Check whether the alphabet is Vowel or Consonant in C language
- Program Description:
- Example Input and Output:
- Pre-Requisites:
- Check Vowel or Consonant in C Program Explanation:
- Method 1: Program to check Vowel or Consonant in C Language:
- Program Output:
- Method 2: Program to check vowel or consonant in C Programming:
- Program Output:
- Method 3: Program to check Vowel or Consonant in C using Switch Statement:
- Program Output:
- Related Programs:
- C Tutorials Index:
- Program Description:
- Example Input and Output:
- Pre-Requisites:
- Check Vowel or Consonant in C Program Explanation:
- Method 1: Program to check Vowel or Consonant in C Language:
- Program Output:
- Method 2: Program to check vowel or consonant in C Programming:
- Program Output:
- Method 3: Program to check Vowel or Consonant in C using Switch Statement:
- Program Output:
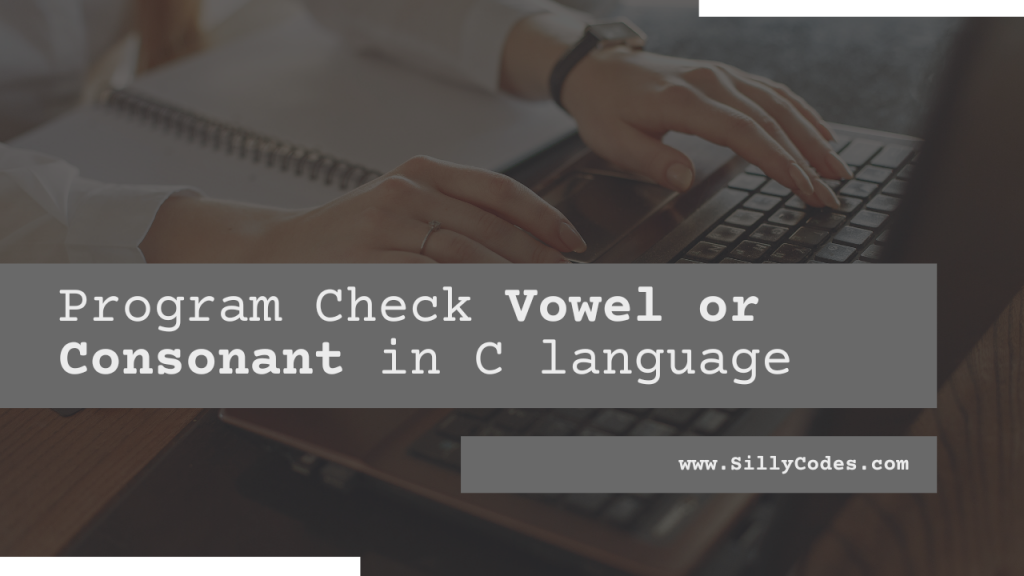
Program Description:
Let’s write a program to check whether the given alphabet is vowel or consonant in C language. We are going to use the if else statement with the comparison operators to check the alphabet
The program should display an error if a value other than the alphabet is entered by the user.
Example Input and Output:
Example 1: Input:
Enter a Character: U
Output:
Given Character U is Vowel
Example 2: Input:
Enter a Character: l
Output:
Given Character l is Consonant
Example 3: Input:
Enter a Character: 10
Output:
Invalid Input : Please enter a Valid alphabet
Pre-Requisites:
We need to have a basic understanding of the if-else statements and the logical operators ( Logical OR and Logical AND)
Check Vowel or Consonant in C Program Explanation:
- We will ask the user to enter a character and store the value in variable ch
- Then we use the if else statement with Logical OR to check whether the ch is Vowel or consonant.
- As we know, if the given character ( ch) is any one of the a , e, i, o, u, Including capital A, E, I, O, U then the character ( ch) is considered as the Vowel.
- We are checking if the ch is equal to any of the above vowel characters using the following logic (ch == 'a' || ch == 'A' || ch == 'e' || ch == 'E' || ch == 'i' || ch == 'I' || ch == 'o' || ch == 'O' || ch == 'u' || ch == 'U') – which is simply comparing the ch with all aeiou characters one by one.
- If above check returns True, Then the character ch is Vowel.
- Otherwise, The given character ch is not Vowel. but we need to check if it valid alphabet.
- To check alphabet, We use the following logic ( (ch >= ‘a’ && ch <= ‘z’) || (ch >= ‘A’ && ch <= ‘Z’)) – We are checking if the ch is in between the a and z ( capital and lower case alphabets)
- If the above condition is True, Then the given character ch is Consonant.
- If the above two conditions are False, Then the character ch is not an Alphabet. It might be number or special character, etc. So display the error message and let the user know about the Invalid Input.
