C Program to perform arithmetic operations using Switch and If else Statement
- Program Description:
- Program Pre-Requisites:
- Program to Perform Arithmetic Operations:
- Method 1: C Program to Perform arithmetic operations Algorithm using Switch Statement:
- Program: C Program to Perform arithmetic operations Algorithm using Switch Statement:
- Program Output:
- Method 2: C Program to Perform arithmetic operations Algorithm using if else ladder Statement:
- Program Arithmetic Operations with if else ladder:
- Program Output:
- Related Programs:
- C Tutorials Index:
- Program Description:
- Program Pre-Requisites:
- Program to Perform Arithmetic Operations:
- Method 1: C Program to Perform arithmetic operations Algorithm using Switch Statement:
- Program: C Program to Perform arithmetic operations Algorithm using Switch Statement:
- Program Output:
- Method 2: C Program to Perform arithmetic operations Algorithm using if else ladder Statement:
- Program Arithmetic Operations with if else ladder:
- Program Output:
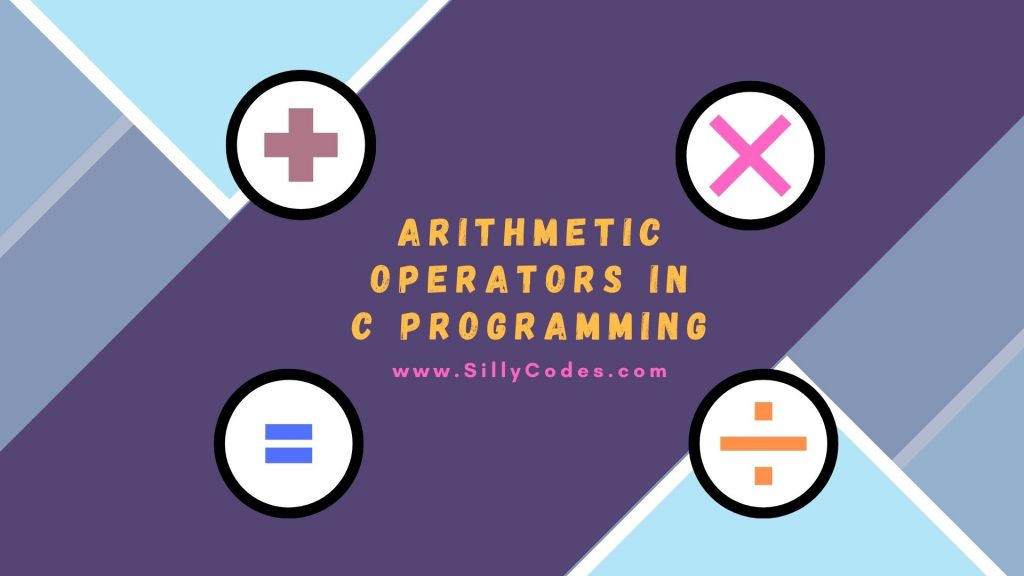
Program Description:
In this article, We are going to write a C Program to perform arithmetic operations using the Switch statement and if else ladder.
The Program should accept Two Numbers and One Operator from the user and perform the arithmetic operations like Addition, Subtraction, Multiplication, Division, and Modulo division on provided numbers. The program should also do the error check and display the error if the user enters the Invalid Input.
Let’s look at a couple of example Inputs and Outputs
Excepted Input and Output:
Example 1:
Input:
Ask the two numbers and Operator from the user.
Enter two Number : 500 1000
Enter the Operator [ +, -, *, /, %] : +
Output:
Addition: 500 + 1000 = 1500
Example 2:
Input:
Enter two Number : 25 5
Enter the Operator [ +, -, *, /, %] : /
Output:
Division: 25 / 5 = 5
Example 3: Invalid Input case:
Input:
Enter two Number : 60 20
Enter the Operator [ +, -, *, /, %] : &
Output:
ERROR: Invalid Operation, Please try again
Program Pre-Requisites:
It is recommended to have knowledge of the switch statement and break statement and arithmetic operations on C. Please go through the following articles to learn more about them.
