C Array – Sorting Ascending and Descending in C Language
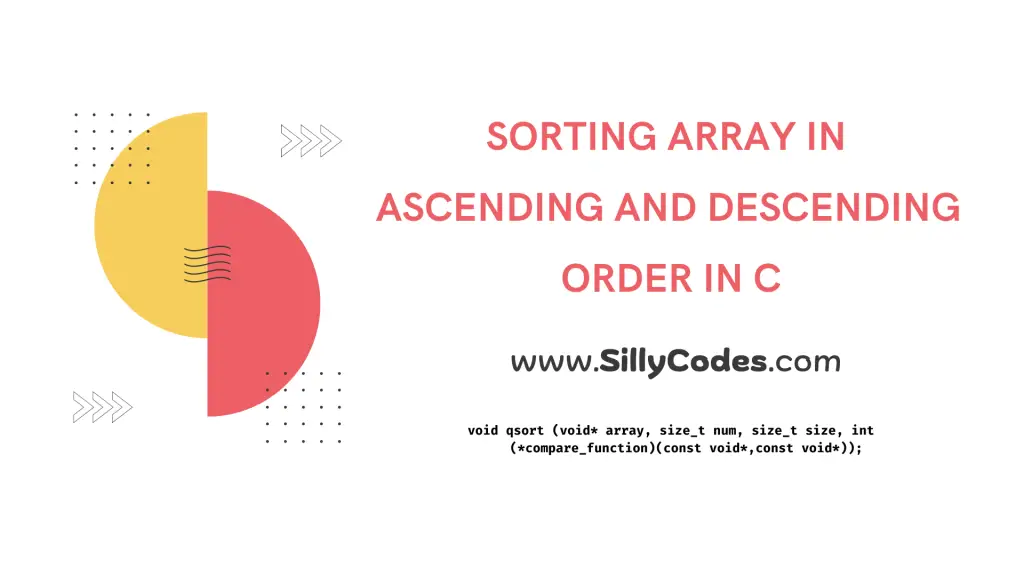
Sorting Ascending and Descending in C – Program Description:
Write a C Program for Sorting Ascending and Descending in C programming language. We are going to use the qsort() function from the stdlib.h header file to sort the array.
We are going to write two programs in this article.
- Sorting Array in Ascending order using QSort.
- Sorting Array in Descending order using QSort.
Syntax of the QSort() function:
1 2 |
void qsort (void* array, size_t num, size_t size, int (*compare_function)(const void*,const void*)); |
- array is the array to be sorted
- num is the size of the array
- size is the size of the data type. If you are using integer data, then it should sizeof(int)
- compare_function is a function pointer, which points to the comparison function.
1. Sorting Array in Ascending Order Using QSort Function:
Sort Array – Ascending Order Program Explanation:
- Declare the values array and desired variables.
- Prompt the user to provide the desired size and update size variable
- Create the read() and display() functions to read and print the array elements
- Take the array elements from the user and update the array using read() function.
- Display the array elements using the display() function.
- Now, We need to sort the array of elements using the qsort() function.
- The
qsort() function needs a
compare_function, Define a compare function named
compare_asc.
- Prototype of the compare_asc function is int compare_asc(const void *x, const void *y)
- Compare function compares the values pointed by the
x and
y variables and returns
- A Positive Number if the variable x should be placed after the variable y in ascending order
- A Negative value if variable x should be placed before y in ascending order
- or Returns Zero(0) if both the variables x and y are equal.
- Make the call to
qsort() function with parameters
values array,
size, and
sizeof(int), and
compare_asc function pointer.
- qsort(values, size, sizeof(int), compare_asc );
- Print the sorted Array on the console.
The QSort Compare function Logic for Sorting Array in Ascending Order:
1 2 3 4 |
int compare_asc(const void *x, const void *y) { return (*(int*)x - *(int*)y); } |
Compare function compares the values pointed out by the x and y variables and returns
- A Positive Number if the variable x should be placed after the variable y in ascending order
- A Negative value if variable x should be placed before y in ascending order
- or Returns Zero(0) if both variables x and y are equal.
