Program to find the LCM of Two numbers in C Language
- Program Description:
- What is LCM or Lowest Common Multiple?
- Program Example Input and Output:
- Pre-Requisites:
- LCM of Two numbers in C Program Algorithm:
- Program to calculate the LCM of Two numbers in C using while loop:
- Find the LCM of Two numbers in C Language using for loop:
- LCM of Two numbers in C language using GCD:
- Related Programs:
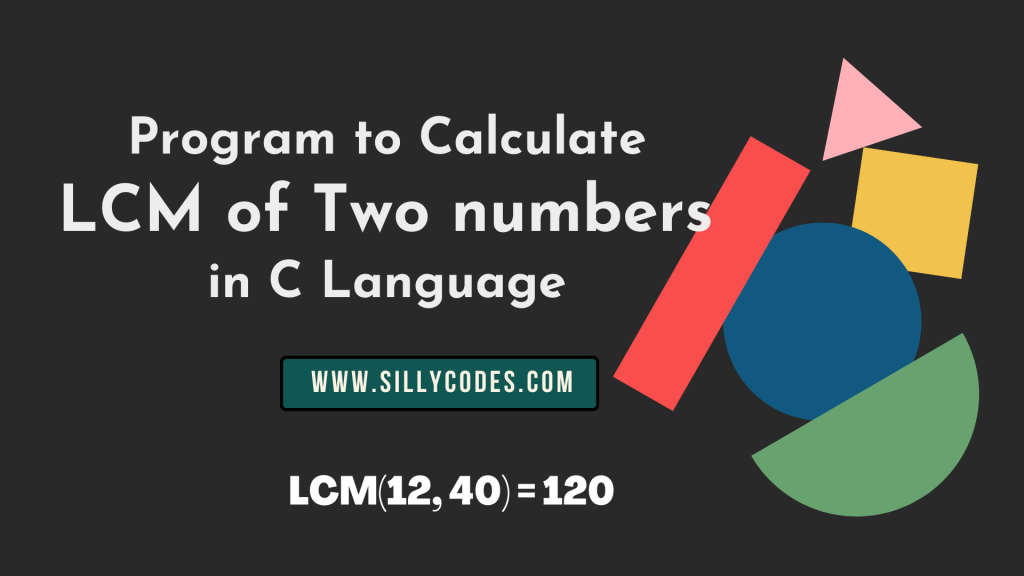
Program Description:
Write a Program to calculate the Lowest Common Multiple or LCM of Two numbers in C programming language. The program should accept two positive numbers from the user and calculate the LCM of the given numbers. Program should also perform the error checks and display error message on Invalid Input.
Before going to write the program. It is necessary to understand what is LCM and how to calculate the LCM.
What is LCM or Lowest Common Multiple?
The LCM or Lowest Common Mulitple of two numbers num1 and num2 is the smallest Integer that is perfectly (Evenly) divisible by both num1 and num2.
📢 The LCM is the smallest positive integer that is evenly divisible by both num1 and num2.
For example,
LCM(4, 30) = 60
LCM(6,10) = 30
There are couple of ways to calculate the LCM of two numbers, There are
- We can calculate the LCM of two numbers by manually calculating the lowest multiple which divisible by both num1 and num2.
- Using the GCD or Greatest Common Divisor of two numbers. – Here is the formula to calculate the LCM based on GCD.
- LCM = (num1 * num2) / GCD
We are going to look at both programs in this tutorial.
Program Example Input and Output:
Input:
Enter two positve numbers to calculate LCM: 30 20
Output:
LCM of 30 and 20 is 60
Pre-Requisites:
We need to have the knowledge of the C Loops and Arithmetic operators like Modulus operators. Please go through following articles to understand more about them
- Modulus Operator in C with Example Programs
- Logical Operators
- While Loop in C Language
- For Loop in C Language with Example Programs
LCM of Two numbers in C Program Algorithm:
- Accept the input of two numbers from the user and store them in variables num1 and num2
- Check for negative numbers and display an error message if the user enters a negative number.
- Calculate the maximum of two numbers ( num1 and num2). We are using Conditional operator to calculate the maximum. Store the maximum in max variable
- Start the Infinite Loop and Check if the max is evenly divisible by num1 and num2 using ((max % num1 == 0) && (max % num2 == 0)).
