Count the Number of Vowels, Consonants, Digits, and Special characters in String
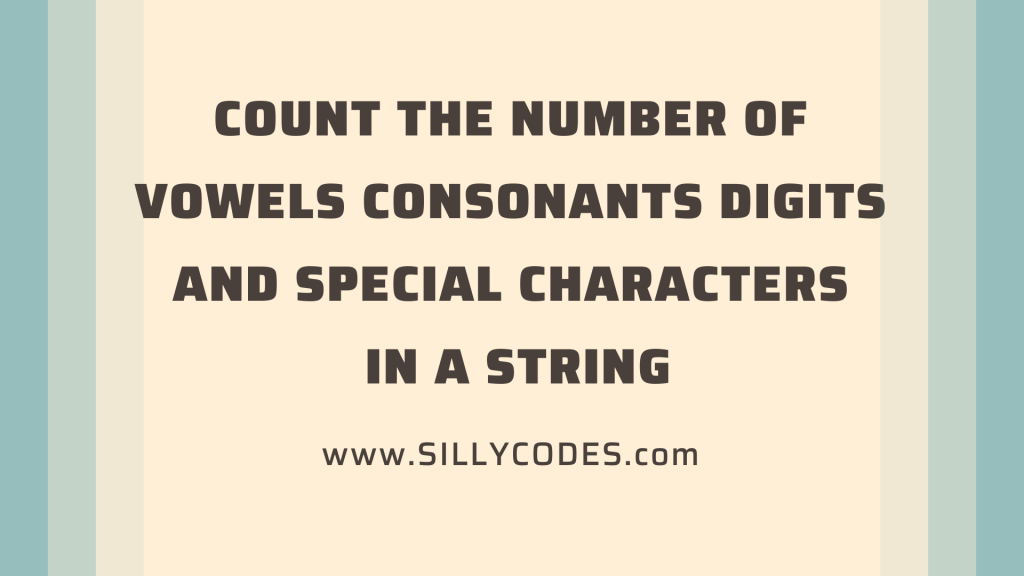
Program Description:
Write a C Program to count the number of vowels, consonants, digits, and special characters in a string. The program should accept an input string from the user and count the number of Vowels, Consonants, Digits, and special characters(i.e other characters) in the input string.
📢 This Program is part of the C String Practice Programs series
Excepted Input and Output:
Input:
1 |
Enter the first string : testing123&*&2 |
Output:
Number of Vowels are 2, consonants are 5, Digits are 4, and Special(Other) Characters are 3
Prerequisites of the Program:
You must know the basics of the strings and arrays in order to better understand the following program. Please go through these articles to learn more about strings and arrays.
- Strings in C Language – Declare, Initialize, and Modify Strings
- C Arrays
- Different ways to Read and Print strings in C
Algorithm Count Number of vowels consonants digits and special characters in a string:
Let’s look at the step-by-step explanation/algorithm of the program.
- Create a Global constant named SIZE and initialize it with 100. This SIZE constant contains the max size of the character array (string)
- Declare the Input string with SIZE size. inputStr[SIZE];
- Prompt the user to provide the input string and update the inputStr string.
- Create four variables to count the number of vowels ( nVowels), number of consonants( nConsonants), number of digits( nDigits), and the number of special characters( nSpecialChars) and initialize with Zero(0).
- To count the vowels, consonants, digits, etc in the string, We need to iterate through all elements(characters) of the string and check each character.
- Start a For loop from
i=0 continue till the
inputStr[i] is not equal to NULL. –
for (i=0; inputStr[i]; i++)
- Count Vowels: Check if the
inputStr[i] is equal to any vowel character i.e ‘a’, ‘e’, ‘i’, ‘o’, ‘u’ ( We need to check both lower case and upper case characters).
- So use the if(ch == 'a' || ch == 'A' || ch == 'e' || ch == 'E' || ch == 'i' || ch == 'I' || ch == 'o' || ch == 'O' || ch == 'u' || ch == 'U') condition.
- If the above condition is True, Then ch i.e inputStr[i] is Vowel Character. So Increment the nVowels variable.
- Count Consonants: Check if the
inputStr[i]/
ch is a valid alphabet. As it is already not a Vowel, Then it must be a consonant.
- User the if( (ch >= ‘a’ && ch <= ‘z’) || (ch >= ‘A’ && ch <= ‘Z’)) condition
- If the above condition is true, Then inputStr[i] / ch is a Consonant. So increment the nConsonant variable.
- Count Digits: Similarly check if the
ch is a digit using the if( (ch >= ‘0’ && ch <= ‘9’)) condition.
- If this condition is true, The ch is a Digit. Increment the nDigits variable.
- Count Other / Special Characters: If any of the above cases are not satisfied, Then the number must be other character or special character. Increment the nSpecialCharacters variable.
