Program to Generate Armstrong numbers upto n in C Language
- Armstrong Numbers upto n in C Program Description:
- What is an N-Digit Armstrong Number?
- Pre-Requisites:
- Armstrong Numbers upto n in C Program Algorithm:
- Armstrong Numbers upto n in C Program using Custom Pow function:
- Program to generate Armstrong Numbers upto n in C using In-Built Pow Function:
- Related Programs:
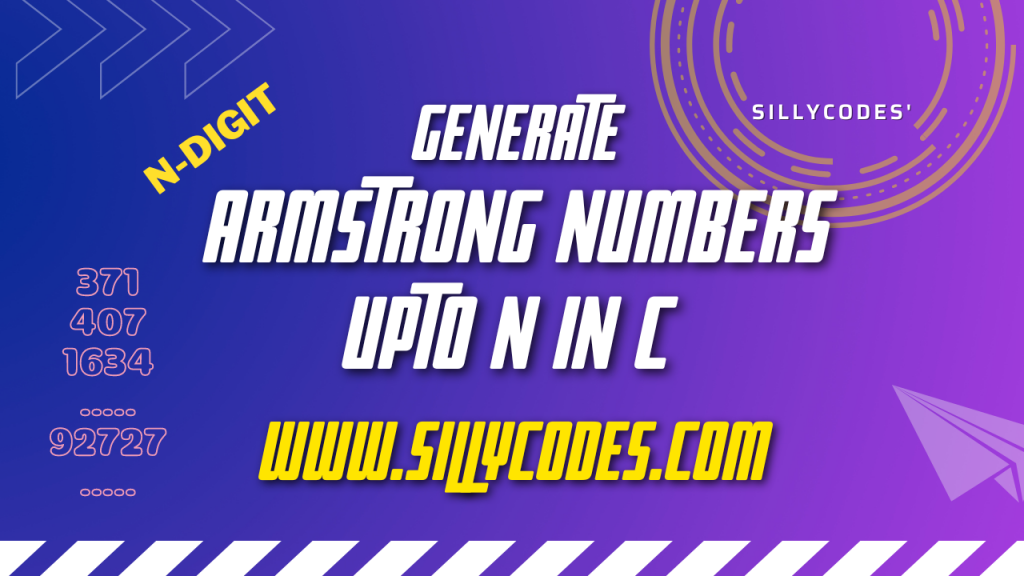
Armstrong Numbers upto n in C Program Description:
In our previous article, We have looked at the 3-Digit and N-digit Armstrong number program. In today’s article, We are going to extend that program and will write a program to generate the Armstrong numbers upto n in C language. n means the user-provided number. So The program should accept a number from the user and generate or print all Armstrong numbers up to the given number.
📢 The Program should handle all digits of Armstrong numbers i.e 3-digit and N-digit Armstrong numbers
Here is the expected Input and output of the program.
Example Input and Output:
Input:
Enter a Number(To generate armstrong number upto it): 5000
Output:
Armstrong numbers upto 5000 are : 1 2 3 4 5 6 7 8 9 153 370 371 407 1634
What is an N-Digit Armstrong Number?
A positive number of n digits is said to be an Armstrong number of order n, If the sum of the power of n of each digit in the number is equal to the original number itself.
If the number is qwer is Armstrong number, Then it should satisfy the below equation.
qwer = pow(q, n) + pow(w, n) + pow(e, n) + pow(r, n)
Here n is the number of digits in the number qwer which is 4
The number 54748 is an Armstrong number, So ( n = 5 i.e number of digits in the 54748)
54748 = pow(5, 5) + pow(4, 5) + pow(7, 5) + pow(4, 5) + pow(8, 5)
54748 = 3125 + 1024 + 16807 + 1024 + 32768
54748 = 54748
As the powers of n of each digit in the number is equal to the number itself. So the number 54748 is an Armstrong number.
📢 It is recommended to look at the 3-Digit / N-digit Armstrong number program. Where we explained about the Armstrong number in detail.
Here are a few Armstrong numbers for your reference.
Digit | Armstrong Numbers |
---|---|
1 | 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 |
3 | 153, 370, 371, 407 |
4 | 1634, 8208, 9474 |
5 | 54748, 92727, 93084 |
6 | 548834 |
7 | 1741725, 4210818, 9800817, 9926315 |
Pre-Requisites:
It is recommended to have knowledge of the C Loops, and Arithmetic Operators to better understand the program. We have already covered these topics in earlier posts, Please go through the following links to learn more about these topics.
