Program to Search for a Substring in a String in C
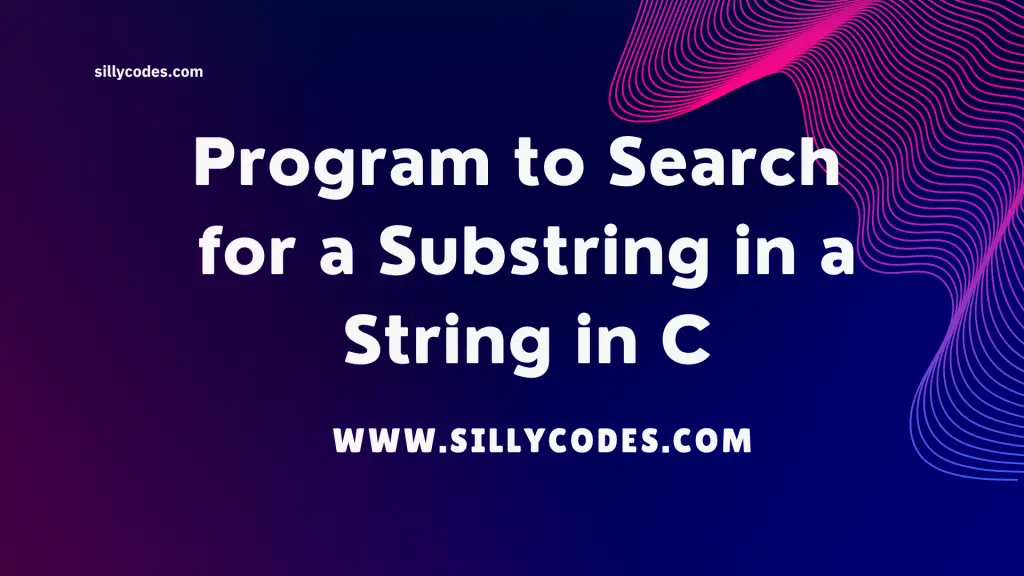
Program Description:
Write a Program to Search for a substring in a string in C programming language. The program should accept two strings from the user, one for the main string and one for the substring. The program must then look for the substring within the main string and return the result.
📢 This Program is part of the C String Practice Programs series
We are going to look at a few methods to search for a substring in a string and each program will be followed by a detailed step-by-step explanation of the program and program output. We also included the instructions to compile and run the program.
Let’s look at the excepted input and output of the program.
Expected Input and Output:
Input:
Enter a main string : learn programming at sillycodes.com
Enter the substring(String to search in main string): sillycodes
Output:
FOUND! Substring:'sillycodes' is found in mainString:'learn programming at sillycodes.com'
The sub string sillycodes is found in the main string learn programming at sillycodes.com.
Prerequisites:
To better understand the program, please go through the following articles, where we discussed about the strings and functions in c language.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
- Functions in C – How to Create and Use functions in C
We will look at three methods to search for the substring in the main string. They are
- Manual Approach – Where we use an iterative method to search for the sub-string in the main string
- User-defined function – we use a custom user-defined to search for a string in the main string.
- Library function – We use the strstr() library function to search for the target string in the main string.
Let’s look at the step-by-step instructions for the iterative program.
Algorithm to Search for a Substring in a String (Manual Method):
- Create a Macro( SIZE) to store the max size of the strings. We can also use a global constant.
- Start the main() function by declaring the two strings and name them as mainStr and subStr. The size of both strings is equal to SIZE macro.
- Take two strings from the user and store them in mainStr and subStr respectively. We use the gets function to read the strings.
- To search for the substring( subStr) in the main string( mainStr), Iterate through the main string( mainStr), looking for the first character of the substring( subStr[0]). If we found the first character of the substring, we should then determine whether all other characters of the substring match. If that’s the case, we found the substring( subStr) in the main string( mainStr).
- Create a For loop by initializing variable i with 0 to iterate over the
mainStr. It will continue looping as long as the current character (
mainStr[i]) is not a
NULL character (
'\0').
