Count Number of Digits in Number program in C
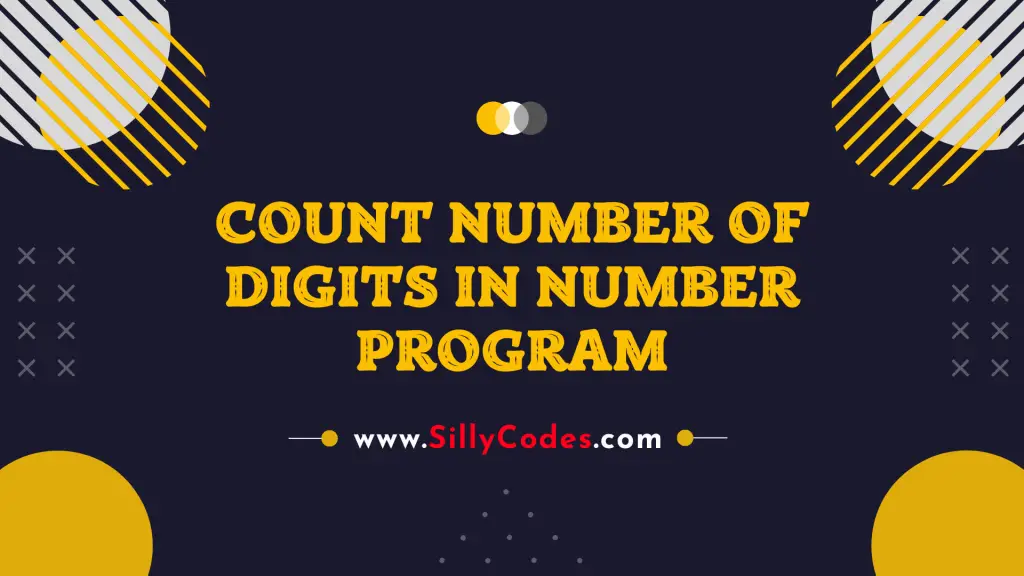
Program Description:
Write a program to Count the number of digits in C language using loops (while and for, etc). The program should accept a positive number from the user and count the number of individual digits in the given number. Also, display the error message on invalid input.
Here is an example of inputs and outputs.
Expected Inputs and Outputs:
Example 1: Input:
Enter a positive number : 76532
Output:
Number of Digits in 76532 number are: 5
Example 2: Input:
Enter a positive number : 2
Output:
Number of Digits in 2 number are: 1
Pre Requisites:
It is recommended to have Knowledge of C Loops and Arithmetic operators. Please go through the following articles.
- While Loop in C Language
- For Loop in C Language with Example Programs
- Goto Statement in C
- Division Operator
Program to Count the number of digits in C Algorithm:
- Take the Input from the user and store it in variable num.
- If the user enters a positive number then iterate over the number using the loop
- Take the backup of the num to temp – This step is optional, You can directly use the num as well.
- We will iterate over the number using the division operator. I.e
temp = temp/10,
- We can remove the last digit from the number by dividing the given number by 10.
- For example: 123 / 10 = 12
- We can remove the last digit from the number by dividing the given number by 10.
- At each iteration, Increment the counter ( digitCnt)
- The loop will run until the number is Greater-than 0 ( temp > 0 )
- The counter ( digitCnt) will have the total number of digits in the number ( num or temp)
- If the user enters invalid input like a negative number. Display the error message –
ERROR: Please enter valid number, Try Again.
- Allow the user to enter the number again using the Goto Statement, which can be used to jump from one statement in the function to another statement.
