Merge Two Arrays in C Programming Language
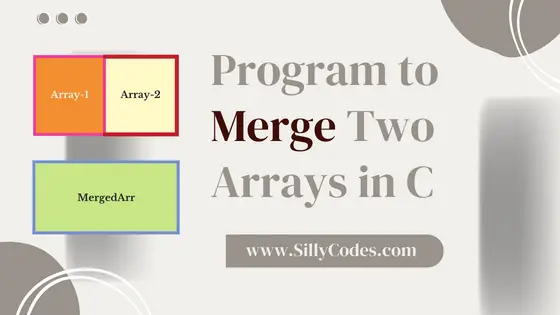
Program Description:
In our previous article, We have looked at the program to Delete All duplicate elements in a sorted array. In today’s article, We are going to write a Program to Merge Two Arrays in C programming language. The program should accept two arrays from the user and merge them.
📢 This Program is part of the Array Practice Programs Collection.
Here are the excepted Input and Output of the program
Example Input and Output:
Input:
Enter desired size for array1 (1-100): 5
Please enter array elements(5) : 12 34 74 89 21
Enter desired size for array2 (1-100): 8
Please enter array elements(8) : 100 200 300 600 700 800 900 1000
Output:
Array-1: 12 34 74 89 21
Array-2: 100 200 300 600 700 800 900 1000
Merged Array: 12 34 74 89 21 100 200 300 600 700 800 900 1000
As you can see from the above example input and output, The program prompts for the Array-1 Size and Array-1 elements, Then Array-2 size and elements, Finally it merges both arrays and prints the results on the console.
Prerequisites:
We need to know the C Arrays and Functions to better understand the following program.
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- How to pass arrays to functions in C
- Functions in C Language
Algorithm to Merge Two Arrays in C:
First, we need to take the two input arrays from the user, Let the two input arrays are arr1 and arr2. and Then We need to create a third array which should be large enough to hold the arr1 and arr2. Let’s say the third array name as the mergedArr.
To Merge the two arrays, We will go through the all elements of the first array ( arr1) and copy them the mergedArr element by element using a For Loop. Once the first array is copied to mergedArr. Then we will go through the second input array( arr2), and Appends the arr2 elements to the mergedArr using another For Loop.
Once the above two for loops are completed, the mergedArr will contain the Merged Array.
Merge Two Arrays in C Program Explanation:
Here is a step-by-step explanation of the program.
- Declare two input arrays with the max size of 100, They are arr1[100] and arr2[100]. Also, declare another array that is going to hold the merged array, Call the third array as the mergedArr, The mergedArrsize is 200 ( Sum of both arr1 and arr2 sizes).
- We defined two helper functions, which are
read() and
display() functions. These functions are useful to read and print arrays.
- The read() function takes an array as formal arguments and reads the elements from the user and updates the given array.
- The display() function used to print the elements of the given array.
- Now, We need to take the input arrays ( arr1 and arr2) sizes and elements from the user.
- So prompt the user to provide size for the arr1, Then call the read() function with the arr1 and size as the actual arguments. – read(arr1, size);. This function call to read() function updates the arr1 with user values.
- Repeat the above step-3 and step-4 steps for the arr2 to get the arr2size and elements from the user.
- We got the two input arrays from the user. Display the
arr1 and
arr2 by using the
display() function. So make two calls to
dispaly() function from the
main() function and provide the
arr1 details for one function call,
arr2 details for another function call.
- display(arr1, size1);
- display(arr2, size2);
- To Merge the two arrays, Use two for loops and go through the elements of the both arr1 and arr2 and copy them to mergedArr array.
- We will traverse through all elements of the
arr1 using a
For Loop and copy all elements to the
mergedArr.
- Start a For Loop from i=0 and go till i<size1
- Copy the arr1[i] element to mergedArr[i] – mergedArr[i] = arr1[i];
- Similarly, Go through the elements of the
arr2 and copy them to
mergedArr.
- Start a For Loop with (i = size1, j = 0) and go till the (i < (size1+size2) && j<size2)
- Copy the arr2[i] to mergedArr[i] element – mergedArr[i] = arr2[j];
- Display the Merged Array on the console by calling the display() function – display(mergedArr, size1+size2);
Program to Merge Two Arrays in C Language:
Let’s convert the above Algorithm in the C Program – Here is the program to Merge Two Arrays in C Programming Langauge.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 |
/* Program Merge two arrays and create third Array Author: SillyCodes.com */ #include <stdio.h> /** * @brief - Read the user input and update the 'arr' array * * @param arr - 'arr' array * @param size - size of the 'arr' array */ void read(int arr[], int size) { int i; // User input for array elements printf("Please enter array elements(%d) : ", size); for(i = 0; i < size; i++) { // printf("arr[%d] : ", i); scanf("%d", &arr[i]); } } /** * @brief - Display the 'arr' array * * @param arr - Array * @param size - Array size */ void display(int arr[], int size) { int i; for(i = 0; i < size; i++) { printf("%d ", arr[i]); } printf("\n"); } int main() { // declare the 'arr1', 'arr2', and 'mergedArr' array int arr1[100], arr2[100], mergedArr[200]; int i, j, size1, size2; // Take the Array1('arr1') details from the user printf("Enter desired size for array1 (1-100): "); scanf("%d", &size1); // check for array bounds if(size1 >= 100 || size1 <= 0) { printf("Invalid Size, Max Array size reached, Try again \n"); return 0; } // User input for array elements read(arr1, size1); // Take the Array2('arr2') values from the user. printf("Enter desired size for array2 (1-100): "); scanf("%d", &size2); // check for array bounds if(size2 >= 100 || size2 <= 0) { printf("Invalid Size, Max Array size reached, Try again \n"); return 0; } // Take User input for array elements read(arr2, size2); // Print the 'arr1' array printf("Array-1: "); display(arr1, size1); // Print the 'arr2' array printf("Array-2: "); display(arr2, size2); // Merge the two arrays. // First of all, Copy 'arr1' elements to 'mergedArr' array for(i = 0; i<size1; i++) { mergedArr[i] = arr1[i]; } // Append the 'arr2' elements to 'mergedArr' array. for(i = size1, j = 0; i < (size1+size2) && j<size2; i++, j++) { mergedArr[i] = arr2[j]; } // Print the merged Array printf("Merged Array: "); display(mergedArr, size1+size2); return 0; } |
Program Output:
Compile and Run the Program.