Armstrong Numbers upto n in C Program Algorithm:
We are going to use the N-Digit Armstrong number program, As it will cover all digits cases (Including the 3-digit Armstrong numbers).
The generate the N-digit Armstrong numbers, We need to calculate the digit with the power of nDigits i.e pow(digit, nDigits).
Program Algorithm:
- Start the Program by taking input from the user and storing it in variable n ( We are going to generate all Armstrong numbers up to this number n)
- Quickly check if the number n is a Negative number, If so display the error message and terminate the program. Otherwise, proceed to the next step.
- As specified earlier, We need to calculate the pow(digit, nDigits) to check the Armstrong number.
- So we need to count the number of digits in the given number n. To do that, We use a function called countDigits(), Which takes a number as input and returns the number of digits.- int nDigits = countDigits(temp);
- We are going to use two loops, Outer-Loop and Inner-Loop.
- The Outer-Loop: This starts from the number
1 and goes all the way up to the number
n. We use variable
i to control Outer-Loop.
- Take the back up of i to variable temp. As we need i for later comparisons.
- The Inner-Loop: Inner-Loop will help us to check if the outer loop variable
i is an Armstrong number or not. If the
i is Armstrong number, Then it will print it onto the console.
- At Each Iteration,
- Get the last digit from the number using rem = temp %10;
- Calculate the power of rem with nDigits(Number of digits in n) – i.e power(rem, nDigits);. We are using the Power of Number Program in C to calculate the power instead of the pow built-in function. Then Update the arm variable.
- Remove the last digit using the temp/10 operation.
- Check if the variable arm is equal to i. If both are equal, Then i is an Armstrong number. Print it onto the console.
- The above step-6 will continue until i reaches the given number n. ( Outer Loop Condition – i<=n )
- Once the above two loops are terminated, We will get all Armstrong numbers from the number 1 to n. ( user-provided number)
Armstrong Numbers upto n in C Program using Custom Pow function:
We are using a custom power function in this program. You can see the custom power function definition at line 31. We also defined another function countDigits(), which helps us to calculate the number of digits in a number.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 |
/*     Program to generate Armstrong numbers up to the given number     Author: SillyCodes.com */  #include<stdio.h>  /* * count number of digits of given number 'num' * Lean more at - https://sillycodes.com/program-to-count-number-of-digits-in-c/ */ int countDigits(int num) {     int digitCnt = 0;     // Number is positive     // take backup of 'num', we can also directly use 'num'     int temp = num;     // Iterate over each digit using division operator.     while(temp > 0) {         // increment the number of digits count (digitCnt)         digitCnt++;         // remove the last element using division operator.         temp = temp / 10;     }      return digitCnt; }  /* * Calculate the power of number * Learn more at - https://sillycodes.com/program-to-find-power-of-number-in-c/#5-method-11-calculate-the-power-of-number-in-c-language-using-while-loop- */ double power(double base, double exponent) {     long double res = 1.0;     // The power of base and exponent is equal to ( base multiplied exponent times)     // So loop until the exponent became zero.     while(exponent > 0) {         res = res * base;  // res *= base;         exponent--;     }     return res; }  int main() {     int n, temp, rem, arm, i;      // Take the input from the user.     printf("Enter a Number(To generate armstrong number upto it): ");     scanf("%d",&n);      // check if the 'n' is negative. Display the error message.     if(n <= 0)     {         printf("Error: Please enter positive numbers\n");         return 0;     }      printf("Armstrong numbers upto %d are : ", n);      // Start the loop from '1' go till 'n'     for(i=1; i<=n; i++)     {         // Take backup of number 'n'         temp = i;          // reset 'rem', 'arm' variables         rem = 0;         arm = 0;          // count number of digits in Number         // Use - https://sillycodes.com/program-to-count-number-of-digits-in-c/         int nDigits = countDigits(temp);          // Go through the each digit and calculate the cube of each digit         // add the cubes to 'arm' variable. which will be holding sum of all cubes         while(temp>0)         {             // get the last digit of number or current digit using modulus             rem = temp %10;                         // Using custom power function. Pass 'base' and 'exponent'             // Source - https://sillycodes.com/program-to-find-power-of-number-in-c/             // calculate the power of 'rem' with 'nDigits'             // You can also use `math.h` in-built 'pow' function             arm = arm + power(rem, nDigits);                         // Remove the last digit by dividing the 'temp' by '10'             temp = temp/10;         }          // After all iterations, The 'arm' variable contains the sum of cubes of each digit.         // if 'arm' is equal to the 'i', Then the 'i' is Armstrong number.         if(arm == i)             printf("%d ", i);     }         printf("\n");     return 0; } |
Program Output:
Compile the program.
