Set, Clear, Toggle Bit, and Check a single Bit in C Language
- Set, Clear, Toggle bit, and Check bit Introduction:
- Set, Clear, Toggle bit, and Check bit in C Pre-Requisites:
- Set a Bit in C ( Set Nth bit) using bitwise Operators:
- Set Nth Bit in C Program:
- Check Nth Bit in C language:
- Program to Check Nth Bit is SET or CLEAR:
- Clear Nth Bit in C Programming:
- CLEAR Nth bit Program in C Language:
- Toggle Nth Bit or Toggle a Bit in C Programming:
- Toggle Nth Bit Program in C Language:
- Toggling Nth bit Program in C using bitwise Operators:
- Set, Clear, Toggle bit, and Clear bit in C Programs Conclusion:
- Related Programs:
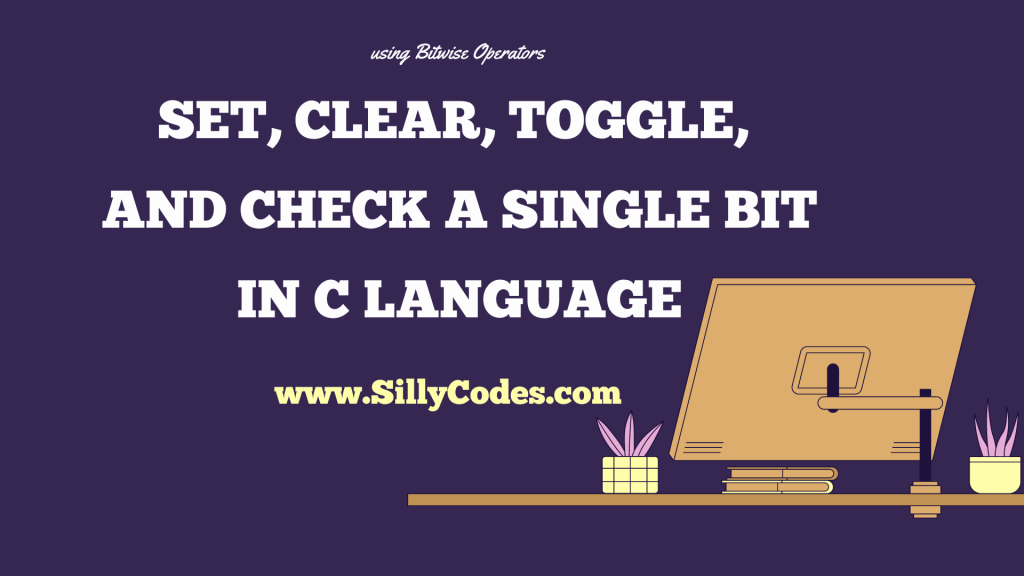
Set, Clear, Toggle bit, and Check bit Introduction:
We have looked at the Bitwise operators in C language and explained them with Examples in our earlier articles, In today’s article, We are going to look at a few uses of the bitwise operators, Like how to Set, Clear, Toggle, and Check a Bit in C language using bitwise operators.
Set, Clear, Toggle bit, and Check bit in C Pre-Requisites:
It is recommended to know how the bitwise operators work in C language. Please go through the following link to learn more about them.
Let’s start with the Set a bit in C.
Set a Bit in C ( Set Nth bit) using bitwise Operators:
To set a bit or Nth bit in C language, We use the Bitwise OR operator with the bitwise Left shift operator.
The SETting an Nth bit means, We need to make the Nth bit as One(1). So if the bit in the Nth position is Zero(0), Then we need to change it to One(1). If the bit at the Nth position is One(1), We don’t do anything and the bit will have the value as One(1).
Logic to Set Nth bit or Set a bit:
To understand the logic of Set Nth bit, We need to know the truth table of OR. Here is the truth table of bitwise OR
Bit-1 | Bit-2 | OR (Bit- 1 | Bit-2) |
---|---|---|
0 | 0 | 0 |
1 | 0 | 1 |
0 | 1 | 1 |
1 | 1 | 1 |
To Set the Nth bit, Use the following bitwise OR logic.
InputNumber |= ( 1 << bitPosition )
with is equal to
InputNumber = InputNumber | (1 << bitPosition)
Here,
The
InputNumber is the number where we are trying to SET the bit
The
bitPosition is the position of the bit (Nth bit) in the binary sequence of
InputNumber
So we are shifting the number 1, The bitposition times in the left-side direction. Then performing the bitwise OR operator.
Set Nth Bit Example:
Let’s look at an example: Set the 2nd bit in number 120.
