Swap two numbers without using third variable using XOR (Bitwise)
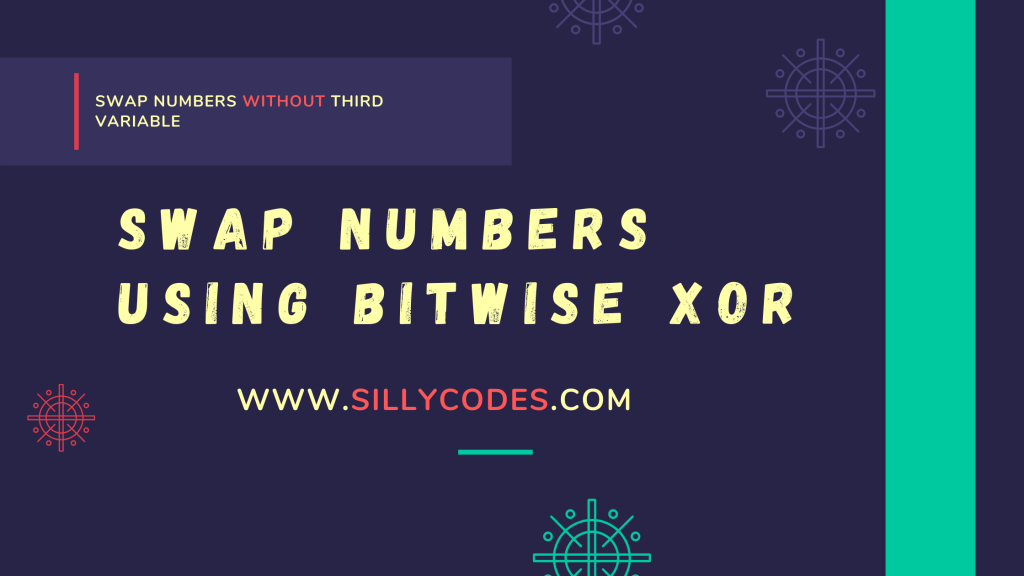
Introduction:
In our previous articles, We have discussed different ways to swap the two numbers. In this article, We are going to discuss the swap two numbers without using third variable here we will use the bitwise XOR Operator to swap numbes.
Swap two numbers without using third variable ( Swap using Bitwise XOR ):
The Bitwise XOR operator gives us the ability to swap the numbers without using third/extra number.
Before going to discuss the swapping using the bitwise XOR operator. Let’s understand how the bitwise XOR works.
Bitwise XOR Truth Table:
Bit A Value | Bit B Value | A ^ B ( A XOR B ) |
---|---|---|
0 | 0 | 0 |
1 | 0 | 1 |
0 | 1 | 1 |
1 | 1 | 0 |
From the truth table, We can say
- The Bitwise XOR operator returns True only if one of the input is 1 ( Other must be 0)
- XOR Return False, If both the inputs are the same.
Swap numbers using XOR Operator Algorithm:
Here is the logic to swap number using XOR operator
1 |
a ^= b ^= a ^= b; |
Above statement is a complex statement, Which is the combination of following statements.
1 2 3 |
a = a ^ b; b = b ^ a; a = a ^ b; |
Here is the explanation about above swap logic
- Step 1, We apply bitwise XOR on variable 'a' and variable 'b'. And assign the result to variable 'a'.
- Step 2: We will apply the bitwise XOR on variable 'b' and variable 'a'. And then assign the result to variable 'b'.
- Step 3: We will perform the bitwise XOR on variable 'a' and variable 'b'. And then assign the result to variable 'a'.
- After performing above three steps, The values of variable ‘a’and variable ‘b’ will be swapped.
Swap two number using XOR operator Example Walkthrough:
Let’s take two variables
'a'and
'b'.
value of
a=11, value of
b=22.
At First step, We will perform
a=a^b;
To apply a^b, We need to have variables 'a'and 'b' values in Binary. And then we will apply the bitwise XOR on them.
- Please note, Bitwise operators operates on the bit level, So we need to imagine the numbers in binary format to understand better.
