Program to Read and Print array in C language
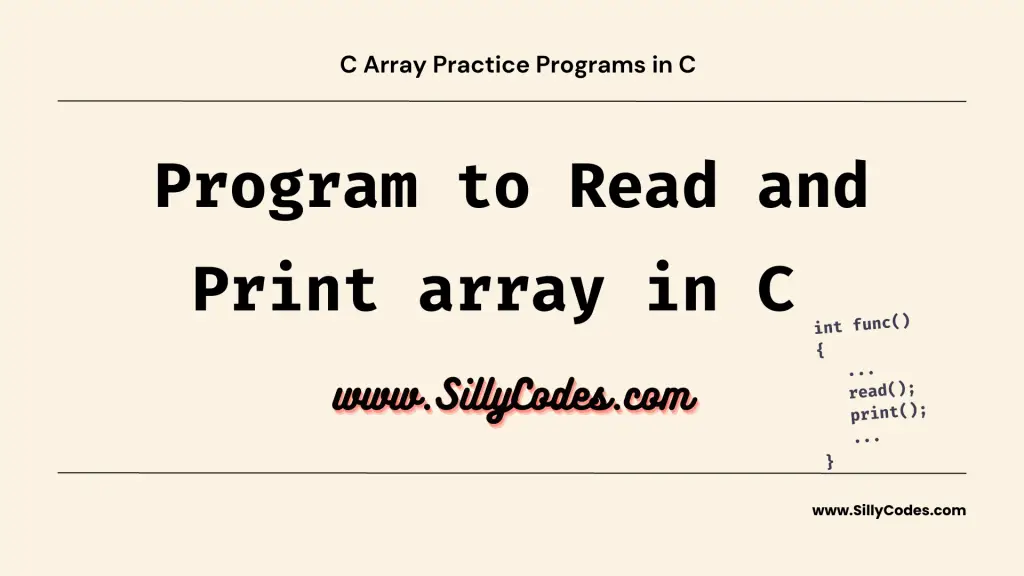
Program Description:
Write a program to Read and Print array in C programming language. The program should accept the integer numbers from the user and store them as the array elements and then print it on the standard output (console)
Example Input and Output:
Input:
1 2 3 4 5 6 7 8 9 10 11 |
Please provide input values for Array array[0] : 84 array[1] : 43 array[2] : 98 array[3] : 44 array[4] : 23 array[5] : 92 array[6] : 74 array[7] : 90 array[8] : 12 array[9] : 67 |
Output:
1 2 3 4 5 6 7 8 9 10 11 |
----- Array Elements -------- array[0] = 84 array[1] = 43 array[2] = 98 array[3] = 44 array[4] = 23 array[5] = 92 array[6] = 74 array[7] = 90 array[8] = 12 array[9] = 67 |
Prerequisites:
As we are going to use the arrays in this program, It is recommended to know the basics of the C Arrays. Please go through the following articles to learn more about Arrays in C
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- 2D arrays in C with Example programs
- How to pass arrays to functions in C
Read and Print array in C Program Explanation:
- Start the program by declaring an
array with the size of
SIZE global constant –
int array[SIZE];
- We created the SIZE global constant and initialized with 10. So the array size is 10. We also use the macro instead of the global constant – const int SIZE = 10;
- Read Array: We need to take the input from the user and update the elements of the array. Use a for loop to iterate over the array and prompt the user for input values for each element of the array(Â array). Then read the values using scanf and store them in the respective array element.
- Print Array: Use for loop to print the elements of the array to the console. The for loop will start from and goes till the SIZE
Program to Read and Print array in C language:
Let’s write the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
/*     Program to read and print the array elements     Author: SillyCodes.com */ #include <stdio.h> const int SIZE = 10; int main() {     int i;     // declare an 'array'     int array[SIZE];      printf("Please provide input values for Array\n");     // Read the input from the user     for(i=0; i<SIZE; i++)     {         printf("array[%d] : ", i);         scanf("%d", &array[i]);     }      // print the array elements on console     printf("----- Array Elements --------\n");     for(i = 0; i<SIZE; i++)     {         printf("array[%d] = %d\n", i, array[i]);     }      return 0; } |
In the above program, We no need to use the size as the extra parameter to the function, As we already have SIZE global constant, We can use it directly.
Program Output:
Let’s compile the program using GCC (Any compiler)
gcc read-print-arr.c
The above command creates an executable file a.out.
