Program to Read and Print 2d Array in C Language
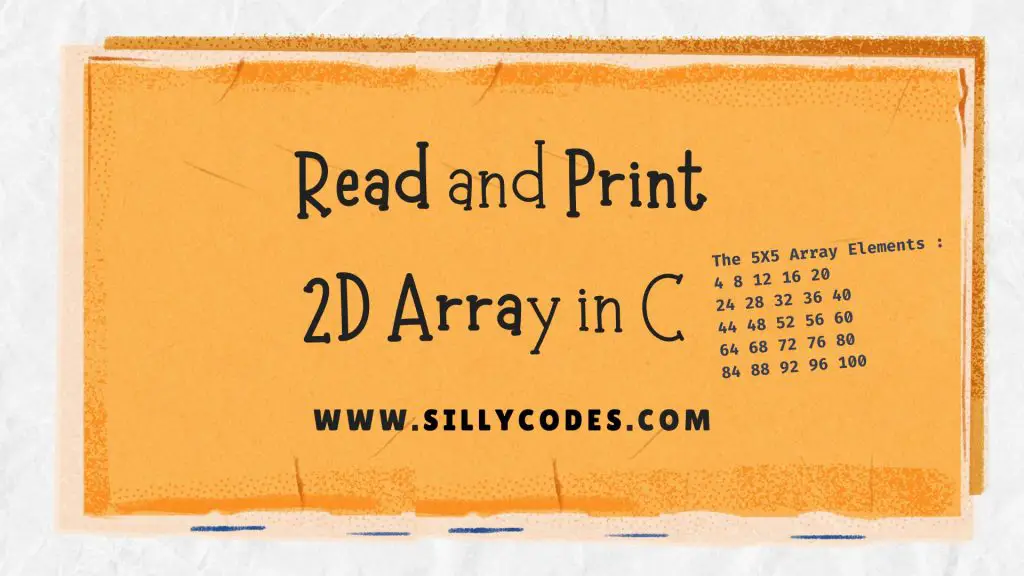
Read and Print 2d Array in C Program Description:
Write a Program to Read and Print 2D Array in C programming language. The program should take the user input for the 2D Array or Maxtrix and update the Matrix. Then it should print the given 2D-Array on the console.
This program will help us to understand how to take user input and update the 2D Array (Matrix) and How to iterate over the 2D Array and print the elements of the 2D Array.
What is a 2D Array or Matrix:
The Two Dimensional Arrays or 2-D arrays are collections of one-dimensional arrays ( Array of 1-D Arrays). We can create two-dimensional arrays by specifying the two subscripts [ ].
The two-dimensional array elements are stored in the contiguous memory. We can represent a 2-D array as the Matrix.
The 2-D arrays are used to store and manipulate data in tabular format, with each row representing a different record and each column representing a different field or attribute of that record.
Declaring the 2D Array or Matrix:
data_type array_name[row_size][column_size];
We have already covered the 2D Arrays in detail in earlier articles, Please go through the following links to learn more about the 2D Arrays and Multi-dimensional arrays in C.
- 2D-Arrays in C – How to Declare, Access, and Modify 2D arrays
- One-dimensional Arrays in C
- Passing 2D Arrays to Functions
Let’s look at the Example Input and Output of the Program.
Example Input and Output:
Input:
1 2 3 4 5 6 7 8 9 10 |
Read 2DArray or Matrix: Provide Elements for 3X3 Array values[0][0] : 100 values[0][1] : 200 values[0][2] : 300 values[1][0] : 400 values[1][1] : 500 values[1][2] : 600 values[2][0] : 700 values[2][1] : 800 values[2][2] : 900 |
Output:
1 2 3 4 |
Print 2DArray or Maxtrix: Given 3X3 Array Elements : 100 200 300 400 500 600 700 800 900 |
As we can see from the above output, The program should take the user-provided values and update the 2D array and print it back on the console.
Read and Print 2d Array in C Program Explanation:
Let’s look at the step-by-step explanation of the Read and Print 2D array in the C Program.
- Start the program by creating two Constants called ROWS and COLUMNS. The ROWS constant holds the number of rows in the 2D Array and the COLUMN constant holds the number of columns in the 2D Array. ( We can also use Macros instead of constants)
- Create a 2D Array or Matrix named values with the row size of ROWS and column size of COLUMN. ( values[ROWS][COLUMNS];)
- Now, We need to Read the input from the user and update the values 2D Array.