Program to Perform Arithmetic Operations:
We can write the program using the switch statement and the if else statement. Let’s look at both methods in this program.
Method 1: Perform arithmetic operations using the switch statement
Method 2: Perform arithmetic operations using the if else ladder.
Method 1: C Program to Perform arithmetic operations Algorithm using Switch Statement:
- The Program will start by taking the two numbers from the user and storing them in the variables x and y.
- Then we also ask for the Operator, i.e Addition, Subtraction, etc.
- Users need to specify the + for Addition
- - for Subtraction
- * for product or multiplication
- / for division
- % for modulo division
- Once we got the operator, We are storing it in a variable called op
- Then We are using the switch statement to compare the operator op with available options ( +, -, etc) using switch (op) condition.
- If the user selects the Addition i.e + as the Operation, Then the first case case '+': will be executed and we are calculating the addition and printing output to the console. Make sure to break out of the loop using the break statement.
- Similarly, If the user selects Multiplication operator (*) Then the case '*': will be executed.
- This process continues based on User Input.
- If the user enters Invalid Numbers or the Invalid Operator, Then the Switch statement’s default case will be executed. Which displays the Error Message ERROR: Invalid Operation, Please try again Then we are using the goto INPUT to take the control back to the top of the program. The Goto statement is used to jump from one statement in the program to another.
Let’s convert the above pseudo code into the C program.
Program: C Program to Perform arithmetic operations Algorithm using Switch Statement:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
/* Program: C Program to perform arithmetic operations using switch statement */ #include<stdio.h> int main() { int x, y; // If user provides wrong operator, we are using goto statement // This 'INPUT' is goto LABEL. INPUT: // Take the input from the user // Store them in 'x' and 'y' variables. printf("Enter two Number : "); scanf("%d%d", &x, &y); // Take the operator from the user char op; printf("Enter the Operator [ +, -, *, /, %%] : "); scanf(" %c", &op); // Let's use the 'switch statement' to find operation switch (op) { case '+': // Operator is Addition ('+') printf("Addition: %d + %d = %d \n", x, y, x+y); break; case '-': // Subtraction ('-') printf("Subtraction: %d - %d = %d \n", x, y, x-y); break; case '*': // Product/Mutliplication ('*') printf("Product: %d * %d = %d \n", x, y, x*y); break; case '/': // Division ('/') printf("Division: %d / %d = %d \n", x, y, x/y); break; case '%': // Modulo division ('%') printf("Modulo Division: %d %% %d = %d \n", x, y, x%y); break; default: // Display the Error printf("ERROR: Invalid Operation, Please try again\n"); // Let's use the goto statement to // Take the control back to Operator input statement goto INPUT; break; } return 0; } |
Program Output:
We are using the GCC Compiler to compile and run the program.
$ gcc arithmetic-operations-switch.c
The gcc compiler by default generates the executable file with the a.out Name. So we are going to run the a.out using the ./a.out command. ( As we are in the same directory as where the file is located )
Example 1: Addition test case:
1 2 3 4 |
$ ./a.out Enter two Number : 50 100 Enter the Operator [ +, -, *, /, %] : + Addition: 50 + 100 = 150 |
Example 2: Subtraction:
1 2 3 4 |
$ ./a.out Enter two Number : 30 10 Enter the Operator [ +, -, *, /, %] : - Subtraction: 30 - 10 = 20 |
More Tests: Example 3: Multiplication, Division, and Modulo division:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter two Number : 20 50 Enter the Operator [ +, -, *, /, %] : * Product: 20 * 50 = 1000 $ ./a.out Enter two Number : 25 5 Enter the Operator [ +, -, *, /, %] : / Division: 25 / 5 = 5 $ ./a.out Enter two Number : 20 3 Enter the Operator [ +, -, *, /, %] : % Modulo Division: 20 % 3 = 2 $ |
Example 4: If user enters the wrong number:
1 2 3 4 5 6 7 |
$ ./a.out Enter two Number : # Enter the Operator [ +, -, *, /, %] : ERROR: Invalid Operation, Please try again Enter two Number : 70 20 Enter the Operator [ +, -, *, /, %] : % Modulo Division: 70 % 20 = 10 $ |
Example 5: If the user enters the wrong Operator:
1 2 3 4 5 6 7 8 |
$ ./a.out Enter two Number : 60 20 Enter the Operator [ +, -, *, /, %] : & ERROR: Invalid Operation, Please try again Enter two Number : 60 20 Enter the Operator [ +, -, *, /, %] : + Addition: 60 + 20 = 80 $ |
Method 2: C Program to Perform arithmetic operations Algorithm using if else ladder Statement:
The Program logic is the same as the above method, We are going to take the two numbers and the operator from the user. Now instead of using the switch statement, We are going to use the if else ladder.
