Program to calculate nth prime number in C Langauge
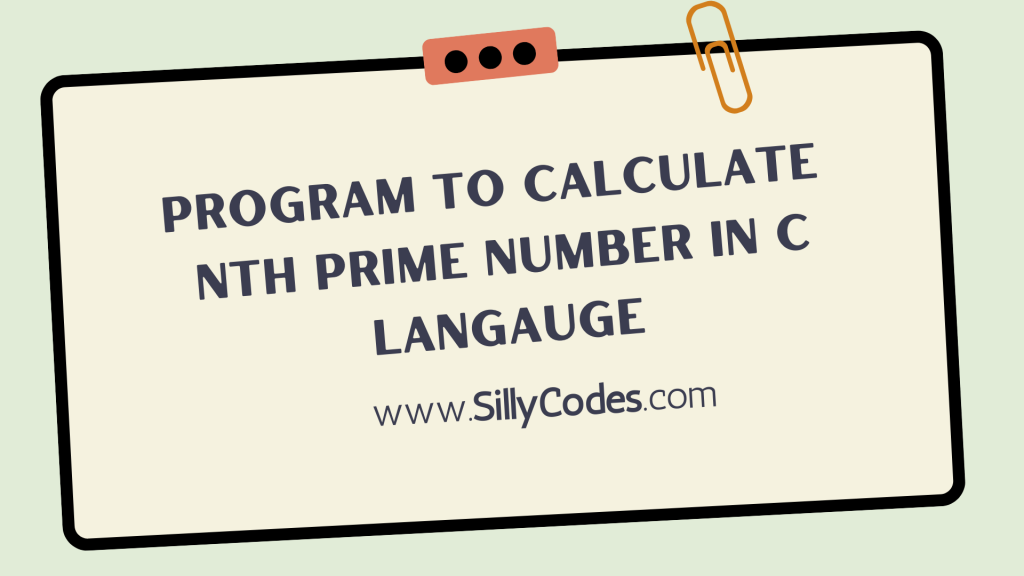
Program Description:
Write a program to calculate the nth prime number in C language. The program will accept a positive number from the user (let’s say the number as n) and provides the nth (user-provided number) prime number.
For example, If the user enters 6, Then the program should calculate and display the 6th prime number which is 13
Here is the list of the first 100 prime numbers
First 100 Prime Numbers:
2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241, 251, 257, 263, 269, 271, 277, 281, 283, 293, 307, 311, 313, 317, 331, 337, 347, 349, 353, 359, 367, 373, 379, 383, 389, 397, 401, 409, 419, 421, 431, 433, 439, 443, 449, 457, 461, 463, 467, 479, 487, 491, 499, 503, 509, 521, 523, 541
Excepted Input and Output of Program:
Input:
Enter a Positive Number (n) : 10
Output:
10(th) Prime Number : 29
Related Prime Numbers Programs:
This program is part of a series of prime number generation programs. Here are other articles.
- What is Prime Number and C program to Check given number is Prime or Not
- Check given Number is Prime or not Using Square Root(sqrt) Function.(Efficient way)
- C Program to generate prime numbers between two numbers
- C Program to generate first N prime numbers.
- C program to print prime numbers up to n
Pre-Requisites:
It will be helpful to know the C-Language Loops. Please go through the following links to learn about the C Loops.
nth prime number in C program Algorithm:
- First of all, Take the input from the user and store it in a variable n
- Check if the
n is a Positive number or a Negative number. If the n is a negative number, Display an error message –
Please enter positive number, Try Again and ask for input again.
- We use the Goto Statement to jump from one statement to another. So we used goto START; (at line 16), So the program will jump from this line to goto label START: (at line 7).
- We will use two for loops. Outer loop and Inner loop to calculate the nth prime number.
- The Outer loop is will count the number of prime numbers we got, So that we can calculate prime numbers up to n and stop the loop once we reach the nth prime number.