- Count Vowels: Check if the
inputStr[i] is equal to any vowel character i.e ‘a’, ‘e’, ‘i’, ‘o’, ‘u’ ( We need to check both lower case and upper case characters).
- Once the above for loop is completed, The nVowels, nConsonants, nDigits, and nSpecialCharacters contain the number of Vowels, Consonants, Digits, and Special characters in the input string.
- Display the results on the console.
Count Number of vowels consonants digits and special characters in string Program:
Convert the above logic to C Program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
/* program to count the number of vowels consonants digits and special characters in a string sillycodes.com */ #include<stdio.h> const int SIZE = 100; int main() { // declare a string with 'SIZE' char inputStr[SIZE]; int i; // get the string from the user printf("Enter the first string : "); // scanf("%s", inputStr); gets(inputStr); // Init the 'nVowels' and 'nConsonants' with Zero int nVowels = 0; int nConsonants = 0; int nDigits = 0; int nSpecialChars = 0; char ch; for(i = 0; inputStr[i]; i++) { ch = inputStr[i]; // check if the character is Vowel // We check if the 'ch' is equal to any of the vowels - 'a', 'e', 'i', 'o', 'u' // We check both Lower and Capital case Vowels with 'ch' if(ch == 'a' || ch == 'A' || ch == 'e' || ch == 'E' || ch == 'i' || ch == 'I' || ch == 'o' || ch == 'O' || ch == 'u' || ch == 'U') { // 'ch' matched - so it is a Vowel nVowels++; } else if( (ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')) { // 'ch' is consonant, Above condition checks if the 'ch' is valid Alphabet. nConsonants++; } else if( (ch >= '0' && ch <= '9')) { // Neither Vowel or consonant. // Digit nDigits++; } else { // Must be special character nSpecialChars++; } } printf("Number of Vowels are %d, consonants are %d, Digits are %d, and Special(Other) Characters are %d\n", nVowels, nConsonants, nDigits, nSpecialChars ); return 0; } |
Program Output:
Let’s compile and Run the Program.
1 2 3 4 5 6 |
$ gcc cnt-vowels-cons.c $ ./a.out Enter the first string : A wise man will make more opportunities than he finds. Total number of Vowels are 18 Number of Vowels are 18, consonants are 26, Digits are 0, and Special(Other) Characters are 10 $ |
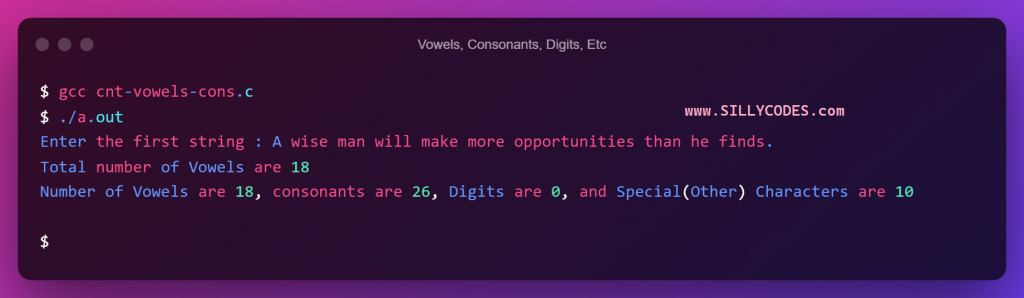
As we can see from the above output, The program is properly counting the vowels, consonants, digits, etc.
Let’s try another example.
1 2 3 4 |
$ ./a.out Enter the first string : testing123&*&2 Number of Vowels are 2, consonants are 5, Digits are 4, and Special(Other) Characters are 3 $ |
Count Number of vowels consonants digits and special characters in string using user-defined functions:
Let’s divide the above program into sub-functions where each function does a specific task. In this case, We can have three functions called isVowel(), isConsonants(), and isDigits() to check the vowels, consonants, and digits respectively.
As we have discussed in the functions tutorial, It is always recommended to divide the large program into functions. Functions improve debugging readability and modularity.
