Print Numbers in Reverse order in C Language
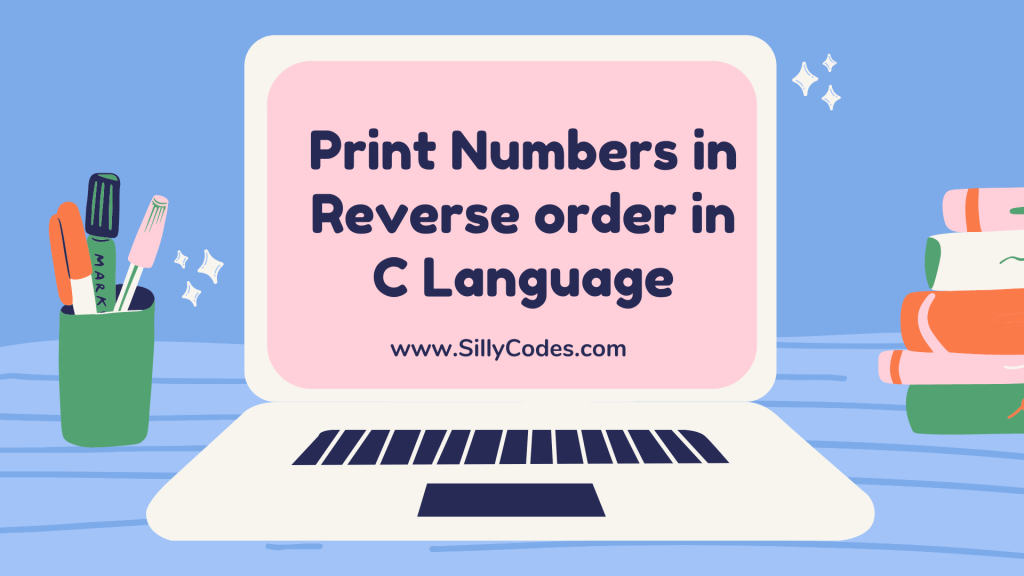
Program Description:
Write a Program to Print Numbers in Reverse Order in C programming language. The program should accept a positive number( num) from the user and display all the numbers from num to 1 in reverse order or Descending order.
Example Input and Output:
Input:
Enter a Number : 31
Output:
Numbers in Reverse Order (31 - 1) : 31, 30, 29, 28, 27, 26, 25, 24, 23, 22, 21, 20, 19, 18, 17, 16, 15, 14, 13, 12, 11, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1,
Pre-Requisites:
We are going to use the C loops and the modulus operators in this program. Please go through the following tutorials to learn more about them
- Modulus Operator
- While Loop in C Language
- For Loop in C Language with Example Programs
- do while loop in Language with example programs
Program to Print Numbers in Reverse order in C Algorithm:
- Take a number from the user and store it in the variable num.
- Make sure num is a positive number, If num is a negative number, Please display an error message and stop the program using the return statement.
- We are going to use a Loop to display the numbers in Reverse Order ( Descending order ).
- Take a Counter variable cnt and make copy the num to cnt. As we need to print from the num to 1.
- Start the loop. The loop will run until the cnt value becomes . while (cnt > 0)
- At Each Iteration,
- Print the counter variable i.e cnt
- Decrement the counter using the Decrement operator i.e cnt--.
- The will terminate, Once the cnt value becomes .
📢 This Program is part of the Series of C Loops Practice Programs
Print Numbers in Reverse order (Descending Order) in C Program using While Loop:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
/* Program: C Program to print numbers in reverse order using while loop */ #include<stdio.h> int main() { int num; printf("Enter a Number : "); scanf("%d", &num); if(num <= 0) { printf("Error: Please Enter a Positive Number\n"); return 0; } printf("Numbers in Reverse Order (%d - 1) : ", num); // Take counter variable to iterate over numbers // 'cnt' starts from 'num' as we are printing in reverse int cnt = num; while(cnt > 0) { // print the number using printf printf("%d ", cnt); // decrement the counter cnt--; } printf("\n"); return 0; } |
Program Output:
Compile and run the program using your favorite compiler, We are using the GCC compiler here.
$ gcc print-reverse-while-loop.c
The above command generates a.out file. Then Run the executable file and provide a number as input.
