Local variables and Global variables in C Language
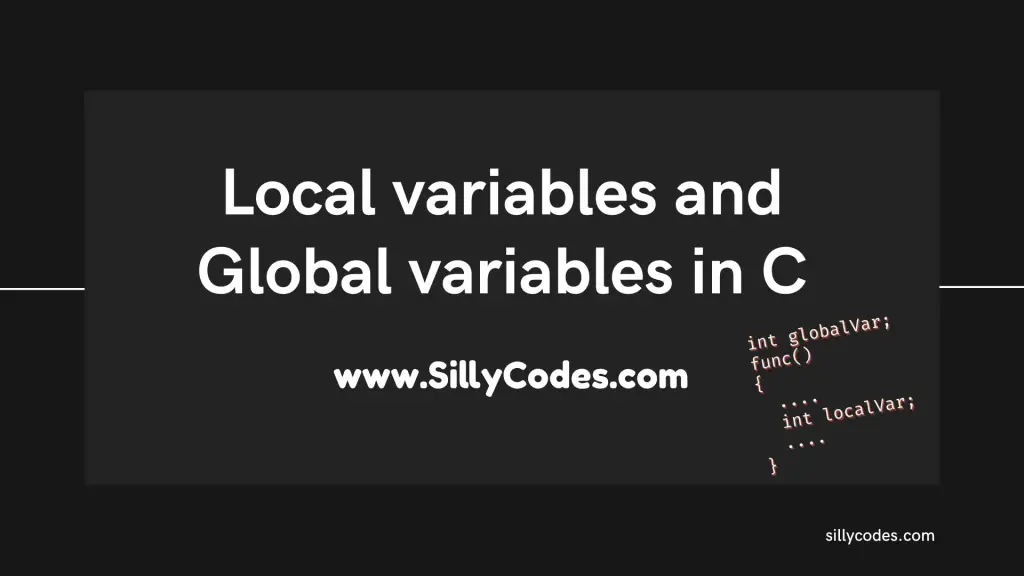
Local variables and Global variables in C Language Introduction:
We have looked at the Functions in C and Recursion in C in our earlier articles, In today’s article, We are going to look at the Local variables and Global variables in C programming language with example programs.
Local Variables in C language:
The variables which are declared within the block of code ( block scope ) are called Local Variables. The block of code can be a function block, if-else block, Loop block, etc.
📢 The Code block (block of code) is a collection of statements that are enclosed within the curly braces { … }.
So All the variables which are declared within the function are also local variables. The local variables are accessible only within the declared function. So you can’t access them from other functions or outside of the declared function.
1 2 3 4 5 6 7 |
{ int num1, num2;Â Â Â Â // local variables in this block scope // statements // statements .... .... } |
The local variables are created when the function is called and they will be destroyed once the function returns (completes). Local variables are stored in the Stack section. The local variables by default contain the garbage value. So it is a good idea to Initialize with an appropriate default value.
The formal arguments of a function are also considered as the local variables, As they are bound by the function scope and lifetime, but these variables are Initialized with the values passed through the function call.
So If variables are declared inside the function block, They will be accessible only within the function, Similarly, If they are declared within the if-else block or Loop or any nested block statement, Then they will only be accessible in the respective block scope.
Local variables declared within the Code block (Block Scope { ... }):
The Variables declared within the block of code enclosed by curly braces { .. } will be local to that block.
Let’s look at the program to understand the block scope.
Program to understand Block Scope in C Langauge:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
/*     Program to understand block scope in C. */  #include<stdio.h> int main() {     int num=63, outerNum = 77;      // num, outerNum are available in this scope     printf("Outside Block : num:%d, outerNum:%d \n", num, outerNum);      {         int num = 44;         int innerNum = 10;         // num, innerNum, outerNum are available in this scope         printf("Inside Block : num:%d, outerNum:%d, innerNum:%d \n", num, outerNum, innerNum);     }      // num, outerNum are available in this scope     printf("Outside Block : num:%d, outerNum:%d \n", num, outerNum);      // If you try to access innerNum, we will get error, So following line will give error.     // printf("Inside Block : num:%d, outerNum:%d, innerNum:%d \n", num, outerNum, innerNum);      return 0; } |
Program Output:
Compile and run the program.