Program Count number of digits in C language using for loop:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
/* Program: Number of digits of number using while loop */ #include<stdio.h> int main() { int num, temp; // goto statement label. If user enters invalid input, we will request for number again. INPUT: // User Input printf("Enter a Positive Number: "); scanf("%d", &num); // digitCnt (digitCount) will be zero at the start int digitCnt = 0; if(num > 0) { // Number is positive // take backup of 'num', we can also directly use 'num' temp = num; // Iterate over each digit using division operator. while(temp > 0) { // increment the number of digits count (digitCnt) digitCnt++; // remove the last element using division operator. temp = temp / 10; } } else { // Negative Number, Display error message and return. printf("ERROR: Please enter valid number, Try Again.\n"); goto INPUT; } // print the product of digits on console. printf("Number of Digits in %d number are: %d \n", num, digitCnt); return 0; } |
Output:
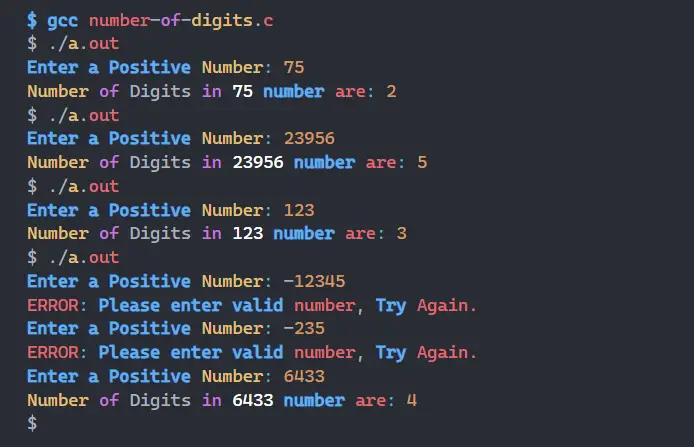
Compile and run the program.
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc number-of-digits.c $ ./a.out Enter a Positive Number: 75 Number of Digits in 75 number are: 2 $ ./a.out Enter a Positive Number: 23956 Number of Digits in 23956 number are: 5 $ ./a.out Enter a Positive Number: 123 Number of Digits in 123 number are: 3 $ |
If the user enters a Negative number.
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Positive Number: -12345 ERROR: Please enter valid number, Try Again. Enter a Positive Number: -235 ERROR: Please enter valid number, Try Again. Enter a Positive Number: 6433 Number of Digits in 6433 number are: 4 $ |
As you can see from the above output, If the user enters a Negative number, The program asked for the number again. ( because of the goto INPUT; and INPUT goto statement and labels.)
Count the Number of Digits in a Number Program in C using for loop:
Here is the program with the for loop. The Program logic remains the same as the above while loop example, but as it is for loop, We can use the for loops Init and Update steps to update our number ( temp).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
/* Program: Number of digits of number using for loop */ #include<stdio.h> int main() { int num, temp; // goto statement label. If user enters invalid input, we will request for number again. INPUT: // User Input printf("Enter a Positive Number: "); scanf("%d", &num); // digitCnt (digitCount) will be zero at the start int digitCnt = 0; if(num > 0) { // Number is positive // take backup of 'num', we can also directly use 'num' // Iterate over each digit using division operator. for(temp = num; temp > 0; temp = temp/10) { // increment the number of digits count (digitCnt) digitCnt++; } } else { // Negative Number, Display error message and return. printf("ERROR: Please enter valid number, Try Again.\n"); goto INPUT; } // print the product of digits on console. printf("Number of Digits in %d number are: %d \n", num, digitCnt); return 0; } |
Program Output:
1 2 3 4 5 6 7 8 |
$ gcc number-of-digits.c $ ./a.out Enter a Positive Number: 123 Number of Digits in 123 number are: 3 $ ./a.out Enter a Positive Number: 564678 Number of Digits in 564678 number are: 6 $ |
Let’s test the negative number test case as well.
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Positive Number: -3647 ERROR: Please enter valid number, Try Again. Enter a Positive Number: -495 ERROR: Please enter valid number, Try Again. Enter a Positive Number: 4 Number of Digits in 4 number are: 1 $ |
Practice Program:
- Try to rewrite the above program using the do while loop and goto statement.
5 Responses
[…] Program to count number of digits of a Number in C […]
[…] C Program to Count the number of Digits of a Number […]
[…] C Program to Count the number of Digits of a Number […]
[…] need to Calculate the Number of digits in the number. Which is used to calculate the power. We used a function countDigits and passed the input number […]
[…] C Program to Count the number of Digits of a Number […]