- To Read user input, We need to create two For Loops, One For Loop will iterate over the ROWS, and the Second For Loop will iterate over the COLUMNS.
- Create the First For Loop ( Outer Loop), Start the iteration from i=0 and go till the i<ROWS (i.e for(i=0; i<ROWS; i++) ). The Outer Loop will iterate over the ROWS and At Each iteration of the Outer Loop.
- Create the second For Loop ( Inner Loop). This loop is a Nested loop that will be inside the Outer Loop. Start the Loop from j=0 and go till the j<COLUMNS (i.e for(j=0; j<COLUMNS; j++) ). The Inner Loop will iterate over the COLUMNS. At each iteration of the Inner Loop
- Prompt the user to provide the Matrix or 2D Array element and read it using the scanf function and Update the values[i][j] element.
- Repeat the above step for all elements in the array
- Once the above Two Loops are completed, The values[][] 2D Array will be updated with user-provided values.
- Now, We need to Print the Array elements on the Standard Output.
- To Print the 2D Array elements or Matrix Elements, Similar to the reading of a 2D array, We need to use two For Loops. One for iterating over the ROWS and another for Iterating over the COLUMNS.
- Create Outer For Loop. Start from i=0 to i<ROWS (which is for(i=0; i<ROWS; i++) )
- Create Inner For Loop, Start from j=0 to j<COLUMNS ( which is for(j=0; j<COLUMNS; j++) )
- Print the 2D Array or Matrix Element values[i][j] using the printf function.
- printf("%d ", values[i][j]);
- Create Inner For Loop, Start from j=0 to j<COLUMNS ( which is for(j=0; j<COLUMNS; j++) )
- Create Outer For Loop. Start from i=0 to i<ROWS (which is for(i=0; i<ROWS; i++) )
- Once the above two for loops are completed, All the elements of the values array will be printed on the console.
- Stop the Program.
Program to Read and Print 2d Array in C Language:
Here is the program to Read and Print 2D array or Matrix in C programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
/* Program to Read and Print the 2D array or Matrix Sillycodes.com */ #include<stdio.h> const int ROWS = 3; // 2DArray Rows const int COLUMNS = 3; // 2DArray Columns int main() { int i, j; // Initialize the 2-D array // Array with 3-Rows and 3-Columns int values[ROWS][COLUMNS]; // Read 2DArray or Matrix // Take the user input and update the array. printf("Read 2DArray or Matrix: Provide Elements for %dX%d Array\n", ROWS, COLUMNS); // iterate over each row for(i=0; i<ROWS; i++) { // iterate over each column for(j=0; j<COLUMNS; j++) { // Take user input and update `values[i][j]` printf("values[%d][%d] : ", i, j); scanf("%d", &values[i][j]); } } // Print the 2DArray or Matrix printf("Print 2DArray or Maxtrix: Given %dX%d Array Elements : \n", ROWS, COLUMNS); // iterate over each ROW for(i=0; i<ROWS; i++) { // iterate over each column for(j=0; j<COLUMNS; j++) { printf("%d ", values[i][j]); } // add new line after each row printf("\n"); } return 0; } |
Program Output:
Let’s compile and Run the Program.
Compile the program using the following command.
