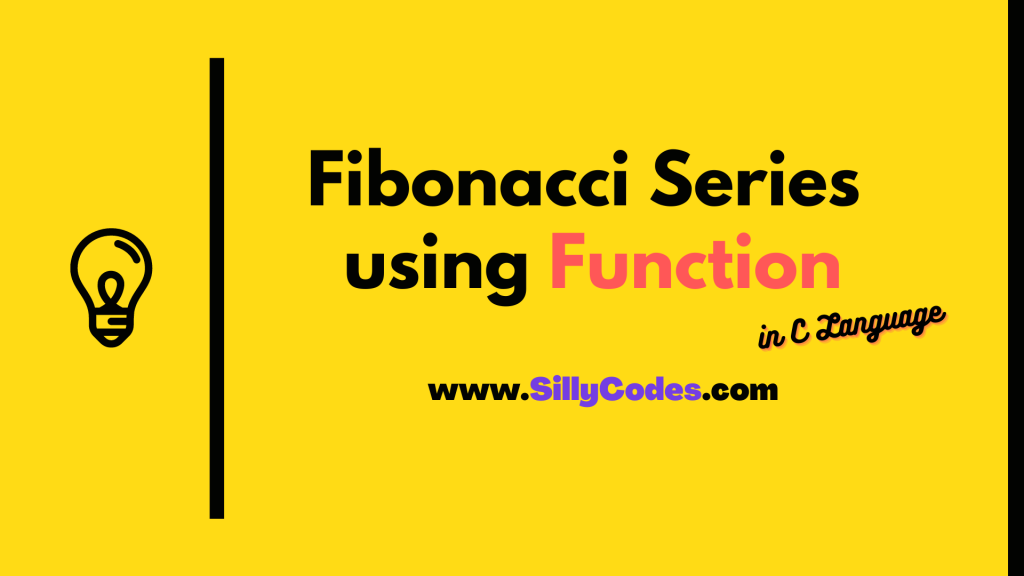
Fibonacci Series in C using Function Program Description:
Write a Program to generate the Fibonacci Series in C using Function. The program should accept an integer from the user and print the Fibonacci series (Fibonacci numbers) up to the given number using a function.
The Fibonacci series is a series of whole numbers in which each number is the sum of the two preceding numbers. Beginning with and 1, the sequence of Fibonacci numbers would be 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, etc.
The formula to calculate Fibonacci Series is n = (n-1) + (n-2).
F{n} = F{n-1} + F{n-2} so F{0} = 0 and F{1} = 1, etc.
where (n-1) means “the last number before n in the series” and (n-2) refers to “the second last one before n in the series.”
Example Input and Output:
Input:
Enter a Number: 1000
Output:
0 1 1 2 3 5 8 13 21 34 55 89 144 233 377 610 987
Related Fibonacci Series Programs:
- C Program to generate Fibonacci Series up to a given number
- C Program to Print First n Fibonacci numbers
- C Program to get Nth Fibonacci number
Pre-Requisites:
Fibonacci Series in C using Function Program Algorithm:
- Take the input from the user and store it in a variable named num
- If the num is a negative number, display an error message and allow the user to try again.
- Once we got the valid num. Call the generateFibonacci function by passing the num to generate the Fibonacci number. The program control jumps to generateFibonaccifunction.
- The
generateFibonaccifunction accepts a number as input (
num) and generates the Fibonacci numbers up to the number
num.
- To generate the Fibonacci series, We use the F{n} = F{n-1} + F{n-2} formula.
- Take three variables a, b, and c. Initialize the first two Fibonacci numbers with and 1. i.e a = 0 and b = 1
- Then generate the third Fibonacci number by adding the previous two numbers in the Fibonacci series. Here we used the variable c to track the present Fibonacci series.
- Repeat the above step using a Loop until we reach the user-provided number num. i.e while(c <= num)
- At Each Iteration:
- Print the present Fibonacci number c
- Then move forward by assigning the b value a ( a = b), c value to b ( b = c)
- Finally, generate the next number in the Fibonacci series by adding a and b ( c = a + b)
- This loop will continue until the Loop condition c <= num becomes False
- Once we reached the num, The loop will stop and the function generateFibonacci will return. ( here we are not returning anything, So the return type of the generateFibonacci function is void). The program control comes back to main() function.
Fibonacci Series in C using Function Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
/* Program to generate Fibonacci series in C using function */ #include<stdio.h> /* generateFibonacci accepts a number and generates fibonacci series upto given number. argument_list : int return_type : void (doesn't return anything) */ void generateFibonacci(int num) { int a, b, c; // initialize first two fibonacci numbers i.e 0, 1 a = 0; b = 1; printf("%d %d ", a, b); // n is equal to (n-1) + (n-2) c = a + b; // check if the 'num' is grether than 'c' while(c <= num) { printf("%d ", c); a = b; b = c; c = a+b; } // new line printf("\n"); } int main() { // Take input from the user int num; INPUT: printf("Enter a Number: "); scanf("%d", &num); // Check for the negative number if(num <= 0) { printf("Error: Invalid Input, Please enter positive number\n"); goto INPUT; } // call generateFibonacci function generateFibonacci(num); return 0; } |
Program Explanation:
In the above Fibonacci series program, We have used a function named generateFibonacci , The generateFibonacci series function expects an integer number ( num) as input and generates the Fibonacci number up to num
The generateFibonacci function doesn’t return anything, So we have specified the return type as the void.