1 2 3 4 |
$ ./a.out Enter a Number : 31 Numbers in Reverse Order (31 - 1) : 31, 30, 29, 28, 27, 26, 25, 24, 23, 22, 21, 20, 19, 18, 17, 16, 15, 14, 13, 12, 11, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1, $ |
As you can see from the above output, The program is printing the numbers in Descending order or Reverse order.
Example 2: Print Numbers in Reverse order from 100 to 1 and also try 1000 to 1 in descending order.
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Number : 100 Numbers in Reverse Order (100 - 1) : 100, 99, 98, 97, 96, 95, 94, 93, 92, 91, 90, 89, 88, 87, 86, 85, 84, 83, 82, 81, 80, 79, 78, 77, 76, 75, 74, 73, 72, 71, 70, 69, 68, 67, 66, 65, 64, 63, 62, 61, 60, 59, 58, 57, 56, 55, 54, 53, 52, 51, 50, 49, 48, 47, 46, 45, 44, 43, 42, 41, 40, 39, 38, 37, 36, 35, 34, 33, 32, 31, 30, 29, 28, 27, 26, 25, 24, 23, 22, 21, 20, 19, 18, 17, 16, 15, 14, 13, 12, 11, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1, $ $ ./a.out Enter a Number : 1000 Numbers in Reverse Order (1000 - 1) : 1000, 999, 998, 997, 996, 995, 994, 993, 992, 991, 990, 989, 988, 987, 986, 985, 984, 983, 982, 981, 980, 979, 978, 977, 976, 975, 974, 973, 972, 971, 970, 969, 968, 967, 966, 965, 964, 963, 962, 961, 960, 959, 958, 957, 956, 955, 954, 953, 952, 951, 950, 949, 948, 947, 946, 945, 944, 943, 942, 941, 940, 939, 938, 937, 936, 935, 934, 933, 932, 931, 930, 929, 928, 927, 926, 925, 924, 923, 922, 921, 920, 919, 918, 917, 916, 915, 914, 913, 912, 911, 910, 909, 908, 907, 906, 905, 904, 903, 902, 901, 900, 899, 898, 897, 896, 895, 894, 893, 892, 891, 890, 889, 888, 887, 886, 885, 884, 883, 882, 881, 880, 879, 878, 877, 876, 875, 874, 873, 872, 871, 870, 869, 868, 867, 866, 865, 864, 863, 862, 861, 860, 859, 858, 857, 856, 855, 854, 853, 852, 851, 850, 849, 848, 847, 846, 845, 844, 843, 842, 841, 840, 839, 838, 837, 836, 835, 834, 833, 832, 831, 830, 829, 828, 827, 826, 825, 824, 823, 822, 821, 820, 819, 818, 817, 816, 815, 814, 813, 812, 811, 810, 809, 808, 807, 806, 805, 804, 803, 802, 801, 800, 799, 798, 797, 796, 795, 794, 793, 792, 791, 790, 789, 788, 787, 786, 785, 784, 783, 782, 781, 780, 779, 778, 777, 776, 775, 774, 773, 772, 771, 770, 769, 768, 767, 766, 765, 764, 763, 762, 761, 760, 759, 758, 757, 756, 755, 754, 753, 752, 751, 750, 749, 748, 747, 746, 745, 744, 743, 742, 741, 740, 739, 738, 737, 736, 735, 734, 733, 732, 731, 730, 729, 728, 727, 726, 725, 724, 723, 722, 721, 720, 719, 718, 717, 716, 715, 714, 713, 712, 711, 710, 709, 708, 707, 706, 705, 704, 703, 702, 701, 700, 699, 698, 697, 696, 695, 694, 693, 692, 691, 690, 689, 688, 687, 686, 685, 684, 683, 682, 681, 680, 679, 678, 677, 676, 675, 674, 673, 672, 671, 670, 669, 668, 667, 666, 665, 664, 663, 662, 661, 660, 659, 658, 657, 656, 655, 654, 653, 652, 651, 650, 649, 648, 647, 646, 645, 644, 643, 642, 641, 640, 639, 638, 637, 636, 635, 634, 633, 632, 631, 630, 629, 628, 627, 626, 625, 624, 623, 622, 621, 620, 619, 618, 617, 616, 615, 614, 613, 612, 611, 610, 609, 608, 607, 606, 605, 604, 603, 602, 601, 600, 