Area of Triangle Program in C Language
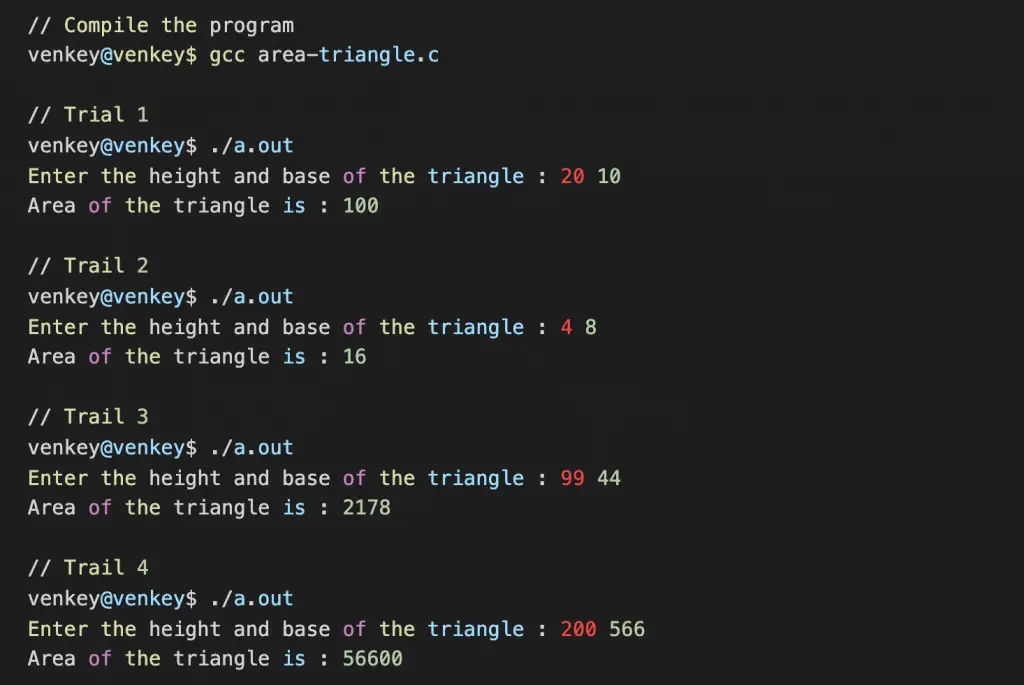
Introduction:
In this article, We are going to look at the Area of Triangle Program in C Language.
Most of us already know, What is a triangle and the formula to calculate the area of the triangle. Here is the area formula.
Area of Triangle Formula:
Area = ½ × b × h
Where b = Base and h = Vertical Height
Area of Triangle Program Algorithm:
- The Program should accept the two integer values from the user and store them as the Height and Base of Triangle.
- Then We are going to apply the above Area formula ( 1/2 * Height * Base ) or ( 0.5 * Height * Base ) on the given numbers.
- Which gives us the Area of the triangle. Display the result onto the console.
Area of Triangle Program in C Language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
/* Write a c program to find the area of any triangle */ #include <stdio.h> int main() { // Create variables to store 'height', 'base' and 'area' int height, base, area; // Take the User input for 'Height' and 'base' and // Store them in 'height' and "base" variables. printf("Enter the height and base of the triangle : "); scanf("%d%d", &height, &base); // Apply the Area of Traignel Formula on given 'height' and 'base' values // The Area formula is '1/2 * height * base' // Which is equal to '0.5 * height * base' // Store the result into the 'area' variable area = 0.5 * height * base; // Print the 'area' onto the console. printf("Area of the triangle is : %d \n", area); return 0; } |
Program Output:
We are going to compile the program using the GCC compiler under the Ubuntu Linux system.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
venkey@venkey$ gcc area-triangle.c // Trail 1 venkey@venkey$ ./a.out Enter the height and base of the triangle : 20 10 Area of the triangle is : 100 // Trail 2 venkey@venkey$ ./a.out Enter the height and base of the triangle : 4 8 Area of the triangle is : 16 // Trail 3 venkey@venkey$ ./a.out Enter the height and base of the triangle : 99 44 Area of the triangle is : 2178 // Trail 4 venkey@venkey$ ./a.out Enter the height and base of the triangle : 200 566 Area of the triangle is : 56600 // Trail 5 venkey@venkey$ ./a.out Enter the height and base of the triangle : 431 788 Area of the triangle is : 169814 // Trail 6 venkey@venkey$ ./a.out Enter the height and base of the triangle : 1 2 Area of the triangle is : 1 // Trail 7 venkey@venkey$ ./a.out Enter the height and base of the triangle : 8 6 Area of the triangle is : 24 venkey@venkey$ |
2 Responses
[…] C Program to Calculate Area of Triangle […]
[…] C Program to Calculate Area of Triangle […]