Method 1: Program to check Vowel or Consonant in C Language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
/* Program: Program to check given character is vowel or consonant. */ #include<stdio.h> int main() { char ch; // Take the user input printf("Enter a Character: "); scanf(" %c", &ch); // check if the character is Vowel // We check if the 'ch' is equal to any of the vowels - 'a', 'e', 'i', 'o', 'u' // We check both Lower and Capital case Vowels with 'ch' if(ch == 'a' || ch == 'A' || ch == 'e' || ch == 'E' || ch == 'i' || ch == 'I' || ch == 'o' || ch == 'O' || ch == 'u' || ch == 'U') { // 'ch' matched - so it is a Vowel printf("Given Character %c is Vowel \n", ch); } else if( (ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')) { // 'ch' is consonant, Above condition checks if the 'ch' is valid Alphabet. printf("Given Character %c is Consonant \n", ch); } else { // Invalid Input printf("Invalid Input : Please enter a Valid alphabet \n"); } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
$ gcc Vowels-and-consonant.c $ ./a.out Enter a Character: U Given Character U is Vowel $ ./a.out Enter a Character: i Given Character i is Vowel $ ./a.out Enter a Character: l Given Character l is Consonant $ ./a.out Enter a Character: V Given Character V is Consonant $ ./a.out Enter a Character: 10 Invalid Input : Please enter a Valid alphabet $ ./a.out Enter a Character: ? Invalid Input : Please enter a Valid alphabet $ ./a.out Enter a Character: A Given Character A is Vowel $ |
Method 2: Program to check vowel or consonant in C Programming:
We are going to modify above program, Instead of manually checking the given number is alphabet ( using – ( (ch >= ‘a’ && ch <= ‘z’) || (ch >= ‘A’ && ch <= ‘Z’)) ), We can use the Inbuilt function isalpha() from the ctype.h headerfile.
Syntax of isalpha function:
The isalpha() function is available in the ctype.h library and isalpha() function takes a character as input and returns ‘ True if it is an Alphabet
Let’s change the above program and re-write using the isalpha() function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
/* Program: Program to check given character is vowel or consonant. */ #include<stdio.h> #include<ctype.h> int main() { char ch; // Take the user input printf("Enter a Character: "); scanf(" %c", &ch); // By using the 'isalpha()' functionWe will check if the given character 'ch' is alphabet // The 'isalpha()' function is available in the ctype.h library // 'isalpha()' function takes character as input and return 'true' if it is alphabet. if(isalpha(ch)) //is alpha available in ctype.h { // Given character 'ch' is Alphabet // check if the character is Vowel // We check if the 'ch' is equal to any of the vowels - 'a', 'e', 'i', 'o', 'u' // We check both Lower and Capital case Vowels with 'ch' if(ch == 'a' || ch == 'A' || ch == 'e' || ch == 'E' || ch == 'i' || ch == 'I' || ch == 'o' || ch == 'O' || ch == 'u' || ch == 'U') { // 'ch' matched - so it is a Vowel printf("Given Character %c is Vowel \n", ch); } else { // 'ch' is consonant, Above condition checks if the 'ch' is valid Alphabet. printf("Given Character %c is Consonant \n", ch); } } else { // Invalid Input printf("Invalid Input : Please enter a Valid alphabet \n"); } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
$ gcc Vowels-and-consonant.c $ ./a.out Enter a Character: E Given Character E is Vowel $ ./a.out Enter a Character: l Given Character l is Consonant $ ./a.out Enter a Character: I Given Character I is Vowel $ ./a.out Enter a Character: Z Given Character Z is Consonant $ ./a.out Enter a Character: ? Invalid Input : Please enter a Valid alphabet $ ./a.out Enter a Character: 45 Invalid Input : Please enter a Valid alphabet $ |
Method 3: Program to check Vowel or Consonant in C using Switch Statement:
We have earlier discussed that, If you don’t specify the break after each case, The subsequent case also executes. This often causes bugs but it is also useful to create operator OR like behavior.
To understand better let’s look at the following program.
We are going to use the switch case to detect the Vowels and Consonants.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
/* Program to detect Vowels or Consonants */ #include <stdio.h> #include <ctype.h> int main(void) { // Take a alphabet from user char ch; printf("Enter alphabet(Lower case): "); scanf(" %c", &ch); // By using the 'isalpha()' functionWe will check if the given character 'ch' is alphabet // The 'isalpha()' function is available in the ctype.h library // 'isalpha()' function takes character as input and return 'true' if it is alphabet. if(isalpha(ch)) //is alpha available in ctype.h { // Switch will check the given alphabet with all cases switch(ch) { case 'a': case 'e': case 'i': case 'o': case 'u': case 'A': case 'E': case 'I': case 'O': case 'U': // if you note, we have skipped the 'break' statement for above all cases // which means if 'ch' matches any of the above characters, The following printf will executes. printf("Given alphabet %c is Vowel \n", ch); break; default: printf("Given alphabet %c is consonant \n", ch); break; } } else { // Invalid Input printf("Invalid Input : Please enter a Valid alphabet \n"); } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
$ gcc vowels-and-consonants-switch.c $ ./a.out Enter alphabet(Lower case): u Given alphabet u is Vowel $ ./a.out Enter alphabet(Lower case): I Given alphabet I is Vowel $ ./a.out Enter alphabet(Lower case): m Given alphabet m is consonant $ ./a.out Enter alphabet(Lower case): V Given alphabet V is consonant $ ./a.out Enter alphabet(Lower case): ^ Invalid Input : Please enter a Valid alphabet $ ./a.out Enter alphabet(Lower case): a Given alphabet a is Vowel $ |
We used isalpha() from ctypes.h and the switch case to check the vowels and consonants.
As you can see from the code, We have intentionally not used break statement after the cases a, e, i , o and u and upper case A, E, I, O, and Finally used break statement after the case U. So It behaves like a OR operation.
We can check for vowel and consonant alphabets using if else check also
/* Check if input alphabet is member of set{A,E,I,O,U,a,e,i,o,u} */
if(c == ‘a’ || c == ‘e’ || c ==’i’ || c==’o’ || c==’u’ || c==’A’
|| c==’E’ || c==’I’ || c==’O’ || c==’U’){
printf(“%c is a Vowel\n”, c);
} else {
printf(“%c is a Consonant\n”, c);
}