Program to Find Unique Elements in Array in C Language
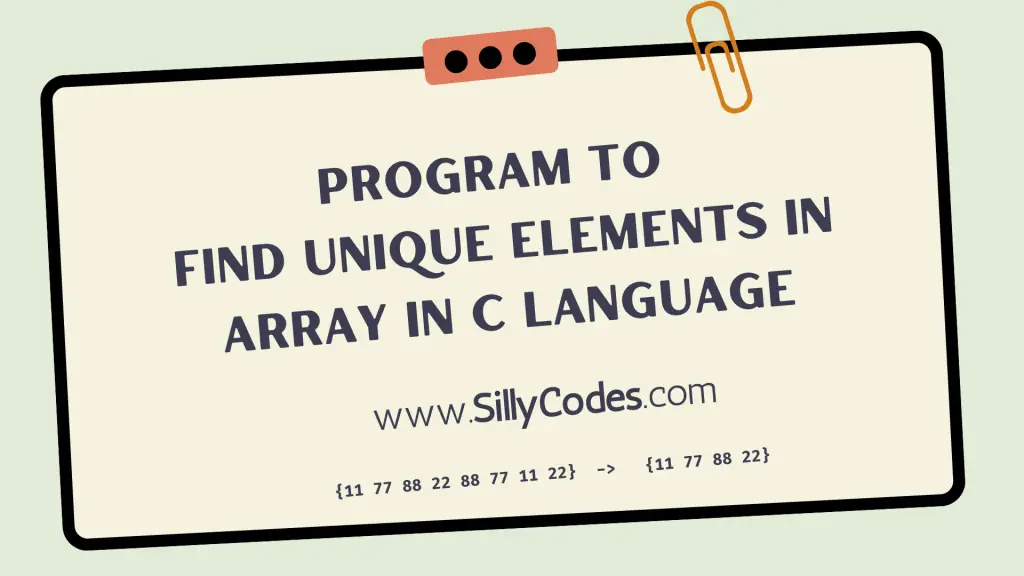
Program Description:
Write a C Program to Find Unique Elements in Array in C programming language. The program accepts a input array from the user and finds the unique elements or distinct elements in the given array.
📢 Related Programs:
Example Input and Output of the Program:
Input:
Enter desired array size(1-1000): 8
Please enter array elements(8) : 11 77 88 22 88 77 11 22
Output:
Original Array: 11 77 88 22 88 77 11 22
Array (Unique Elements): 11 77 88 22
Note that the output only contains the distinct element or Unique elements in the given array.
Prerequisites:
To better understand the following program, Please go through the C Arrays, Functions in C, and How to Pass Arrays to Functions Articles.

Algorithm to Find the Unique Elements in Array in C language:
To find the unique elements in the array, We are going to create a new array which is going to store only unique elements from the original array.
So we need to go through the original array element by element and Insert the element into the new array only if the element is not present in the new array.
Once the above loop is completed, The new array will contain only the unique elements.
At the end, If we want to replace the original array, We can replace the original array elements with the new array elements.
📢 We are using extra space O(n) in this example.
Now let’s look at the step by step explanation of the program.
Unique Elements in Array in C Program Explanation:
- Declare the numbers array, with the size of 1000. Also, create an array named unique with 1000 elements. The unique array is going to store the unique elements of the numbers array.
- Take the desired array size from the user and update the size variable.
- Check for the array bounds using the if(size >= 1000 || size <= 0), Display an error message if the size goes beyond the bounds.
- Request input from the user for the array’s elements, and update the array.
- To get the unique elements of the array, Traverse through the original array (i.e numbers) and Update the unique array only if the present element not found in the unique array.
- Start a For loop from i=0 to i<size to iterate over the original array.
- use isExists element to check if the element is found in the unique array. Set isExists=0 at the start of each iteration.
- Create another for loop from j=0 to j<uniqueSize
- check if the present number number[i] is present in the unique array.