- The above step 4 will continue until we get the LCM or Lowest Common Multiple. Once we break out of the Infinite loop, The variable max contain the LCM of num1 and num2
Program to calculate the LCM of Two numbers in C using while loop:
We are going to use the Infinite while loop with the while(1) syntax.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/* Program: LCM program in C using while loop Author: SillyCodes.com */ #include<stdio.h> int main() { int num1, num2; // User Input printf("Enter two positve numbers to calculate LCM: "); scanf("%d%d", &num1, &num2); // check for negative numbers and display error if(num1 <= 0 || num2 <= 0) { // got negative number. Display Error printf("Invalid Input. Please provide valid numbers\n"); return 0; } // get the 'max' of 'num1' and 'num2' using conditional operator. int max = (num1 > num2) ? num1 : num2; // Infinite Loop - https://sillycodes.com/infinite-loop-in-c-programming/ while(1) { // check if 'max' is evenly divisable by 'num' and 'num2' if((max % num1 == 0) && (max % num2 == 0)) { // got LCM printf("LCM of %d and %d is %d \n", num1, num2, max); break; } // Not found LCM, Increment max. max++; } return 0; } |
Program Output:
Save the program using .c extension and compile and run program. We are using GCC compiler to compile the program
Let’s check the positive number test cases.
1 2 3 4 5 6 7 8 |
$ gcc lcm.c $ ./a.out Enter two positve numbers to calculate LCM: 4 30 LCM of 4 and 30 is 60 $ ./a.out Enter two positve numbers to calculate LCM: 24 80 LCM of 24 and 80 is 240 $ |
As we can see from the above output, We are getting the desired result and program is able to calculate the LCM of given numbers.
Let’s try the negative numbers test case. As the LCM is only for positive numbers, The program should display an error message
1 2 3 4 5 6 7 |
$ ./a.out Enter two positve numbers to calculate LCM: -10 200 Invalid Input. Please provide valid numbers $ ./a.out Enter two positve numbers to calculate LCM: 5 -90 Invalid Input. Please provide valid numbers $ |
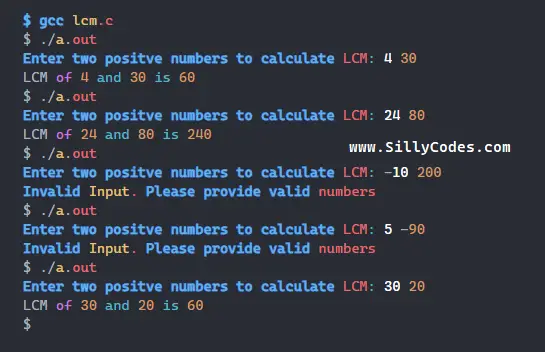
As shown in the output from the program, whenever a user enters a negative number, an error message is displayed. Also take note that the program displays an error if the user enters a negative integer for either num1 or num2.
Find the LCM of Two numbers in C Language using for loop:
Let’s re-write the above program using the for loop. We can create the infinite loops using the for loop using the following for loop syntax.
for (; ; )
If you don’t specify any condition to for loop, Then for loop will run forever. Thus creating Infinite loop.
Here is the LCM program using the for loop in C.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
/* Program: LCM program in C using for loop Author: SillyCodes.com */ #include<stdio.h> int main() { int num1, num2; // take input from the user. printf("Enter two positve numbers to calculate LCM: "); scanf("%d%d", &num1, &num2); // check for negative numbers. if(num1 <= 0 || num2 <= 0) { // got negative number. Display Error printf("Invalid Input. Please provide valid numbers\n"); return 0; } // get 'max' using conditional operator. int max = (num1 > num2) ? num1 : num2; // Loop infinitely and break when you found the LCM for(; ; max++) { // check if both 'num1' and 'num2' evenly divide 'max' if((max % num1 == 0) && (max % num2 == 0)) { // got LCM printf("LCM of %d and %d is %d \n", num1, num2, max); break; } } return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc lcm.c $ ./a.out Enter two positve numbers to calculate LCM: 40 19 LCM of 40 and 19 is 760 $ ./a.out Enter two positve numbers to calculate LCM: -30 12 Invalid Input. Please provide valid numbers $ ./a.out Enter two positve numbers to calculate LCM: 56 -32 Invalid Input. Please provide valid numbers $ ./a.out Enter two positve numbers to calculate LCM: 80 41 LCM of 80 and 41 is 3280 $ |
The program is giving the valid output and also showing the error message on Invalid input.
