Print String Elements using Pointers in C Language
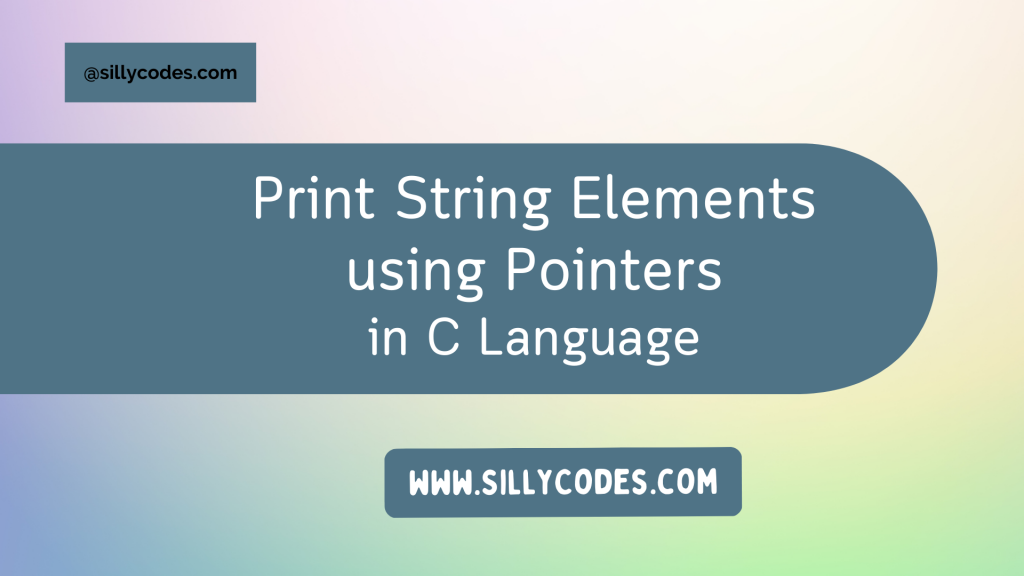
Print String Elements using Pointers Program Description:
Write a Program to demonstrate how to print string elements using pointers in c programming language. The program should accept a string from the user and print all string characters using a pointer. To access the string elements, we will use standard pointer notation (by dereferencing the pointer) as well as pointer subscript notation.
Here is the example input and output of the program.
Example Input and Output:
Input:
Enter a string : code
Output:
chPtr[0]:c
chPtr[1]:o
chPtr[2]:d
chPtr[3]:e
Prerequisites of the program:
Please go through the following C tutorials to better understand the program.
- Pointers in C Programming Language – Declare, Initialize, and use pointers
- Pointer Arithmetic in C Language
- Arrays in C with Example Programs
- Strings in C language – How to Create and use strings in C
Program to Print string elements using pointers in C Language:
Here we used the character pointer chPtr to print the elements of the string inputStr.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
/* program to understand strings and pointers in c sillycodes.com */ #include<stdio.h> // Max Size of string const int SIZE = 1000; int main() { // Declare a string with 'SIZE' char inputStr[SIZE]; int start, end, temp; // Get the string from the user printf("Enter a string : "); gets(inputStr); // Initialize a character pointer and assign 'inputStr' char * chPtr = inputStr; // Display the string using pointer 'chPtr' for(int i=0; chPtr[i]; i++) { printf("chPtr[%d]:%c\n", i, *(chPtr+i)); } return 0; } |
We started the program by declaring a string called inputStr to hold the input string with the SIZE characters. Then we prompted the user to provide the string and stored it in the inputStr using a gets function.
Then we created a character pointer chPtr and initialized the chPtr with the inputStr string.