InputNumber = 120.
bitPosition = 2
The binary equivalent of the number 120 is 00000000000000000000000001111000
Related Program: Program to Convert Decimal Number to Binary Sequence in C
Now, To set the bit at position 2. The bit position starts with index 0, So the Least significant bit(LSB) in the above binary sequence position is 0.
To Set the 2nd bit in the number 120, Use.
InputNumber = InputNumber | (1 << 2);
InputNumber = 120 | (1 << 2);
Here is the calculation
➡️ Bitwise OR : (InputNumber | (1 << 2)) is:
–> 0111 1000 (InputNumber – 120 in Binary)
–> 0000 0100 (1 << 2)
–> 0111 1100 ( Result = (InputNumber | (1 << 2)) which is equal to 124 )
After the above operation, The bit at the 2nd position of the InputNumber(120) is changed from zero(0) to One(1). So we are able to SET the 2nd bit and The InputNumber value became 124.
The final value of InputNumber is 124 ( Which is 00000000000000000000000001111100 in binary )
📢 The Bit positions start with the Index 0 ( from the left side). So when we SET the bit at Index 2, We actually changed the 3rd from LSB(If you take Index as 1).
Set Nth Bit in C Program:
Let’s look at the set Nth bit algorithm, Then we will look at the program as well.
Set Nth bit Algorithm:
- Take the Input from the user and store it in variable num
- Get the bit number to set from the user and store it in variable bit
- Take backup of num to variable called backup for later use
- Now Set the bit using the above bitwise OR logic i.e num = num | (1 << bit);
- Display the results on the console.
Set Nth bit Program in C Language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
#include<stdio.h> int main() { int num, bit, i; // Take input from the user printf("Enter a number: "); scanf("%d", &num); printf("Enter bit number to Set : "); scanf("%d", &bit); // Don't allow negative numbers if(num < 0 || bit < 0) { printf("Invalid Input: Try again\n"); return 0; } // Take backup of 'num' int backup = num; // Set the bit num = num | (1 << bit); // num |= (1<< bit) printf("Number(%d) after SETing bit at %d position became %d \n", backup, bit, num); return 0; } |
Program Output:
Let’s compile and run the program using your compiler.
gcc set-bit.c
Example 1: InputNumber 120 and BitPosition 2.
1 2 3 4 5 |
$ ./a.out Enter a number: 120 Enter bit number to Set : 2 Number(120) after SETing bit at 2 position became 124 $ |
As you can see from the above output, We are getting the expected results – 124.
Let’s check the output for a few more cases
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter a number: 90 Enter bit number to Set : 5 Number(90) after SETing bit at 5 position became 122 $ ./a.out Enter a number: 269 Enter bit number to Set : 8 Number(269) after SETing bit at 8 position became 269 $ ./a.out Enter a number: 320 Enter bit number to Set : 10 Number(320) after SETing bit at 10 position became 1344 $ |
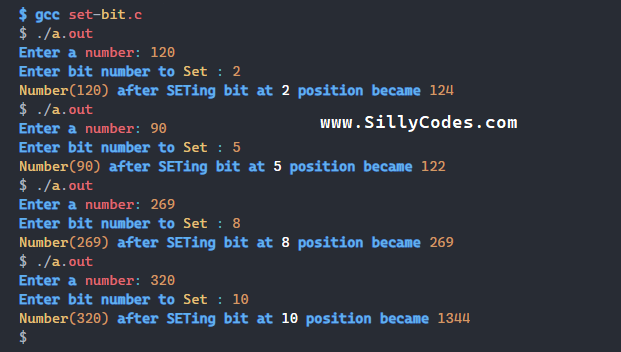
As expected, We are getting the desired results. And the program is able to SET the bits properly.
Check Nth Bit in C language:
The check Nth bit means We need to check if the bit at the Nth position is One or Zero. This check Nth bit logic will help us to convert decimal number to binary number.
We have already covered the Decimal to Binary Number conversion program, We have used the Check Nth bit logic there as well.
To check Nth bit, We use the bitwise AND operator with the bitwise left shift operator. Here is the logic to check Nth bit.
( InputNumber & (1 << bitPosition) )
Here, InputNumber is the given number and bitPosition is the Nth bit ( The bit we want to check)
So we are left shifting the 1, bitPositions times and performing the bitwise AND operation with InputNumber
Check Nth bit Example:
Let’s look at an example to understand how the check Nth bit logic works.
InputNumber = 45
bitPosition = 3
Apply the above logic.
