Find First Occurrence of a Character in String in C Language
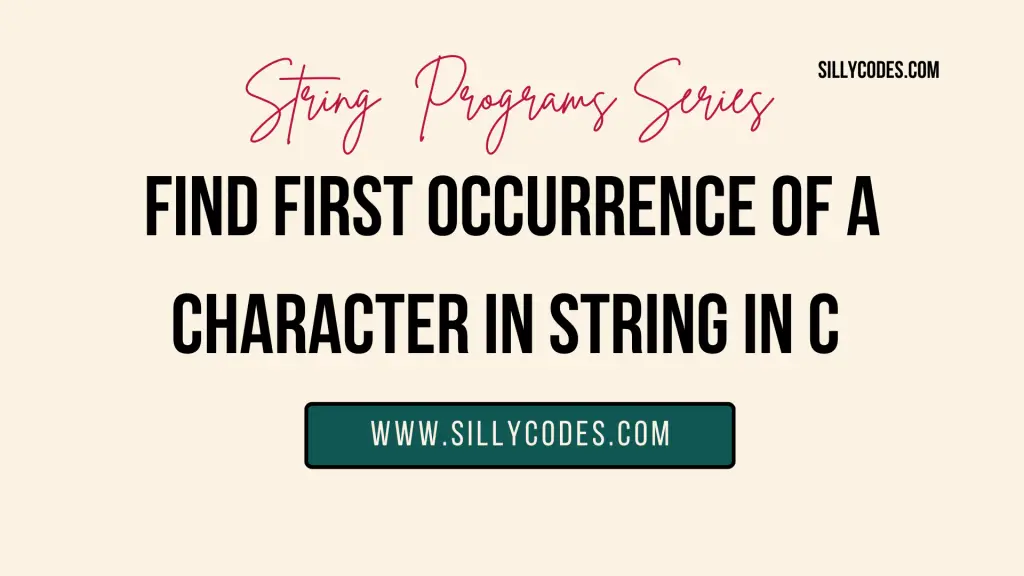
Program Description:
Write a Program to Find First Occurrence of a Character in String in C Programming language. The program should accept a string and a character from the user and find the first occurrence of the given character in the string.
The program is case-sensitive. So the Uppercase character and Lowercase characters are different.
📢 This Program is part of the C String Practice Programs series
We will look at a few methods to search for the first occurrence of a character in the string program. Each method will contain a step-by-step explanation of the program with program output. We also included the instructions to compile and run the program using your compiler.
Before going to write the program. Let’s understand the program requirements by looking at an example input and output of the program.
Example Input and Output:
Input:
Enter a string : CProgramming
Enter character to search in the string: o
Output:
FOUND: First Occurance of o character is found at 3 index in given string
The character o is at the index 3 in the input string CProgramming
Prerequisites:
To gain a better understanding of the program. Please review the C language string tutorials below.
- Strings in C Language – Declare, Initialize, and Modify Strings
- Different ways to Read and Print strings in C
- Arrays in C with Example Programs
- Functions in C – How to Create and Use functions in C
We will look at the three different methods to search for the character in a string in C Language.
- Iterative Method
- User-defined Functions
- Library Function ( strchr library function)
First Occurrence of a Character in String in C Program Explanation:
- Start the program by defining a Macro to hold the max size of the array – #define SIZE 100
- Declare the input string( inputStr)
- Take the input string and character to search from the user and update the inputStr and ch variables. We use the gets function to read the string.
- To search for the first occurrence of the character( ch) in a string( inputStr), Iterate over the string and check each character.
- For loop starts by initializing i to 0. It will continue looping as long as the current character (
inputStr[i]) is not a NULL character (
'\0'). At each iteration, i is incremented by 1.
- Check if the current character( inputStr[i]) is equal to ch- i.e if(inputStr[i] == ch)
- If the above condition is true, Then we found the character( ch) in the inputStr. print the result and stop the program.
- If we reached out of the loop, Then ch is not found in the inputStr. So print the NOT FOUND message on the console.
Method 1: First Occurrence of a Character in String in C Program (Iterative Method):
Here is the program to search for a character in a string in C programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
/* program to Search for a character in a string in C sillycodes.com */ #include<stdio.h> // Max Size of string #define SIZE 100 int main() { // declare a string with 'SIZE' char inputStr[SIZE]; char ch; int i; // Take the string from the user printf("Enter a string : "); gets(inputStr); // Also take the search character printf("Enter character to search in the string: "); scanf(" %c", &ch); // Iterate over the string for(i = 0; inputStr[i]; i++) { // check if the current character is equal to target('ch') character if(inputStr[i] == ch) { // Found the character printf("FOUND: First Occurance of %c character is found at %d index in given string\n", ch, i); // stop the program. return 0; } } // If we reached here, Then we haven't found the character printf("NOT FOUND: Character %c is not found in the given string\n", ch); return 0; } |
Program Output:
Let’s compile and run the program.
