Program to Count Occurrences of substring in String in C Language
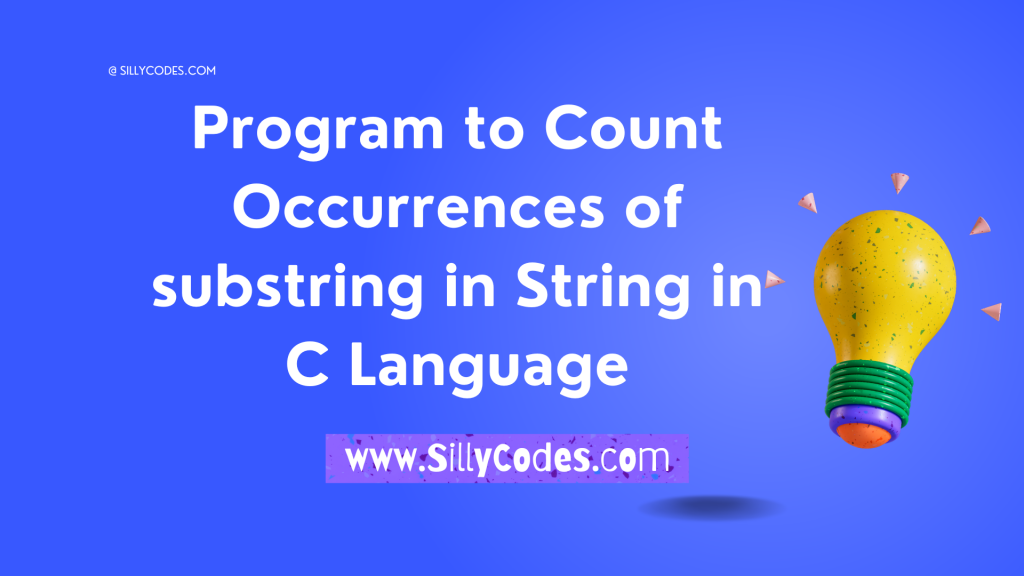
Program Description:
Write a Program to Count Occurrences of substring in String in C Programming language. The program should accept two strings from the user, one for the main string and one for the substring. The program needs to count all occurrences of the substring within the main string and return the result.
📢 This Program is part of the C String Practice Programs series
We are going to look at a couple of methods to find all occurrences of a substring in a string and each program will be followed by a step-by-step explanation and program output. We also provided instructions for compiling and running the program using your compiler.
Let’s look at the example input and output of the program.
Input and Output:
Input:
Enter a main string : git init, git add, and git commit
Enter the substring(String to search in main string): git
Output:
FOUND! Substring:'git' appeared 3 times in the main string
The substring git is appeared three times in the input string.
📢 Note: The program is case-sensitive. As a result, upper-case characters will differ from lower-case characters.
Prerequisites:
Please go through the C-Strings, C-Arrays, and Functions in C to understand the following program better.
We will look at two methods to count all occurrences of the substring in the string. They are
- Manual Approach(Iterative)
- User-defined function
Count All Occurrences of a substring in a string in C Program Explanation:
The instructions to find all occurrences of a string in a string using an iterative method.
- Start the main() function by declaring the two strings and let’s say the main string as mainStr and the substring as subStr. The size of both strings is equal to SIZE macro.
- Initialize a variable called counterwith the value Zero(0). The counter variable will be used to count the number of occurrences of the substring in the main string.
- Read the two strings from the user and store them in mainStr and subStr respectively. We use the gets function to read the strings.
- To Count number of occurrences of substring( subStr) in the main string( mainStr), Iterate through the main string( mainStr), looking for the first character of the substring( subStr[0]). If we found the first character of the substring, we should then determine whether all other characters of the substring match. If that’s the case, we found the substring( subStr) in the main string( mainStr). Similar way we need to look for all occurrences of the substring.
- Create a For loop by initializing variable i with 0 to iterate over the
mainStr. It will continue looping as long as the current character (
mainStr[i]) is not a
NULL character (
'\0').
- At each iteration, Check if the current character( mainStr[i]) is equal to the first character of the substring( subStr[0]) - i.e if(mainStr[i] == subStr[0])
- If the above condition is
true, Then search for all characters of the
subStr in the
mainStr. We need to use another for loop to iterate over the contents of the
subStr – i.e
for(j=0; subStr[j]; j++).
- Check if the mainStr[k] (where k = i) is not matching to subStr[j], If so then the subString is not found. Otherwise, continue to check all elements of the subStr.
- Once the above loop is completed, Check if we iterated complete subStr ( use j == strlen(substr) ). If we iterated the complete substring( subStr), then we found the substring in the main string. So increment the counter variable – counter++;
- Continue to the next iteration to search for remaining occurrences of the substring ( subStr).
- If step 6 is completed, The
counter variable contains the total number of times the substring appeared in the main string.
- If counter is equal to zero(0), Then the substring( str) is not found the main string( mainStr). Display the NOT FOUND message on the console.