( InputNumber & (1 << bitPosition) ) ? 1 : 0
(45 & ( 1 << 3)) ? 1 : 0;
➡️ (45 & (1 << 3)) ? 1 : 0:
–> 0010 1101 (InputNumber – 45 in Binary)
–> 0000 1000 (1 << 3)
Now if you observe the above binary sequence(of number 45), The bit at position 3 is 1 (SET). So we will get the result as SET i.e 1.
Program to Check Nth Bit is SET or CLEAR:
Check Nth Bit Algorithm:
- Take the input number and bit number from the user and store them in the variables num and bit respectively.
- We won’t allow negative numbers, So check for negative numbers and display an error message
- Check the bit using the check Nth logic – result = (num & (1 << bit)) ? 1 : 0;
- If the result is 1, Then bit is SET i.e one(1), Otherwise bit is CLEAR or Not-Set. i.e Zero(0).
Check Nth bit Program in C:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
#include<stdio.h> int main() { int num, bit, i; // Take input from the user printf("Enter a number: "); scanf("%d", &num); // Take bit number from the user. printf("Enter bit number to check : "); scanf("%d", &bit); // Don't allow negative numbers if(num < 0 || bit < 0) { printf("Invalid Input: Try again\n"); return 0; } // Check the bit int result = (num & (1 << bit)) ? 1 : 0; // if the result is 1, Bit is set if(result) printf("Bit at position-%d in Number(%d) is SET(1)\n", bit, num); else printf("Bit at position-%d in Number(%d) is Not-SET(0)\n", bit, num); return 0; } |
Program Output:
Run the program
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
$ gcc check-bit.c $ ./a.out Enter a number: 45 Enter bit number to check : 3 Bit at position-3 in Number(45) is SET(1) $ ./a.out Enter a number: 634 Enter bit number to check : 13 Bit at position-13 in Number(634) is Not-SET(0) $ ./a.out Enter a number: 32 Enter bit number to check : 5 Bit at position-5 in Number(32) is SET(1) $ ./a.out Enter a number: -55 Enter bit number to check : 6 Invalid Input: Try again $ |
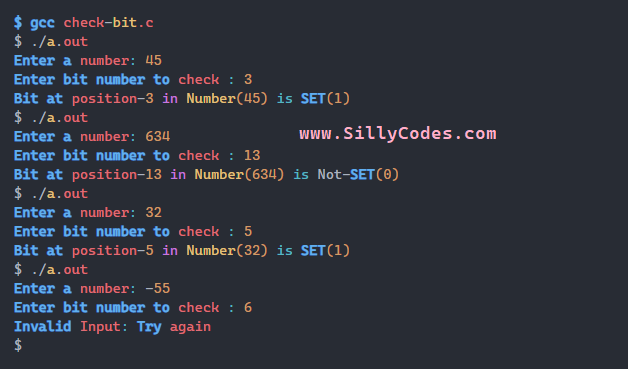
As we can see from the above output, We are getting the expected results.
Clear Nth Bit in C Programming:
To CLEAR a bit or CLEAR Nth bit in C language, We use the Bitwise AND operator with the bitwise Left shift operator and One’s complement Operator.
The CLEARing an Nth bit means, We need to make the Nth bit as Zero(0). So if the bit in the Nth position is One(1), Then we need to change it to Zero(0). If the bit at the Nth position is Zero(1), We don’t do anything and the bit will have the value as Zero(0).
Logic to Clear Nth bit or Clear a bit:
To understand the logic of Clear Nth bit, We need to know the truth table of AND. Here is the truth table of bitwise AND
Bit-1 | Bit-2 | AND (Bit- 1 & Bit-2) |
---|---|---|
0 | 0 | 0 |
1 | 0 | 0 |
0 | 1 | 0 |
1 | 1 | 1 |
To CLEAR the Nth bit, Use the following bitwise AND logic.
InputNumber &= ~( 1 << bitPosition )
with is equal to
InputNumber = InputNumber & (~ (1 << bitPosition))
Here,
The
InputNumber is the Given Number
The
bitPosition is the position of the bit (Nth bit) in the binary sequence of
InputNumber
So we are shifting the number 1, The bitposition times in the left-side direction and Applying the one’s complement. Finally performing the bitwise AND operation.
CLEAR Nth Bit Example:
Let’s look at an example: Set the 4th bit in number 63.
InputNumber = 63.
bitPosition = 4.
The binary equivalent of the number 63 is 00000000000000000000000000111111
To set the 4th bit in the number 63, Use.
