Program to Count Number of Even and Odd numbers in Array in C language
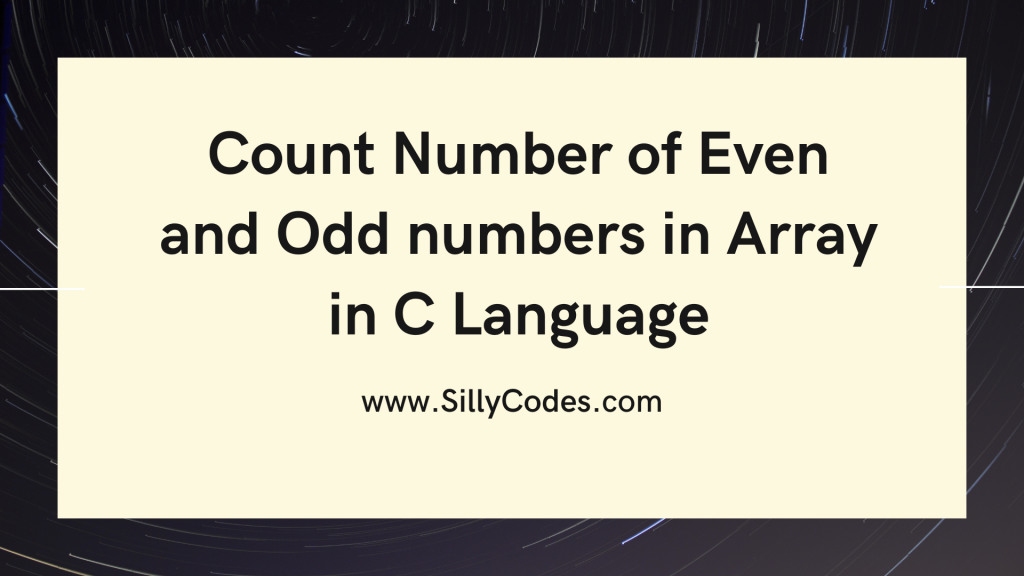
Program Description:
Write a program to count the Number of Even and Odd numbers in Array in C programming language. The program takes an input array from the user and counts the number of even numbers and odd numbers in the given array.
Expected Input and Output:
Input:
1 2 |
Enter desired array size(1-100): 5 Please enter array elements(5) : 01 23 49 20 34 |
Output:
1 2 |
Number of Even Numbers in array is : 2 Number of Odd in array is : 3 |
Program Prerequisites:
It is recommended to know the basic of the Arrays and functions and how to pass arrays to the functions in C language. Please go through the following articles to learn more about these concepts.
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- 2D arrays in C with Example programs
- How to pass arrays to functions in C
- Functions in C Language
Number of Even and Odd numbers in Array Explanation:
- Declare an array called numbers with a size of 100. Also declare the variables size and i.
- Create two variables to track the count of the total number of even numbers and odd numbers. So Initialize the nEven and nOdd variables with Zero(0).
- Take the desired size of the array from the user and update the size variable.
- Now, We need to prompt the user to provide the values for the array.
- We are using a for loop to iterate over the array and prompt the user to provide the number.
- Read the number using scanf function and update the numbers[i] element of the array.
- Once we got the elements from the user. The next step is to count the number of Even numbers and Odd numbers in an array.
- To calculate the count of Even and Odd numbers, We need to traverse through the array and check each element.
- We use another For loop to traverse the array. At each iteration,
- Check if the numbers[i] is perfectly divisible by the 2. If the numbers[i] is perfectly divisible, Then the numbers[i] is an Even Number. So Increment the nEven variable by 1.
- Else then the number is an Odd Number, So increment the nOdd variable by 1.
- Once the above loop is completed, The variables nEven and nOdd contains the total number of even numbers and odd numbers in the numbers array respectively.
- Print the result on the standard output using printf function.
C Program to count Even and Odd numbers in Array Algorithm:
- Start the program
- Declare numbers[100], i, and size variables.
- Initialize nEven and nOdd with Zero.
- Take array
size from user
- scanf(“%d”, &size);
- Update array elements
- Loop from zero(0) to size-1 using for loop
- update numbers[i] element – scanf(“%d”, &numbers[i]);
- Traverse the Array using another for loop and check the each element
- For Loop from Zero(0) to size-1
- check if(numbers[i]%2 == 0) is true, Then increment nEven
- Else increment nOdd
- Display results on the console.