1 2 3 4 5 6 |
$ gcc block-scope.c $ ./a.out Outside Block : num:63, outerNum:77 Inside Block : num:44, outerNum:77, innerNum:10 Outside Block : num:63, outerNum:77 $ |
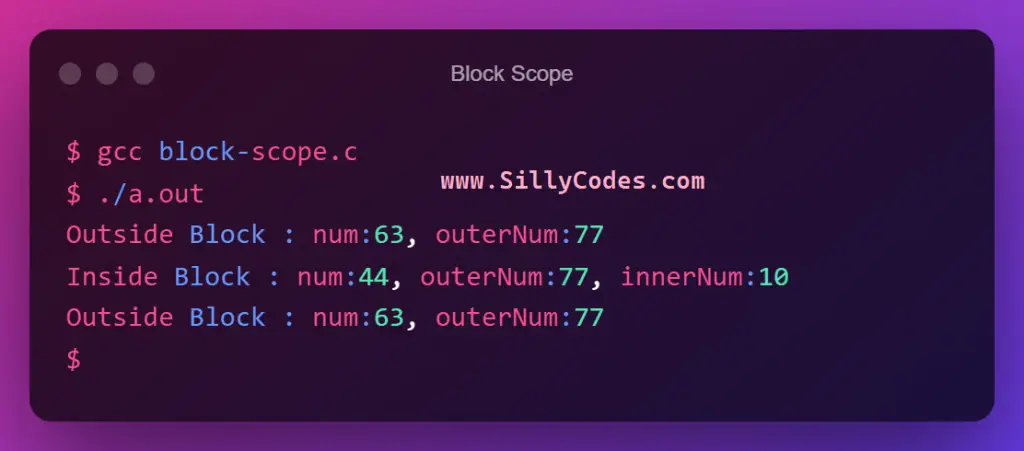
Local Variable Program Explanation:
In the above program, We have two scopes, One is the main() function scope. and another scope is created by following the code block.
1 2 3 4 5 6 |
{     int num = 44;     int innerNum = 10;     // num, innerNum, outerNum are available in this scope     printf("Inside Block : num:%d, outerNum:%d, innerNum:%d \n", num, outerNum, innerNum); } |
From the program output, We can observe the following things.
- The variables declared within the code block are accessible within the respective block ( nested blocks if any) only. They are local to the code block itself. That is the reason, The variable innerNum is only available within the block, and can’t be accessible outside of the block, like the main() function. If we try to access the variable innerNum from main function, We will get an error ( error: ‘innerNum’ undeclared).
- We can access the outer scope variables inside the inner scope.
- In the above example, We are able to access the variable outerNum in both the main() function scope and block scope. Inside Block : num:44, outerNum:77, innerNum:10
- We can create multiple variables with the same name in different scopes.
- Here we have created two num variables, One in the main function scope and another one in the code block scope.
- When we create multiple variables with the same name in different scopes, The innermost scope variable value will be considered.
- So when we printed the num value inside the code block, It printed as num value as 44, But when we printed the num value in the main function, we got the num value as 63. As the innermost scope overrides or shadow’s the outer scope variable value.
1 2 |
Inside Block : num:44, outerNum:77, innerNum:10 Outside Block : num:63, outerNum:77 |
Local Variables with Function in C Language:
As the function is a block of code, Any variables which are defined inside the function are only available within the function and can’t be accessed from outside the function.
Let’s quickly look at an example
Program to understand Function scope in C Langauge:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
/*     Program to understand function scope in C. */  #include<stdio.h>  void welcome(int num) {     int funcVar = 20;      // 'num' and 'funcVar' are local to 'welcome' function     printf("Welcome function - num:%d, funcVar:%d\n", num, funcVar); }  int main() {     int a = 10;     printf("InMain: a: %d \n", a);     welcome(a);      // The following 'printf' will give error.     // as 'funcVar' is local to 'welcome' function and not defined in 'main'     // printf("funcVar: %d \n", funcVar);      return 0; } |
Program Output:
1 2 3 4 |
$ ./a.out InMain: a: 10 Welcome function - num:10, funcVar:20 $ |
As we can see from the above output, The variables num and funcVar are local to the welcome function, So we can’t access them in the main() function, If we try to access the funcVar inside the main function, We will get error: ‘funcVar’ undeclared error.
The formal argument num also local to the welcome function, The value of the actual argument a is copied to the num formal argument.
Similarly, We can have local variables for the if-else block and Loop block.
Global Variables in C Programming Language:
The variables which are declared outside of the functions are called global variables. The global variables can be accessed from any function.
There are two types of global variables,
- Extern global variables
- The extern global variables can be accessed from any other file( source file ) as well.
- static global variables.
- The static global variables have file scope, So they will available in the declared file scope only and can’t be accessed from other C source files of the application. So if you want to have a variable, which is only used in a single file, Then you can go for the static global variable.
📢 The externand static are storage classes of the variables, We are going to dive deep into the storage classes in the next article. Where we explain the extern and static storage classes with example programs.
The global variables are lives throughout the life of the program. They will be created when the program starts and destroyed once the program ends.
Global variables are stored in the data section and by default Initialized with the value Zero.
📢The global variables can be accessed and modified by any function in the program.
