Program to Generate First n Fibonacci Numbers in C
- Program Description:
- What is the Fibonacci Number?
- Pre-Requisites:
- First n Fibonacci numbers in C Program Algorithm:
- Program to Print first n Fibonacci numbers in C Language using while loop:
- First n Fibonacci numbers program in C using for loop:
- Generate First n Fibonacci numbers Use the Goto statement on Invalid Input:
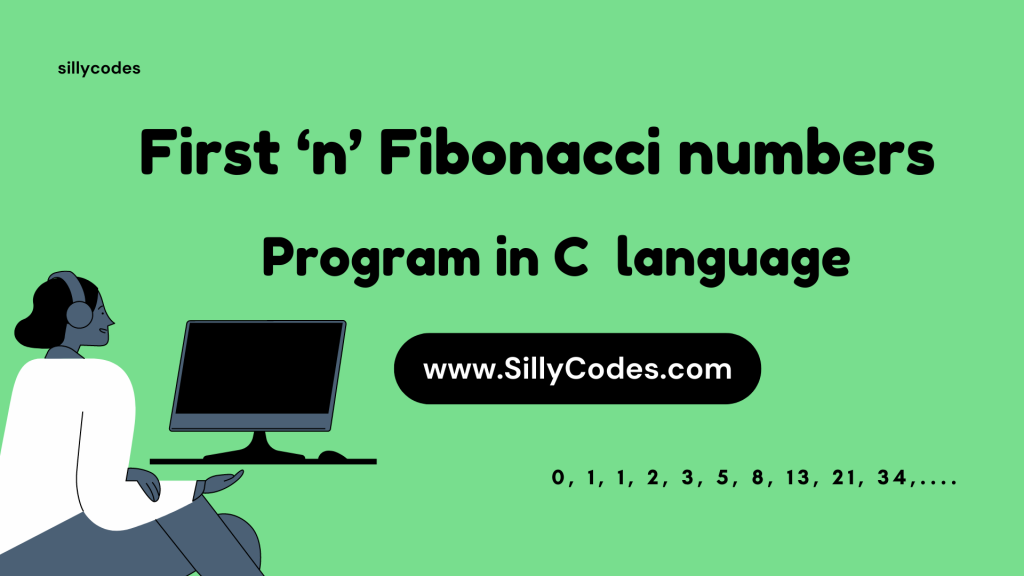
Program Description:
Write a Program to Generate First n Fibonacci Numbers in C language. The program should accept a positive number ( n) from the user and generate first n Fibonacci Numbers. For example, If the user enters 3, The program should generate the first 3 Fibonacci numbers, which are 0, 1, 1. The program should display an error message on Invalid Input.
Here is the program expected Input and Output:
Example Input and Output:
Input:
How many fibonacci numbers do you want to print : 5
Output:
First 5 Fibonacci numbers are : 0 1 1 2 3
Also Read:
What is the Fibonacci Number?
The Fibonacci series is a series of whole numbers in which each number is the sum of the two preceding numbers. Beginning with and 1, the sequence of Fibonacci numbers would be 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, etc.
The formula to calculate Fibonacci Series is n = (n-1) + (n-2).
where (n-1) means “the last number before n in the series” and (n-2) refers to “the second last one before n in the series.”
Pre-Requisites:
It is good to have an understanding of C Loops. Please go through the following articles to learn more about the C Loops
- While Loop in C Language
- For Loop in C Language with Example Programs
- do while loop in Language with example programs
First n Fibonacci numbers in C Program Algorithm:
- Take the input number from the user and store it as a variable num
- Check if the num is positive or negative, If the num is Negative, Display the error message – Invalid Input, Please provide valid number
- If the num is a positive number, Proceed to the next step
- Initialize the first two Fibonacci numbers i = 0 and j = 1
- Check if the num is equal to 1, If so, print the first Fibonacci number and return. Otherwise, print the first two base Fibonacci numbers
- Then calculate the next Fibonacci number k using the i and j ( i.e k = i + j )
- We use cnt variable as the counter. As we already printed the first two Fibonacci numbers, The Counter cnt starts from 2. So update cnt = 2.
- Start the loop, The will continue until the num > cnt
- At Each Iteration
- Print the present Fibonacci number k
- Then move forward by assigning the j value i ( i = j), k value to j ( j = k)
- Finally, create the next number in the Fibonacci series by adding i and j ( k = i + j)
- Also, Increment the cnt by 1.
