Binary to Decimal in C using function
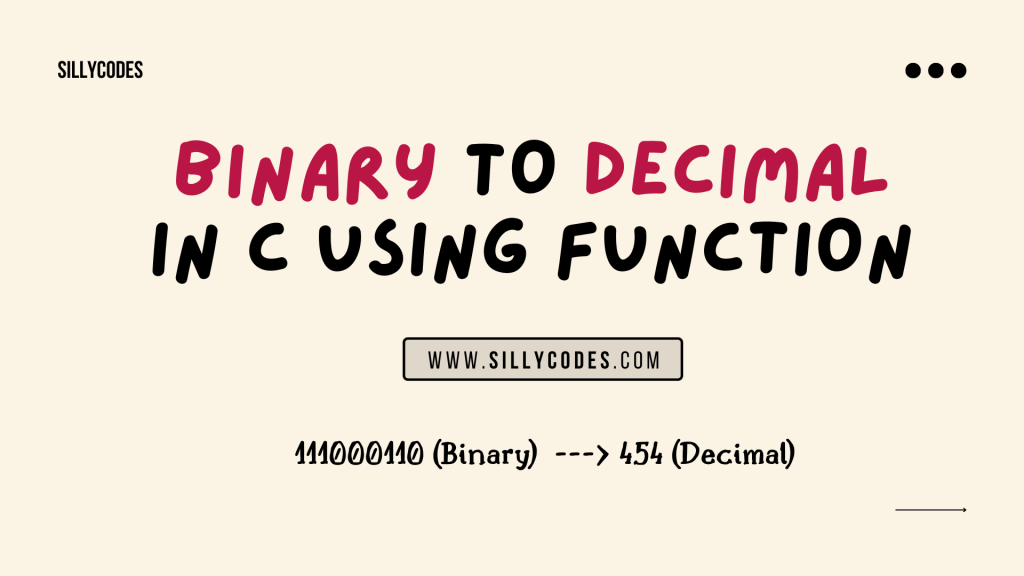
Binary to Decimal in C using function Program Description:
Write a Program to convert the Binary to Decimal in C using function. We need to create a function which takes a binary number as a input and returns decimal equivalent of it., The input number should only contain the digits zero’s and one’s.
Example Input and Output:
Input:
Enter a Binary Number: 0011010
Output:
Binary number 11010 is equal to decimal 26
Pre-Requisites:
It is recommended to go through the following articles to better understand the binary to decimal conversion program using functions.
Binary to Decimal in C using function Algorithm:
- Start the program by accepting a integer number from the user and store it variable called num
- Define a function called
binaryToDecimal, The binaryToDecimal function should accept an
long integer as input value and convert it into a decimal value. Here are the details of the binaryToDecimal function.
- The function accepts a long integer and returns an integer.
- If the user provides invalid input, binaryToDecimal function return -1 value. The input can be considered invalid if it is a negative number or contains any number other than the zeroes and ones.
- If the number is valid, Then we use the the for loop to convert the binary number to a decimal number.
- The logic is to get each digit of the binary number num and use the power function to calculate the power of 2 (as it is a binary number) with respect to the digit at the given position.
- We use the math.h header file pow function to calculate the power. i.e pow(2, i)
- Once the loop is terminated, The variable decimal contains the converted number. return the decimal variable.
- Call the binaryToDecimal function from the main function. As the decimalToBinary function returns a integer number, store it in variable called result.
- Check the
resultvariable
- if result is -1, Then num is invalid number, display the error message.
- otherwise, The binaryTodecimal function successfully converted the binary to a decimal number. Display the result.
- stop
Binary to Decimal in C using function Program:
As we are using the pow function, We need to include the math.h header file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
/*     Program to convert binary number to decimal using function */  #include<stdio.h> #include<math.h>  /** * @brief Convert Binary number to Decimal Number * * @param num - Number to convert (int) * @return onError  -1 (int) * @return onSuccess Decimal Number (int) */  int binaryToDecimal(long int num) {     int rem, i = 0, decimal = 0;      if(num <= 0)     {          // Invalid Input, Return -1         return -1;     }      // Start the loop     for(; num>0; num = num/10) {         // Get the last digit of number         rem = num%10;         // make sure if 'rem' is only 1 or 0         if(rem != 0 && rem != 1) {             // got number other than '0' or '1'             return -1;         }         // calculate the decimal         decimal = decimal + rem * pow(2, i);         // increment i;         i++;     }      return decimal; }  int main() {     long int num;     printf("Enter a Binary Number: ");     scanf("%ld", &num);      int binary = binaryToDecimal(num);     if(binary == -1)     {         // Invalid Input, Terminate         printf("Invalid Input, Try Again \n");     }     else     {         // Converted Number, Print the result         printf("Binary number %ld is equal to decimal %d \n", num, binary);     }      return 0; } |
Here is the prototype details of the binaryToDecimal function.
