Allocate memory dynamically using malloc function in C language
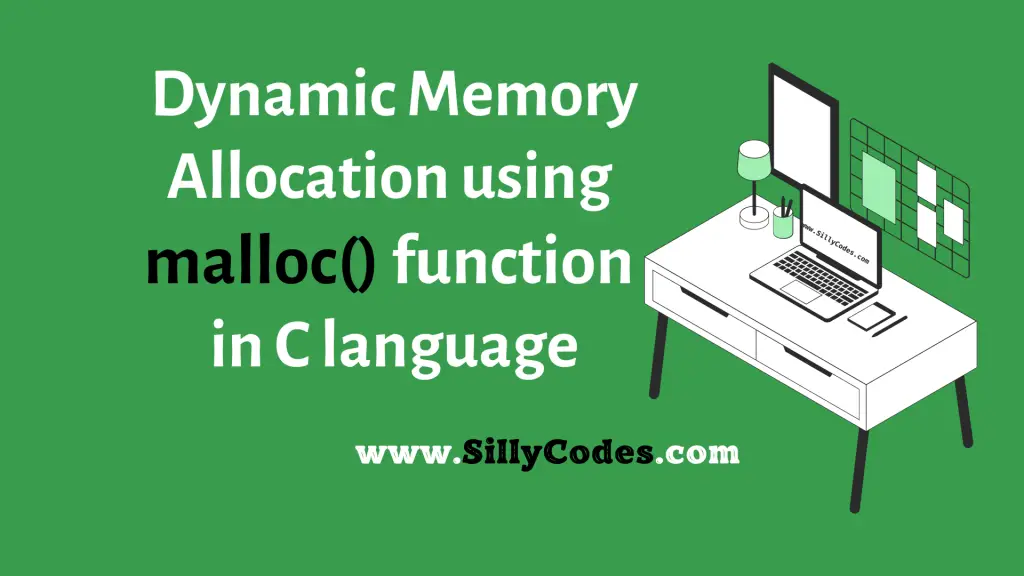
Introduction:
We have looked at the pointers in c programming in earlier articles. In today’s tutorial, we will look at the dynamic memory allocation using malloc function in c language with example programs.
Prerequisites:
It is recommended to know the pointers in c language. So please go through the following pointers tutorials to better understand this tutorial.
The malloc() function in C – Allocate Memory Dynamically using Malloc() function:
The malloc() function is used to dynamically allocate memory. The malloc() function takes size as an argument and allocates the specified number of bytes in the heap.
Here is the prototype of the malloc function in c programming.
1 |
void *malloc(size_t size); |
The size_t is an unsigned integer type. So The malloc() function allocates the size number of bytes and returns a pointer to the allocated block of memory. Do note that, the returned pointer is a void pointer, so we need to typecast it to the appropriate data type before using it.
If for any reason malloc() is not able to allocate the given number of bytes, Then it will return NULL.
The malloc() function is declared in the stdlib.h header file. so don’t forget to include the stdlib.h header file in your program.
📢malloc – memory allocation – Allocates a contiguous block of memory in the heap section.
Let’s look at the syntax and an example of the malloc() function in c programming.
Syntax of malloc() function:
1 |
pointer_name = (data_type *) malloc (size); |
Here
- pointer_name is the pointer name – This pointer_name will be pointing to the first block of allocated memory.
- (data_type *) is the typecast operation, As malloc returns a void pointer.
- size is the number of bytes malloc need to allocate.
Example Usage of malloc() function in C language:
Let’s allocate the 40 Bytesof memory using the malloc() function.
1 |
int *iPtr = (int *) malloc(40); |
If the above call to the malloc() function is successful, Then the malloc() function will allocate 40 Bytes of memory in the HEAP section and The starting address of the allocated memory will be stored in the iPtr pointer variable.
If the malloc() is not able to allocate the 40 Bytes in the Heap, Then the iPtr will have the NULL value. So it is always recommended to check the pointer variable( iPtr) to verify the status of the memory allocation. We can accommodate 10 integer variables in the 40 Bytes of memory (assuming 4 Bytes for each integer)
1 2 3 4 5 6 7 8 9 |
int *iPtr = (int *) malloc(40);  if( iPtr == NULL) {     // Memory not allocated     printf("ERROR! malloc() Failed Allocate the Memory\n"); }  // Memory is allocated. |
We can also use the sizeof() operator to properly allocate the memory. For example, If you want to allocate memory for 90 integers, Then you can pass sizeof(int) * 90 to the malloc() function.