- This loop will continue until the Loop condition num > cnt becomes False
- Once we reach the n Fibonacci numbers, The loop will terminate and control comes out of the loop.
Program to Print first n Fibonacci numbers in C Language using while loop:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
/* C Program to Generate first n Fibonacci Numbers This program accepts one number from user let's say it as 'n' and prints first 'n' Fibonacci Numbers on Console. */ #include<stdio.h> int main() { // Take the Input from the user int i,j,k,num,cnt; printf("How many fibonacci numbers do you want to print : "); scanf("%d",&num); // check 'num', display error on Invalid input. if(num <= 0) { printf("Invalid Input, Please provide valid number \n"); return 0; } /* * because fibonacci series starts with 0,1 * so we initialize 'i' and 'j' as 0 and 1 */ i=0; j=1; printf("First %d Fibonacci numbers are : ", num); // If 'num' is 1, Print first Fibonacci number. i.e 0. if(num == 1) { printf(" %d\n",i); return 0; } // print first two fibonacci numbers. printf(" %d %d ",i,j); // increment the counter as we printed i, j cnt = 2; // 'k' is equal to 'i' + 'j' // as in Fibonacci n is equal to (n-1) + (n-2) k = i+j; // Loop until cnt reaches 'num' while(num > cnt) { // print fibonacci number. update i, j, k printf("%d ",k); i=j; j=k; k=i+j; cnt++; // increment count } printf("\n"); return 0; } |
Program Output:
Compile and Run the program using your IDE. We are using the GCC Compiler on Linux OS.
Here is the output of the program
Test Case 1: When the user provided a positive number:
1 2 3 4 5 6 7 8 |
$ gcc first-n-fibonacci-numbers.c $ ./a.out How many fibonacci numbers do you want to print : 5 First 5 Fibonacci numbers are : 0 1 1 2 3 $ ./a.out How many fibonacci numbers do you want to print : 10 First 10 Fibonacci numbers are : 0 1 1 2 3 5 8 13 21 34 $ |
We are getting the expected output. When user entered num as 5, The program displayed the First 5 Fibonacci numbers which are 0 1 1 2 3
Similarly, Program gave the first 10 Fibonacci numbers when the user provided num as 10 – 0 1 1 2 3 5 8 13 21 34
Test 2: When the user provided a Negative Number:
1 2 3 4 |
$ ./a.out How many fibonacci numbers do you want to print : -24 Invalid Input, Please provide valid number $ |
As you can see from the above output, When the user provided a Negative number, The program is displaying the error message Invalid Input, Please provide valid number
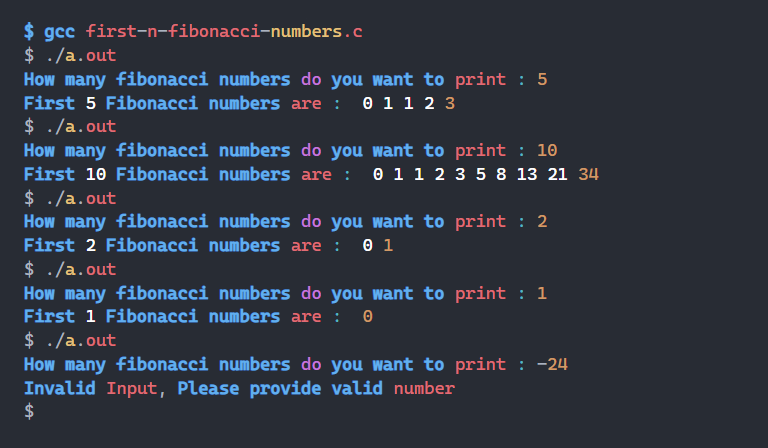
📢 Note: This program generates Fibonacci numbers up to any number but You need to use sufficient Datatype for our i, j, and k variables. In the above program, we used an Integer datatype for i, j, and k variables so that it will generate up to 2+ Giga numbers. After that, it will give Negative numbers. Therefore, depending on your needs, use long int or long long int. To learn about the fundamental C datatypes and their sizes, please refer to the below article
First n Fibonacci numbers program in C using for loop:
Let’s look write the above program using the for loop as well.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 |
/* C Program to Generate first n Fibonacci Numbers This program accepts one number from user let's say it as 'n' and prints first 'n' Fibonacci Numbers on Console. */ #include<stdio.h> int main() { // Take the Input from the user int i,j,k,num,cnt; printf("How many fibonacci numbers do you want to print : "); scanf("%d",&num); // check 'num', display error on Invalid input. if(num <= 0) { printf("Invalid Input, Please provide valid number \n"); return 0; } /* * because fibonacci series starts with 0,1 * so we initialize 'i' and 'j' as 0 and 1 */ i=0; j=1; printf("First %d Fibonacci numbers are : ", num); // If 'num' is 1, Print first Fibonacci number. i.e 0. if(num == 1) { printf(" %d\n",i); return 0; } // print first two fibonacci numbers. printf(" %d %d ",i,j); // increment the counter as we printed i, j cnt = 2; // 'k' is equal to 'i' + 'j' // as in Fibonacci n is equal to (n-1) + (n-2) // Loop until cnt reaches 'num' for(k = i+j; num > cnt; k=i+j) { // print fibonacci number. update i, j, k printf("%d ",k); i=j; j=k; cnt++; // increment count } // we can also change the for like below. // for(k = i+j; num > cnt; i=j, j=k, k=i+j) { // printf("%d ",k); // cnt++; // } printf("\n"); return 0; } |
