Program to find Second Largest Element in Array in C
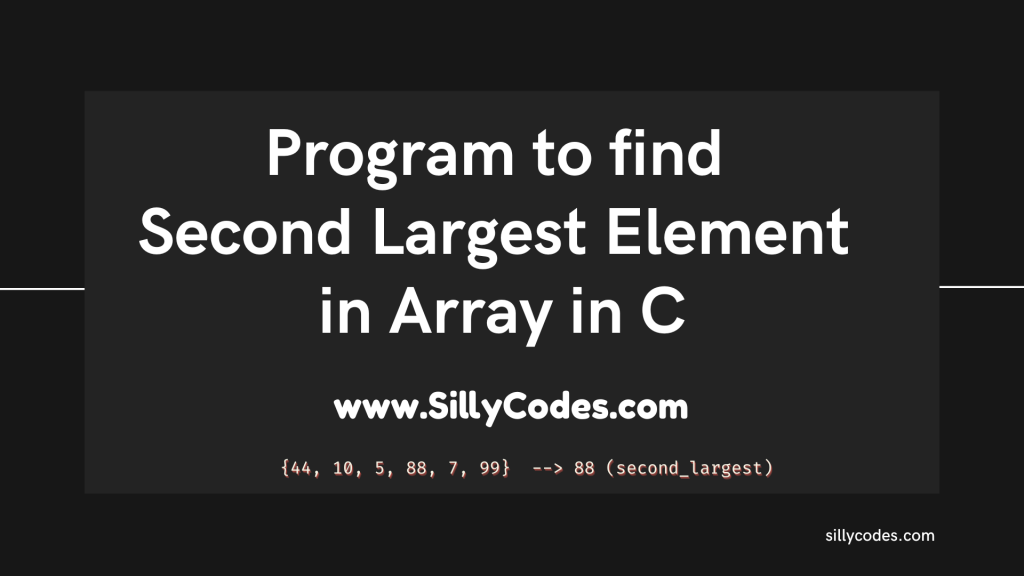
- Second Largest Element in Array in C Program Explanation:
- Second Largest Element in Array in C Program Algorithm:
- Program to find Second Largest Element in Array in C:
- Largest and Second Largest Element with using Custom Array:
- Program to find Second Largest Element in Array in C using Functions:
- Exercise: Sort the array and Get the last and last-second numbers:
Program Description:
Write a Program to find the Second Largest Element in Array in C programming language. We have looked at the Minimum number in the array and Maximum Element in Array programs in earlier articles, In today’s article, We will look at the Second Largest element in the given array.
Example Inputs and Outputs:
Input:
1 2 3 4 5 6 |
Please enter array elements numbers[0] : 83 numbers[1] : 23 numbers[2] : 84 numbers[3] : 47 numbers[4] : 29 |
Output:
1 2 |
Largest element in array is : 84 Second Largest element in array is : 83 |
Here we have provided the Largest and Second Largest Numbers as output.
Prerequisites:
We need to know the basics of the Arrays and functions in C to better understand the program. Please go through the following articles to learn more.
- Introduction to C Arrays – How to Declare, Access, and Modify Arrays
- Functions in C with Example Programs
- How to pass arrays to functions in C
Second Largest Element in Array in C Program Explanation:
Here is the explanation to find the largest and second largest number in the array Program.
- First of all, Declare a numbers array, The numbers array holds 5 elements. Here we have hardcoded the size but you can also use a constant( const) or Macro.
- Take the input from the user and update the numbers[] array elements.
- Then, create two variables, largest and second_largest, and initialize them with the INT_MIN. ( Learn more about the INT_MIN at Size and Limits of datatypes in C)
- Iterate through all array elements
- Update the second_largest and largest variables if you find a number that is larger than the present largest (i.e ( numbers[i] > largest)). First, update the second_largest with largest, Then update the largest with number[i].
- If you find any number which is smaller than largest number and greater than the second_largest ( i.e (numbers[i] > second_largest && numbers[i] < largest)) Then update the second_largest number with number[i].
- Once the above loop is completed, The largest and second_largest variables hold the largest and second largest numbers correspondingly.
- Finally, display the largest and second_largest numbers on the console.
Second Largest Element in Array in C Program Algorithm:
- Start
- Declare numbers array – int numbers[5];.
- Update the array with user input.
- Iterate from 0 to 4 and update &numbers[i] using scanf() function.
- Create largest and second_largest. Initialize with INT_MIN.
- Iterate over array elements and update the
largest and
second_largest elements.
- Iterate from 0 to 4 ( size-1).
- If numbers[i] > largest, Then update second_largest = largest; and largest = numbers[i];
- else if(numbers[i] > second_largest && numbers[i] < largest), Then update the second_largest = numbers[i];
- Print the largest and second_largest using printf.