1 2 3 4 5 6 7 |
int *iPtr = (int *) malloc ( sizeof(int) * 90 );  if( iPtr == NULL) {     // Memory not allocated     printf("ERROR! malloc() Failed Allocate the Memory\n"); } |
Example 1: Program to understand the malloc() function in C language:
Here is the program to understand how the malloc() function works in the c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
/*     Program to allocate memory using malloc() function     sillycodes.com */  #include <stdio.h> #include <stdlib.h>  int main() {     int numInt;      printf("Enter the number of integers you want to generate dynamically: ");     scanf("%d", &numInt);      int *iPtr;      iPtr = (int *) malloc(numInt * sizeof(int));      // check iPtr     if(iPtr == NULL)     {         printf("ERROR! Failed to Allocate Memory\n");         return 0;     }      printf("SUCCESS! Memory allocated successfully\n");      // free the memory before returning.     free(iPtr);      return 0; } |
We have used the malloc() function to dynamically allocate the memory for the integers. The program prompts the user to provide a number and stores it in numInt variable. Then it allocates the memory for numInt number of integers in Heap using the malloc() function.
1 |
iPtr = (int *) malloc(numInt * sizeof(int)); |
The malloc() function allocates the memory and returns a void pointer. As we are going to store the integers, We need to typecast the pointer to the integer pointer.
Finally, We are checking the pointer value iPtr and print the result on the console.
📢We also used the free() function to free the dynamically allocated memory. We will look at the free() function in detail in the next sections of this post.
Program Output:
Let’s compile and run the program using your favorite compiler. We are using the GCC compiler in this example.
1 2 3 4 5 |
$ gcc malloc.c $ ./a.out Enter the number of integers you want to generate dynamically: 10 SUCCESS! Memory allocated successfully $ |
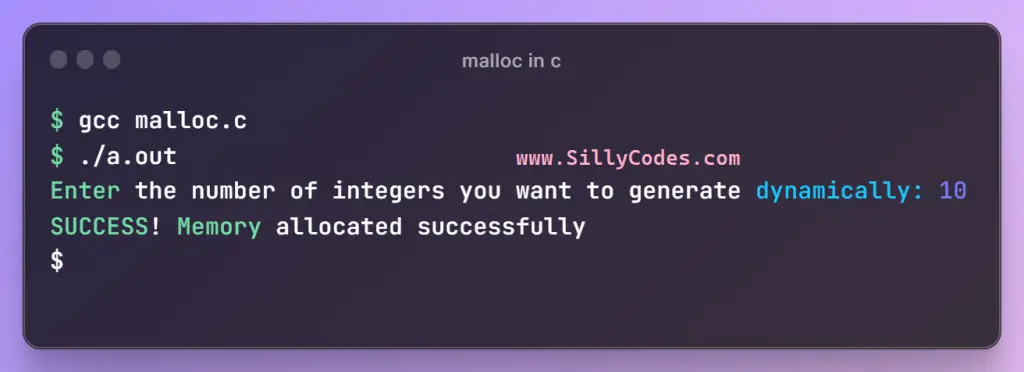
As we can see from the above output, The malloc() function is able to allocate the memory successfully.
Example 2: Allocate memory dynamically using malloc() function in C language:
Let’s create a program that takes and prints the user-supplied dynamic number of integers on the console.
For example, the user may want 5 integers, 10 integers, or 1000 integers, so we must create memory dynamically based on the user input(number of integers) and then take the integer numbers from the user and print them on the console.
Here is the program to dynamically create integer variables using malloc() function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
/*     Program to allocate memory using malloc() function     sillycodes.com */  #include <stdio.h> #include <stdlib.h>  int main() {     int numInt, i;      printf("Enter the number of integers you want to generate dynamically: ");     scanf("%d", &numInt);      int *iPtr;      iPtr = (int *) malloc(numInt * sizeof(int));      // check iPtr     if(iPtr == NULL)     {         printf("ERROR! Failed to Allocate Memory\n");         return 0;     }      printf("SUCCESS! Memory allocated successfully\n");         // Take the use input and update the integers     for(i = 0; i<numInt; i++)     {         printf("Enter an Integer: ");         scanf("%d", iPtr+i);     }      // print the values     printf("Entered Integers are : ");     for(i = 0; i<numInt; i++)     {         printf("%d ", iPtr[i]);    // we can also use *(iPtr+i)     }     // new line     printf("\n");      // free the memory before returning.     free(iPtr);      return 0; } |
The program allocates memory for the numInt integers, As the malloc() allocated memory is a contiguous block of memory, We used a for loop to iterate over the memory block and updated it with user-provided values. Then, We used the iPtr+i to get the address of the memory location. Where i is the index and iPtr is the pointer returned by malloc() function.