InputNumber = InputNumber & ~ (1 << 4);
InputNumber = 120 & ~ (1 << 4);
Here is the calculation
➡️ (InputNumber & ~(1 << 4)) is:
–> 0001 0000 (1 << 4)
–> 1110 1111 ~(1 << 4)
–> 0011 1111 (InputNumber – 63 in Binary)
–> 0010 1111 ( Result = (InputNumber & ~(1 << 4)) which is equal to 47 )
After the above operation, The bit at the 4th position of the InputNumber(63) is changed from One(1) to Zero(0).
So we are able to CLEAR the bit at position 4 using the InputNumber = InputNumber & ~ (1 << bit); Logic.
The final value of InputNumber is 47 ( Which is 00000000000000000000000000101111 in binary )
CLEAR Nth bit Program in C Language:
Clear Nth bit Algorithm:
- Accept the input number from the user and store it in variable num, Also take the bit position and store it in bit variable.
- Check for negative numbers and display an error notice to the user.
- Take a backup of the num to backup variable for later usage.
- CLEAR the bit using the Clear Nth bit logic – num = num & ~(1 << bit);
- Display the Results.
Clear Nth bit in C Program using Bitwise AND Operator:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
#include<stdio.h> int main() { int num, bit, i; // Take input from the user printf("Enter a number: "); scanf("%d", &num); printf("Enter bit number to Clear : "); scanf("%d", &bit); // Don't allow negative numbers if(num < 0 || bit < 0) { printf("Invalid Input: Try again\n"); return 0; } // Take backup of 'num' int backup = num; // Clear the bit num = num & ~(1 << bit); // num &= ~(1<< bit) printf("Number(%d) after CLEARing bit at %d position became %d \n", backup, bit, num); return 0; } |
Program Output:
Compile and Run the Program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
$ gcc clear-bit.c $ ./a.out Enter a number: 63 Enter bit number to Clear : 4 Number(63) after CLEARing bit at 4 position became 47 $ ./a.out Enter a number: 300 Enter bit number to Clear : 3 Number(300) after CLEARing bit at 3 position became 292 $ ./a.out Enter a number: 431 Enter bit number to Clear : 7 Number(431) after CLEARing bit at 7 position became 303 $ ./a.out Enter a number: -778 Enter bit number to Clear : 4 Invalid Input: Try again $ |
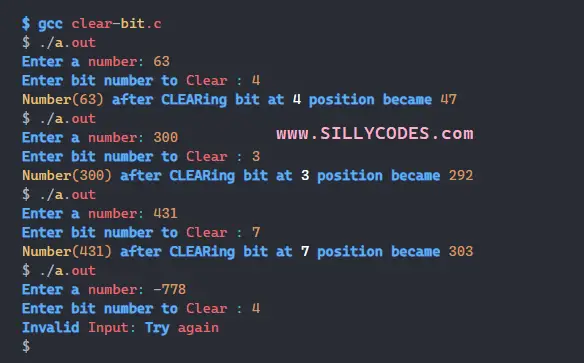
As you can see from the above output, We are getting the results correctly, We also added logic to display an error on a negative number.
Toggle Nth Bit or Toggle a Bit in C Programming:
Toggling Nth Bit means, If the Nth bit is One(1), Then we need to Invert it and make it Zero(0). Similarly, If the Nth bit is Zero(0), We need to change it to One(1).
To Toggle Nth bit, We use the Bitwise XOR operator with the bitwise Left shift operator.
Logic to Toggle Nth bit or Invert a bit:
To understand the logic of the Toggle Nth bit, We need to know the truth table of XOR.
Bit-1 | Bit-2 | XOR (Bit- 1 ^ Bit-2) |
---|---|---|
0 | 0 | 0 |
1 | 0 | 1 |
0 | 1 | 1 |
1 | 1 | 0 |
To Toggle the Nth bit, Use the following bitwise XOR logic.
InputNumber ^= ( 1 << bitPosition )
with is equal to
InputNumber = InputNumber ^ (1 << bitPosition)
Here,
The
InputNumber is the Given Number
The
bitPosition is the position of the bit (Nth bit) in the binary sequence of
InputNumber
So we are shifting the number 1, The bitposition number of positions in the left-side direction and Performing the bitwise XOR operation.
📢 Bitwise XOR Operator is used to Invert or Toggle a Bit
Toggle Nth Bit Example:
Let’s look at an example: Set the 4th bit in number 38.
InputNumber = 38.
bitPosition = 4.
The binary equivalent of the number 63 is 00000000000000000000000000100110
To set the 4th bit in the number 38, Use.