- At each iteration, Check if the current character( mainStr[i]) is equal to first character of substring( subStr[0]) - i.e if(mainStr[i] == subStr[0])
- If the above condition is
true, Then search for all characters of the
subStr in the
mainStr. We need to use another for loop to iterate over the contents of the
subStr – i.e
for(j=0; subStr[j]; j++).
- Check if the mainStr[i] is not matching to subStr[j], If so then the subString is not found. Otherwise, continue to check all elements of the subStr.
- Once the above loop is completed, Check if we iterated complete subStr ( use j == strlen(substr) ). If we iterated the complete subStr, then we found the match. Display the message saying FOUND on the console. Terminate the program.
- If step 5 is completed and the program is not exited, Then it means the substring subStr is not found in the main string mainStr. Display the NOT FOUND message on the console and stop the program.
Search for a Substring in a String in C Language – Manual Method:
Here is the program to search for a substring in a main string in c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
/* program to Search for a substring in a string in C sillycodes.com */ #include <stdio.h> #include <string.h> // Max Size of string #define SIZE 100 int main() { // declare two strings with 'SIZE' char mainStr[SIZE], subStr[SIZE]; int i, j; // Take the main string from the user printf("Enter a main string : "); gets(mainStr); // take the sub string printf("Enter the substring(String to search in main string): "); gets(subStr); // Iterate over the main string // We can also iterate only up to (i < strlen(mainStr)-strlen(subStr)) for(i = 0; mainStr[i]; i++) { // search for the first character in substring if(mainStr[i] == subStr[0]) { // The first character of substring is matched. // Check if all characters of substring matching. for(j=0; subStr[j]; j++) { if(mainStr[i] != subStr[j]) { // Not matched break; } i++; } // check if the 'j' is equal to length of the 'subString', Then we found the match if(j == strlen(subStr)) { printf("FOUND! Substring:'%s' is found in mainString:'%s' \n", subStr, mainStr); return 0; } } } // If we reached here, Then string is not found printf("NOT FOUND! Substring:'%s' is NOT found in mainString:'%s' \n", subStr, mainStr); return 0; } |
As we are using the strlen() library function, We need to include the string.h header file.
Program Output:
Compile the program using GCC compiler (Any of your favorite compilers)
$gcc search-string-iterative.c
The above command generates an executable file named a.out. Run the executable file.
1 2 3 4 5 |
$ ./a.out Enter a main string : learn programming at sillycodes.com Enter the substring(String to search in main string): sillycodes FOUND! Substring:'sillycodes' is found in mainString:'learn programming at sillycodes.com' $ |
As we can see from the above output, The substring sillycodes.com is found in the main string.
If we look at the step-by-step execution of the program.
The program looks for the first character of the substring( subStr[0]) in the main string. In the above example, The first character subStr[0] is found at index 21 of the main string.
mainStr[21]=s, subStr[0]=s
Then we will try to match all characters of the substring with the main string. Here is how the flow looks like
mainStr[22]=i, subStr[1]=i
mainStr[23]=l, subStr[2]=l
mainStr[24]=l, subStr[3]=l
mainStr[25]=y, subStr[4]=y
mainStr[26]=c, subStr[5]=c
mainStr[27]=o, subStr[6]=o
mainStr[28]=d, subStr[7]=d
mainStr[29]=e, subStr[8]=e
mainStr[30]=s, subStr[9]=s
Once all characters are matched, The inner for loop will be completed, Then we are checking the length of the j with the subStr length.
If we look at the j and sub string Length.
i:31, j:10, length:10
Both are the same. So we found the sub-string sillycodes.com in the main string learn programming at sillycodes.com
FOUND! Substring:’sillycodes’ is found in mainString:’learn programming at sillycodes.com’
Program to Search for a substring in main string using a user-defined function:
In the above program, We have written the complete program inside the main function. Let’s divide the above program and convert it to use a function to search for the substring in the main string.