Program to find Number of Even and Odd numbers in Array in C:
Here is the program to count the number of even numbers and odd numbers in an array using C programming language
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
/* Program to Number of Even and Odd Numbers in an array sillycodes.com */ #include <stdio.h> int main() { // declare the 'numbers' array int numbers[100]; int i, size; // initialize 'nEven' and 'nOdd' with zero. int nEven = 0, nOdd = 0; // int nEven = nOdd = 0; printf("Enter desired array size(1-100): "); scanf("%d", &size); // User input for array elements printf("Please enter array elements(%d) : ", size); for(i = 0; i < size; i++) { // printf("numbers[%d] : ", i); scanf("%d", &numbers[i]); } // Iterate over array, Check the numberss for(i = 0; i < size; i++) { // Check the number[i] if(numbers[i]%2 == 0) { // Even Number, Increment 'nEven' counter nEven++; } else { // Odd Number nOdd++; } } // display the results. printf("Number of Even Numbers in array is : %d \n", nEven); printf("Number of Odd in array is : %d \n", nOdd); return 0; } |
Program Output:
Compile the program using GCC compiler (Any compiler)
gcc even-odd.c
Run the program.
Test 1:
1 2 3 4 5 6 |
$ ./a.out Enter desired array size(1-100): 10 Please enter array elements(10) : 23 83 22 88 90 23 475 92 -12 -33 Number of Even Numbers in array is : 5 Number of Odd in array is : 5 $ |
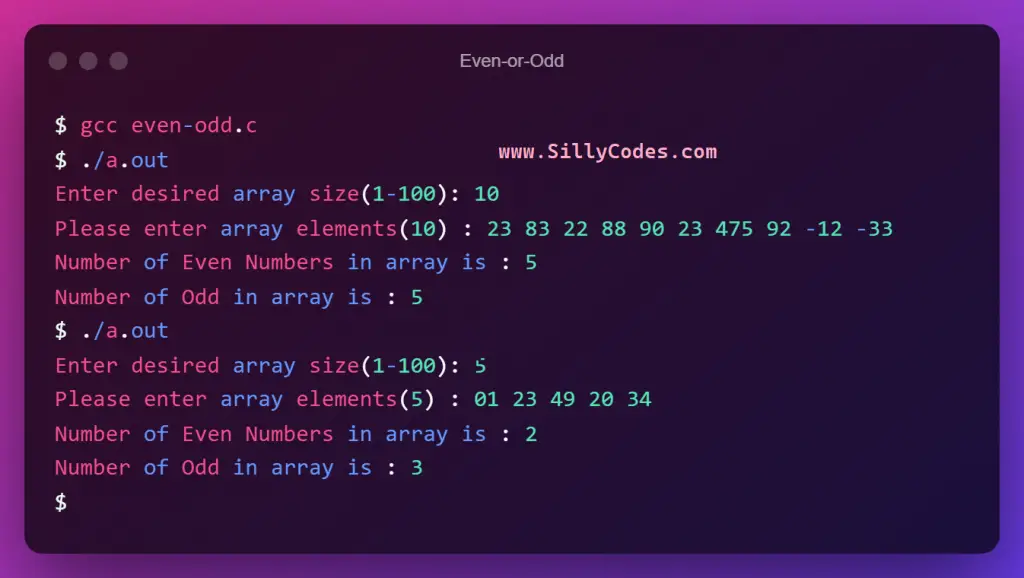
Test 2:
1 2 3 4 5 6 |
$ ./a.out Enter desired array size(1-100): 5 Please enter array elements(5) : 01 23 49 20 34 Number of Even Numbers in array is : 2 Number of Odd in array is : 3 $ |
The program is providing the desired results.
Number of Even and Odd numbers in Array using user-defined functions:
In the above program, We used only one function to write the code. Let’s divide the above program and create a few more functions to do a specific task like reading a number, etc. By using the functions, We can reuse the code and the program becomes modular and easily write, and debug.
📢 Advantages of Functions
Let’s rewrite the above number of even and odd number programs and create a few functions like getNOdd and getNEven
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 |
/* Program to Number of Even and Odd Numbers in an array using functions Author: sillycodes.com */ #include <stdio.h> /** * @brief - Read the user input and update the array * * @param numbers - 'numbers' input array * @param size - size of 'numbers' array */ void read(int numbers[], int size) { int i; // User input for array elements printf("Please enter array elements(%d) : ", size); for(i = 0; i < size; i++) { // printf("numbers[%d] : ", i); scanf("%d", &numbers[i]); } } /** * @brief - Get number of Even numbers in array * * @param numbers - Array * @param size - Size of array * @return int - number of Even numbers */ int getNEven(int numbers[], int size) { int nEven = 0, i; // Iterate over array, check each each number for(i = 0; i < size; i++) { // Check the number[i] if(numbers[i]%2 == 0) { // Even Number, Increment nEven nEven++; } } return nEven; } /** * @brief - Get number of Odd numbers in array * * @param numbers - Array * @param size - size of 'numbers' array * @return int - Number of Odd numbers in 'numbers' array */ int getNOdd(int numbers[], int size) { int nOdd = 0, i; // Iterate over array, check each each number for(i = 0; i < size; i++) { // Check the number[i] if (numbers[i]%2 == 1) { // Odd Number, Increment nOdd by 1. nOdd++; } } return nOdd; } int main() { // declare the 'numbers' array int numbers[100]; int i, size; printf("Enter desired array size(1-100): "); scanf("%d", &size); read(numbers, size); // Call the 'getNEven' and 'getNOdd' functions int nEven = getNEven(numbers, size); int nOdd = getNOdd(numbers, size); // display the results. printf("Number of Even Numbers in array is : %d \n", nEven); printf("Number of Odd in array is : %d \n", nOdd); return 0; } |
Here we have defined three user-defined functions(except the main() function).
