Program to find Second Smallest number in Array in C Language
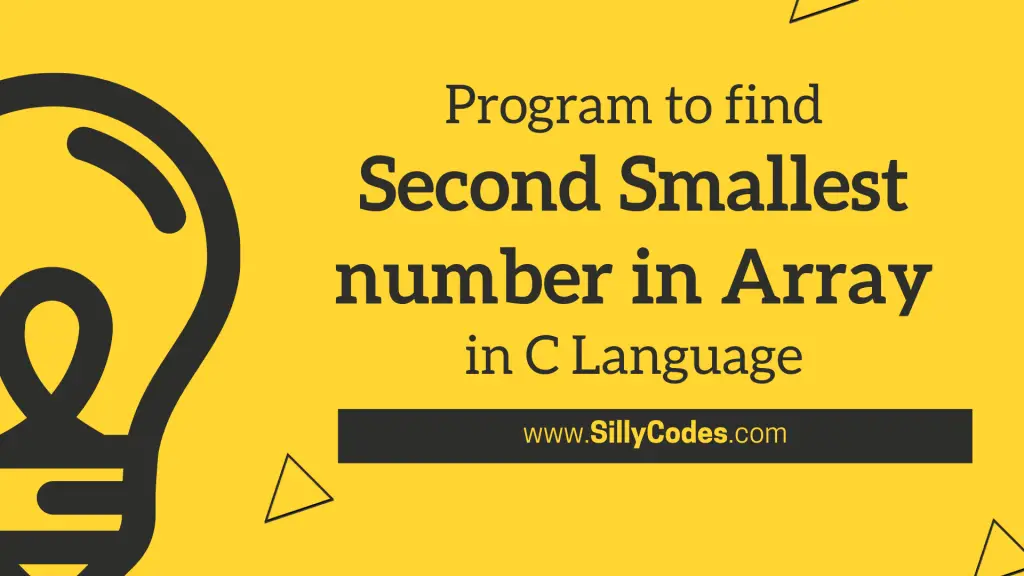
Program Description:
Write a program to find the Second Smallest number in Array in C programming language. The program should accept the size of the array and array elements from the user and find the smallest number in the array.
Example Input and Output:
Input:
1 2 3 4 5 6 7 8 9 10 |
Enter desired array size(1-100): 8 Please enter array elements numbers[0] : 23 numbers[1] : 0 numbers[2] : -1 numbers[3] : 34 numbers[4] : 85 numbers[5] : 34 numbers[6] : 21 numbers[7] : 47 |
Output:
1 2 |
smallest element in array is : -1 Second smallest element in array is : 0 |
Prerequisites:
It is recommended to have knowledge of the Arrays in C and functions in C language. So please go through the following articles to learn more about them
- Arrays in C with example programs
- Functions in C – how to declare, define, and call functions.
- Passing Arrays to functions in C
Second Smallest number in Array in C Program Explanation:
- First of all, Declare a numbers array, The numbers can hold 100 elements(Max). Take the size of the array from the user and update it in size variable.
- Prompt the user to provide the array elements. Update the array elements with provided user input
- Then, create two variables, smallest and second_smallest, and initialize them with the INT_MAX. ( Learn more about the INT_MAX at Size and Limits of datatypes in C)
- Iterate through all array elements
- Update the second_smallest and smallest variables if you find a number that is smaller than the present smallest (i.e ( numbers[i] < smallest)). First, update the second_smallest with present smallest, Then update the smallestwith number[i].
- If you find any number which is larger than smallest number and less than the second_smallest ( i.e (numbers[i] < second_smallest && numbers[i] > smallest)) Then update the second_smallest number with number[i].
- Once the above loop is completed, The smallest and second_smallest variables hold the Smallest and second smallest numbers correspondingly.
- Finally, display the smallestand second_smallest numbers on the console.
The Second Smallest number in Array in C Algorithm:
- Declare numbers array with 100 size.
- Take the desired array size from the user and update the size variable.
- Update the array with user input.
- Iterate from 0 to size-1 and update &numbers[i] using scanf() function.