- The Outer loop will start from
2 (loop variable
i will be used) and goes until we find our
nth prime number.
- In order to keep track of the number of prime numbers, we also utilize a counter ( cnt). Each time we discover a prime number, we add one to the counter or cnt variable.
- The Inner loop will be normal prime number check logic. We will check if the
i (Outer loop variable) is evenly divisible by any number from
2 to
j/2 ( We can also use the Square root method to check prime numbers, But for simplicity, we are sticking to this method)
- A number won’t be a prime number if it is perfectly divisible by any other integer (aside from 1 and the number itself).
- The Outer loop will continue until we get the n prime numbers ( Loop condition cnt<n ) and the Outer loop will terminate once we got our nth prime number
nth prime number in c Program using for loop:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
/* Program: nth prime number in C Program Author: sillycodes.com */ #include<stdio.h> int main() { int i,j,cnt,FLAG,n,nthPrime; cnt = 0; // we will increase this count by 1 for each prime START: // goto statement label // Take the input from the user printf("Enter a Positive Number (n) : "); scanf("%d",&n); if(n <= 0) { printf("Please enter positive number, Try Again , Try Again \n"); goto START; } // starts from '2', As we know the first prime is '2' for(j=2; cnt<n ; j++) { FLAG=0; // set this flag to zero for each iteration // Inner Loop goes from '2' to 'j/2' // we can also use the sqrt method - https://sillycodes.com/prime-number-program-in-c-using-sqrt/ for(i=2;i <= j/2; i++) { if(j%i == 0) { // Set FLAG to 1 if given number is evenly // divisible by any other number b/w 2 & j/2 FLAG = 1; break; } } // if flag is still zero, that means Given Number is Prime. if(FLAG == 0) { // Number is primes, so let's print it now. // increase count for each prime number cnt++ ; // update the nth prime nthPrime = j; } } printf("%d(th) Prime Number : %d \n", n, nthPrime); return 0; } |
Program Output:
We are using the GCC compiler to compile the program
$ gcc nth-prime.c
The above command generates the executable file, by default a.out is our executable file name. Run the Program.
1 2 3 4 5 6 7 |
$ ./a.out Enter a Positive Number (n) : 10 10(th) Prime Number : 29 $ ./a.out Enter a Positive Number (n) : 100 100(th) Prime Number : 541 $ |
If the user enters a negative number, Program should display an error message
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Positive Number (n) : -8 Please enter positive number, Try Again Enter a Positive Number (n) : -2 Please enter positive number, Try Again Enter a Positive Number (n) : 6 6(th) Prime Number : 13 $ |
The program will display an error notice if the user enters any negative numbers, as we can see from the output above. Additionally, we asked the user to input again. Until the user provides a valid positive number, this process continues.
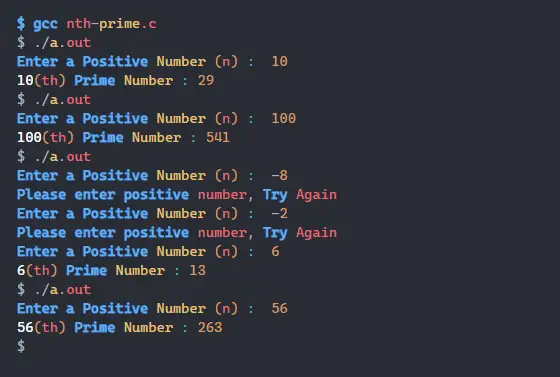
Exercise:
Here are a couple of practice programs you can try.
- Rewrite the above program using the while loop
- Calculate the nth prime number using the sqrt method
📢 You can learn more about the Square root-based prime number checking program in the following article
5 Responses
[…] C Program to Print nth Prime Number in C […]
[…] C Program to Print nth Prime Number in C […]
[…] C Program to Calculate Nth Prime Number […]
[…] C Program to Calculate Nth Prime Number […]
[…] C Program to Calculate Nth Prime Number […]