char * chPtr = inputStr;
Finally, We used a for loop to iterate over the characters of the input string inputStr and accessed/printed the string elements using the pointer chPtr and index ( i) – *(chPtr+i)
1 2 3 4 |
for(int i=0; chPtr[i]; i++) { printf("chPtr[%d]:%c\n", i, *(chPtr+i)); } |
Program Output:
Let’s compile and run the program using your favorite compiler. We are using the GCC compiler on ubuntu Linux OS.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
$ gcc access-string.c $ ./a.out Enter a string : Learn C Programming at SillyCodes.com chPtr[0]:L chPtr[1]:e chPtr[2]:a chPtr[3]:r chPtr[4]:n chPtr[5]: chPtr[6]:C chPtr[7]: chPtr[8]:P chPtr[9]:r chPtr[10]:o chPtr[11]:g chPtr[12]:r chPtr[13]:a chPtr[14]:m chPtr[15]:m chPtr[16]:i chPtr[17]:n chPtr[18]:g chPtr[19]: chPtr[20]:a chPtr[21]:t chPtr[22]: chPtr[23]:S chPtr[24]:i chPtr[25]:l chPtr[26]:l chPtr[27]:y chPtr[28]:C chPtr[29]:o chPtr[30]:d chPtr[31]:e chPtr[32]:s chPtr[33]:. chPtr[34]:c chPtr[35]:o chPtr[36]:m $ |
As we can see from the above output, The program is properly printing the string elements using the pointer.
Method 2: Print string elements using pointers subscript notation in C:
Instead of using the *(chPtr+i) we can simply use the pointer subscript [ ] notation chPtr[i] ( Both these notations are the same). Here is the program to print the string characters using pointer subscript notation in C programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
/* program to print string elements using pointers in c sillycodes.com */ #include<stdio.h> // Max Size of string const int SIZE = 1000; int main() { // Declare a string with 'SIZE' char inputStr[SIZE]; int start, end, temp; // Get the string from the user printf("Enter a string : "); gets(inputStr); // Initialize a character pointer and assign 'inputStr' char * chPtr = inputStr; // Display the string using pointer 'chPtr' for(int i=0; chPtr[i]; i++) { printf("chPtr[%d]:%c\n", i, chPtr[i]); } return 0; } |
Note that, We used the pointer subscript notation chPtr[i] in this example.
Program Output:
Compile the program.
$ gcc print-string-ptr.c
Run the program. ( ./a.out)
1 2 3 4 5 6 7 |
$ ./a.out Enter a string : code chPtr[0]:c chPtr[1]:o chPtr[2]:d chPtr[3]:e $ |
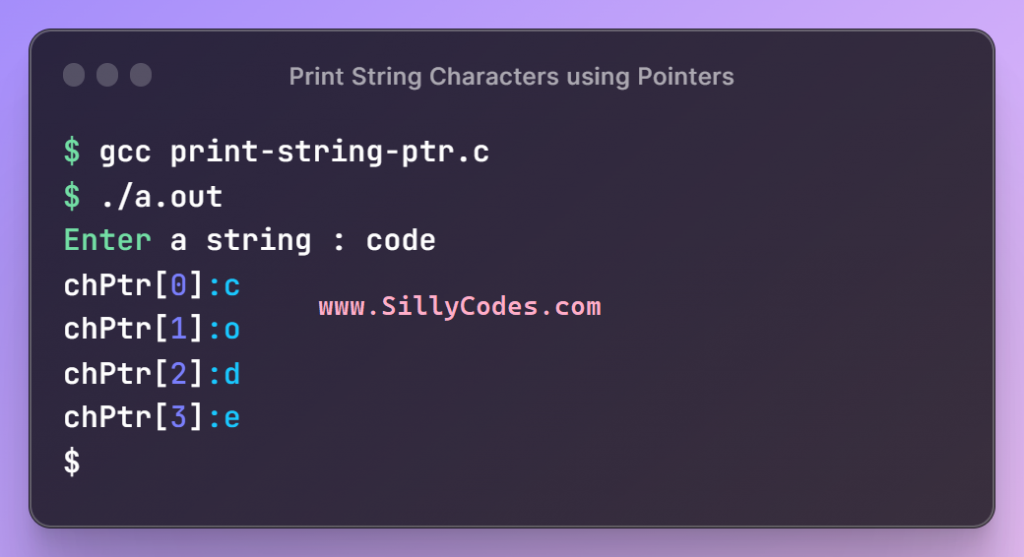
As we can see from the above output, We are getting the expected output.
Related Pointers Programs:
- Program to Demonstrate Address of Operator (’&’) in C Language
- Program to Understand the Dereference or Indirection Operator in C Language
- Size of Pointer variables in C Language
- Program to Add Two Numbers using Pointers in C
- Program to perform Arithmetic Operations using Pointers in C
- Swap Two Numbers using Pointers in C Language
- Accessing Array elements using pointers in C language
- C Tutorials Index
- C Programs Index – 300+ Programs