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc first-n-fib.c $ ./a.out How many fibonacci numbers do you want to print : 10 First 10 Fibonacci numbers are : 0 1 1 2 3 5 8 13 21 34 $ ./a.out How many fibonacci numbers do you want to print : 3 First 3 Fibonacci numbers are : 0 1 1 $ ./a.out How many fibonacci numbers do you want to print : 9 First 9 Fibonacci numbers are : 0 1 1 2 3 5 8 13 21 $ ./a.out How many fibonacci numbers do you want to print : -23 Invalid Input, Please provide valid number $ |
We are getting the expected output.
Generate First n Fibonacci numbers Use the Goto statement on Invalid Input:
So far we are displaying the error message when the user enters Invalid input ( Invalid Input, Please provide valid number ).
Now, If the user enters invalid Input, Let’s show the error message and also allow the user to provide the new input. To do that, We use the Goto statement in C. goto statement helps us to take the program control back to the start of the program
📢 The Goto statement is used to jump from one statement to another within a function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
/* C Program to Generate first n Fibonacci Numbers Use gotostatement on Invalid Input */ #include<stdio.h> int main() { // Take the Input from the user int i,j,k,num,cnt; INPUT: printf("How many fibonacci numbers do you want to print : "); scanf("%d",&num); // check 'num', display error on Invalid input. if(num <= 0) { printf("Invalid Input, Please provide valid number \n"); goto INPUT; } /* * because fibonacci series starts with 0,1 * so we initialize 'i' and 'j' as 0 and 1 */ i=0; j=1; printf("First %d Fibonacci numbers are : ", num); // If 'num' is 1, Print first Fibonacci number. i.e 0. if(num == 1) { printf(" %d\n",i); return 0; } // print first two fibonacci numbers. printf(" %d %d ",i,j); // increment the counter as we printed i, j cnt = 2; // 'k' is equal to 'i' + 'j' // as in Fibonacci n is equal to (n-1) + (n-2) // Loop until 'cnt' reaches 'num' for(k = i+j; num > cnt; k=i+j) { // print fibonacci number. update i, j, k printf("%d ",k); i=j; j=k; cnt++; // increment count } printf("\n"); return 0; } |
If you notice, We have added a INPUT: (at line 10) and goto INPUT; (at line 17). The INPUT: is the label. So whenever the user enters Invalid inputs like Negative numbers, Then the goto INPUT; Statement will be executed, which takes the program control back to the label INPUT:. So the printf statement will be printed with the message and we can take the input from the user again using the scanf.
Program Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc first-n-fib.c $ ./a.out How many fibonacci numbers do you want to print : 6 First 6 Fibonacci numbers are : 0 1 1 2 3 5 $ ./a.out How many fibonacci numbers do you want to print : -8 Invalid Input, Please provide valid number How many fibonacci numbers do you want to print : -45 Invalid Input, Please provide valid number How many fibonacci numbers do you want to print : -12 Invalid Input, Please provide valid number How many fibonacci numbers do you want to print : 5 First 5 Fibonacci numbers are : 0 1 1 2 3 $ |
As you can see from the output above, the program displayed an error notice and requested for the user’s input once more when the user entered a negative number. This continued until the user entered a valid input.
3 Responses
[…] C Program to Print First n Fibonacci numbers […]
[…] C Program to Print First n Fibonacci numbers […]
[…] C Program to Print First n Fibonacci numbers […]