Program to Sum of Two Numbers in C using a Function
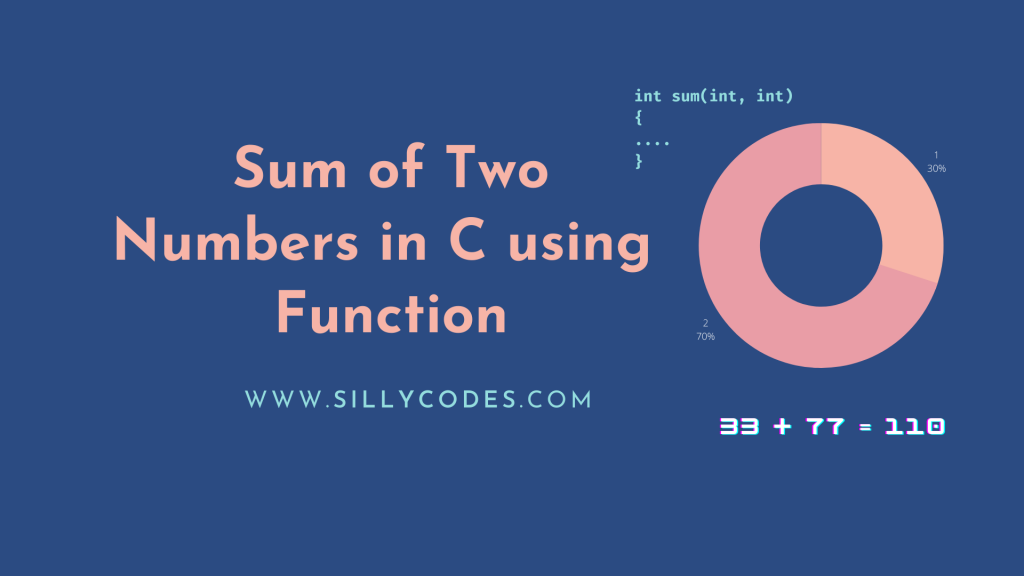
Program Description:
Write a program to Sum of Two Numbers in C using a Function. This program is to understand how functions work in c language.
The program accepts two numbers from the user and calculates the sum of those numbers. The program should create a function, which should accept two numbers as input and return the integer number ( which is the sum of given numbers).
Example Input and Output:
Input:
Enter two numbers : 10 20
Output:
Sum of 10 and 20 is : 30
Pre-Requisites:
- C Functions
- Arithmetic Operators in C Language
Sum of two Numbers in C using Function Program Explanation:
- Accept two numbers from the user as input, And store them in variables number1 and number2 respectively.
- We are going to create a function called
sum.
- The sum function takes two variables as input number1 and number2 and calculate the sum of number1 and number2 and returns the result.
- Here number1 and number2 are formal arguments of the sum function.
- As the sum function is defined after the function call, So we need to declare the function using the function declaration. We have added the sum function declaration at the start of the program using int <strong>sum</strong>(int, int);
- Call the
sum function by passing the
number1 and
number2 from the
main function. As the
sum function returns an integer which is the sum of the given numbers, Assign the function call to an integer variable (i.e
result). So that the return value of the
sum function will be stored in
result variable
- result = <strong>sum</strong>(number1, number2);
- Print the result on the console using printf function.
Sum of two Numbers in C using function Program:
Here is the sum of two numbers program using functions in the C programming language
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/*     C Program to calculate the sum of two numbers using function. */  #include<stdio.h> // 'sum' function declaration. int sum(int, int);  // main() function int main() {      // Take two numbers from the user     int number1, number2, result;     printf("Enter two numbers : ");     scanf("%d%d", &number1, &number2);      // call the function 'sum'     result = sum(number1, number2);      // print the results     printf("Sum of %d and %d is : %d \n", number1, number2, result);      return 0; }  /*     function_name: sum     argument_list: (int, int)     return: int     Description: 'sum' function takes two numbers and calculates the sum and returns it. */  int sum(int number1, int number2) {     // return sum of 'number1' and 'number2'     return number1 + number2; } |
The sum function prototype details.
function_name: sum
argument_list: (int, int)
return_type: int
Sum of two Numbers in C Program Output:
We are using the GCC compiler to compile and run the program.
📢 Learn More – Compiling and running C Program using GCC on Linux Operating System
Run the program and provide two integer values.
1 2 3 4 5 |
$ gcc add-numbers-func.c $ ./a.out Enter two numbers : 55 99 Sum of 55 and 99 is : 154 $ |
Let’s try a few more examples
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter two numbers : 100 200 Sum of 100 and 200 is : 300 $ ./a.out Enter two numbers : 100 -50 Sum of 100 and -50 is : 50 $ ./a.out Enter two numbers : -124 -300 Sum of -124 and -300 is : -424 $ ./a.out Enter two numbers : 10 20 Sum of 10 and 20 is : 30 $ |
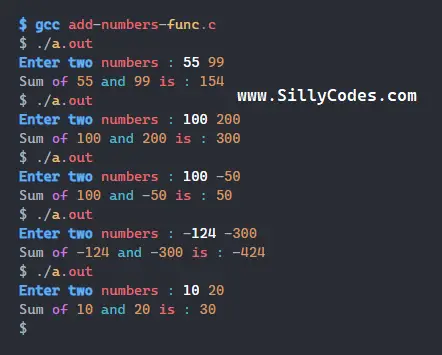
As we can see from the above output, We are getting the expected results.
Related Programs:
- C Program to Calculate the Power of Number
- C Program to Check Number is Power of 2
- C Program to find power of number without Loops
- C Program to Find all Factors of a Number
- C Program to find all Prime Factors of numberÂ
- C Program to Calculate the GCD or HCF of Two Number
- C Program to Calculate the LCM of two Numbers
- C Program to Check Palindrome Number
- C Program to Check 3-Digit and N-Digit Armstrong Number
- C Program to Generate Armstrong Numbers upto N ( User-Provided Number)
- C Program to Generate Armstrong Numbers between two Intervals
2 Responses
[…] Add Two Numbers using Functions in C […]
[…] Add Two Numbers using Functions in C […]