- Create smallest and second_smallest. Initialize them with INT_MAX.
- Iterate over array elements and update the
smallest and
second_smallest elements.
- Iterate from zero(0) to ( size-1).
- If numbers[i] < smallest, Then update second_smallest = smallest; and smallest = numbers[i];
- else if(numbers[i] < second_smallest && numbers[i] > smallest), Then update the second_smallest = numbers[i];
- Print the smallestand second_smallestnumbers of the array using printf function.
Program to find Second Smallest number in Array in C:
📢 Note: As we are using the INT_MAX, make sure to include the limits.h header file
Here is the C program to find the smallest and second smallest numbers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 |
/*     Program to find Second smallest element of Array     sillycodes.com */ #include <stdio.h> #include <limits.h>  int main() {     // declare the 'numbers' array     int numbers[100];     int i, size;      printf("Enter desired array size(1-100): ");     scanf("%d", &size);      // User input for array elements     printf("Please enter array elements\n");     for(i = 0; i < size; i++)     {         printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     }         // initialize the 'smallest', 'second_smallest' variable with 'INT_MAX' from limits.h file     // Look at - https://sillycodes.com/size-and-limits-datatypes-in-c-programming/ for info.     int smallest = INT_MAX;     int second_smallest = INT_MAX;         // find the smallest and second_smallest of array elements     for(i = 0; i < size; i++)     {         // check for 'smallest'         if( numbers[i] < smallest)         {             // numbers[i] is smallest. so update 'second_smallest' with present 'smallest'             second_smallest = smallest;             smallest = numbers[i];         }         else if(numbers[i] < second_smallest && numbers[i] > smallest)         {             // update the second_smallest             second_smallest = numbers[i];         }     }      // print the result     printf("smallest element in array is : %d\n", smallest);     printf("Second smallest element in array is : %d\n", second_smallest);      return 0; } |
Program Output:
Let’s compile and Run the Program.
Compile the Program
gcc second_smallest.c
Run the program.
Test 1:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
$ ./a.out Enter desired array size(1-100): 8 Please enter array elements numbers[0] : 23 numbers[1] : 0 numbers[2] : -1 numbers[3] : 34 numbers[4] : 85 numbers[5] : 34 numbers[6] : 21 numbers[7] : 47 smallest element in array is : -1 Second smallest element in array is : 0 |
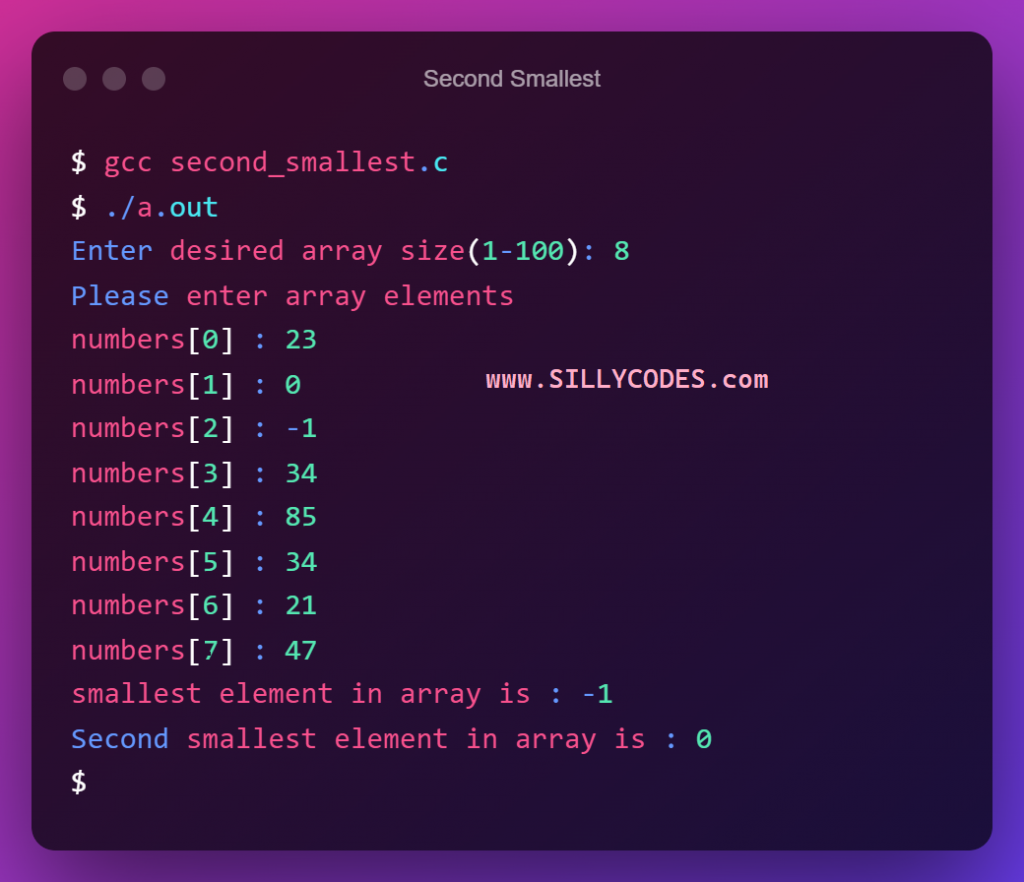
Test 2:
1 2 3 4 5 6 7 8 9 10 11 |
$ ./a.out Enter desired array size(1-100): 5 Please enter array elements numbers[0] : 23 numbers[1] : 84 numbers[2] : 38 numbers[3] : 87 numbers[4] : 23 smallest element in array is : 23 Second smallest element in array is : 38 $ |
Second Smallest Number in Array using Functions in C Langauge:
Let’s rewrite the above program and divide the main() function into the sub-functions. Create a couple of functions. One function is to read the input from the user and another function is to calculate the second smallest number.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 |
/*     Program to find Second smallest element of Array     sillycodes.com */ #include <stdio.h> #include <limits.h>  /** * @brief  - Read the elements from the user and update 'numbers' array * * @param numbers  - 'numbers[]' array * @param size    - size of the 'numbers' array */ void read(int numbers[], int size) {     int i;     printf("Please enter array elements\n");     // Read the input from the user     for(i = 0; i < size; i++)     {         printf("numbers[%d] : ", i);         scanf("%d", &numbers[i]);     } }  /** * @brief Get the second smallest element from the 'numbers' array * * @param numbers - Array * @param size    - size of the 'numbers' array * @return int    - second smallest element */ int get_second_smallest(int numbers[], int size) {     int i;     // initialize the 'smallest', 'second_smallest' variable with 'INT_MAX' from limits.h file     // Look at - https://sillycodes.com/size-and-limits-datatypes-in-c-programming/ for info.     int smallest = INT_MAX;     int second_smallest = INT_MAX;         // find the smallest and second_smallest of array elements     for(i = 0; i < size; i++)     {         // check for 'smallest'         if( numbers[i] < smallest)         {             // numbers[i] is smallest. so update 'second_smallest' with present 'smallest'             second_smallest = smallest;             smallest = numbers[i];         }         else if(numbers[i] < second_smallest && numbers[i] > smallest)         {             // update the second_smallest             second_smallest = numbers[i];         }     }      // return the 'second_smallest' element     return second_smallest; }  int main() {     // declare the 'numbers' array     int numbers[100];     int i, size;      printf("Enter desired array size(1-100): ");     scanf("%d", &size);      // Take the user input     read(numbers, size);      // Call the 'get_second_smallest' function     int second_smallest = get_second_smallest(numbers, size);      // print the result     printf("Second smallest element in array is : %d\n", second_smallest);      return 0; } |
We have defined two functions in the above program.
- The
read() function.
- Prototype of the read() function – void read(int numbers[], int size);
- The read() function takes two arguments – numbers array and its size. The read() function prompts the user for array elements and updates the number array.
- The
get_second_smallest() function.
- Prototype of the get_second_smallest() function – int get_second_smallest(int numbers[], int size);
- The get_second_smallest() function also takes two formal arguments one is the input array numbers[] and the sizeof the number’s array.