599, 598, 597, 596, 595, 594, 593, 592, 591, 590, 589, 588, 587, 586, 585, 584, 583, 582, 581, 580, 579, 578, 577, 576, 575, 574, 573, 572, 571, 570, 569, 568, 567, 566, 565, 564, 563, 562, 561, 560, 559, 558, 557, 556, 555, 554, 553, 552, 551, 550, 549, 548, 547, 546, 545, 544, 543, 542, 541, 540, 539, 538, 537, 536, 535, 534, 533, 532, 531, 530, 529, 528, 527, 526, 525, 524, 523, 522, 521, 520, 519, 518, 517, 516, 515, 514, 513, 512, 511, 510, 509, 508, 507, 506, 505, 504, 503, 502, 501, 500, 499, 498, 497, 496, 495, 494, 493, 492, 491, 490, 489, 488, 487, 486, 485, 484, 483, 482, 481, 480, 479, 478, 477, 476, 475, 474, 473, 472, 471, 470, 469, 468, 467, 466, 465, 464, 463, 462, 461, 460, 459, 458, 457, 456, 455, 454, 453, 452, 451, 450, 449, 448, 447, 446, 445, 444, 443, 442, 441, 440, 439, 438, 437, 436, 435, 434, 433, 432, 431, 430, 429, 428, 427, 426, 425, 424, 423, 422, 421, 420, 419, 418, 417, 416, 415, 414, 413, 412, 411, 410, 409, 408, 407, 406, 405, 404, 403, 402, 401, 400, 399, 398, 397, 396, 395, 394, 393, 392, 391, 390, 389, 388, 387, 386, 385, 384, 383, 382, 381, 380, 379, 378, 377, 376, 375, 374, 373, 372, 371, 370, 369, 368, 367, 366, 365, 364, 363, 362, 361, 360, 359, 358, 357, 356, 355, 354, 353, 352, 351, 350, 349, 348, 347, 346, 345, 344, 343, 342, 341, 340, 339, 338, 337, 336, 335, 334, 333, 332, 331, 330, 329, 328, 327, 326, 325, 324, 323, 322, 321, 320, 319, 318, 317, 316, 315, 314, 313, 312, 311, 310, 309, 308, 307, 306, 305, 304, 303, 302, 301, 300, 299, 298, 297, 296, 295, 294, 293, 292, 291, 290, 289, 288, 287, 286, 285, 284, 283, 282, 281, 280, 279, 278, 277, 276, 275, 274, 273, 272, 271, 270, 269, 268, 267, 266, 265, 264, 263, 262, 261, 260, 259, 258, 257, 256, 255, 254, 253, 252, 251, 250, 249, 248, 247, 246, 245, 244, 243, 242, 241, 240, 239, 238, 237, 236, 235, 234, 233, 232, 231, 230, 229, 228, 227, 226, 225, 224, 223, 222, 221, 220, 219, 218, 217, 216, 215, 214, 213, 212, 211, 210, 209, 208, 207, 206, 205, 204, 203, 202, 201, 200, 199, 198, 197, 196, 195, 194, 193, 192, 191, 190, 189, 188, 187, 186, 185, 184, 183, 182, 181, 180, 179, 178, 177, 176, 175, 174, 173, 172, 171, 170, 169, 168, 167, 166, 165, 164, 163, 162, 161, 160, 159, 158, 157, 156, 155, 154, 153, 152, 151, 150, 149, 148, 147, 146, 145, 144, 143, 142, 141, 140, 139, 138, 137, 136, 135, 134, 133, 132, 131, 130, 129, 128, 127, 126, 125, 124, 123, 122, 121, 120, 119, 118, 117, 116, 115, 114, 113, 112, 111, 110, 109, 108, 107, 106, 105, 104, 103, 102, 101, 100, 99, 98, 97, 96, 95, 94, 93, 92, 91, 90, 89, 88, 87, 86, 85, 84, 83, 82, 81, 80, 79, 78, 77, 76, 75, 74, 73, 72, 71, 70, 69, 68, 67, 66, 65, 64, 63, 62, 61, 60, 59, 58, 57, 56, 55, 54, 53, 52, 51, 50, 49, 48, 47, 46, 45, 44, 43, 42, 41, 40, 39, 38, 37, 36, 35, 34, 33, 32, 31, 30, 29, 28, 27, 26, 25, 24, 23, 22, 21, 20, 19, 18, 17, 16, 15, 14, 13, 12, 11, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1, $ |
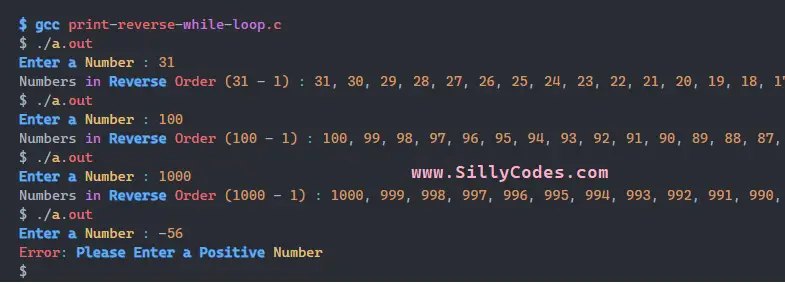
Example 3: Re-Run the program by providing a negative number as input.
