C program to Calculate the number of positive and negative numbers in an Array
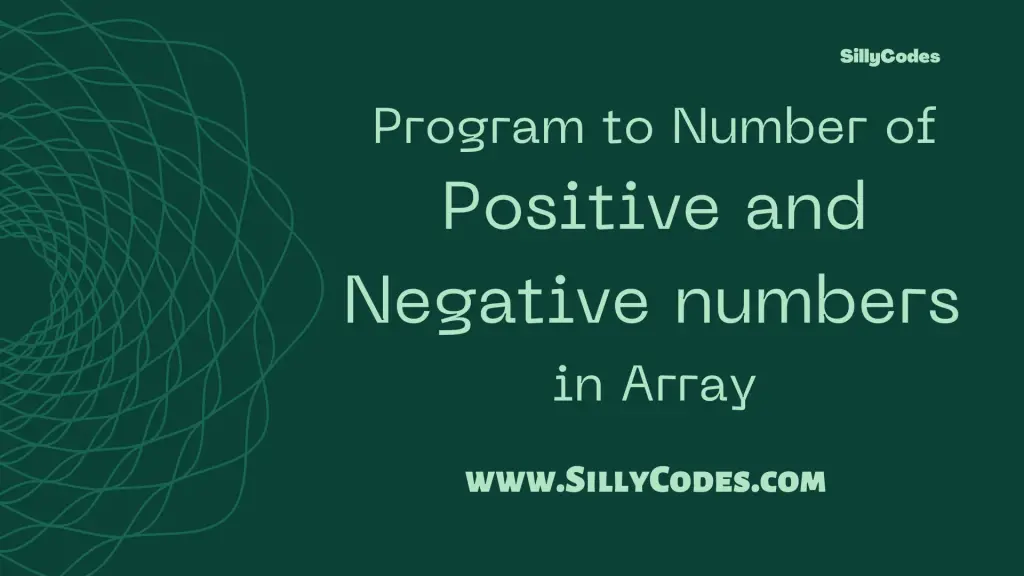
- The number of positive and negative numbers in an array Program Explanation:
- Number of positive and negative numbers in an array Algorithm:
- Program to calculate Number of positive and negative numbers in an Array:
- Number of positive and negative numbers in an array using a user-defined function:
- Related Array Programs:
Program Description:
Write a Program to count the Number of positive and negative numbers in an array in the C programming language. The program will accept an array from the user and then displays the total number of Positives numbers, Negatives numbers, and Zeros on the console.
Example Input and Output:
Input:
1 2 |
Enter desired array size(1-100): 10 Please enter array elements(10) : 23 84 -34 -74 0 -283 455 0 83 0 0 |
Output:
1 2 3 |
Number of Positive Numbers in array is : 4 Number of Negative Numbers in array is : 3 Number of Zeroes in array is : 3 |
Prerequisites:
It is recommended to go through the following prerequisites to better understand the program.
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- How to pass arrays to functions in C
- Functions in C Language
The number of positive and negative numbers in an array Program Explanation:
- Create an array named numbers with size 100
- Also, initialize the other variables like size, nPositive, nNegative, and nZeros variables with Zero.
- Take the size of the array from the user. and update the size variable.
- Now, Prompt the user to provide the elements to fill the array. Use a for loop to iterate over the numbers array. Start the loop from zero(0) and run it till the size-1 and update each element using the scanf function.
- To count the number of positive and negative and zeros in the given array. We need to traverse the array element by element and check if it is positive or negative or zero.
- Create another for loop to iterate over the array. The loop starts from
Zero and goes till
size-1
- Check if the number[i] is positive, If it is true, Then increment the nPositive variable.
- If the number[i] is a Negative number, Then increment the nNegative variable.
- If the above two conditions are false, Then the number must be Zero(0). So increment the nZeros variable.
- Once the above loop is completed, The variables nPositive, nNegative, and nZeros contains the number of positive, negative, and zeros in the array.