$ gcc armstrong-upto-n.c
As we are using our own power function, We no need to add the -lm compilation option.
📢 If you are using the in-built pow function, Then don’t forget to include the math.h in your C Program. And also pass the -lm option while compiling the program.
Let’s run the program
1 2 3 4 |
$ ./a.out Enter a Number(To generate armstrong number upto it): 5000 Armstrong numbers upto 5000 are : 1 2 3 4 5 6 7 8 9 153 370 371 407 1634 $ |
As you can see from the above output, When a user entered the number n as 5000, The program generated all Armstrong (3-digit and N-digit) numbers up to the number 5000. i.e 1 2 3 4 5 6 7 8 9 153 370 371 407 1634
Let’s look at a few more examples
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter a Number(To generate armstrong number upto it): 200Â Â Armstrong numbers upto 200 are : 1 2 3 4 5 6 7 8 9 153 $ ./a.out Enter a Number(To generate armstrong number upto it): 10000 Armstrong numbers upto 10000 are : 1 2 3 4 5 6 7 8 9 153 370 371 407 1634 8208 9474 $ ./a.out Enter a Number(To generate armstrong number upto it): 100000 Armstrong numbers upto 100000 are : 1 2 3 4 5 6 7 8 9 153 370 371 407 1634 8208 9474 54748 92727 93084 $ |
The program is generating the Armstrong numbers upto the user-provided number correctly. We can see the from the output, The program generated Armstrong numbers up to 100000 properly.
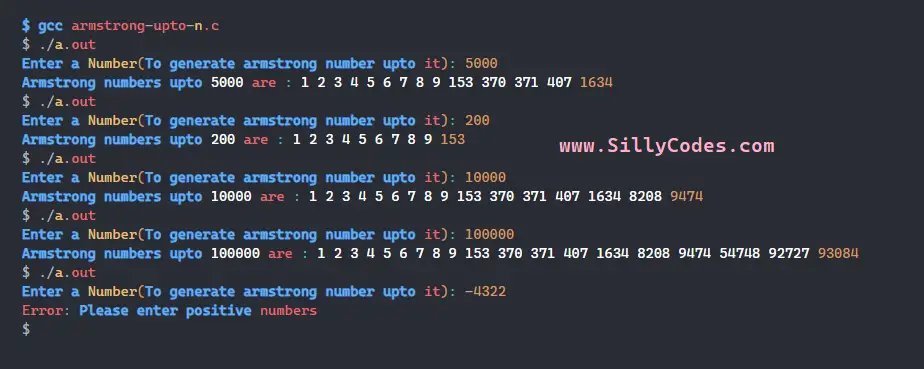
What if the user enters a negative number? Let’s test one test case for it as well.