To compile the program use the following command.
gcc merge-two-arrays.c
The above command, Generates an executable file named a.out. Run the executable file.
Test 1:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$ ./a.out Enter desired size for array1 (1-100): 10 Please enter array elements(10) : 1 2 3 4 5 6 7 8 9 10 Enter desired size for array2 (1-100): 5 Please enter array elements(5) : 55 66 77 88 99 Array-1: 1 2 3 4 5 6 7 8 9 10 Array-2: 55 66 77 88 99 Merged Array: 1 2 3 4 5 6 7 8 9 10 55 66 77 88 99 $ ./a.out Enter desired size for array1 (1-100): 5 Please enter array elements(5) : 12 34 74 89 21 Enter desired size for array2 (1-100): 8 Please enter array elements(8) : 100 200 300 600 700 800 900 1000 Array-1: 12 34 74 89 21 Array-2: 100 200 300 600 700 800 900 1000 Merged Array: 12 34 74 89 21 100 200 300 600 700 800 900 1000 $ |
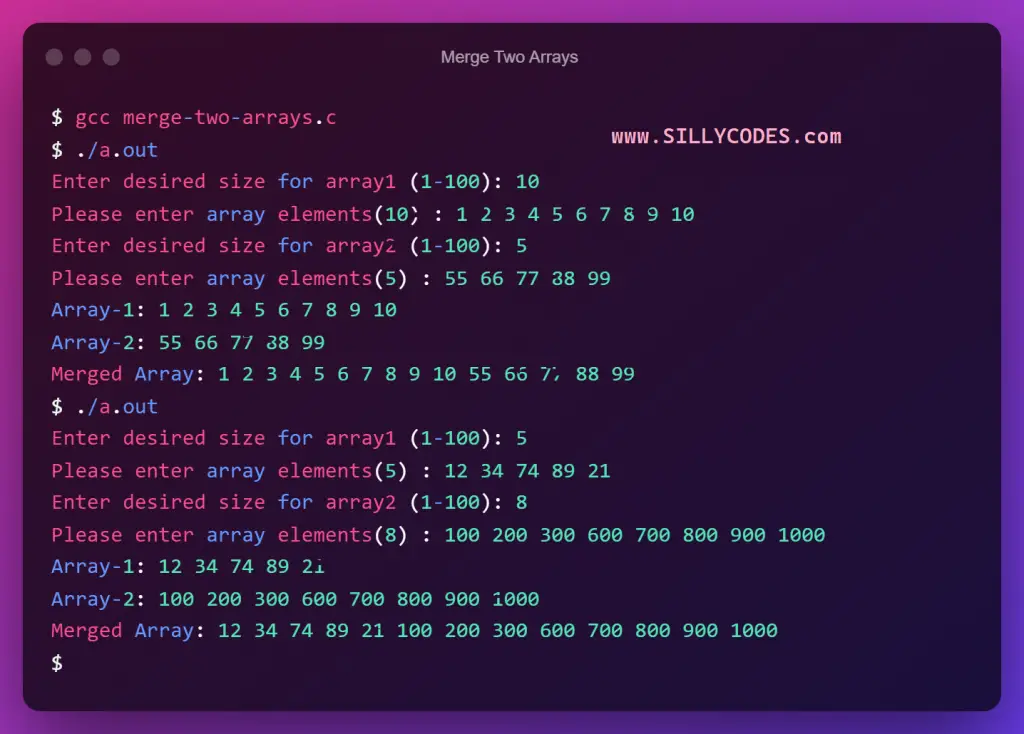
As we can see from the above output, The program is properly merging the two input arrays and creating the new one.
For example, When we entered the two arrays 1 2 3 4 5 6 7 8 9 10 and 55 66 77 88 99, Then the program merged the arrays and provided the 1 2 3 4 5 6 7 8 9 10 55 66 77 88 99 array as output.
Test 2: Negative Test Case:
When a user enters an invalid size.
1 2 3 4 5 6 7 8 9 10 |
// Negative test Case $ ./a.out Enter desired size for array1 (1-100): 200 Invalid Size, Max Array size reached, Try again $ ./a.out Enter desired size for array1 (1-100): 3 Please enter array elements(3) : 1 2 3 Enter desired size for array2 (1-100): 0 Invalid Size, Max Array size reached, Try again $ |
If the user enters the wrong size for any of the input arrays ( arr1 or arr2), Then the program is displaying an error message( Invalid Size, Max Array size reached, Try again).
Related Programs:
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Delete Duplicate Elements from Sorted Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
- C Program to Count Number of Unique Elements in Array
1 Response
[…] have covered the Merging of Two unsorted arrays in our earlier articles. In today’s article, We will look at a Program to Merge Two Sorted Arrays […]