- if the number[i] == unique[j] condition is true, then update the isExists=1. and break out of the loop.
- Check the isExists is still zero(0), If so, Then the number[i] is not found in the unique array, So update the unique array with the number[i] – unique[uniqueSize] = numbers[i];
- Increment the uniqueSize – uniqueSize++
- Once the above two loops are completed, The unique array will contain the Unique elements in Array.
- Display the results. First of display the input array by using a For loop, Then display the unique array using another for loop.
📢We can also replace the elements of the input array( numbers) with the new array( unique) elements.
Program to Get Unique Elements in Array in C:
Let’s convert the above algorithm into the C program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 |
/* Program to find Unique elements in Array Author: SillyCodes.com */ #include <stdio.h> int main() { // declare the 'numbers' array and Initialize 'unique' array int numbers[1000], unique[1000] = {0}; int i, j, size, uniqueSize = 0, isExists = 0; printf("Enter desired array size(1-1000): "); scanf("%d", &size); // check for array bounds if(size >= 1000 || size <= 0) { printf("Invalid Size, Try again \n"); return 0; } // User input for array elements printf("Please enter array elements(%d) : ", size); for(i = 0; i < size; i++) { // printf("numbers[%d] : ", i); scanf("%d", &numbers[i]); } // Iterate over the original array 'numbers' // and update the 'unique' array only if the element is not found in the 'unique' array for(i=0; i<size; i++) { // update the isExists with '0' at the start of each iteration isExists = 0; for(j=0; j<uniqueSize; j++) { if(numbers[i] == unique[j]) { // 'numbers[i] is found in 'unique' array // so mark the 'isExists' as 1 isExists = 1; break; } } // update the 'unique' array if 'isExists' is equal to zero if(isExists == 0) { // 'number[i]' is not found in the 'unique' array // 'uniqueSize' starts from '0' unique[uniqueSize] = numbers[i]; uniqueSize++; } } // Print the original array printf("Original Array: "); for(i = 0; i < size; i++) { printf("%d ", numbers[i]); } printf("\n"); // If you want to replace, you can replace the original array like below // Replace the original Array with new array. // for(i=0; i<uniqueSize; i++) // { // numbers[i] = unique[i]; // } // size = uniqueSize; // Print the 'unique' array printf("Array (Unique Elements): "); for(i = 0; i < uniqueSize; i++) { printf("%d ", unique[i]); } printf("\n"); return 0; } |
Program Output:
Compile the program using the your favorite compiler. We are using the GCC compiler in this example.
gcc unique-elements.c