Run the program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
$ ./a.out Please provide input values for Array array[0] : 84 array[1] : 43 array[2] : 98 array[3] : 44 array[4] : 23 array[5] : 92 array[6] : 74 array[7] : 90 array[8] : 12 array[9] : 67 ----- Array Elements -------- array[0] = 84 array[1] = 43 array[2] = 98 array[3] = 44 array[4] = 23 array[5] = 92 array[6] = 74 array[7] = 90 array[8] = 12 array[9] = 67 $ |
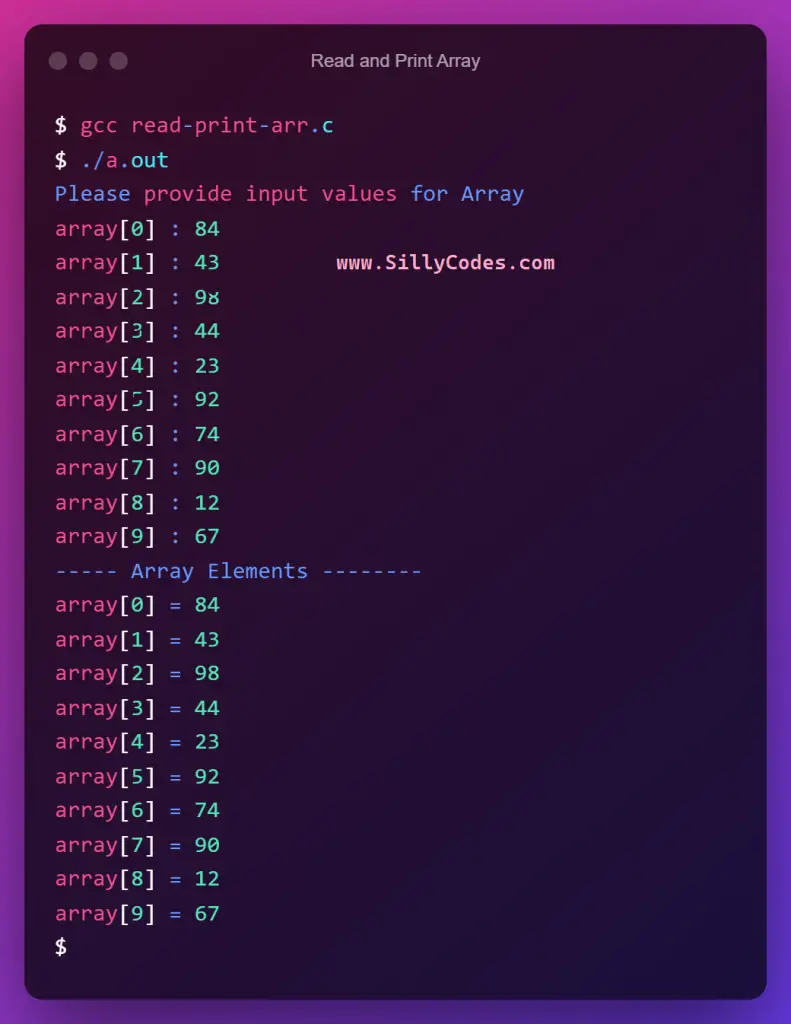
As we can see from the above program, The program is reading the input from the user and printing it back to the console.
Alternative Way: Read and Print the Array in C:
Instead of printing the array element by element, let’s print it in a single line.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
/*     Program to read and print the array elements     Author: SillyCodes.com */ #include <stdio.h> const int SIZE = 10; int main() {     int i;     // declare an 'array'     int array[SIZE];      printf("Please provide input values for Array\n");     // Read the input from the user     for(i=0; i<SIZE; i++)     {         printf("array[%d] : ", i);         scanf("%d", &array[i]);     }      // print the array elements on console     printf("Array Elements are : ");     for(i = 0; i<SIZE; i++)     {         printf("%d ", array[i]);     }     printf("\n");      return 0; } |
Program Output:
Compile and run the program
📢 Related Program: Compile and Run the program in Linux OS.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
$ gcc read-print-arr.c $ ./a.out Please provide input values for Array array[0] : 90 array[1] : 1 array[2] : 09 array[3] : 23 array[4] : 54 array[5] : 75 array[6] : 94 array[7] : 34 array[8] : 12 array[9] : 43 Array Elements are : 90 1 9 23 54 75 94 34 12 43 $ |
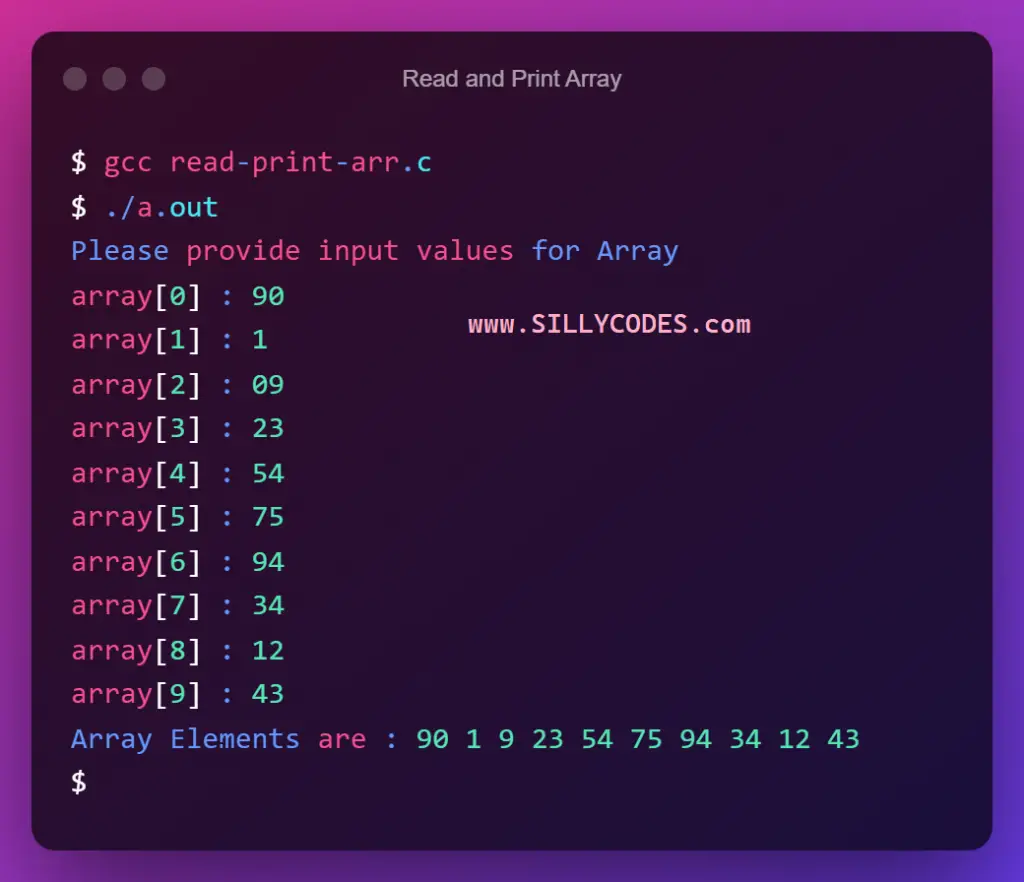
Read and Print array using functions in C programming language:
Read and Print array using Function Program Explanation:
- Create a constant named ARRAY_SIZE with the size of the array.
- In the main() function, declare an integer array arr with ARRAY_SIZE elements.
- Call the
read() function from the
main() function and pass the
arr and its size as arguments. The
read() function will prompt the user for input values for each element of the array and update the array.
- The read() function takes an integer pointer arr and its size as formal arguments – void read(int *arr, int size)
- It then prompts the user for input values for each element of the array( arr).
- The values are read using scanf() function and stored in the corresponding array( arr) element.
- Call the
print() function and pass the
arr and its size as arguments. The
print() function will print the elements of the array to