1 2 3 4 |
$ ./a.out Enter a Number : -56 Error: Please Enter a Positive Number $ |
Print Numbers in Reverse Order in C language using For loop:
We can use the For loop’s Init step to initialize our cnt=num. and the update step to decrement cnt variable ( cnt--).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
/* Program: C Program to print numbers in reverse order using for loop */ #include<stdio.h> int main() { int num, cnt; printf("Enter a Number : "); scanf("%d", &num); if(num <= 0) { printf("Error: Please Enter a Positive Number\n"); return 0; } printf("Numbers in Reverse Order (%d - 1) : ", num); // 'cnt' starts from 'num' as we are printing in reverse /* Init: cnt with 'num' condition: check if cnt>0 Update: decrement the 'cnt' - cnt-- */ for(cnt = num; cnt > 0; cnt--) { // print the number using printf printf("%d ", cnt); } printf("\n"); return 0; } |
Program Output:
Compile and run the program. Here is the output.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc print-reverse-for-loop.c $ ./a.out Enter a Number : 45 Numbers in Reverse Order (45 - 1) : 45, 44, 43, 42, 41, 40, 39, 38, 37, 36, 35, 34, 33, 32, 31, 30, 29, 28, 27, 26, 25, 24, 23, 22, 21, 20, 19, 18, 17, 16, 15, 14, 13, 12, 11, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1, $ ./a.out Enter a Number : 10 Numbers in Reverse Order (10 - 1) : 10, 9, 8, 7, 6, 5, 4, 3, 2, 1, $ ./a.out Enter a Number : -43 Error: Please Enter a Positive Number $ ./a.out Enter a Number : -2 Error: Please Enter a Positive Number $ |
Exercise: Do while to print the numbers in Reverse order:
As we need to print at least 1 number, We can use the do while also to print the numbers. Try yourself to write this program.
If you need any Hints, Click on the show more button below to see the program.
Program to Print Only Even Numbers from N in Reverse Order in C:
Let’s change the above program to only print the Even numbers in reverse order.
You can check Even or Odd Number Program for more details. We are using the modulus operator ( cnt%2 == 0) to check if the cnt is even at each iteration.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/* Program: C Program to print numbers in reverse order using for loop */ #include<stdio.h> int main() { int num, cnt; printf("Enter a Number : "); scanf("%d", &num); if(num <= 0) { printf("Error: Please Enter a Positive Number\n"); return 0; } printf("Even Numbers in Reverse Order (%d - 1) : ", num); // 'cnt' starts from 'num' as we are printing in reverse /* Init: cnt with 'num' condition: check if cnt>0 Update: decrement the 'cnt' - cnt-- */ for(cnt = num; cnt > 0; cnt--) { // print only Even Numbers if(cnt % 2 == 0) { // Even Number print printf("%d, ", cnt); } } printf("\n"); return 0; } |
Program Output:
Compile and Run the Program.