Program to find Second Largest Element in Array in C:
Here is the C Program to calculate the Largest and Second Largest element in C
📢 As we are using the INT_MIN, We need to include the limits.h header file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
/*     Program to find Second largest element of Array     sillycodes.com */ #include <stdio.h> #include <limits.h>  int main() {     // declare the 'numbers' array     int numbers[5];     int i;      // User input for array elements     printf("Please enter array elements\n");     for(i = 0; i < 5; i++)     {         printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     }         // initialize the 'largest', 'second_largest' variable with 'INT_MIN' from limits.h file     // Look at - https://sillycodes.com/size-and-limits-datatypes-in-c-programming/ for info.     int largest = INT_MIN;     int second_largest = INT_MIN;         // find the largest and second_largest of array elements     for(i = 0; i < 5; i++)     {         // check for 'largest'         if( numbers[i] > largest)         {             // numbers[i] is largest. so update 'second_largest' with present 'largest'             second_largest = largest;             largest = numbers[i];         }         else if(numbers[i] > second_largest && numbers[i] < largest)         {             // update the second_largest             second_largest = numbers[i];         }     }      // print the result     printf("Largest element in array is : %d\n", largest);     printf("Second Largest element in array is : %d\n", second_largest);      return 0; } |
Program Output:
Compile and Run the Program using GCC.
Test Case 1: Positive Numbers:
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc second_largest.c $ ./a.out Please enter array elements numbers[0] : 83 numbers[1] : 23 numbers[2] : 84 numbers[3] : 47 numbers[4] : 29 Largest element in array is : 84 Second Largest element in array is : 83 $ |
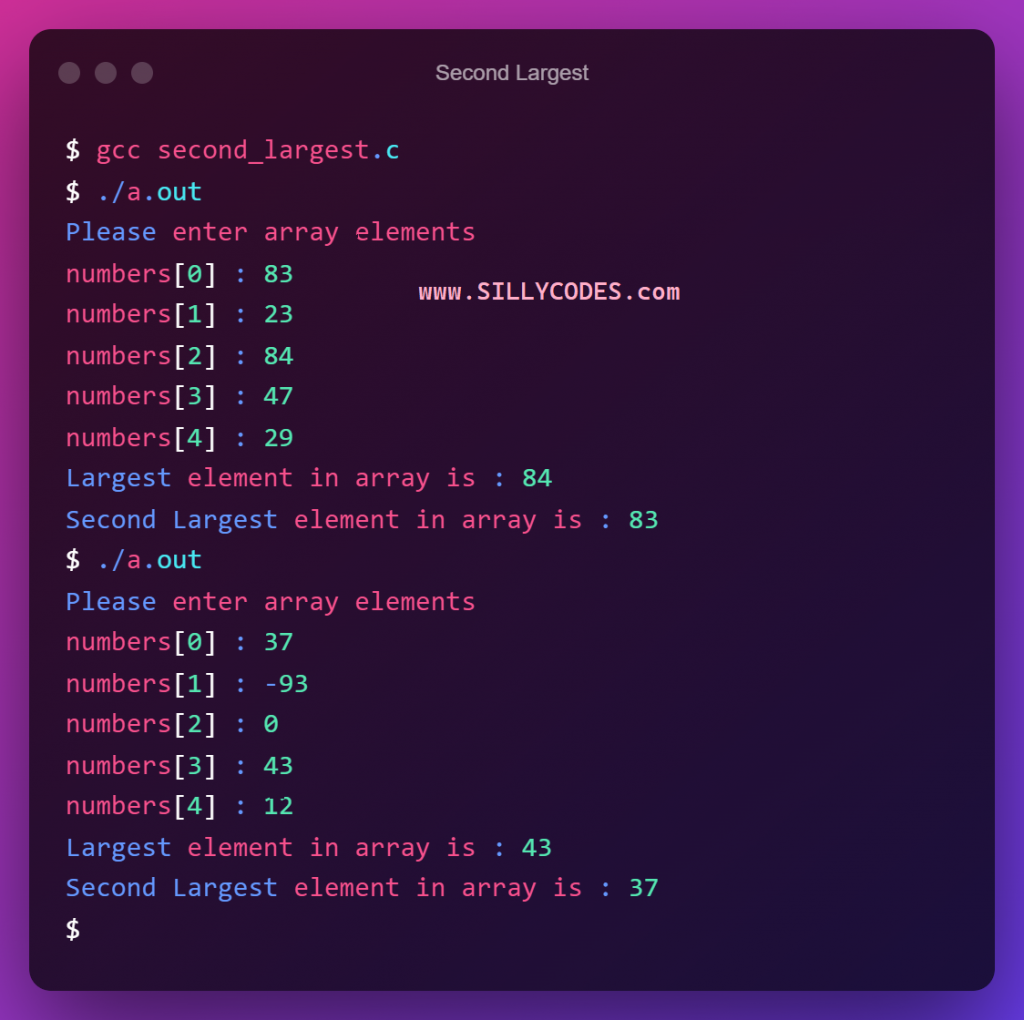
Test Case 2: Negative Numbers and Zeros:
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Please enter array elements numbers[0] : 37 numbers[1] : -93 numbers[2] : 0 numbers[3] : 43 numbers[4] : 12 Largest element in array is : 43 Second Largest element in array is : 37 $ |
Largest and Second Largest Element with using Custom Array:
In the above program, We have hard-coded array size as 5. Let’s create a large array and allow the user to specify the required size.