- The get_second_smallest() function finds the second smallest element in the array by comparing all elements.
- Finally, It returns the second smallest element in the array back to the caller.
📢 Here we have returned only a single value from the get_second_smallest() function. If you want to return multiple values like smallest and second_smallest, Then create a static array and return it back to the caller.
Program Output:
Let’s compile and run the program.
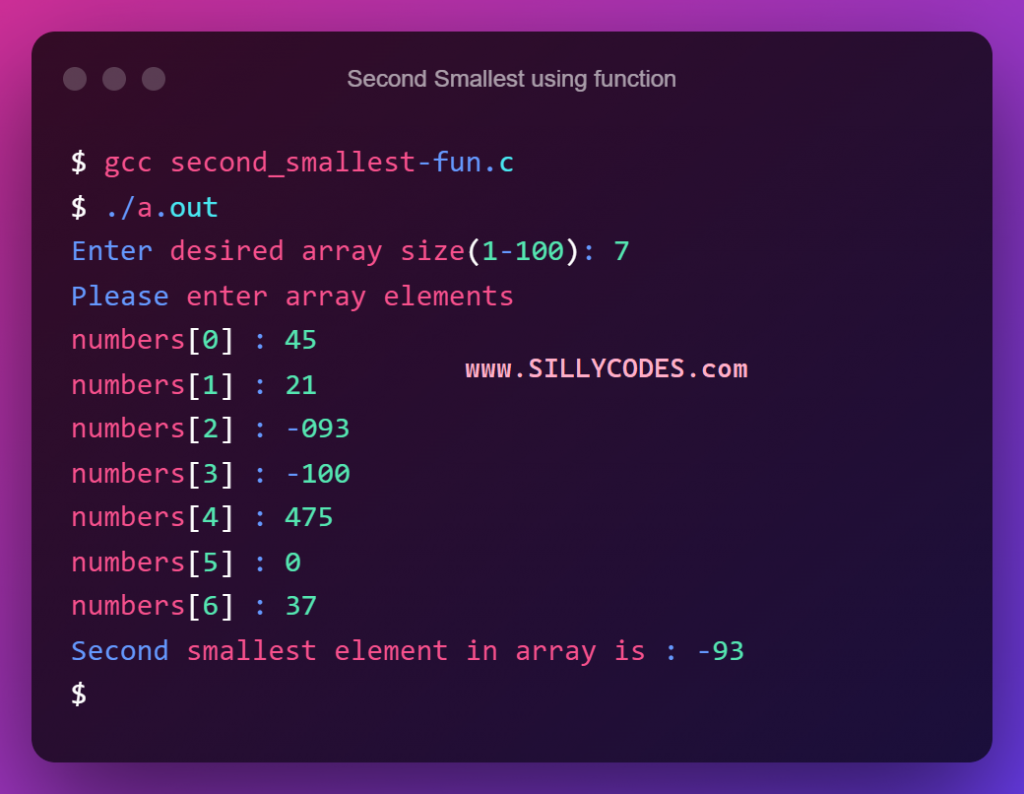
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
$ gcc second_smallest-fun.c $ ./a.out Enter desired array size(1-100): 7 Please enter array elements numbers[0] : 45 numbers[1] : 21 numbers[2] : -093 numbers[3] : -100 numbers[4] : 475 numbers[5] : 0 numbers[6] : 37 Second smallest element in array is : -93 $ ./a.out Enter desired array size(1-100): 5 Please enter array elements numbers[0] : 43 numbers[1] : 98 numbers[2] : 0 numbers[3] : 2 numbers[4] : 34 Second smallest element in array is : 2 $ |
Related Array Programs:
- Index – C Language Practice Programs
- C Tutorials Index – Step by step tutorials to master C Langauge
- C Program to Find Second Largest Element in Array
- C Program to Read and Print Arrays
- Reverse Print Array Program in C
- C Program to calculate Sum of all array Elements
- C Program to Calculate the Average of all array Elements
- C Program to find minimum element in Array
- C Program to Find Maximum Element in Array
1 Response
[…] C Program to Find Second Smallest Element in Array […]