Example 1: Print All Even numbers from number 100 to 1 in Reverse Order:
1 2 3 4 5 |
$ gcc print-reverse-even-num.c $ ./a.out Enter a Number : 100 Even Numbers in Reverse Order (100 - 1) : 100, 98, 96, 94, 92, 90, 88, 86, 84, 82, 80, 78, 76, 74, 72, 70, 68, 66, 64, 62, 60, 58, 56, 54, 52, 50, 48, 46, 44, 42, 40, 38, 36, 34, 32, 30, 28, 26, 24, 22, 20, 18, 16, 14, 12, 10, 8, 6, 4, 2, $ |
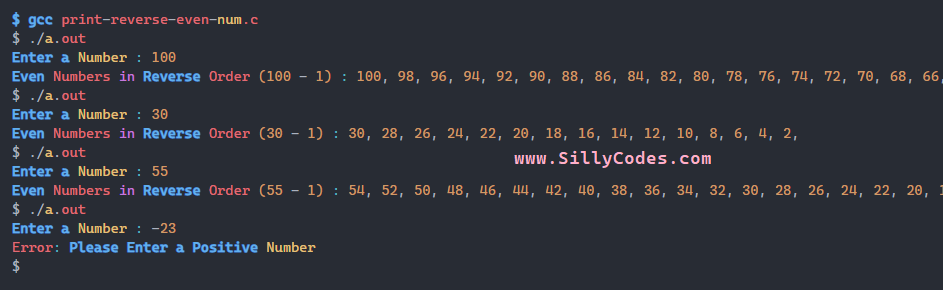
Here are a few more examples:
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter a Number : 30 Even Numbers in Reverse Order (30 - 1) : 30, 28, 26, 24, 22, 20, 18, 16, 14, 12, 10, 8, 6, 4, 2, $ ./a.out Enter a Number : 55 Even Numbers in Reverse Order (55 - 1) : 54, 52, 50, 48, 46, 44, 42, 40, 38, 36, 34, 32, 30, 28, 26, 24, 22, 20, 18, 16, 14, 12, 10, 8, 6, 4, 2, $ ./a.out Enter a Number : -23 Error: Please Enter a Positive Number $ |
Also Note, That program is displaying an error on a negative number.
Program to Print Only Odd Numbers from N in Reverse Order using Loops:
Let’s print all Negative numbers from the N (Given number) up to 1 in Reverse Order using the For loop.
At each iteration, Check if the cnt is an Odd number by using the cnt%2 == 1.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/* Program: C Program to print Odd numbers in reverse order using for loop */ #include<stdio.h> int main() { int num, cnt; printf("Enter a Number : "); scanf("%d", &num); if(num <= 0) { printf("Error: Please Enter a Positive Number\n"); return 0; } printf("Odd Numbers in Reverse Order (%d - 1) : ", num); // 'cnt' starts from 'num' as we are printing in reverse /* Init: cnt with 'num' condition: check if cnt>0 Update: decrement the 'cnt' - cnt-- */ for(cnt = num; cnt > 0; cnt--) { // print only Odd Numbers if(cnt % 2 == 1) { // Odd number printf("%d, ", cnt); } } printf("\n"); return 0; } |
Program Output:
Here is the program output.
Example 1: Print all Odd numbers from 100 to 1 in Reverse Order:
1 2 3 4 5 |
$ gcc print-reverse.c $ ./a.out Enter a Number : 100 Odd Numbers in Reverse Order (100 - 1) : 99, 97, 95, 93, 91, 89, 87, 85, 83, 81, 79, 77, 75, 73, 71, 69, 67, 65, 63, 61, 59, 57, 55, 53, 51, 49, 47, 45, 43, 41, 39, 37, 35, 33, 31, 29, 27, 25, 23, 21, 19, 17, 15, 13, 11, 9, 7, 5, 3, 1, $ |
Let’s try a few more examples
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter a Number : 47 Odd Numbers in Reverse Order (47 - 1) : 47, 45, 43, 41, 39, 37, 35, 33, 31, 29, 27, 25, 23, 21, 19, 17, 15, 13, 11, 9, 7, 5, 3, 1, $ ./a.out Enter a Number : -11 Error: Please Enter a Positive Number $ ./a.out Enter a Number : 9 Odd Numbers in Reverse Order (9 - 1) : 9, 7, 5, 3, 1, $ |
Related Programs:
- C Loops Programs Index
- C Program to Print Prime Numbers between Two numbers
- C Program to print First N Prime numbers
- C Program to generate Prime numbers up to N
- C Program to Calculate Nth Prime Number
- C Program Check Prime Number [Mutliple Methods]
- C Program to Convert Decimal Number to Binary Number
- C Program to Convert Binary Number to Decimal Number
- C Program to Convert Octal Number to Decimal Number
- C Program to Calculate the Factorial of Number
- C Program to Calculate the Power of Number
- C Program to Check Number is Power of 2
- C Program to find power of number without Loops
- C Program to Find all Factors of a Number
3 Responses
[…] C Program to Print Numbers in Reverse Order […]
[…] C Program to Print Numbers in Reverse Order […]
[…] C Program to Print Numbers in Reverse Order […]