InputNumber = InputNumber ^ (1 << 4);
InputNumber = 38 ^ (1 << 4);
Here is the calculation
➡️ (InputNumber ^ (1 << 4)) is:
–> 0010 0110 (InputNumber – 38 in Binary)
–> 0001 0000 (1 << 4)
–> 0011 0110 ( Result = (InputNumber ^ (1 << 4)) which is equal to 54 )
We have toggled the 4th bit in the number 38 using the bitwise XOR operator.
The bit at the 4th position i.e zero(0), has been Toggled/inverted and changed to One(1).
The final value of InputNumber is 54 ( Which is 00000000000000000000000000110110 in binary )
Toggle Nth Bit Program in C Language:
Toggling Nth bit Algorithm:
- Take the input number num and bit position bit from the user.
- Display error on negative numbers
- Take back up of the num and store it in a variable named backup
- Now Toggle the bit using the Toggle Nth bit logic – num = num ^ (1 << bit);
- Print the results onto the console.
Toggling Nth bit Program in C using bitwise Operators:
Let’s convert the above pseudo code into the C program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
/* C Program to Toggle a Bit */ #include<stdio.h> int main() { int num, bit, i; // Take input from the user printf("Enter a number: "); scanf("%d", &num); // Take bit number printf("Enter bit number to Clear : "); scanf("%d", &bit); // Don't allow negative numbers if(num < 0 || bit < 0) { printf("Invalid Input: Try again\n"); return 0; } // Take backup of 'num' int backup = num; // Toggle the bit or Invert the bit num = num ^ (1 << bit); // num ^= ~(1<< bit) // Print result printf("Number(%d) after Toggling bit at %d position became %d \n", backup, bit, num); return 0; } |
Program Output:
We are compiling the program using the GCC compiler.
Verify the results of the program
1 2 3 4 5 6 |
$ gcc toggle-bit.c $ ./a.out Enter a number: 38 Enter bit number to Clear : 4 Number(38) after Toggling bit at 4 position became 54 $ |
As you can see from the above output, We are getting the correct results and the program is able to toggle the bit at the specified position.
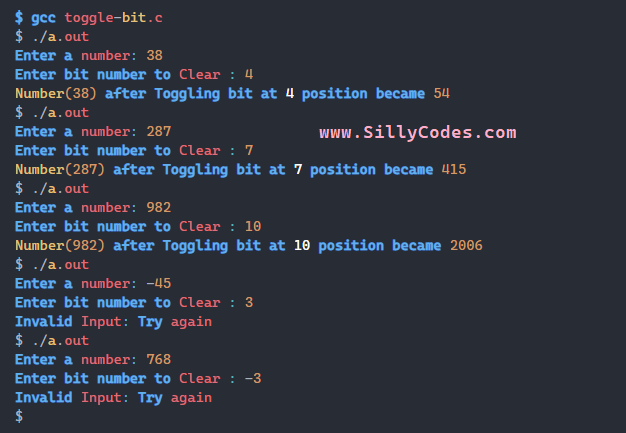
Let’s check a few more examples.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$ ./a.out Enter a number: 287 Enter bit number to Clear : 7 Number(287) after Toggling bit at 7 position became 415 $ ./a.out Enter a number: 982 Enter bit number to Clear : 10 Number(982) after Toggling bit at 10 position became 2006 $ ./a.out Enter a number: -45 Enter bit number to Clear : 3 Invalid Input: Try again $ ./a.out Enter a number: 768 Enter bit number to Clear : -3 Invalid Input: Try again $ |
Set, Clear, Toggle bit, and Clear bit in C Programs Conclusion:
In this article, We have learned about the Set, Clear, Toggle bit, and clear a bit in C language. We looked at the example for each section with detailed step-by-step explanations, Finally, we have written a C program for each section to make our understanding concrete.
If you have reached here, Then Thank you for taking the time to check out the full article. Here are similar programs to set, clear, toggle bit, and a clear bit in the C program.
Related Programs:
- C Loops Practice Programs [List of Programs]
- C Program to Calculate the Factorial of Number
- C Program to Calculate the Power of Number
- C Program to Check Number is Power of 2
- C Program to find power of number without Loops
- C Program to Find all Factors of a Number
- C Program to find all Prime Factors of number
- C Program to Calculate the GCD or HCF of Two Number
- C Program to Calculate the LCM of two Numbers
- C Program to Check Palindrome Number
1 Response
[…] Set, Clear, Toggle Bit, and Check a single Bit in C Language 🆕 […]