Program to perform Arithmetic Operations using Pointers in C
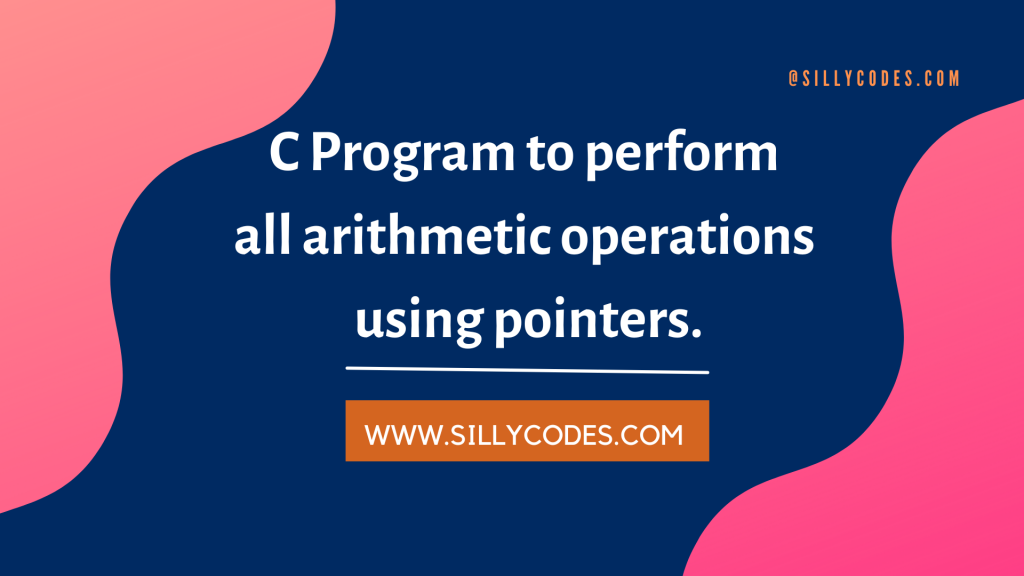
Program Description:
Write a Program to perform all arithmetic operations using pointers in c programming language. The program will accept two integer variables from the user and calculates the addition, subtraction, multiplication, division, and modulo division using the pointers.
📢This program is part of the Pointers practice programs series.
Let’s look at the example input and output of the program.
Example Input and Output:
Input:
Enter value for first number(num1): 200
Enter value for second number(num2): 100
Output:
Sum of 200+100 = 300
Subtraction of 200-100 = 100
Product of 200*100 = 20000
Division of 200/100 = 2
Modulo Division of 200%100 = 0
The program took two integers from the user, performed all arithmetic operations, and provided the result.
Program Prerequisites:
To better understand the following program, Please go through the below Pointers and Function tutorials.
- Pointers in C Programming Language – Declare, Initialize, and use pointers
- Functions in C Language with Example Programs
- Operators in C Language
Arithmetic Operations using Pointers in C Program Explanation:
We are going to define five functions to perform arithmetic operations. They are sum(), subtract(), product(), division(), and moduloDivision() functions.
The prototype of all functions is the same, They accept three integer pointer variables as formal arguments and perform the respective operation.
For example, The subtract() function takes three integer pointers num1, num2, and result. and subtracts the num2 from the num1 variable and stores the results in the result variable.
Here is the prototype of the subtract() function.
void subtract(int *num1, int *num2, int * result)
The subtract() function dereferences the num1 and num2 pointer variables to get the values of num1 and num2.
*result = *num1 - *num2;
Similarly, All other functions also dereference and perform the respective operations on the num1 and num2
-
sum() function
- *result = *num1 + *num2;
-
division() function
- *result = *num1 / *num2;
-
product() function
- *result = *num1 * *num2;
-
moduloDivision() function
- *result = *num1 % *num2;
Finally, Call the sum(), subtract(), product(), moduloDivision(), and division() functions from the main() function and pass the num1 and num2 and result variables with addresses using the address of operator(‘&’)
Ex:
sum(&num1, &num2, &result);
Print the results on the console.
📢As we are not changing the
num1 and
num2 variables, We can simply pass them as normal variables as well (without addresses)
So change the above program like below.