Here is the rewritten version of the above program with user-defined functions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 |
/* program to count the number of vowels consonants digits and special characters in a string sillycodes.com */ #include<stdio.h> const int SIZE = 100; /** * @brief - Check the 'ch' character is a Vowel * * @param ch - character * @return int - 1 if 'ch' is Vowel, otherwise 0 */ int isVowel(char ch) { // check if the character is Vowel if(ch == 'a' || ch == 'A' || ch == 'e' || ch == 'E' || ch == 'i' || ch == 'I' || ch == 'o' || ch == 'O' || ch == 'u' || ch == 'U') { // 'ch' matched - so it is a Vowel return 1; } return 0; } /** * @brief - Check the 'ch' character is a Consonant * * @param ch - character * @return int - 1 if 'ch' is Consonant, otherwise 0 */ int isConsonant(char ch) { if( (ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')) { return 1; } return 0; } /** * @brief - Check the 'ch' character is a digit * * @param ch - character * @return int - 1 if 'ch' is digit, otherwise 0 */ int isDigit(char ch) { if(ch >= '0' && ch <= '9') { return 1; } return 0; } int main() { // declare a string with 'SIZE' char inputStr[SIZE]; int i; // get the string from the user printf("Enter the first string : "); // scanf("%s", inputStr); gets(inputStr); // Init the 'nVowels' and 'nConsonants' with Zero int nVowels = 0; int nConsonants = 0; int nDigits = 0; int nSpecialChars = 0; char ch; for(i = 0; inputStr[i]; i++) { ch = inputStr[i]; // call 'isVowel()' to check if 'ch' is Vowel if(isVowel(ch)) { // 'ch' is Vowel nVowels++; } else if(isConsonant(ch)) { // 'ch' is consonant nConsonants++; } else if(isDigit(ch)) { // 'ch' is a Digit nDigits++; } else { // None of above - special character nSpecialChars++; } } // print the results printf("Number of Vowels: %d\n", nVowels); printf("Number of Consonants: %d\n", nConsonants); printf("Number of Digits: %d\n", nDigits); printf("Number of Other Characters: %d\n", nSpecialChars); return 0; } |
We have defined three user-defined functions(except the main() function) in the above program.
- The
isVowel() Function
- Prototype: int isVowel(char ch)
- The isVowel() function takes a Formal argument i.e ch and checks if the ch is a Vowel.
- It returns 1 if the ch is a Vowel, Otherwise, It returns Zero(0).
- The
isConsonant() Function.
- Prototype: int isConsonant(char ch)
- The isConsonant() function also takes a formal argument it is a character( ch). And verifies if the ch is a Consonant.
- It returns 1 if the ch is a consonant, Otherwise, It returns Zero(0).
- The
isDigit() function.
- Prototype: int isDigit(char ch)
- The isDigit() function also similar to the above two functions and Returns True(1) if the ch is a Digit. Otherwise returns Zero(0).
Program Output:
Let’s Compile and Run the Program using GCC (Any compiler).
1 2 3 4 5 6 7 8 |
$ gcc cnt-vowels-cons-func.c $ ./a.out Enter the first string : Belief creates the actual fact 12345 Number of Vowels: 11 Number of Consonants: 15 Number of Digits: 5 Number of Other Characters: 5 $ |
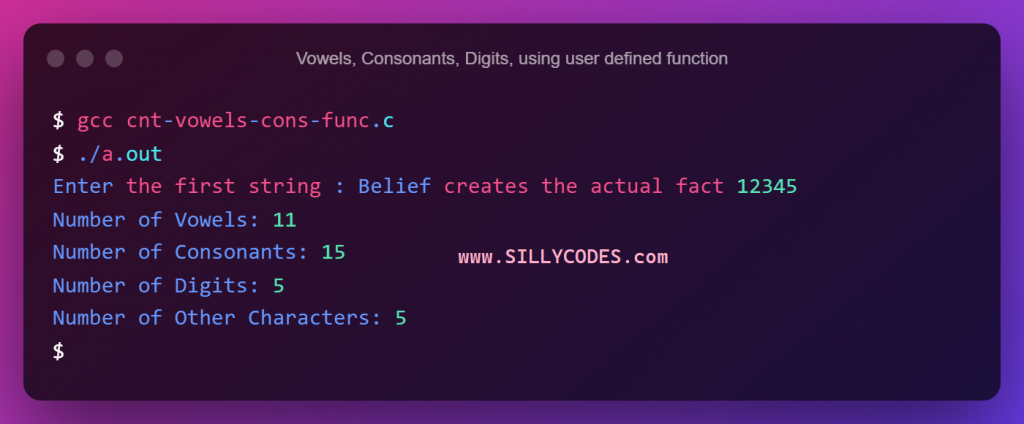
In the input string Belief creates the actual fact 12345, We have 11 Vowels, 15 consonants, 5 Digits, and 5 Other characters.
Exercise:
Rewrite the program using the ctype.h functions like isalpha(), etc.
Related Programs:
- C Programs Index
- C Tutorials Index
- C Program to generate Fibonacci series using Recursion
- C Program to print first N Natural Numbers using Recursion
- C Program to calculate the sum of N Natural Numbers using Recursion
- C Program to calculate the Product of N Natural Numbers using Recursion
- C Program to Count the Number of digits in a Number using Recursion
- C Program to Calculate the Sum of Digits using Recursion
- C Program to Product the Number of digits in a Number using Recursion
- C Program to Reverse a Number using Recursion.
- C Program to check whether the number is Palindrome using Recursion
- C Program to Calculate LCM using Recursion.
1 Response
[…] Count the Number of Vowels, Consonants, Digits, and Special characters in String […]