Here is an example program to demonstrate the behavior of global variables in the C Programming Language:
Program to demonstrate Global variables in C programming:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
/* Â Â Â Â Program to understand Global Variables in C Language. */ Â #include<stdio.h> Â // Global Variable // Intentionally not initialized, As global variables initialized with zero by default int championNum; Â /* Â Â Â Â Update the global variable ('championNum') with Champion number of 2020 */ Â void year2020() { Â Â Â Â championNum = 44; Â Â Â Â printf("In Year2020 - championNum: %d\n", championNum); } Â /* Â Â Â Â Update the global variable ('championNum') with Champion number of 2021 */ void year2021() { Â Â Â Â championNum = 33; Â Â Â Â printf("In Year2021 - championNum: %d\n", championNum); } Â int main() { Â Â Â Â // main can access the 'championNum' Â Â Â Â printf("Main Function Start - championNum : %d \n", championNum); Â Â Â Â Â // calling year2020 Â Â Â Â year2020(); Â Â Â Â Â // calling year2021 Â Â Â Â year2021(); Â Â Â Â Â // Main gets the changed 'championNum' Â Â Â Â printf("Main function End - championNum : %d \n", championNum); Â Â Â Â return 0; } |
Program Output:
compile and run the program using the GCC compiler.
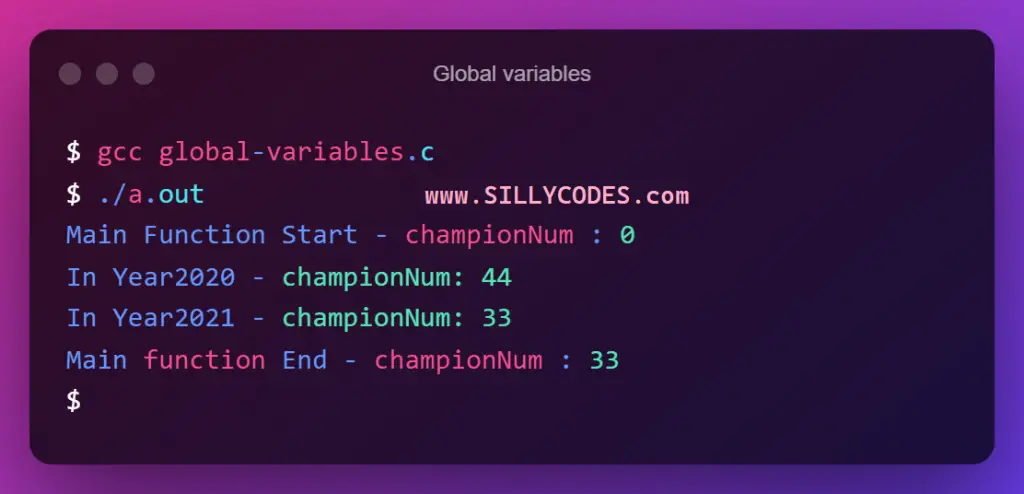
1 2 3 4 5 6 7 |
$ gcc global-variables.c $ ./a.out Main Function Start - championNum : 0 In Year2020 - championNum: 44 In Year2021 - championNum: 33 Main function End - championNum : 33 $ |
Global variables in C program explanation:
We have defined three functions in the above program
- main function
- year2020 function
- year2021 function
We also created a global variable called championNum. As it is a global variable, We created it outside of all functions. As it is a global variable, the Compiler initialized it with the Zero.
Then we are able to access and modify the championNum global variable from all the functions. If you notice, The functions year2020 and year2021 both modified the championNum.
Local variables and Global variables in C – Conclusion:
We learned about the Local variables and global variables in C programming language with example programs. We looked at the variable scopes like block scope, function scope, and global scope variables. How scope affects the variable visibility to the program.
In the next tutorial, We will look at the storage classes in C Langauge.
Functions Practice Programs:
- Arithmetic Operations using functions
- Fibonacci Series using function
- Factorial of a Number using functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C
Recursion Practice Programs:
- C Program to Find Factorial of Number using Recursion
- C Program to Generate Fibonacci Number using Recursion.
- C Program to calculate the sum of Digits of Number using Recursion
- C Program to Print First N Natural Numbers using Recursion
- C Program to find sum of Fist N Natural Number using Recursion
- Product of N natural Number using Recursion C Program
- Program to Count number of Digits in a Number using Recursion
- 100+ C Practice Programs
- C Tutorials Index
4 Responses
[…] Local Variable and Global Variables in C […]
[…] by declaring a string and naming it as inputStr with the size of 100 characters (We are using a global constant to store the […]
[…] the program by declaring the input string( inputStr) with the size of SIZE character. The SIZE is a global constant, which holds the max size of […]
[…] Local Variable and Global Variables in C […]