- To convert Integer to Binary you can following program – Decimal (Integer) to Binary conversion program in C (sillycodes.com)
The Binary Value of a(i.e 11) is 00001011
The Binary value of b(i.e 22) is 00010110
1 2 3 4 5 6 7 |
a=a^b; (first operation) Â 00001011 (variable 'a' or 11 Binary Equivalent) 00010110 (Variable 'b' or 22 Binary Equivalent) --------- 00011101 (Result of (a ^ b) Operation ) -------- |
Now store above result to variable 'a'. So variable a become 00011101
Now let’s perform the second step b = b^a;
1 2 3 4 5 6 7 8 9 |
// The second step // Perform XOR of variable 'b' and variable 'a' b = b^a; Â 00010110 (Value of 'b') 00011101 (Value of variable 'a', Which we got from first step) -------- 00001011 (Result of (b ^ a). Store this result to variable 'b' ) -------- |
Store the Above (b^a) operation result into the variable 'b'. So Variable 'b' became 00001011
Now perform the third step a = a^b;
1 2 3 4 5 6 7 8 9 |
// XOR variable 'a' and Variable 'b' a = a^b; Â Â 00011101 ( Value of 'a', Which we got from step 1 ) 00001011 ( Value of 'b', Which we got from step 2 ) -------- 00010110 Â (Result of (a^b) and store result to variable 'a') -------- |
Save the result of above a^b operation to variable 'a'.
Now the variable 'a' became 00010110, Which is equal to Decimal value 22.
and the variable 'b' became 00001011, Which is equal to decimal value of 11.
As you can see the values of variable 'a' and variable 'b'are swapped. We are able to swap two numbers without using third number.
Program : Swap two numbers without using third variable ( Bitwise XOR ):
Let’s convert above XOR swap logic into the code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
#include<stdio.h> int main() {     // Take two variable, Two variable 'a' and 'b' for input     int a, b;     printf("Enter Two Numbers : ");     scanf("%d%d", &a, &b);      // Let's print the values of 'a' and 'b' before swapping     printf("Before Swapping :: Values of a : %d \t  b : %d \n", a, b);      // In this method, We are going to use XOR operator to swap the numbers.     a ^= b ^= a ^= b;      // Another represntation of same logic     // a ^= b;     // b ^= a;     // a ^= b;      // More readable representation of above logic     // a = a ^ b;     // b = b ^ a;     // a = a ^ b;      // Now the numbers are swapped.     // Let's print the values of 'a' and 'b' After swapping     printf("After Swapping  :: Values of a : %d \t  b : %d \n", a, b);     return 0; } |
Program Output:
We are using GCC compiler to compile the program on Ubuntu Linux.
📢. Learn More above compiling and running program following article – Hello World Program in C language – SillyCodes
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
// Compilling program using GCC compiler venkey@Ubuntu$ gcc swap3.c  // Run the Executable venkey@Ubuntu$ ./a.out Enter Two Numbers : 11 22 Before Swapping :: Values of a : 11      b : 22 After Swapping  :: Values of a : 22      b : 11  // Example 2 venkey@Ubuntu$ ./a.out Enter Two Numbers : 555 444 Before Swapping :: Values of a : 555      b : 444 After Swapping  :: Values of a : 444      b : 555  // Trail 3 venkey@Ubuntu$ ./a.out Enter Two Numbers : 33 999 Before Swapping :: Values of a : 33      b : 999 After Swapping  :: Values of a : 999      b : 33  // Trail 4 venkey@Ubuntu$ ./a.out Enter Two Numbers : 10 20 Before Swapping :: Values of a : 10      b : 20 After Swapping  :: Values of a : 20      b : 10 venkey@Ubuntu$ |
Conclusion:
In this article, We have learned about the Bitwise XOR operator and how it operates with the input data, and We also learned how to swap two number using the bitwise XOR operator ( without using third/extra variable)
Related Articles:
Related Programs:
- Another Way to Swap : Swap Without Third Variable or Temporary Variable – SillyCodes
1 Response
[…] we are using a temp variable to do the swapping, But we can also use Swapping without using the temporary variable method. for simplicity, we are using the temporary variable to swap the […]