Compile the program.
$ gcc search-character.c
Run the program.
1 2 3 4 5 |
$ ./a.out Enter a string : CProgramming Enter character to search in the string: o FOUND: First Occurance of o character is found at 3 index in given string $ |
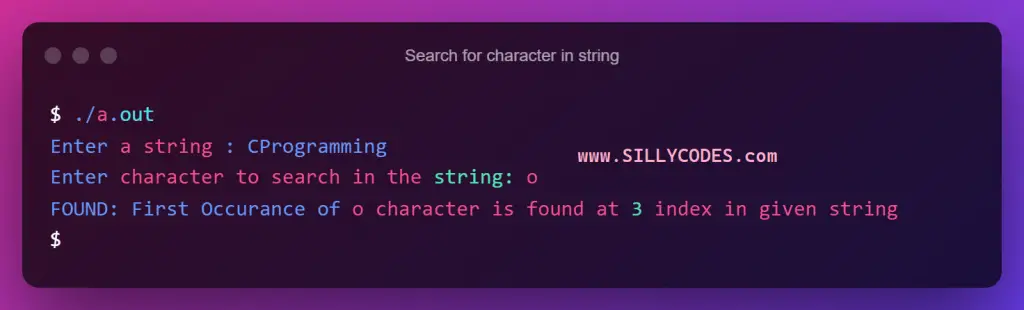
In the above example, the user provided the input string as CProgramming and the character to search is o.
The program is able to find the character o at the index 3 in the given CProgramming string.
Let’s look at a few more examples.
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter a string : www.SillyCodes.com Enter character to search in the string: l FOUND: First Occurance of l character is found at 6 index in given string $ $ ./a.out Enter a string : learn programming online Enter character to search in the string: r FOUND: First Occurance of r character is found at 3 index in given string $ |
The program is generating the excepted results.
Method 2: First Occurrence of a Character in String using user-defined functions:
In the above program, We have written the complete program inside the main function. It is not a recommended method, Let’s rewrite the above program to use a function search for the character in the string.
Define a function named searchChar() to search the character in a string.
Here is the prototype of the searchChar() function.
int searchChar(char * str, int ch)
Search for the character 'ch'in String(‘ str’) and If the ch is found in the str return the index of ch. Otherwise, return -1.
Here is the modified program to search for a string using a user-defined function
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
/* program to Search for a character in a string in C using Functions sillycodes.com */ #include<stdio.h> // Max Size of string #define SIZE 100 /** * @brief - Search for the character 'ch' in String('str') * * @param str - Input String * @param ch - Character to search in string 'str' * @return int - Index if the 'ch' is found in 'str'. Otherwise, returns -1. */ int searchChar(char *str, int ch) { int i; // Iterate over the string for(i = 0; str[i]; i++) { // check if the current character is equal to target('ch') character if(str[i] == ch) { // Found the first occurance of character // Return Index return i; } } // Not found return -1; } int main() { // declare a string with 'SIZE' char str[SIZE]; char ch; // Take the string from the user printf("Enter a string : "); gets(str); // Also take the search character printf("Enter character to search in the string: "); scanf(" %c", &ch); // call the 'searchChar' function to search for the character in string int result = searchChar(str, ch); // Check the result if(result == -1) { // If we reached here, Then we haven't found the character printf("NOT FOUND: Character %c is not found in the given string\n", ch); } else { // Found the character printf("FOUND: First Occurance of %c character is found at %d index in given string\n", ch, result); } return 0; } |
Call the searchChar() function from main() function and pass the input string and character( ch).
1 |
int result = searchChar(str, ch); |
Capture the return value in result variable.
Program Output:
Compile and Run the program.
