check character is uppercase or lowercase in C language
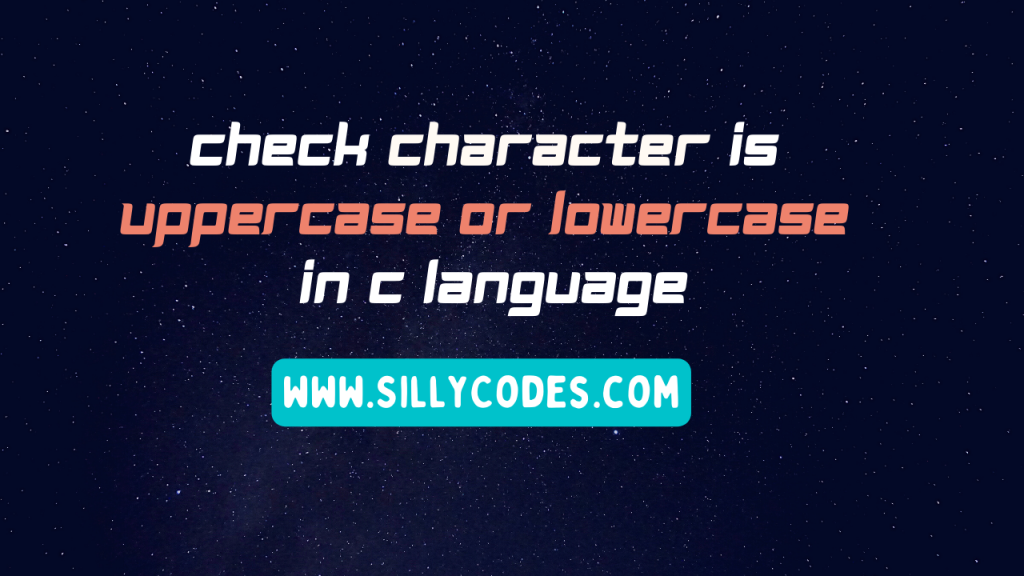
Check Character is Uppercase or Lowercase Program Description:
Write a C Program to check character is uppercase or lowercase using the if else statement.
The program should accept a character from the user and display whether the character is Lower case or Uppercase character.
Excepted Input and Output:
Example 1: Input:
Enter a character : m
Output:
m is Lower Case Character
Example 2: Input:
Enter a character : V
Output:
V is Upper case character
Example 3: Input:
Enter a character : 8
Output:
Invalid Input, Please enter valid Alphabet
Pre-Requisites:
It is recommended to know the understanding of if else statement and goto statements
please look at the following articles for more details
- Decision making statements if and if…else in C
- Nested if else and if..else ladder in C-Language
- Goto Statement in C Language
Program Explanation:
We can use two different methods to check the case of the alphabet
- Using Predefined functions like islower() and isupper()
- Using Manual ASCII Checks
Method 1: Check character is uppercase or lowercase using the predefined functions:
- We can use predefined functions like islower() and isupper() from the ctype.h header file.
- We use the if else statement with the islower() and isupper() functions.
- The islower(ch) function will check the character ch and returns true if the ch is Lower Case Character
- The isupper(ch) function will check the character ch and returns true if the ch is Upper Case Character
- If the above two conditions are False, Then the given character ch is not a valid Alphabet. So we display the error. And takes the controls back to the start of the program using the goto statement.
Check character is uppercase or lowercase in C Language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
#include<stdio.h> #include<ctype.h> int main() { INPUT: // Take the input from the User char ch; printf("Enter a character : "); scanf(" %c", &ch); // use 'islower()' inbuilt function from 'ctype.h' headerfiles if(islower(ch)) { // 'ch' is Lower Case printf("%c is Lower Case Character\n", ch); } else if (isupper(ch)) { // 'ch' is Upper case printf("%c is Upper case character \n", ch); } else { // Invalid Input, display the error // Let's take the control back to start of the program using the goto statement printf("Invalid Input, Please enter valid Alphabet \n"); goto INPUT; } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ gcc lower-upper-alphabet.c $ ./a.out Enter a character : m m is Lower Case Character $ ./a.out Enter a character : V V is Upper case character $ ./a.out Enter a character : 8 Invalid Input, Please enter valid Alphabet Enter a character : Q Q is Upper case character $ |
Method 2: Check whether the character is uppercase or lowercase Using Manual ASCII Checks
In this method, We manually compare the ASCII value of the given character ch manually with the lower case characters and upper case characters ASCII Values.
If the character ch appears between the lowercase characters a and z, Then ch is Lower Case character. We can use the following logic to check it – (ch >= ‘a’ && ch <= ‘z’)
Similarly, We can check for upper case letters as well, If the ch ASCII value is in between the A and Z ASCII values. We are using the following logic to check the upper case character. (ch >= ‘A’ && ch <= ‘Z’)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
/* Program: Check whether given character is lowercase or uppercase */ #include<stdio.h> int main() { INPUT: // Take the input from the User char ch; printf("Enter a character : "); scanf(" %c", &ch); // if the 'ch' is betwen the ASCII character 'a' and 'z' if( (ch >= 'a' && ch <= 'z')) { // 'ch' is Lower Case printf("%c is Lower Case Character\n", ch); } else if ((ch >= 'A' && ch <= 'Z')) { // 'ch' is Upper case printf("%c is Upper case character \n", ch); } else { // Invalid Input, display the error // Let's take the control back to start of the program using the goto statement printf("Invalid Input, Please enter valid Alphabet \n"); goto INPUT; } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
$ gcc lower-upper-alphabet.c $ ./a.out Enter a character : C C is Upper case character $ ./a.out Enter a character : l l is Lower Case Character $ ./a.out Enter a character : R R is Upper case character $ ./a.out Enter a character : ? Invalid Input, Please enter valid Alphabet Enter a character : q q is Lower Case Character $ |
1 Response
[…] C Program to check character is Uppercase or Lowercase […]