- The print function also takes an integer pointer arr and size as formal arguments – void print(int arr[], int size)
- Then it uses a for loop to iterate over each element of the arr , it prints the element’s index and its corresponding value.
Read and Print array using Function Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
/*     Program to read and print the array elements using functions     Author: SillyCodes.com */ #include <stdio.h> const int SIZE = 5; void print(int arr[], int size);  // function delcaration of 'print' function.  /** * @brief - Read input from the user and update 'arr' array *  * @param arr - Array to update * @param size - Size of the arry */ void read(int *arr, int size) {     int i;     printf("Please input array elements \n");     // Read the input from the user     for(i=0; i<size; i++)     {         printf("array[%d] : ", i);         scanf("%d", &arr[i]);     } }  int main() {     int i;     // declare an 'arr'     int arr[SIZE];      // Call the 'read' function to take user input     read(arr, SIZE);         // call the 'print' function to print the data on standard out     print(arr, SIZE);      return 0; }  /** * @brief - Print the elements of the array 'arr' * * @param arr - Array * @param size - size of the array */ void print(int arr[], int size) {     int i;     // print the array elements on console     printf("----- Array Elements --------\n");     for(i = 0; i<size; i++)     {         printf("array[%d] = %d\n", i, arr[i]);     } } |
📢In the above program, We no need to pass the size as the extra parameter to the function, As we already have SIZE global constant.
Program Output:
Compile and run the program
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
$ gcc read-print-func.c $ ./a.out Please input array elements array[0] : 77 array[1] : 33 array[2] : 88 array[3] : 99 array[4] : 11 ----- Array Elements -------- array[0] = 77 array[1] = 33 array[2] = 88 array[3] = 99 array[4] = 11 $ |
Related Programs:
- C Program to Print First n Fibonacci numbers
- C Program to get Nth Fibonacci number
- C Program to Check number is Prime Number or not?
- C Program to Check Prime Number using Square Root (Sqrt) Method
- C Program to Print Prime Numbers between Two numbers
- C Program to print First N Prime numbers
- C Program to generate Prime numbers up to N
- C Program to Calculate Nth Prime Number
- C Program Check Prime Number [Mutliple Methods]
- C Program to Convert Decimal Number to Binary Number
- C Program to Convert Binary Number to Decimal Number
- C Program to Convert Octal Number to Decimal Number
- C Program to Calculate the Factorial of Number
2 Responses
[…] Define a read() function to update the array elements with user-provided values. […]
[…] C Program to Read and Print Arrays […]