We called the generateFibonacci from the main() function.
As the generateFibonacci function is defined before it is called, So there is no need to specify the function declaration, That is why we haven’t added the function declaration at the top of the program for the generateFibonacci function.
Program Output:
Compile the program using the C compiler, We are using the GCC compiler to compile the program under the ubuntu operating system.
gcc fib-function.c
The above command generates the executable file a.out. Run the program.
Test-Case 1: Enter a positive number and check the results.
1 2 3 4 |
$ ./a.out Enter a Number: 100 0 1 1 2 3 5 8 13 21 34 55 89 $ |
Program generating the Fibonacci series correctly, Let’s try few more examples.
1 2 3 4 5 6 7 |
$ ./a.out Enter a Number: 1000 0 1 1 2 3 5 8 13 21 34 55 89 144 233 377 610 987 ./a.out Enter a Number: 7000 0 1 1 2 3 5 8 13 21 34 55 89 144 233 377 610 987 1597 2584 4181 6765 $ |
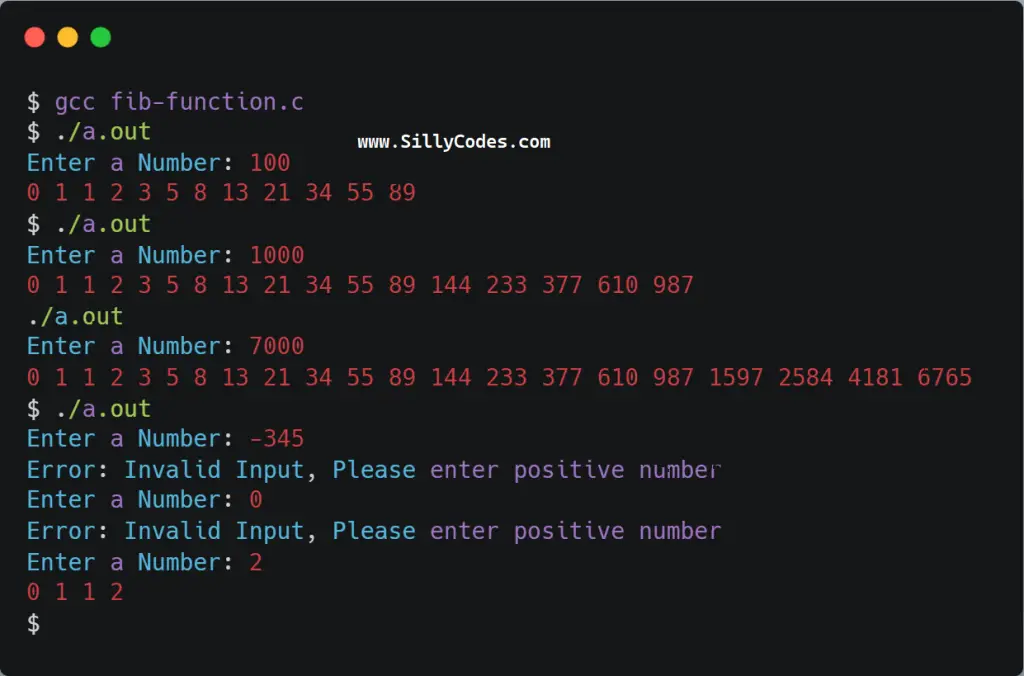
Test Case 2: Enter a Negative number:
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Number: -345 Error: Invalid Input, Please enter positive number Enter a Number: 0 Error: Invalid Input, Please enter positive number Enter a Number: 2 0 1 1 2 $ |
As you can see from the above output, The program is generating an error message ( Error: Invalid Input, Please enter positive number) when the user enters a negative number. And Program is also asking for the user input again. We are using the Goto statement to take the program control back to the INPUT label.
Related C Language Programs:
- C Program to find power of number without Loops
- C Program to Find all Factors of a Number
- C Program to find all Prime Factors of number
- C Program to Calculate the GCD or HCF of Two Number
- C Program to Calculate the LCM of two Numbers
- C Program to Check Palindrome Number
- C Program to Check 3-Digit and N-Digit Armstrong Number
- C Program to Generate Armstrong Numbers upto N ( User-Provided Number)
- C Program to Generate Armstrong Numbers between two Intervals
3 Responses
[…] Fibonacci Series using function […]
[…] Fibonacci Series using function […]
[…] Fibonacci Series using function […]