The above command, Generates an executable file named a.out. Run the executable file using the ./a.out command.
Test Case 1: Let’s the positive test cases
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$ gcc unique-elements-arr.c $ ./a.out Enter desired array size(1-1000): 10 Please enter array elements(10) : 1 2 3 4 5 3 5 2 1 8 Original Array: 1 2 3 4 5 3 5 2 1 8 Array (Unique Elements): 1 2 3 4 5 8 $ ./a.out Enter desired array size(1-1000): 6 Please enter array elements(6) : 1 1 1 1 1 1 Original Array: 1 1 1 1 1 1 Array (Unique Elements): 1 $ ./a.out Enter desired array size(1-1000): 8 Please enter array elements(8) : 11 77 88 22 88 77 11 22 Original Array: 11 77 88 22 88 77 11 22 Array (Unique Elements): 11 77 88 22 $ |
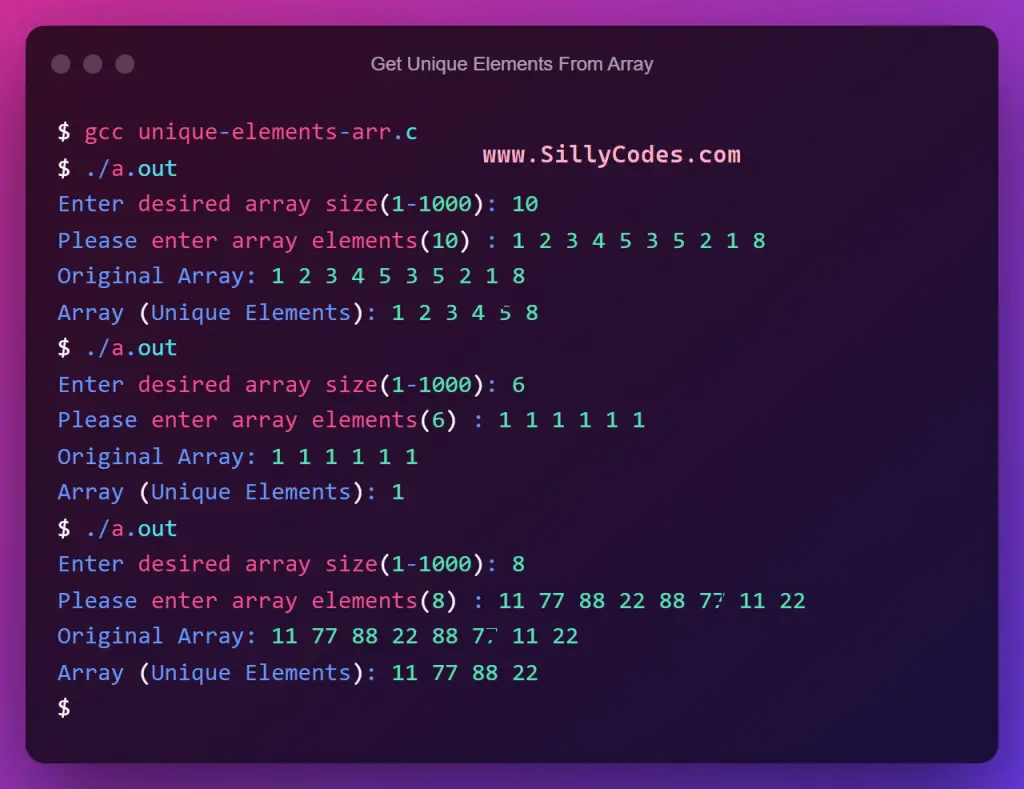
As we can see from the above output, The program is generating the excepted results.
For example, If the input array is Please enter array elements(6) : 1 1 1 1 1 1, Then the unique elements in the array are Array (Unique Elements): 1.
Test Case 2: Negative test cases
1 2 3 4 5 6 7 8 |
// Negative Test Case $ ./a.out Enter desired array size(1-1000): 3000 Invalid Size, Try again $ ./a.out Enter desired array size(1-1000): 0 Invalid Size, Try again $ |
The max array size is 1000, So if user enters size beyond the bounds of the array, Then display an error message ( Invalid Size, Try again).
⚠️ Please change the
1000 value in the array declaration, If you want to use the larger arrays.
Additionally, We can also use a Macro or Const variable to hold the value of the
1000. For the brevity, we have directly used the
1000.
Excersice:
Rewrite the above program to use the user defined functions for
- Reading the user input and updating the array
- Displaying the Array elements
- getUnique function to get the unique elements from the array.
Related Array Programs:
- Index – C Language Practice Programs
- C Tutorials Index – Step by step tutorials to master C Langauge
- C Program to Read and Print Arrays
- Reverse Print Array Program in C
- C Program to calculate Sum of all array Elements
- C Program to Calculate the Average of all array Elements
- C Program to find minimum element in Array
- C Program to calculate the total number of Positive numbers, Negative numbers, and Zeros in an Array
- C Program to Count Even and Odd numbers in Array
- C Program to Find Maximum Element in Array
- C Program to Reverse the Array Elements
- C Program to Reverse Array Elements using Recursion
- C Program to Sort Array Elements in Ascending order
- C Program to Sort Array in Ascending and Descending Order using QSort function
2 Responses
[…] C Program to Find Unique Elements in an Array […]
[…] C Program to Find Unique Elements in an Array […]