By using the functions we can define a function once and can call it as many times as we want. Functions also increase the readability of the program and make it easier to debug issues. Here are the benefits using the functions in programming.
Here is the rewritten version of the above program, Where we used a custom function to search for a substring in the main string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 |
/* program to Search for a substring in a string in C using function sillycodes.com */ #include <stdio.h> #include <string.h> // Max Size of string #define SIZE 100 /** * @brief - Search for the 'subStr' in 'mainStr' and return the result * * @param mainStr - Main String * @param subStr - Sub String ( string to be searched in main string) * @return int - Returns 1 if 'subStr' found in 'mainStr'. Otherwise, returns Zero. */ int searchString(char * mainStr, char * subStr) { int i, j; // Iterate over the main string // We can also iterate only up to (i < strlen(mainStr)-strlen(subStr)) for(i = 0; mainStr[i]; i++) { // search for the first character in substring if(mainStr[i] == subStr[0]) { // The first character of substring is matched. // Check if all characters of substring matching. for(j=0; subStr[j]; j++) { if(mainStr[i] != subStr[j]) { // Not matched break; } i++; } // check if the 'j' is equal to length of the 'subString', Then we found the match if(j == strlen(subStr)) { // found return 1; } } } // Not found return 0; } int main() { // declare two strings with 'SIZE' char mainStr[SIZE], subStr[SIZE]; // Take the main string from the user printf("Enter a main string : "); gets(mainStr); // take the sub string printf("Enter the substring(String to search in main string): "); gets(subStr); int isFound = searchString(mainStr, subStr); if(isFound) { // Found printf("FOUND! Substring:'%s' is found in mainString\n", subStr); } else { // Not found printf("NOT FOUND! Substring:'%s' is NOT found in mainString\n", subStr); } return 0; } |
In the above program, We have created a function called searchString to search for the target string the main string. THe Prototype of the function is
1 2 3 4 5 6 7 8 9 |
/** * @brief - Search for the 'subStr' in 'mainStr' and return the result * * @param mainStr - Main String * @param subStr - Sub String ( string to be searched in main string) * @return int - Returns 1 if 'subStr' found in 'mainStr'. Otherwise, returns Zero. */ int searchString(char * mainStr, char * subStr) |
As we can see from the prototype, The searchString function takes two character pointers( char *) that point to the main string( mainStr) and substring( subStr) respectively.
It also returns an integer to the caller. It returns One(1) if the subStr is found in the mainStr, Otherwise, it returns Zero(0).
Finally, call the searchString() function from the main() function with the main string and substring as the parameters.
1 |
int isFound = searchString(mainStr, subStr); |
Store the return value in isFoundvariable and display the results based on the isFound variable.
Program Output:
Let’s compile and Run the program.
Test 1: Positive test case
1 2 3 4 5 6 |
$ gcc search-string-fun.c $ ./a.out Enter a main string : git and github or bitbucket Enter the substring(String to search in main string): github FOUND! Substring:'github' is found in mainString $ |
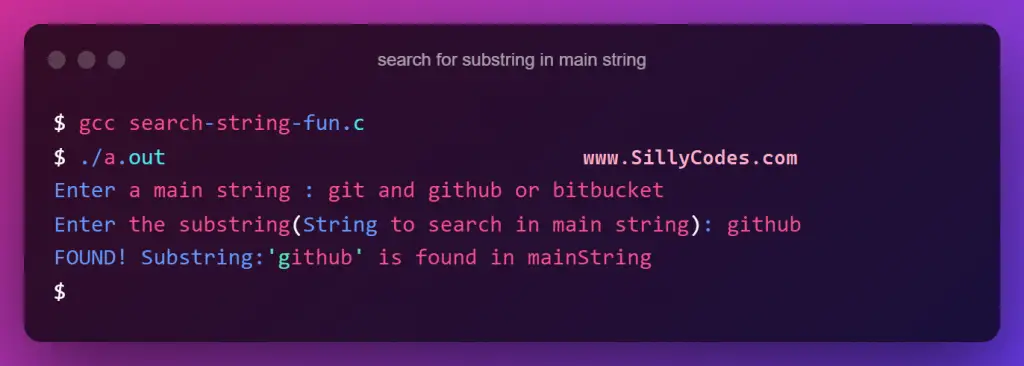
In the above example, The sub-string is found in the main string.