Modify the above program to accept the array size from the user. Note: This is not a dynamic array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
/*     Program to find Second largest element of Array     sillycodes.com */ #include <stdio.h> #include <limits.h>  int main() {     // declare the 'numbers' array     int numbers[100];     int i, size;      printf("Enter desired array size(1-100): ");     scanf("%d", &size);      // User input for array elements     printf("Please enter array elements\n");     for(i = 0; i < size; i++)     {         printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     }         // initialize the 'largest', 'second_largest' variable with 'INT_MIN' from limits.h file     // Look at - https://sillycodes.com/size-and-limits-datatypes-in-c-programming/ for info.     int largest = INT_MIN;     int second_largest = INT_MIN;         // find the largest and second_largest of array elements     for(i = 0; i < size; i++)     {         // check for 'largest'         if( numbers[i] > largest)         {             // numbers[i] is largest. so update 'second_largest' with present 'largest'             second_largest = largest;             largest = numbers[i];         }         else if(numbers[i] > second_largest && numbers[i] < largest)         {             // update the second_largest             second_largest = numbers[i];         }     }      // print the result     printf("Largest element in array is : %d\n", largest);     printf("Second Largest element in array is : %d\n", second_largest);      return 0; } |
Program Output:
Let’s compile and run the C Program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
$ gcc second_largest.c $ ./a.out Enter desired array size(1-100): 10 Please enter array elements numbers[0] : 2 numbers[1] : 39 numbers[2] : 23 numbers[3] : 0 numbers[4] : -45 numbers[5] : 75 numbers[6] : 34 numbers[7] : 199 numbers[8] : 38 numbers[9] : 48 Largest element in array is : 199 Second Largest element in array is : 75 $ ./a.out Enter desired array size(1-100): 7 Please enter array elements numbers[0] : 23 numbers[1] : 43 numbers[2] : 28 numbers[3] : 98 numbers[4] : 27 numbers[5] : 45 numbers[6] : 23 Largest element in array is : 98 Second Largest element in array is : 45 $ |
Program to find Second Largest Element in Array in C using Functions:
We have used only one function (i.e main()) in the above programs. So let’s divide the program and use the C Functions.
The functions improve the code reusability and readability. ( Advantages of Functions )
Let’s rewrite the above program and create two functions named read() and get_second_largest()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 |
/*     Program to find Second largest element of Array     sillycodes.com */ #include <stdio.h> #include <limits.h>  /** * @brief  - Read the elements from the user and update 'numbers' array * * @param numbers  - 'numbers[]' array * @param size    - size of the 'numbers' array */ void read(int numbers[], int size) {     int i;     printf("Please enter array elements\n");     // Read the input from the user     for(i = 0; i < size; i++)     {         printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     } }  /** * @brief Get the second largest element from the 'numbers' array * * @param numbers - Array * @param size    - size of the 'numbers' array * @return int    - second largest element */ int get_second_largest(int numbers[], int size) {     int i;     // initialize the 'largest', 'second_largest' variable with 'INT_MIN' from limits.h file     // Look at - https://sillycodes.com/size-and-limits-datatypes-in-c-programming/ for info.     int largest = INT_MIN;     int second_largest = INT_MIN;         // find the largest and second_largest of array elements     for(i = 0; i < size; i++)     {         // check for 'largest'         if( numbers[i] > largest)         {             // numbers[i] is largest. so update 'second_largest' with present 'largest'             second_largest = largest;             largest = numbers[i];         }         else if(numbers[i] > second_largest && numbers[i] < largest)         {             // update the second_largest             second_largest = numbers[i];         }     }      // return the 'second_largest' element     return second_largest; }  int main() {     // declare the 'numbers' array     int numbers[100];     int i, size;      // Take the array size from the user     printf("Enter desired array size(1-100): ");     scanf("%d", &size);      // call 'read()' to read numbers from user and update array     read(numbers, size);          // call get_second_largest() function to get the second largest element     int second_largest = get_second_largest(numbers, size);         // print the result     printf("Second Largest element in array is : %d\n", second_largest);      return 0; } |
We have defined two functions here.