- int <strong>binaryToDecimal</strong>(long int num)
- function_name: binaryToDecimal
- arguments_list: long int
- return_type:
int
- onError returns -1
- onSuccess returns decimal value
📢 Related Program: Convert Binary to Decimal using loops
Program Output:
To compile the program we need include the -lm compilation option as we are using the math library’s pow function.
$ gcc binary-decimal-function.c  -lm
The above command produces the executable file ( a.out).
Let’s run the program.
Test Case 1: Try Valid Binary Numbers:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter a Binary Number: 10010101 Binary number 10010101 is equal to decimal 149 $ ./a.out Enter a Binary Number: 10100001111000 Binary number 10100001111000 is equal to decimal 10360 $ ./a.out Enter a Binary Number: 0011010 Binary number 11010 is equal to decimal 26 $ ./a.out Enter a Binary Number: 111000110 Binary number 111000110 is equal to decimal 454 $ |
As we can see from the above output, Program is properly converting the binary number into the a decimal value.
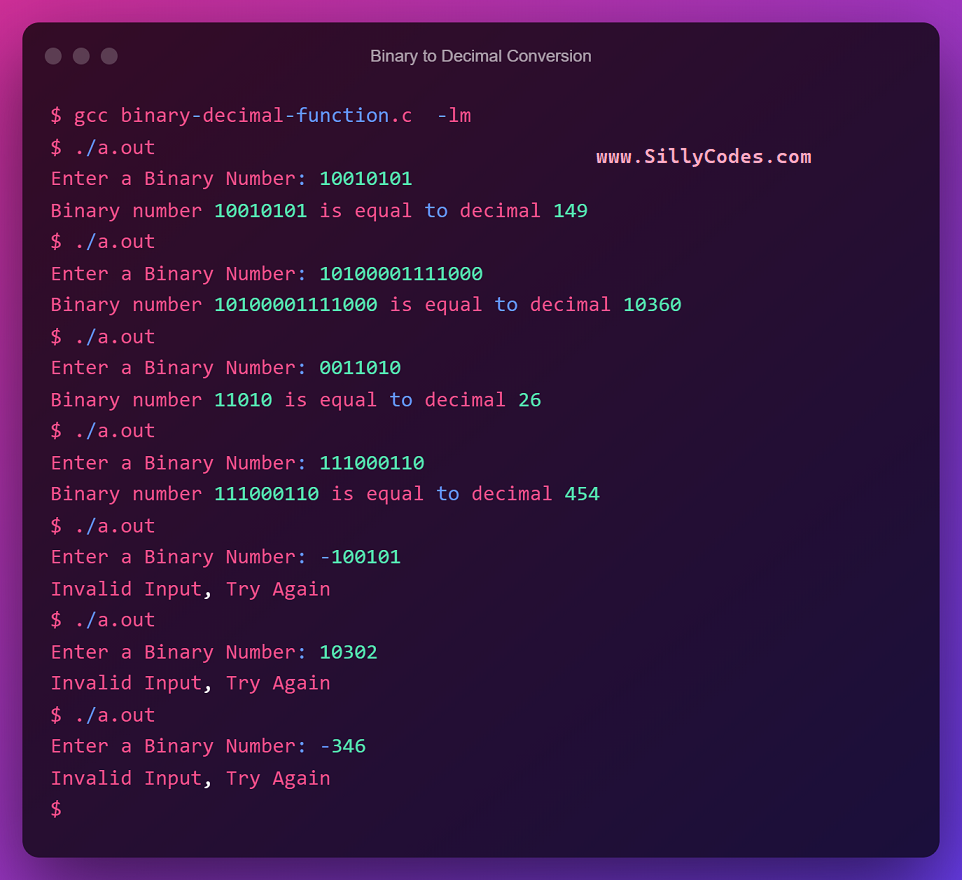
Test Case 2: Negative numbers and invalid numbers test case:
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter a Binary Number: -100101 Invalid Input, Try Again $ ./a.out Enter a Binary Number: 10302 Invalid Input, Try Again $ ./a.out Enter a Binary Number: -346 Invalid Input, Try Again $ |
As excepted, The program is displaying the error message ( Invalid Input, Try Again) on Invalid Numbers like negative numbers and numbers with non-binary digits.
2 Responses
[…] Binary to Decimal Conversion using function in C […]
[…] Binary to Decimal Conversion using function in C […]