LCM of Two numbers in C language using GCD:
We can also find the LCM of two numbers num1 and num2 using their GCD or Greatest Common Divisor using following formula.
LCM = (num1 * num2) / GCD
Let’s use the above formula to calculate the LCM using GCD formula.
We have already covered the Program to calculate the GCD of Two Numbers in C in earlier posts. We are going to use same logic to calculate the GCD in this program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
/* Program: LCM program in C using LCM formula Author: SillyCodes.com */ #include<stdio.h> int main() { int num1, num2, i, gcd, lcm; // User Input printf("Enter two positve numbers to calculate LCM: "); scanf("%d%d", &num1, &num2); // check for negative numbers and display error if(num1 <= 0 || num2 <= 0) { // got negative number. Display Error printf("Invalid Input. Please provide valid numbers\n"); return 0; } // calculate the GCD // Get the 'min' using conditional variable int min = (num1 > num2) ? num2: num1; // Loop from '1' to 'min' for(i = 1; i <= min; i++) { // check if the 'i' is factor of both 'num1' and 'num2' if(num1 % i == 0 && num2 % i == 0) { // update the 'gcd' gcd = i; } } // We got the GCD, // Calculate LCM using - LCM = (num1 * num2) / GCD lcm = (num1 * num2) / gcd; printf("LCM of %d and %d is %d\n", num1, num2, lcm); return 0; } |
Program Output:
Let’s try positive number test cases and negative number test cases and see if we are getting the desired output.
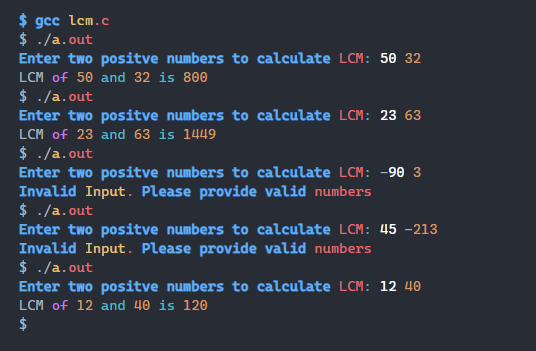
Test Case 1: Test with Positive numbers:
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc lcm.c $ ./a.out Enter two positve numbers to calculate LCM: 50 32 LCM of 50 and 32 is 800 $ ./a.out Enter two positve numbers to calculate LCM: 23 63 LCM of 23 and 63 is 1449 $ ./a.out Enter two positve numbers to calculate LCM: 12 40 LCM of 12 and 40 is 120 $ |
We are getting the LCM of two numbers correctly.
Test Case 2: Test with Negative numbers
1 2 3 4 5 6 7 |
$ ./a.out Enter two positve numbers to calculate LCM: -90 3 Invalid Input. Please provide valid numbers $ ./a.out Enter two positve numbers to calculate LCM: 45 -213 Invalid Input. Please provide valid numbers $ |
As you can see from the above output, Program is displaying the error message.
Related Programs:
- If else practice Programs
- Switch Case Practise Programs
- C program to test Leap year
- C program to Find Area and Perimeter of Rectangle.
- Calculate the Grade of Student.
- Temperature Conversion program in C. ( Centigrade to Fahrenheit ).
- Type Conversion Operators on Assignment operator.
- Prime Numbers up to n
- Calculate Sum of Digits Program in C
- Octal to Decimal Number Conversion
Learn More about LCM at Wiki:
- Further information: Least common multiple
3 Responses
[…] have looked at the LCM of two numbers program in our earlier articles, In today’s article, We are going to write a program to check […]
[…] C Program to Calculate the LCM of two Numbers […]
[…] C Program to Calculate the LCM of two Numbers […]