- Display the results on the console using printf function.
Number of positive and negative numbers in an array Algorithm:
- Declare the array numbers[100] and The i, size variables.
- Initialize nPositive = 0, nNegative = 0, nZeros = 0; variables
- Take the desired array size from user
- Prompt the user to provide the numbers for array elements.
- Loop from Zero(0) to size-1 using for loop.
- Read the value from the user and update the numbers[i] element
- Create another for loop to traverse the array.
- Loop from Zero(0) to size-1 using for loop.
- If the numbers[i] > 0 is true, Increment nPositive – i.e nPositive++;
- else if the numbers[i] < 0 is true, Increment nNegative variable – i.e nNegative++;
- else increment nZeros variable – i.e nZeros++;
- Display the results on standard output.
Program to calculate Number of positive and negative numbers in an Array:
Here is the C Code for the above count number of positive and negative numbers in the array algorithm.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
/* Program to Number of positive and negative numbers in an array sillycodes.com */ #include <stdio.h> int main() { // declare the 'numbers' array int numbers[100]; int i, size; // initialize number of positive, number of negative number with 0. int nPositive = 0, nNegative = 0, nZeros = 0; printf("Enter desired array size(1-100): "); scanf("%d", &size); // User input for array elements printf("Please enter array elements(%d) : ", size); for(i = 0; i < size; i++) { // printf("numbers[%d] : ", i); scanf("%d", &numbers[i]); } // Iterate over array, check each each number for(i = 0; i < size; i++) { // Check the number[i] if(numbers[i] > 0) { // Positive Number, Increment nPositive nPositive++; } else if (numbers[i] < 0) { // Negative Number, Increment nNegative by 1. nNegative++; } else { // zero, Increment nZeros nZeros++; } } // display the results. printf("Number of Positive Numbers in array is : %d \n", nPositive); printf("Number of Negative Numbers in array is : %d \n", nNegative); printf("Number of Zeroes in array is : %d \n", nZeros); return 0; } |
Program Output:
Compile and Run the program.
Test 1:
1 2 3 4 5 6 7 8 |
$ gcc pos-neg.c $ ./a.out Enter desired array size(1-100): 10 Please enter array elements(10) : 23 84 -34 -74 0 -283 455 0 83 0 0 Number of Positive Numbers in array is : 4 Number of Negative Numbers in array is : 3 Number of Zeroes in array is : 3 $ |
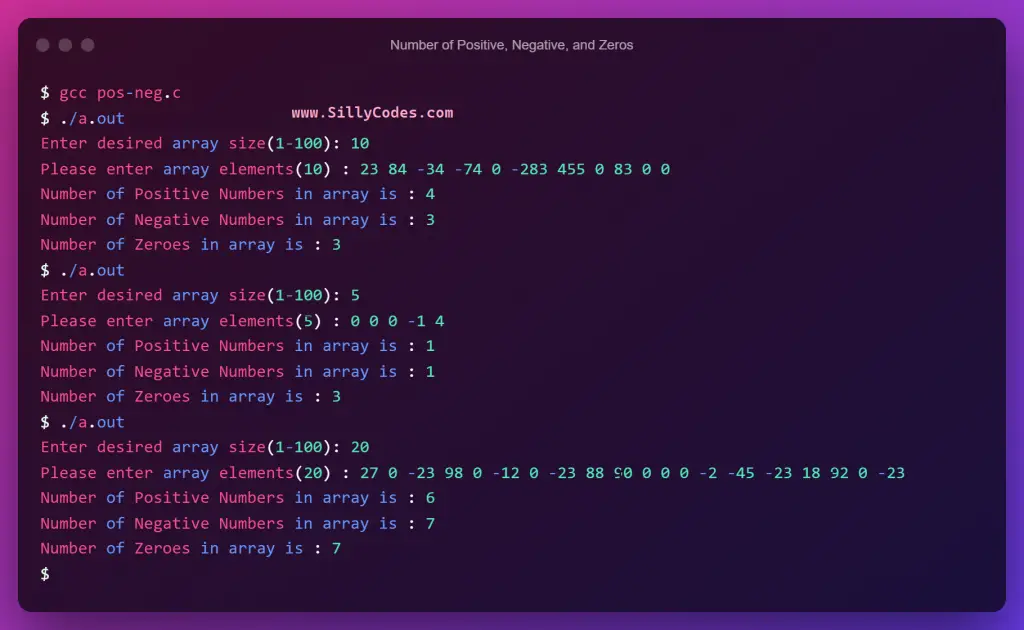
Test 2: Try a few more examples
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter desired array size(1-100): 5 Please enter array elements(5) : 0 0 0 -1 4 Number of Positive Numbers in array is : 1 Number of Negative Numbers in array is : 1 Number of Zeroes in array is : 3 $ ./a.out Enter desired array size(1-100): 20 Please enter array elements(20) : 27 0 -23 98 0 -12 0 -23 88 90 0 0 0 -2 -45 -23 18 92 0 -23 Number of Positive Numbers in array is : 6 Number of Negative Numbers in array is : 7 Number of Zeroes in array is : 7 $ |
As we can see from the above output, The program is providing the number of positive numbers, Negative numbers, and Zeroes in the array.
Number of positive and negative numbers in an array using a user-defined function:
Let’s convert the above program to use the user-defined functions instead of using only one function (i.e main())