$ gcc read-print-2darray.c
Run the executable file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
$ ./a.out Read 2DArray or Matrix: Provide Elements for 3X3 Array values[0][0] : 100 values[0][1] : 200 values[0][2] : 300 values[1][0] : 400 values[1][1] : 500 values[1][2] : 600 values[2][0] : 700 values[2][1] : 800 values[2][2] : 900 Print 2DArray or Maxtrix: Given 3X3 Array Elements : 100 200 300 400 500 600 700 800 900 $ |
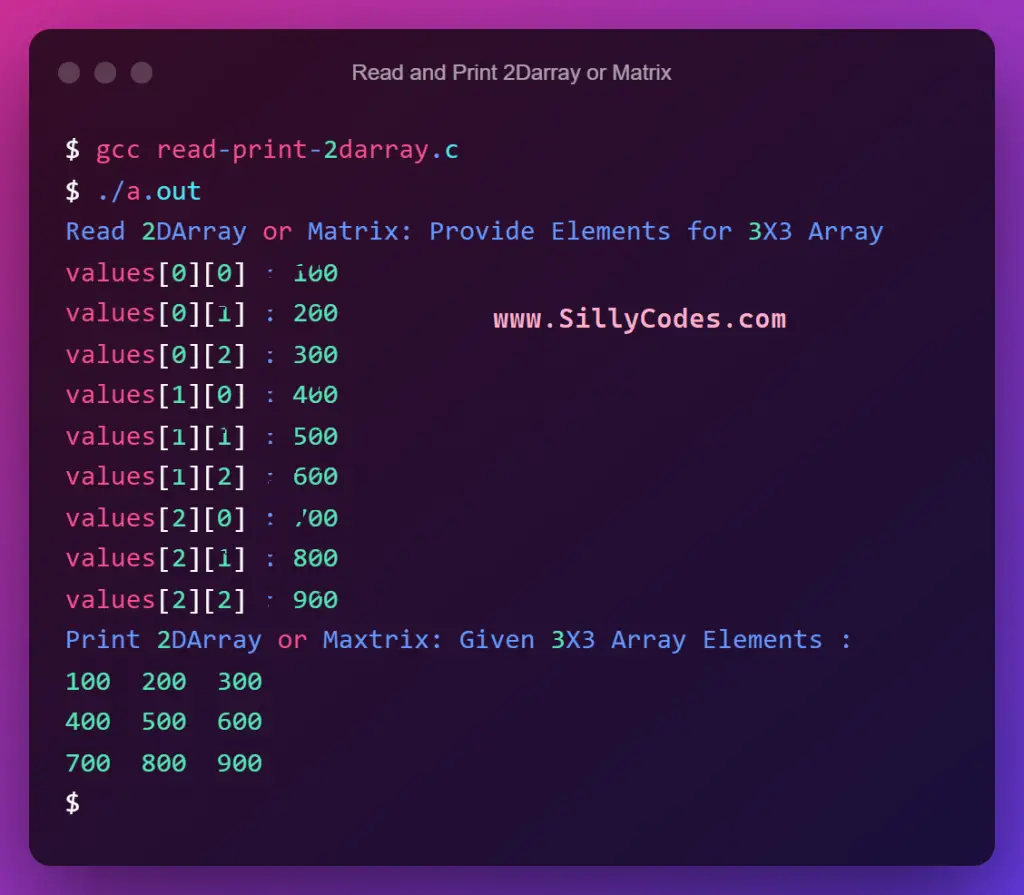
Let’s try a few more examples
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
$ gcc read-print-2darray.c $ ./a.out Read 2DArray or Matrix: Provide Elements for 3X3 Array values[0][0] : 11 values[0][1] : 22 values[0][2] : 33 values[1][0] : 44 values[1][1] : 55 values[1][2] : 66 values[2][0] : 77 values[2][1] : 88 values[2][2] : 99 Print 2DArray or Maxtrix: Given 3X3 Array Elements : 11 22 33 44 55 66 77 88 99 $ $ ./a.out Read 2DArray or Matrix: Provide Elements for 3X3 Array values[0][0] : 1 values[0][1] : 2 values[0][2] : 3 values[1][0] : 4 values[1][1] : 5 values[1][2] : 6 values[2][0] : 7 values[2][1] : 8 values[2][2] : 9 Print 2DArray or Maxtrix: Given 3X3 Array Elements : 1 2 3 4 5 6 7 8 9 $ |
As we can see from the above output, The program is Reading the 2D array elements from the user and displaying the 2D array on the console.
Read and Print Matrix in C with Custom Row and Column sizes:
In the above program, We have hard-coded the array size as 3X3, Let’s modify the program to allow us to use the Custom Row and Column sizes.
To use the custom Row size and column size, We can create an array that can hold a large number of elements. Here we are taking 100 as the max row( MAXROWS) and column( MAXCOLUMNS) sizes. So user can input any row and column size between one(1) to hundred(100).