Sort Array in Ascending order using QSort function:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 |
/* Program to sort the numbers using qsort inbuilt function Author: SillyCodes.com */ #include <stdio.h> #include <stdlib.h> int compare_asc(const void *x, const void *y) { // Compare the values pointed to by 'x' and 'y' and return // a negative value if 'x' should be placed before 'y' in ascending order, // or a positive value if 'x' should be placed after 'y' in ascending order, // or 0 if they are equal return (*(int*)x - *(int*)y); } /** * @brief - Read the user input and update the 'values' array * * @param values - 'values' array * @param size - size of the 'values' array */ void read(int values[], int size) { int i; // User input for array elements printf("Please enter array elements(%d) : ", size); for(i = 0; i < size; i++) { // printf("values[%d] : ", i); scanf("%d", &values[i]); } } /** * @brief - Display the 'values' array * * @param values - Array * @param size - Array size */ void display(int values[], int size) { int i; for(i = 0; i < size; i++) { printf("%d ", values[i]); } printf("\n"); } int main() { // declare the 'values' array int values[100]; int i, j, size, temp; printf("Enter desired array size(1-100): "); scanf("%d", &size); // User input for array elements read(values, size); // Print the original array printf("Original Array: "); display(values, size); // call 'qsort' with 'compare_asc' function // 'compare_asc' is function pointer with // int (*_PtFuncCompare)(const void *, const void *)) syntax qsort(values, size, sizeof(int), compare_asc ); // Print the sorted array printf("Sorted Array (Ascending Order): "); display(values, size); return 0; } |
Program Output:
Compile and Run the program using GCC (any compiler)
Test 1:
1 2 3 4 5 6 7 |
$ gcc sort-array-qsort.c $ ./a.out Enter desired array size(1-100): 10 Please enter array elements(10) : 45 85 25 14 -52 -63 0 74 63 25 Original Array: 45 85 25 14 -52 -63 0 74 63 25 Sorted Array (Ascending Order): -63 -52 0 14 25 25 45 63 74 85 $ |
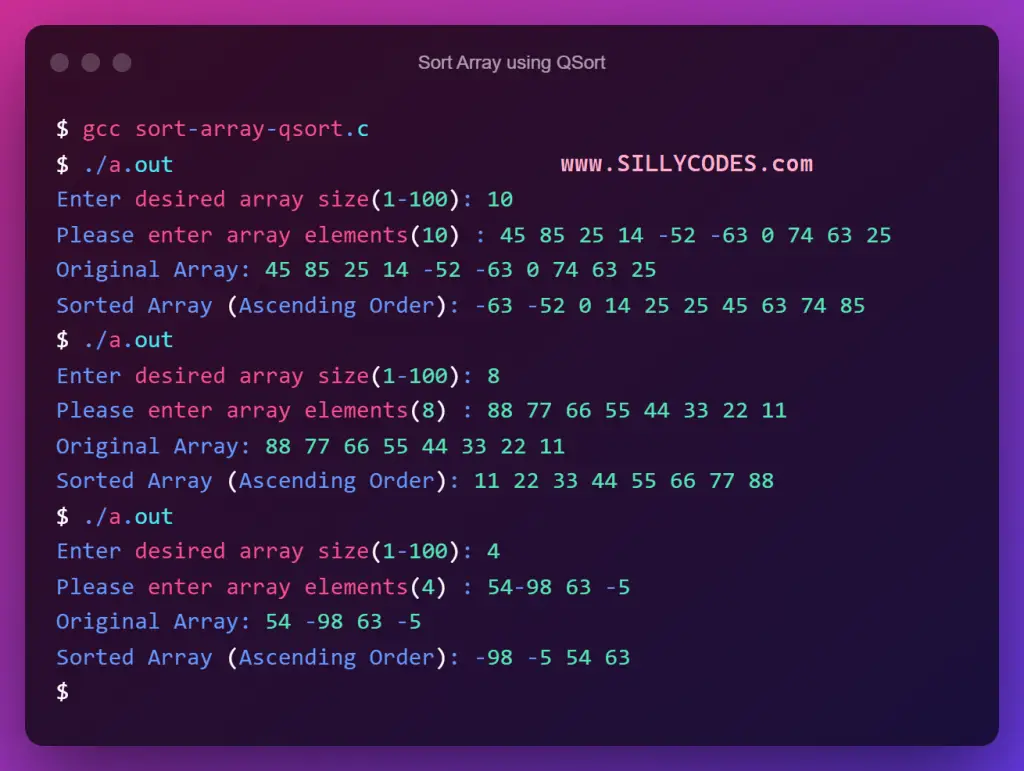
Test 2:
1 2 3 4 5 6 7 8 9 10 11 |
$ ./a.out Enter desired array size(1-100): 8 Please enter array elements(8) : 88 77 66 55 44 33 22 11 Original Array: 88 77 66 55 44 33 22 11 Sorted Array (Ascending Order): 11 22 33 44 55 66 77 88 $ ./a.out Enter desired array size(1-100): 4 Please enter array elements(4) : 54-98 63 -5 Original Array: 54 -98 63 -5 Sorted Array (Ascending Order): -98 -5 54 63 $ |
The program is providing the expected output.
2. Sorting Array in Descending Order Using QSort Function:
Sort Array – Descending Order Program Explanation:
The program is very similar to the above Ascending order program except for the compare function ( compare_func).
We need to change the compare function so that, the qsort() function sorts the array in descending order.
Compare function logic for QSort() to sort elements in Descending order:
Define a compare function named compare_dsc.
1 2 3 4 |
int compare_dsc(const void *x, const void *y) { return (*(int*)y - *(int*)x); } |
- Prototype of the compare_dsc function is int compare_dsc(const void *x, const void *y)
- Compare function compares the values pointed out by the
x and
y variables and returns
- A Positive value if variable x should be placed before y in descending order
- A Negative Number if the variable x should be placed after the variable y in descending order
- or Returns Zero(0) if both variables x and y are equal.