The following program is a rewritten version of the above program, which uses four user-defined functions to do a specific task.
For example, The read function is used to take the user input and update the array. and The getNPositives() function takes the input array and returns the number of positive numbers in the array. Similarly, the other two functions also return negative numbers and zeros.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 |
/* Program to Number of positive and negative numbers in an array using functions Author: sillycodes.com */ #include <stdio.h> // Function declarations int getNPositives(int numbers[], int size); int getNNegatives(int numbers[], int size); int getNZeros(int numbers[], int size); /** * @brief - Read the user input and update the array * * @param numbers - 'numbers' input array * @param size - size of 'numbers' array */ void read(int numbers[], int size) { int i; // User input for array elements printf("Please enter array elements(%d) : ", size); for(i = 0; i < size; i++) { // printf("numbers[%d] : ", i); scanf("%d", &numbers[i]); } } int main() { // declare the 'numbers' array int numbers[100]; int i, size; // initialize number of positive, number of negative number with 0. int nPositive = 0, nNegative = 0, nZeros = 0; printf("Enter desired array size(1-100): "); scanf("%d", &size); // Update the array read(numbers, size); // call 'getNpositives', 'getNNegatives', and 'getNZeroes' // to get the number of positive, negatives and zeroes respectively. nPositive = getNPositives(numbers, size); nNegative = getNNegatives(numbers, size); nZeros = getNZeros(numbers, size); // display the results. printf("Number of Positive Numbers in array is : %d \n", nPositive); printf("Number of Negative Numbers in array is : %d \n", nNegative); printf("Number of Zeroes in array is : %d \n", nZeros); return 0; } /** * @brief - Get number of positive numbers in array * * @param numbers - Array * @param size - Size of array * @return int - number of positive numbers */ int getNPositives(int numbers[], int size) { int nPositive = 0, i; // Iterate over array, check each each number for(i = 0; i < size; i++) { // Check the number[i] if(numbers[i] > 0) { // Positive Number, Increment nPositive nPositive++; } } return nPositive; } /** * @brief - Get number of negative numbers in array * * @param numbers - Array * @param size - size of 'numbers' array * @return int - Number of Negative numbers in 'numbers' array */ int getNNegatives(int numbers[], int size) { int nNegative = 0, i; // Iterate over array, check each each number for(i = 0; i < size; i++) { // Check the number[i] if (numbers[i] < 0) { // Negative Number, Increment nNegative by 1. nNegative++; } } return nNegative; } /** * @brief - get number of zeroes in the array * * @param numbers - numbers array * @param size - Size of the numbers array * @return int - number of zeros in the array */ int getNZeros(int numbers[], int size) { int nZeroes = 0, i; // Iterate over array, check each each number for(i = 0; i < size; i++) { // Check the number[i] if (numbers[i] == 0) { // Zero nZeroes++; } } return nZeroes; } |
We have defined Four functions in the above program. Here are the details of these functions.