Finally, We used another for loop to display the values, We utilized the pointer subscript notation to get the value of the integer through the pointer variable iPtr. – iPtr[i]
Program Output:
Compile the program.
$ gcc create-int-dynamic.c
Run the program.
Test case 1:
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter the number of integers you want to generate dynamically: 5 SUCCESS! Memory allocated successfully Enter an Integer: 77 Enter an Integer: 99 Enter an Integer: 43 Enter an Integer: 76 Enter an Integer: 29 Entered Integers are : 77 99 43 76 29 $ |
As we can see from the above output, The program dynamically allocated the memory for five integers and stored the given numbers on allocated memory, and finally, printed them back on the console.
Test Case 2:
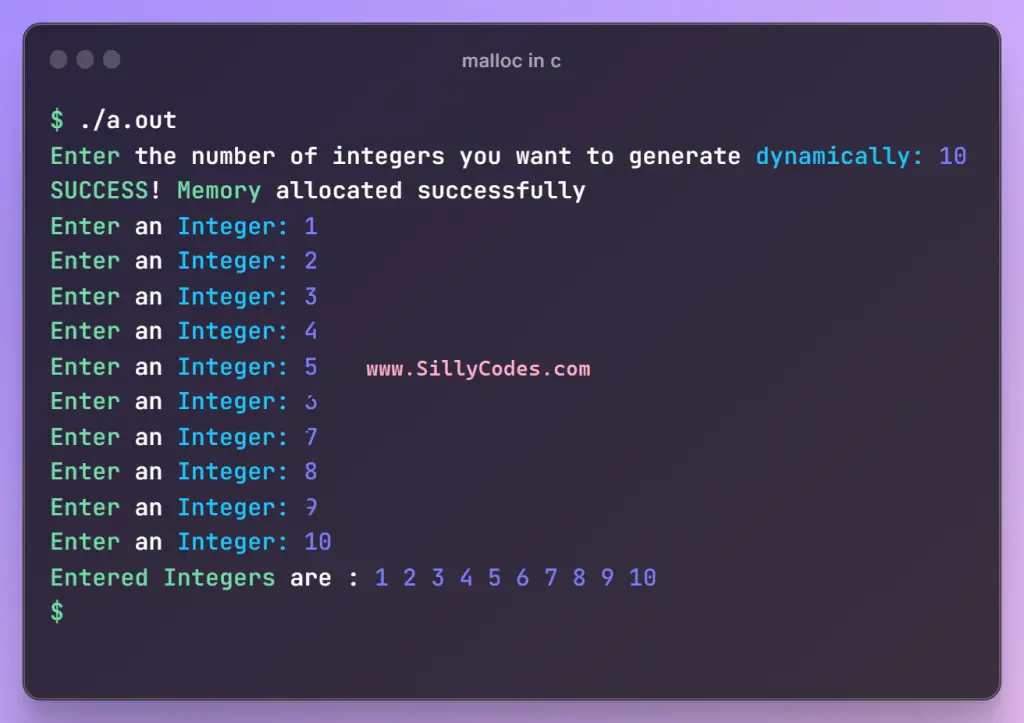
In this example, We have dynamically allocated memory for the ten integers.
Test Case 3 – malloc() function with negative values:
1 2 3 4 |
$ ./a.out Enter the number of integers you want to generate dynamically: -1 ERROR! Failed to Allocate Memory $ |
If you provide a negative number, Then the malloc() function will fail as it only accepts the size_t which is equal to unsigned int.
Related Articles:
- C Tutorials Index
- C Programs Index – 300+ Programs
- Structure of C program
- Compilation stages or Compilation Process in C
- Set, Clear, Toggle Bit, and Check a single Bit in C LanguageÂ
- Call by Value and Call by Address / Call by Reference in C Langauge
- Recursion in C Language with Example Programs and Call Flow
- Local Variable and Global Variables in C
- Variable Argument functions in C Language
3 Responses
[…] have looked at the static vs dynamic memory allocation and malloc() function in earlier articles, In today’s article, We are going to look at the calloc function in […]
[…] malloc() function […]
[…] earlier posts, we looked at static vs dynamic memory allocation, Memory allocation using the malloc() function and calloc() function. In today’s article, We will look at the Reallocation of […]