Sort Array in Descending order using QSort function:
Here is the program to sort the array in descending order using the qsort() function in the c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 |
/* Program to sort the numbers using qsort inbuilt function Author: SillyCodes.com */ #include <stdio.h> #include <stdlib.h> int compare_dsc(const void *x, const void *y) { // Compare the values pointed to by 'x' and 'y' and return // a positive value if 'x' should be placed before 'y' in descending order, // or a negative value if 'x' should be placed after 'y' in descending order, // or 0 if they are equal return (*(int*)y - *(int*)x); } /** * @brief - Read the user input and update the 'values' array * * @param values - 'values' array * @param size - size of the 'values' array */ void read(int values[], int size) { int i; // User input for array elements printf("Please enter array elements(%d) : ", size); for(i = 0; i < size; i++) { // printf("values[%d] : ", i); scanf("%d", &values[i]); } } /** * @brief - Display the 'values' array * * @param values - Array * @param size - Array size */ void display(int values[], int size) { int i; for(i = 0; i < size; i++) { printf("%d ", values[i]); } printf("\n"); } int main() { // declare the 'values' array int values[100]; int i, j, size, temp; printf("Enter desired array size(1-100): "); scanf("%d", &size); // User input for array elements read(values, size); // Print the original array printf("Original Array: "); display(values, size); // call 'qsort' with 'compare_dsc' function // 'compare_dsc' is function pointer with // int (*_PtFuncCompare)(const void *, const void *)) syntax qsort(values, size, sizeof(int), compare_dsc ); // Print the sorted array printf("Sorted Array (Descending Order): "); display(values, size); return 0; } |
Program Output:
Compile and Run the Program.
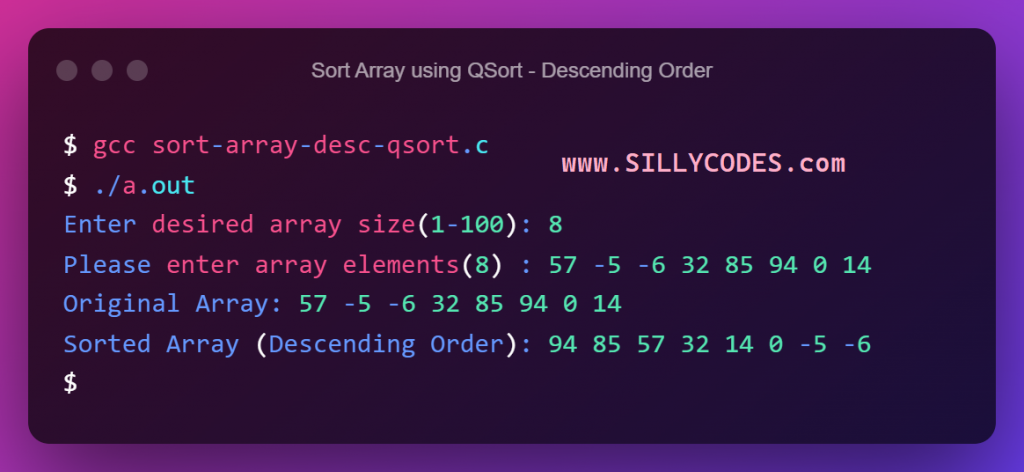
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$ gcc sort-array-desc-qsort.c $ ./a.out Enter desired array size(1-100): 8 Please enter array elements(8) : 57 -5 -6 32 85 94 0 14 Original Array: 57 -5 -6 32 85 94 0 14 Sorted Array (Descending Order): 94 85 57 32 14 0 -5 -6 $ ./a.out Enter desired array size(1-100): 6 Please enter array elements(6) : 111 222 333 777 888 999 Original Array: 111 222 333 777 888 999 Sorted Array (Descending Order): 999 888 777 333 222 111 $ ./a.out Enter desired array size(1-100): 3 Please enter array elements(3) : 5 0 -4 Original Array: 5 0 -4 Sorted Array (Descending Order): 5 0 -4 $ |
As we can from the above output, The program is sorting the array elements in descending order.
Related Array Programs:
- Index – C Language Practice Programs
- C Tutorials Index – Step by step tutorials to master C Langauge
- C Program to Read and Print Arrays
- Reverse Print Array Program in C
- C Program to calculate Sum of all array Elements
- C Program to Calculate the Average of all array Elements
- C Program to find minimum element in Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Find Maximum Element in Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
13 Responses
[…] better understand the following program, Please go through the C Arrays, Functions in C, and How to Pass Arrays to Functions […]
[…] C Program to Sort Array in Ascending and Descending Order using QSort function […]
[…] Arrays in C Language with Example Programs […]
[…] Arrays in C Language with Example Programs […]
[…] C Arrays […]
[…] One-dimensional Arrays in C […]
[…] C Arrays […]
[…] One dimensional Arrays in C […]
[…] C Arrays […]
[…] One dimensional Arrays in C […]
[…] C Program to Sort Array in Ascending and Descending Order using QSort function […]
[…] C Arrays […]
[…] C Program to Sort Array in Ascending and Descending Order using QSort function […]