So We are going to compare the operator op with arithmetic operations ( addition, subtraction, etc) using the condition like if(op == '+') , if (op == '-'), etc.
If the op is not equal to any of the operations, Then the final else statement will be executed, where we are handling the Invalid Input case.
Program Arithmetic Operations with if else ladder:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
/* Program: C Program to perform arithmetic operations using if else ladder */ #include<stdio.h> int main() { int x, y; // If user provides wrong operator, we are using goto statement // This 'INPUT' is goto LABEL. INPUT: // Take the input from the user // Store them in 'x' and 'y' variables. printf("Enter two Number : "); scanf("%d%d", &x, &y); // Take the operator from the user char op; printf("Enter the Operator [ +, -, *, /, %%] : "); scanf(" %c", &op); // Let's use the 'if else ladder' to compare the operator if(op == '+') { // Operator is Addition ('+') printf("Addition: %d + %d = %d \n", x, y, x+y); } else if (op == '-') { // Subtraction ('-') printf("Subtraction: %d - %d = %d \n", x, y, x-y); } else if (op == '*') { // Product/Mutliplication ('*') printf("Product: %d * %d = %d \n", x, y, x*y); } else if (op == '/') { // Division ('/') printf("Division: %d / %d = %d \n", x, y, x/y); } else if (op == '%') { // Modulo division ('%') printf("Modulo Division: %d %% %d = %d \n", x, y, x%y); } else{ // Display the Error printf("ERROR: Invalid Operation, Please try again\n"); // Let's use the goto statement to // Take the control back to Operator input statement goto INPUT; } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
$ gcc arithmetic-operations-if-else-ladder.c $ ./a.out Enter two Number : 80 70 Enter the Operator [ +, -, *, /, %] : - Subtraction: 80 - 70 = 10 $ ./a.out Enter two Number : 4 9 Enter the Operator [ +, -, *, /, %] : * Product: 4 * 9 = 36 $ ./a.out Enter two Number : 45 7 Enter the Operator [ +, -, *, /, %] : / Division: 45 / 7 = 6 $ ./a.out Enter two Number : 30 4 Enter the Operator [ +, -, *, /, %] : % Modulo Division: 30 % 4 = 2 $ ./a.out Enter two Number : 30 40 Enter the Operator [ +, -, *, /, %] : + Addition: 30 + 40 = 70 $ ./a.out Enter two Number : 90 10 Enter the Operator [ +, -, *, /, %] : & ERROR: Invalid Operation, Please try again Enter two Number : 90 10 Enter the Operator [ +, -, *, /, %] : @ ERROR: Invalid Operation, Please try again Enter two Number : 90 10 Enter the Operator [ +, -, *, /, %] : % Modulo Division: 90 % 10 = 0 $ |
As you can see from the above output, The program is able to do the error check as well and display the Error message on Invalid input.
Related Programs:
- C Program to check character is Alphabet or Number of Special Number
- C Program to Calculate the Minimum of Three Numbers
- C Program to find the Biggest number from Three Numbers (Maximum)
- C Program to calculate the Roots of a Quadratic Equation
- C Program to Calculate the Remainder and Quotient
1 Response
[…] C Program to Perform arithmetic Operations using Switch Statement […]