- The read() function – Used to read the user input and update the given array
- Prototype of the read function – void read(<strong>int</strong> numbers[], <strong>int</strong> size)
- read() function takes two formal arguments which are numbers[] array and its size.
- The read() function is used to take the input numbers from the user and update the numbers array.
- The
getNEven() function
- Prototype of the getNEven() function – int getNEven(int numbers[], int size)
- The getNEven() function also takes two formal arguments, which are numbers array and its size and returns an integer.
- The getNEven() function calculates the total number of even numbers in the numbers array and returns the results.
- The
getNOdd() Function.
- Here is the prototype of the getNOdd() function – int getNOdd(int numbers[], int size)
- The getNOdd function takes two formal arguments which are numbers array and size variable.
- The getNOdd function calculates the total number of Odd numbers in the numbers array and returns the results back tot eh caller.
Program Output:
Let’s compile and run the program.
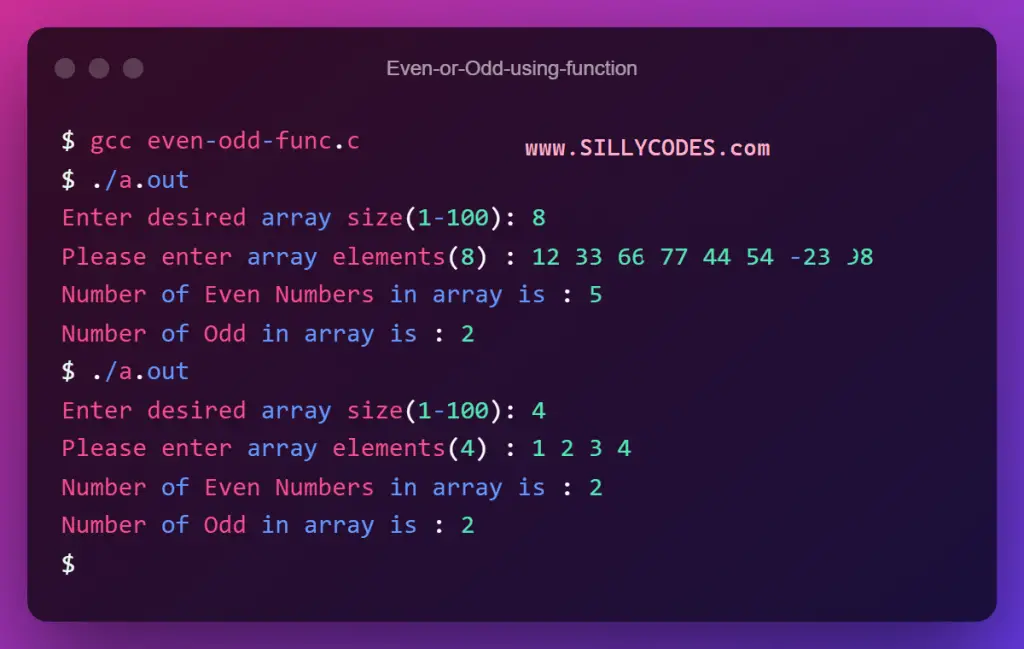
1 2 3 4 5 6 7 8 9 10 11 12 |
$ gcc even-odd-func.c $ ./a.out Enter desired array size(1-100): 8 Please enter array elements(8) : 12 33 66 77 44 54 -23 98 Number of Even Numbers in array is : 5 Number of Odd in array is : 2 $ ./a.out Enter desired array size(1-100): 4 Please enter array elements(4) : 1 2 3 4 Number of Even Numbers in array is : 2 Number of Odd in array is : 2 $ |
As we can see from the above output, the Program is generating the expected results.
Related Array Programs:
- Index – C Language Practice Programs
- C Tutorials Index – Step by step tutorials to master C Langauge
- C Program to Read and Print Arrays
- Reverse Print Array Program in C
- C Program to calculate Sum of all array Elements
- C Program to Calculate the Average of all array Elements
- C Program to find minimum element in Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
1 Response
[…] C Program to Count Even and Odd numbers in Array […]