1 2 3 4 |
$ ./a.out Enter a Number(To generate armstrong number upto it): -4322 Error: Please enter positive numbers $ |
Program to generate Armstrong Numbers upto n in C using In-Built Pow Function:
We have changed a couple of things in this program. We removed our custom power function from the program. As we have the in-built power function pow. The in-built pow function is available as part of the math.h header file. So we included the math.h header file to our program and calculate the power using the pow(rem, nDigits).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 |
/*     Program to generate Armstrong numbers up to the given number     Author: SillyCodes.com */  #include<stdio.h> #include<math.h>  /* * count number of digits of given number 'num' * Lean more at - https://sillycodes.com/program-to-count-number-of-digits-in-c/ */ int countDigits(int num) {     int digitCnt = 0;     // Number is positive     // take backup of 'num', we can also directly use 'num'     int temp = num;     // Iterate over each digit using division operator.     while(temp > 0) {         // increment the number of digits count (digitCnt)         digitCnt++;         // remove the last element using division operator.         temp = temp / 10;     }      return digitCnt; }  int main() {     int n, temp, rem, arm, i;      // Take the input from the user.     printf("Enter a Number(To generate armstrong number upto it): ");     scanf("%d",&n);      // check if the 'n' is negative. Display the error message.     if(n <= 0)     {         printf("Error: Please enter positive numbers\n");         return 0;     }      printf("Armstrong numbers upto %d are : ", n);      // Start the loop from '1' go till 'n'     for(i=1; i<=n; i++)     {         // Take backup of number 'n'         temp = i;          // reset 'rem', 'arm' variables         rem = 0;         arm = 0;          // count number of digits in Number         // Use - https://sillycodes.com/program-to-count-number-of-digits-in-c/         int nDigits = countDigits(temp);          // Go through the each digit and calculate the cube of each digit         // add the cubes to 'arm' variable. which will be holding sum of all cubes         while(temp>0)         {             // get the last digit of number or current digit using modulus             rem = temp %10;                         // calculate the power of 'rem' with 'nDigits'             // We are using `math.h` in-built 'pow' function             arm = arm + pow(rem, nDigits);                         // Remove the last digit by dividing the 'temp' by '10'             temp = temp/10;         }          // After all iterations, The 'arm' variable contains the sum of cubes of each digit.         // if 'arm' is equal to the 'i', Then the 'i' is Armstrong number.         if(arm == i)             printf("%d ", i);     }         printf("\n");     return 0; } |
Program Output:
As we are using the pow function from the math.h header file, We need to include the libm.so library file. To include the libm.so library file, We need to pass the -lm option during the compilation.
$ gcc armstrong-upto-n-inbuilt-pow.c -lm
Run the program.
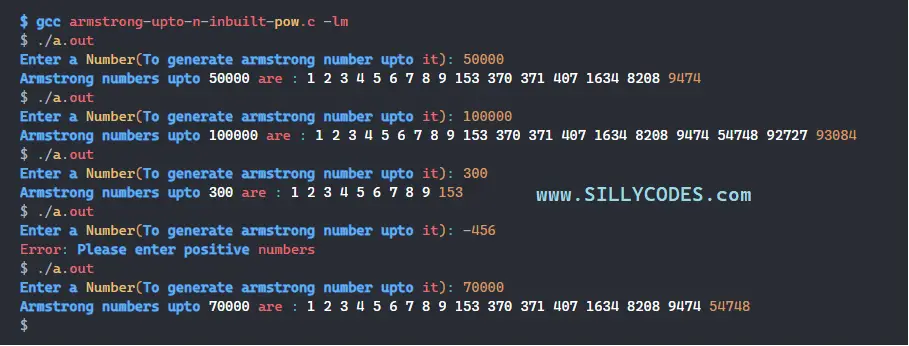
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
$ ./a.out Enter a Number(To generate armstrong number upto it): 50000 Armstrong numbers upto 50000 are : 1 2 3 4 5 6 7 8 9 153 370 371 407 1634 8208 9474 $ ./a.out Enter a Number(To generate armstrong number upto it): 100000 Armstrong numbers upto 100000 are : 1 2 3 4 5 6 7 8 9 153 370 371 407 1634 8208 9474 54748 92727 93084 $ ./a.out Enter a Number(To generate armstrong number upto it): 300 Armstrong numbers upto 300 are : 1 2 3 4 5 6 7 8 9 153 $ ./a.out Enter a Number(To generate armstrong number upto it): -456 Error: Please enter positive numbers $ ./a.out Enter a Number(To generate armstrong number upto it): 70000 Armstrong numbers upto 70000 are : 1 2 3 4 5 6 7 8 9 153 370 371 407 1634 8208 9474 54748 $ |
Related Programs:
- Collection of C Loops Programs [ List of Loop Practice Programs ]
- C Program Check Prime Number [Mutliple Methods]
- C Program to Convert Decimal Number to Binary Number
- C Program to Convert Binary Number to Decimal Number
- C Program to Convert Octal Number to Decimal Number
- C Program to Calculate the Factorial of Number
- C Program to Find all Factors of a Number
- C Program to find all Prime Factors of numberÂ
- C Program to Calculate the GCD or HCF of Two Number
- C Program to Calculate the LCM of two Numbers
- C Program to Check Palindrome Number
2 Responses
[…] C Program to Generate Armstrong Numbers upto N ( User-Provided Number) […]
[…] C Program to Generate Armstrong Numbers upto N ( User-Provided Number) […]