Here is the modified version of Read and Print 2D Array program, Where we will ask user fo the custom Row and Column size.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
/* Program to Read and Print the 2D array or Matrix with Custom rows and columns Sillycodes.com */ #include<stdio.h> // Create 'MAXROWS' and "MAXCOLUMNS" constants. // If you are using same number, then we can also use single constant. const int MAXROWS=100; const int MAXCOLUMNS=100; int main() { // Declare a 2D-Array or matrix with size MAXROWS X MAXCOLUMNS int numbers[MAXROWS][MAXCOLUMNS]; int rows, columns, i, j; // Take the Row size and Column size from user printf("Enter the Row size for Matrices(1-%d): ", MAXROWS); scanf("%d", &rows); printf("Enter the Column Size for Matrices(1-%d): ", MAXCOLUMNS); scanf("%d", &columns); // check for array bounds if(rows >= MAXROWS || rows <= 0 || columns >= MAXCOLUMNS || columns <= 0) { printf("Invalid Row or Column, Please Try again \n"); return 0; } // Read 2DArray or Matrix // Take the user input and update the array. printf("Read 2DArray or Matrix: Provide Elements for %dX%d Array\n", rows, columns); // iterate over each row for(i=0; i<rows; i++) { // iterate over each column for(j=0; j<columns; j++) { // Take user input and update `numbers[i][j]` printf("numbers[%d][%d] : ", i, j); scanf("%d", &numbers[i][j]); } } // Print the 2DArray or Matrix printf("Print 2DArray or Maxtrix: Given %dX%d Array Elements : \n", rows, columns); // iterate over each ROW for(i=0; i<rows; i++) { // iterate over each column for(j=0; j<columns; j++) { printf("%d ", numbers[i][j]); } // add new line after each row printf("\n"); } return 0; } |
Program Output:
Compile and Run the Program.
Test 1: Normal Cases:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
$ gcc read-print-2darray-cust.c $ ./a.out Enter the Row size for Matrices(1-100): 5 Enter the Column Size for Matrices(1-100): 5 Read 2DArray or Matrix: Provide Elements for 5X5 Array numbers[0][0] : 4 numbers[0][1] : 8 numbers[0][2] : 12 numbers[0][3] : 16 numbers[0][4] : 20 numbers[1][0] : 24 numbers[1][1] : 28 numbers[1][2] : 32 numbers[1][3] : 36 numbers[1][4] : 40 numbers[2][0] : 44 numbers[2][1] : 48 numbers[2][2] : 52 numbers[2][3] : 56 numbers[2][4] : 60 numbers[3][0] : 64 numbers[3][1] : 68 numbers[3][2] : 72 numbers[3][3] : 76 numbers[3][4] : 80 numbers[4][0] : 84 numbers[4][1] : 88 numbers[4][2] : 92 numbers[4][3] : 96 numbers[4][4] : 100 Print 2DArray or Maxtrix: Given 5X5 Array Elements : 4 8 12 16 20 24 28 32 36 40 44 48 52 56 60 64 68 72 76 80 84 88 92 96 100 $ $ $ ./a.out Enter the Row size for Matrices(1-100): 