- The
read() function.
- Prototype of the read() function – void read(int numbers[], int size);
- The read function takes two arguments – numbers array and its size. The read() function prompts the user for array elements and updates the number array.
- The
get_second_largest() function.
- Prototype of the get_second_largest() function – int get_second_largest(int numbers[], int size);
- The get_second_largest() function also takes two formal arguments one is the input array numbers[] and the sizeof the number’s array.
- The get_second_largest() function finds the second largest element in the array by comparing all elements.
- Finally, It returns the Second Largest element in the array back to the caller.
We called the read() and get_second_largest() functions from the main() function.
📢 Here we have returned only a single value from the get_second_largest() function. If you want to return multiple values like largest and second_largest, Then create a static array and return it back to the caller.
Program Output:
Compile and Run the Program and observe the output.
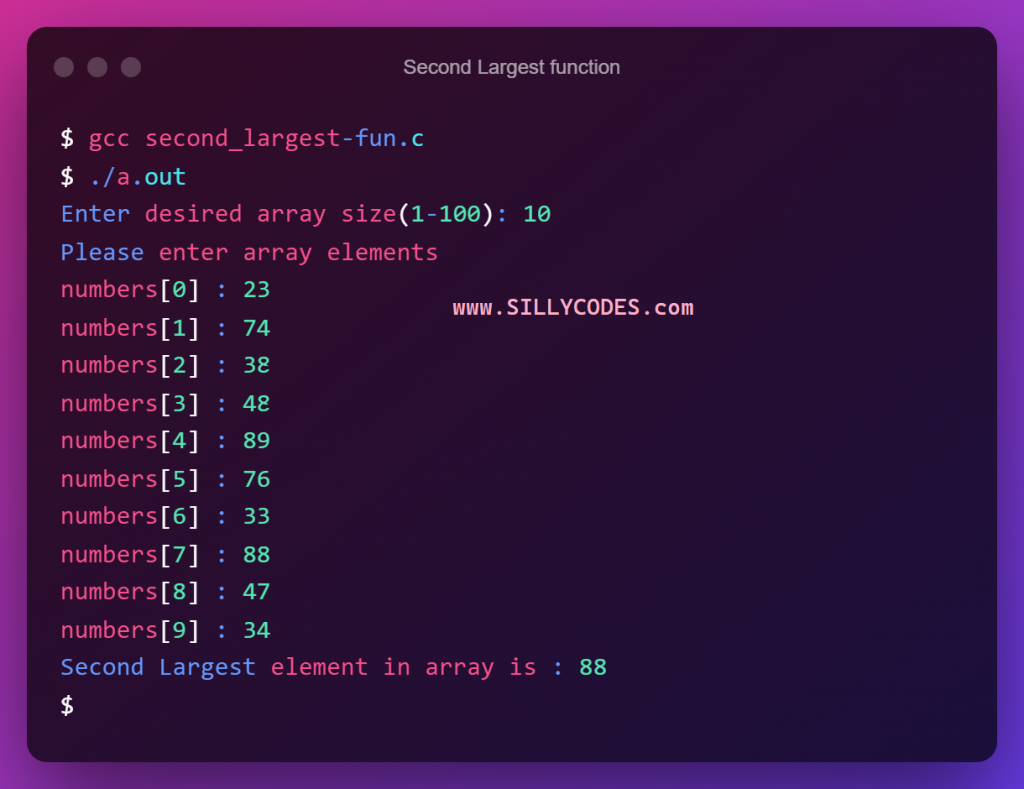
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
$ gcc second_largest-fun.c $ ./a.out Enter desired array size(1-100): 10 Please enter array elements numbers[0] : 23 numbers[1] : 74 numbers[2] : 38 numbers[3] : 48 numbers[4] : 89 numbers[5] : 76 numbers[6] : 33 numbers[7] : 88 numbers[8] : 47 numbers[9] : 34 Second Largest element in array is : 88 $ ./a.out Enter desired array size(1-100): 09 Please enter array elements numbers[0] : -89 numbers[1] : 34 numbers[2] : 834 numbers[3] : 274 numbers[4] : 24 numbers[5] : -0 numbers[6] : 938 numbers[7] : 34 numbers[8] : 23 Second Largest element in array is : 834 $ |
Exercise: Sort the array and Get the last and last-second numbers:
We can also get the largest and second largest elements by sorting the array and accessing the last two elements. Try to sor the array and get the largest and second largest elements.
Related Array Programs:
- Index – C Language Practice Programs
- C Tutorials Index – Step by step tutorials to master C Langauge
- C Program to Read and Print Arrays
- Reverse Print Array Program in C
- C Program to calculate Sum of all array Elements
- C Program to Calculate the Average of all array Elements
- C Program to find minimum element in Array
- C Program to Find Maximum Element in Array