- The
read() function –
void read(int numbers[], int size)
- read() function takes two formal arguments and Array and it’s size and updates it with the user input.
- The
getNPositives() function –
int getNPositives(int numbers[], int size)
- getNPositives() function takes two formal arguments, numbers[] array and its size
- The getNPositives() function calculates the number of positive numbers in the numbers array and returns it back to the caller.
- The
getNNegatives() function –
int getNNegatives(int numbers[], int size)
- getNNegatives() function takes two formal arguments, numbers[] array and its size
- The getNNegatives() function calculates the number of negative numbers in the numbers array and returns it back to the caller.
- The
getNZeros() function –
int getNZeros(int numbers[], int size)
- getNZeros() function takes two formal arguments, numbers[] array and its size
- The getNZeros() function calculates the number of zeros in the numbers array and returns it back to the caller.
Program Output:
Compile and run the program and see the results.
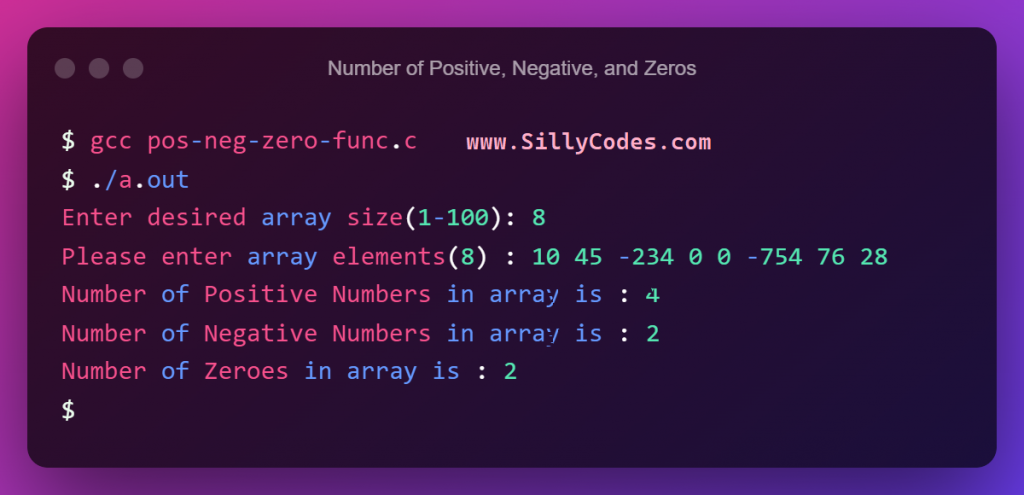
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc pos-neg-zero-func.c $ ./a.out Enter desired array size(1-100): 8 Please enter array elements(8) : 10 45 -234 0 0 -754 76 28 Number of Positive Numbers in array is : 4 Number of Negative Numbers in array is : 2 Number of Zeroes in array is : 2 $ ./a.out Enter desired array size(1-100): 4 Please enter array elements(4) : 88 -45 -3 0 Number of Positive Numbers in array is : 1 Number of Negative Numbers in array is : 2 Number of Zeroes in array is : 1 $ |
As expected, The program is providing the desired results.
Related Array Programs:
- Index – C Language Practice Programs
- C Tutorials Index – Step by step tutorials to master C Langauge
- C Program to Read and Print Arrays
- Reverse Print Array Program in C
- C Program to calculate Sum of all array Elements
- C Program to Calculate the Average of all array Elements
- C Program to find minimum element in Array
1 Response
[…] C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array […]