Ex:
product(num1, num2, &result);
void product(int num1, int num2, int * result);
Program to Calculate all Arithmetic Operations using Pointers in C Language:
Here is the program to calculate all arithmetic operations using pointers in C programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 |
/*     Program to perform all arithmetic operations using pointers     sillycodes.com */  #include <stdio.h>  /** * @brief  - Add 'num1' and 'num2' and update 'result' */ void sum(int *num1, int *num2, int * result) {     // dereference the numbers to get values     *result = *num1 + *num2; }  /** * @brief  - Subtract 'num1' and 'num2' and update 'result' */ void subtract(int *num1, int *num2, int * result) {     // dereference the numbers to get values     *result = *num1 - *num2; }  /** * @brief  - Calculate division of 'num1' and 'num2' and update 'result' */ void division(int *num1, int *num2, int * result) {     // dereference the numbers to get values     *result = *num1 / *num2; }  /** * @brief  - Calculate product of 'num1' and 'num2' and update 'result' */ void product(int *num1, int *num2, int * result) {     // dereference the numbers to get values     *result = *num1 * *num2; }  /** * @brief  - Calculate Modulo division of 'num1' and 'num2' and update 'result' */ void moduloDivision(int *num1, int *num2, int * result) {     // dereference the numbers to get values     *result = *num1 % *num2; }  int main() {     // declare the variables     int num1, num2, result;      // Take two numbers from user     printf("Enter value for first number(num1): ");     scanf("%d", &num1);     printf("Enter value for second number(num2): ");     scanf("%d", &num2);      // call the sum function     // pass addresses of num1, num2, result     sum(&num1, &num2, &result);     // print the sum     printf("Sum of %d+%d = %d\n", num1, num2, result);      // call 'subtract()' function     subtract(&num1, &num2, &result);     // print the subtraction result     printf("Subtraction of %d-%d = %d\n", num1, num2, result);      // call 'product()' function     product(&num1, &num2, &result);     // print the product     printf("Product of %d*%d = %d\n", num1, num2, result);      // call 'division' function     division(&num1, &num2, &result);     // print the division result     printf("Division of %d/%d = %d\n", num1, num2, result);      // call 'moduloDivision()' function     moduloDivision(&num1, &num2, &result);     // print the sum     printf("Modulo Division of %d%%%d = %d\n", num1, num2, result);       return 0; } |
We have provided detailed comments for your convenience.
Arithmetic operations using pointers in C – Program Output:
Let’s compile and Run the program using your favorite Compiler.
$ gcc arith-pointer.c
Run the Program.
Test Case 1:
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter value for first number(num1): 200 Enter value for second number(num2): 100 Sum of 200+100 = 300 Subtraction of 200-100 = 100 Product of 200*100 = 20000 Division of 200/100 = 2 Modulo Division of 200%100 = 0 $ |
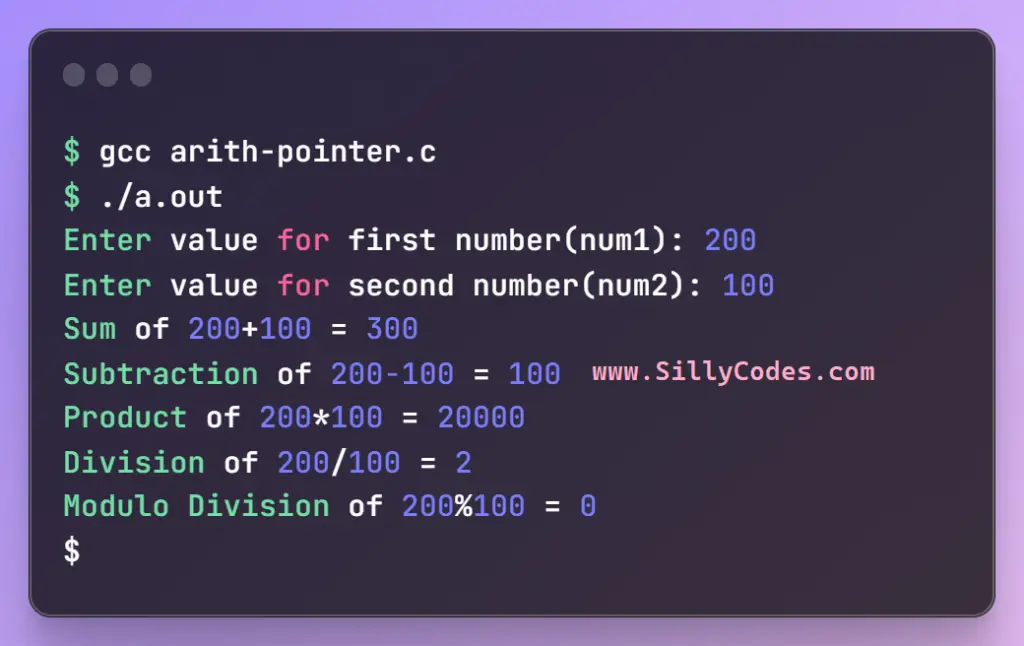
As we can see from the above output, The user provided the num1as 200and num2 as 100. And the program performed all arithmetic operations and displayed the output on the console.
Let’s look at another example.
Test Case 2:
1 2 3 4 5 6 7 8 9 |
$ ./a.out Enter value for first number(num1): -50 Enter value for second number(num2): 20 Sum of -50+20 = -30 Subtraction of -50-20 = -70 Product of -50*20 = -1000 Division of -50/20 = -2 Modulo Division of -50%20 = -10 $ |
The program is generating the desired results.
Related C Practice Programs:
- C Tutorials Index
- C Programs Index – 300+ Programs
- C Program to Perform arithmetic Operations using Switch Statement
- C Program to Check the Sign of the Number ( Positive, Negative, or Zero) Using Switch Case
- C Program to find whether the alphabet is a Vowel or Consonant using Switch Case
- Enter the week number and print the day of week program Using Switch Statement
- C Program to calculate the Number of days in Month using the switch statement
- C Program to check Even or Odd Number using Switch Case
- C Program to find the maximum of two numbers using a switch case
- Menu Driven Calculator Program using Switch Case and Do while
2 Responses
[…] Program to perform Arithmetic Operations using Pointers in C […]
[…] the following program, We have defined a function called performArithOps. The function performs all arithmetic operations like addition, subtraction, product, division, and modulo division on given two integers. Here is […]