Test 2: Negative test case
1 2 3 4 5 |
$ ./a.out Enter a main string : Learn Programming Enter the substring(String to search in main string): online NOT FOUND! Substring:'online' is NOT found in mainString $ |
The substring online is not found in the main string Learn Programming.
Search for a substring in a string using strstr function in C:
The C Programming language also provides a library function called strstr to search for a string in a string. Let’s use the strstr library function to search for the string in the main string.
▶️ We have covered the strstr function in detail in the following article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
/* program to Search for a substring in a string in C using strstr sillycodes.com */ #include <stdio.h> #include <string.h> // Max Size of string #define SIZE 100 int main() { // declare two strings with 'SIZE' char mainStr[SIZE], subStr[SIZE]; int i, j; // Take the main string from the user printf("Enter a main string : "); gets(mainStr); // take the sub string printf("Enter the substring(String to search in main string): "); gets(subStr); // call the 'strstr' function with two strings char * res = strstr(mainStr, subStr); if( res != NULL) { // Found printf("FOUND! Substring:'%s' is found in mainString:'%s' \n", subStr, mainStr); } else { // Not found printf("NOT FOUND! Substring:'%s' is NOT found in mainString:'%s' \n", subStr, mainStr); } return 0; } |
Program Output:
Compile and Run the program.
1 2 3 4 5 6 |
$ gcc search-substring-strstr.c $ ./a.out Enter a main string : The end is the beginning, and the beginning is the end. Enter the substring(String to search in main string): end FOUND! Substring:'end' is found in mainString:'The end is the beginning, and the beginning is the end.' $ |
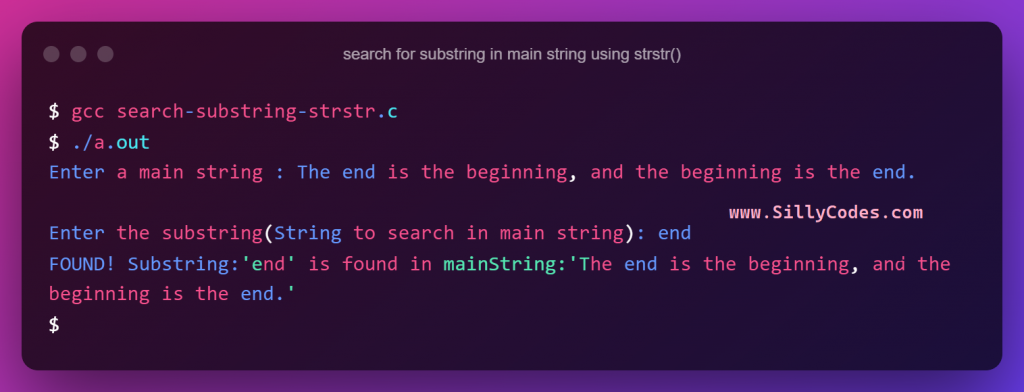
Let’s look at another example.
1 2 3 4 5 |
$ ./a.out Enter a main string : super excited to learn programming Enter the substring(String to search in main string): c programming NOT FOUND! Substring:'c programming' is NOT found in mainString:'super excited to learn programming' $ |
As we can see from the above output, The program is properly searching and finding the target string in the main string.
Related Programs:
- C Tutorials Index
- C Programs Index
- Count the Number of Vowels, Consonants, Digits, and Special characters in String
- C Program to Count Alphabets, Digits, Whitespaces in String
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
- C Program to Count Frequencies of each character in a string
- C Program to Find Highest Frequency character in a String
- C Program to Find Lowest Frequency Character in a String
1 Response
[…] C Program to Search for substring in a String […]