C program to SWAP the nibbles of a character | SWAP the nibbles of given character
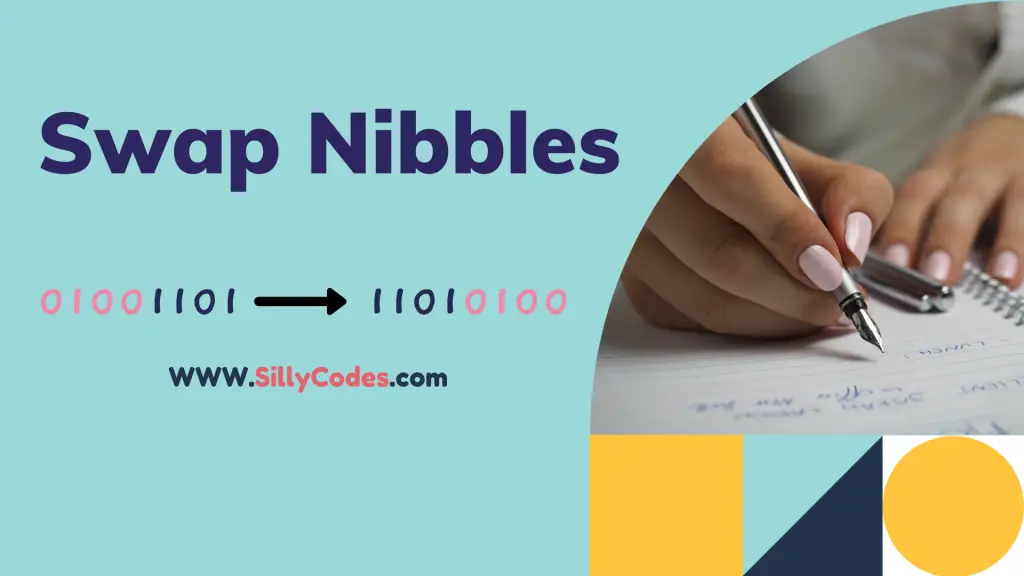
Program Introduction:
Write a C program to swap nibbles of given character.
As the character datatype size is 8 bits. So we need to swap both nibbles (4 bits)
Here are few examples with expected input and output.
Example 1:
Input:
Input an Character.
1 |
M |
Output:
As part of the output, We display the given characters in binary format. Then we swap the nibbles and display the character in Binary format after swapping the nibbles. So that we can easily check if we swapped the nibble properly.
1 2 |
Before Swapping : 01001101 After Swapping  : 11010100 |
Example 2:
Input:
1 |
V |
Output:
1 2 |
Before Swapping : 01010110 After Swapping  : 01100101 |
Example 3:
Input:
1 |
T |
Output:
1 2 |
Before Swapping : 01010100 After Swapping  : 01000101 |
Swap Nibbles Program Logic:
The nibble means 4 bits memory area. The character datatype size is One Byte or 8 bits in the C programming language.
So we need to swap the First four bits with the last four bits.
Let’s look at an example to understand it better. Let’s say the user entered the character ‘ J'.
Then the Binary equivalent of the 'J' is “ 01001010" Here the first nibble of 'J' is "0100" and thesecond nibble of 'J'is "1010". The program should swap these two nibbles.
The expected output after swapping nibbles is "10100100". If you observe the both nibbles are swapped.
The main logic for swap the nibbles is to use the Bitwise right-shift and Bitwise Left-shift operators. Bitwise opertors are useful to do the bit level operation. Like in our present case also we are trying to move the bits.
📌 Learn more about bitwise operators at the following article – Bit wise Operators in C Language – SillyCodes
So we need to right shift the first four bits (Nibble) and left shift the last four bits (Nibble) and then perform the bitwise OR and assign it back to the given number
Here is logic.
1 2 |
ch =Â Â (ch>>4) | (ch<<4); // Here 'ch' is given character |
Swap Nibbles example walk through:
Okay, Above logic might look confusing so lets take a example and go through the step by step.
We will take character 'M'.
The binary representation of 'M'is 01001101
First of all, We need to right shift the first four bits (Nibble) of the 'M'character.
After Right shift first four bits ( 'M' >> 4 ) is 00000100. Remember we are not overriding the original character 'M' here.
Then left shift the last four bits. So ( 'M' << 4 ) becomes 11010000.
Now we need to perform the bitwise OR of the above two values.
i.e
00000100Â Â | 11010000Â Â = 11010100
Now we have successfully swapped the nibbles of character. and our desired output is 11010100
In summary, We need to Bitwise Right shift the first four bits and then Bitwise Left shift the last four bits then perform the bitwise OR.
📢. In the following program, We are using the Character to Binary conversion logic. We will discuss about character to Binary conversion logic in future articles.
Program: Swap Nibbles of Character:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
#include<stdio.h> int main() {     char ch;     int i;      // Take the character from the user     printf("Enter a character : ");     scanf(" %c",&ch);      // Let's print the given character in Binary, So that we can compare later     printf("Before Swapping : ");     for(i=7;i>=0;i--)     {         printf("%d",((ch>>i)&1 ? 1 : 0));     }      // Logic to swap nibbles of characters     // As input is character, We are shifting 4 bits.     // If you want to swap nibbles(double nibbles or swap the bytes) ) of Integer (Short) then shift 8 bits     ch = (ch>>4) | (ch<<4);      // Print the character in Binary after swap     printf("\nAfter Swapping  : ");     for(i=7;i>=0;i--)     {         printf("%d",((ch>>i)&1 ? 1 : 0));     }     printf("\n");  // for new line      return 0; } |
Output:
We are using GCC compiler on Linux Operating system.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
venkey@venkey$ gcc swap-nibbles.c venkey@venkey$ ./a.out Enter a character : M Before Swapping : 01001101 After Swapping : 11010100 venkey@venkey$ gcc swap-nibbles.c venkey@venkey$ ./a.out Enter a character : M Before Swapping : 01001101 After Swapping  : 11010100 venkey@venkey$ ./a.out Enter a character : V Before Swapping : 01010110 After Swapping  : 01100101 venkey@venkey$ ./a.out Enter a character : T Before Swapping : 01010100 After Swapping  : 01000101 venkey@venkey$ ./a.out Enter a character : J Before Swapping : 01001010 After Swapping  : 10100100 venkey@venkey$ ./a.out Enter a character : j Before Swapping : 01101010 After Swapping  : 10100110 venkey@venkey$ |
Conclusion:
In this article, We discussed the technique to swap the nibbles of character. We used bitwise operators to perform the swap. We can even apply the same logic to swap the bits of Integer datatype but what do we need to call for Short integers swapping (double nibble swap or maybe swap the bytes) 😛 ).
2 Responses
[…] C Program to Swap the Nibbles of Character […]
[…] C Program to Swap the Nibbles of Character […]