2 Enter the Column Size for Matrices(1-100): 2 Read 2DArray or Matrix: Provide Elements for 2X2 Array numbers[0][0] : 1 numbers[0][1] : 2 numbers[1][0] : 3 numbers[1][1] : 4 Print 2DArray or Maxtrix: Given 2X2 Array Elements : 1 2 3 4 $ ./a.out Enter the Row size for Matrices(1-100): 2 Enter the Column Size for Matrices(1-100): 5 Read 2DArray or Matrix: Provide Elements for 2X5 Array numbers[0][0] : 10 numbers[0][1] : 20 numbers[0][2] : 30 numbers[0][3] : 40 numbers[0][4] : 50 numbers[1][0] : 60 numbers[1][1] : 70 numbers[1][2] : 80 numbers[1][3] : 90 numbers[1][4] : 100 Print 2DArray or Maxtrix: Given 2X5 Array Elements : 10 20 30 40 50 60 70 80 90 100 $ |
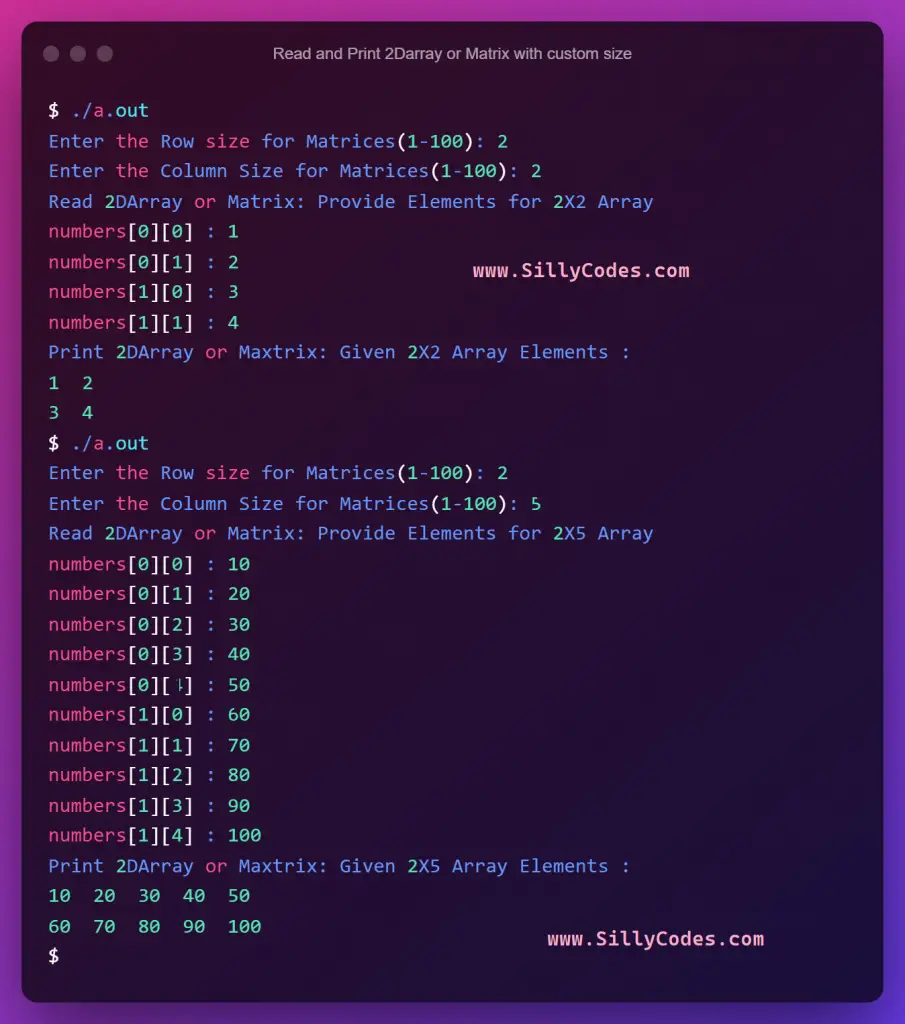
As we can see from the above output, We are able to use the custom Row size and Columns size for the 2D Array or Matrix.
Test 2: When size goes beyond limit.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
// Negative Test Cases $ ./a.out Enter the Row size for Matrices(1-100): 200 Enter the Column Size for Matrices(1-100): 4 Invalid Row or Column, Please Try again $ ./a.out Enter the Row size for Matrices(1-100): 3 Enter the Column Size for Matrices(1-100): 1000 Invalid Row or Column, Please Try again $ ./a.out Enter the Row size for Matrices(1-100): 500 Enter the Column Size for Matrices(1-100): 600 Invalid Row or Column, Please Try again $ |
When the input size for row or column goes beyond the limit, Then program will display an error message ( Invalid Row or Column, Please Try again)
Related Array Programs:
- C Program to find minimum element in Array
- C Program to Find Maximum Element in Array
- C Program to Find Second Largest Element in Array
- C Program to Insert an element in Array
- C Program to Delete an Element in Array
- C Program to Find Unique Elements in an Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Delete Duplicate Elements from Sorted Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
6 Responses
[…] our previous article, We have looked at How to Read and Print the 2D Array elements in C language, In today’s article, We will look at Passing 2d Array to Function in C programming language with […]
[…] have looked at the Reading and Printing Matrices and How to Pass Matrices to functions programs in earlier articles, In today’s article, We will […]
[…] our previous articles, we looked at the Read and Print Matrices Program, In today’s article, We are going to look at the program to perform the Subtraction of […]
[…] Read and Print 2D Arrays in C […]
[…] C Program to Read and Print Matrix or 2D Array […]
[…] Read and Print 2D Arrays in C […]