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc search-character-func.c $ ./a.out Enter a string : Programming is Fun Enter character to search in the string: m FOUND: First Occurance of m character is found at 6 index in given string $ $ ./a.out Enter a string : Tech Enter character to search in the string: r NOT FOUND: Character r is not found in the given string $ |
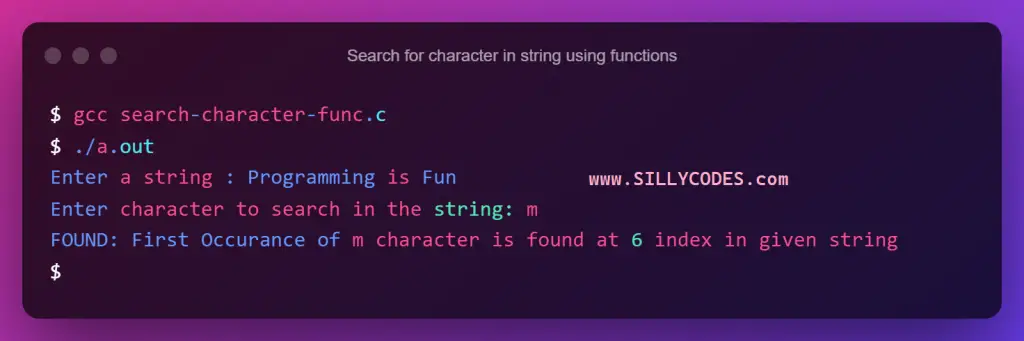
In the first example, The character m is found at the 6th index in Programming is Fun string. Similarly, In the second string, The character r is not found in the string Tech
Method 3: First Occurrence of a Character in String using strchr function in C:
In this method, Let’s use the strchr library function to find first occurrence of a character in string in c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
/* program to Search for a character in a string in C using library function sillycodes.com */ #include <stdio.h> #include <string.h> // For 'strchr' function // Max Size of string #define SIZE 100 int main() { // declare a string with 'SIZE' char str[SIZE]; char ch; // Take the string from the user printf("Enter a string : "); gets(str); // Also take the search character printf("Enter character to search in the string: "); scanf(" %c", &ch); // call the 'strchr' function to search for the character in string char * result = strchr(str, ch); // display the results if(result == NULL) { // Not found printf("NOT FOUND: Character '%c' is not found '%s' string\n", ch, str); } else { // Found printf("FOUND: Character '%c' is Found in the '%s' string \n", ch, str); printf("Result is : %s \n", result); } return 0; } |
Program Output:
Let’s compile and run the program.
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc search-character-strchr.c $ ./a.out Enter a string : Lewis Hamilton Enter character to search in the string: w FOUND: Character 'w' is Found in the 'Lewis Hamilton' string Result is : wis Hamilton $ ./a.out Enter a string : Max Enter character to search in the string: v NOT FOUND: Character 'v' is not found 'Max' string $ |
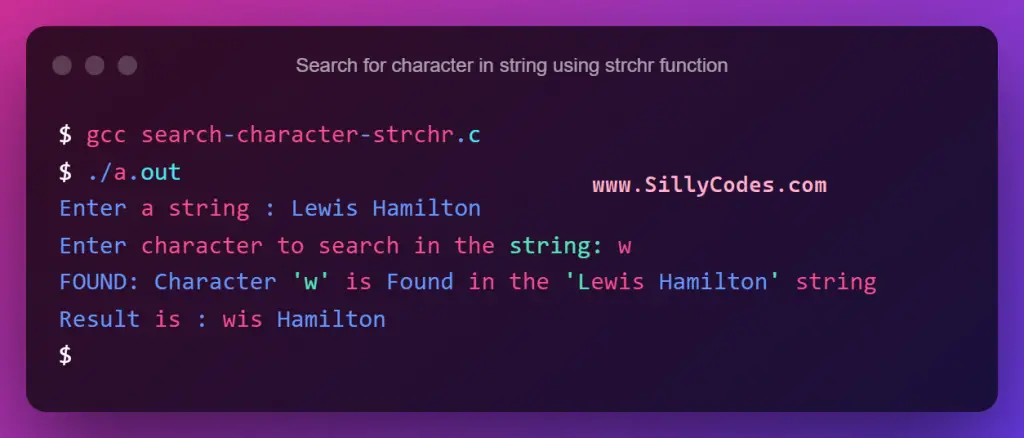
As we can see from the above examples, The program is generating the desired results.
Related String Practice Programs:
- C Tutorials Index
- C Programs Index
- C Program to Convert Lower case string to Upper case string
- C Program to Convert Uppercase string to Lowercase string
- C Program to Reverse a String
- C Program to Check Palindrome String
- C Program to Toggle Case of All Characters in String
- C Program to Remove Leading Whitespaces in a String
- C Program to Remove Trailing Whitespaces in a String
- C Program to Remove Extra Spaces between the Words in String
- C Program to Remove All Whitespaces in a String or Sentence
- C Program to Count Frequencies of each character in a string
- C Program to Find Highest Frequency character in a String