- If counter contains a value greater than zero, Then we found the substring. Display the FOUNDmessage along with the number of appearances.
- Stop the program.
Program to Count All Occurrences of Substring in a sentence in C:
Here is the program count all occurrences of word in a sentence in c.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
/* program to count all occurrences substring in a string in C sillycodes.com */ #include <stdio.h> #include <string.h> // Max Size of string #define SIZE 100 int main() { // declare two strings with 'SIZE' char mainStr[SIZE], subStr[SIZE]; int i, j, k; int counter = 0; // Take the main string from the user printf("Enter a main string : "); gets(mainStr); // take the sub string printf("Enter the substring(String to search in main string): "); gets(subStr); // Iterate over the main string for(i = 0; mainStr[i]; i++) { // search for the first character in substring if(mainStr[i] == subStr[0]) { // The first character of substring is matched. // Check if all characters of substring matching. k = i; for(j=0; subStr[j]; j++) { // printf("mainStr[%d]=%c, subStr[%d]=%c \n", i, mainStr[i],j, subStr[j]); if(mainStr[k] != subStr[j]) { // Not matched break; } k++; } // check if the 'j' is equal to length of the 'subString', Then we found the match if(j == strlen(subStr)) { // increment the counter. counter++; } } } // check the 'counter' if(counter == 0) printf("NOT FOUND! Substring:'%s' is NOT found in main string\n", subStr); else printf("FOUND! Substring:'%s' appeared %d times in the main string\n", subStr, counter); return 0; } |
Program Output:
Let’s compile and Run the program. We are using the GCC compiler to Compile and Run the C programs.
Test Case 1: Successful case:
1 2 3 4 5 6 |
$ gcc count-substr.c $ ./a.out Enter a main string : git init, git add, and git commit Enter the substring(String to search in main string): git FOUND! Substring:'git' appeared 3 times in the main string $ |
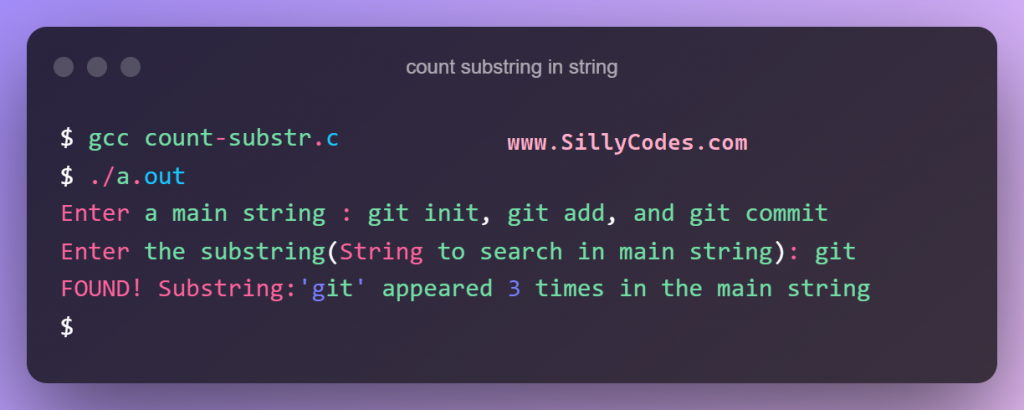
As we can see from the above output, The substring git is found in the main string( git init, git add, and git commit). It appeared three times.
Test case 2: Negative Case:
1 2 3 4 5 |
$ ./a.out Enter a main string : Learn Programming Enter the substring(String to search in main string): C NOT FOUND! Substring:'C' is NOT found in main string $ |
The character/substring C is not found in the main string. So program displayed the NOT FOUND message.
C Program to Count Occurrences of substring in String using a user-defined function:
In this method, we will break the above program into functions. Let’s create function to count the number of appearances of a substring in the main string.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 |
/* program to count all occurrences substring in a string in C using functions sillycodes.com */ #include <stdio.h> #include <string.h> // Max Size of string #define SIZE 100 /** * @brief - Count occurrences of substring in main string * * @param mainStr - main string * @param subStr - sub string * @return int - if subStr found, returns number of times substr found in mainStr, Otherwise returns 0 */ int countSubStr(char *mainStr, char * subStr) { int i, j, k; int counter = 0; // Iterate over the main string for(i = 0; mainStr[i]; i++) { // search for the first character in substring if(mainStr[i] == subStr[0]) { // The first character of substring is matched. // Check if all characters of substring matching. k = i; for(j=0; subStr[j]; j++) { // printf("mainStr[%d]=%c, subStr[%d]=%c \n", i, mainStr[i],j, subStr[j]); if(mainStr[k] != subStr[j]) { // Not matched break; } k++; } // check if the 'j' is equal to length of the 'subString', Then we found the match if(j == strlen(subStr)) { // increment the counter. counter++; } } } // return the 'counter' variable return counter; } int main() { // declare two strings with 'SIZE' char mainStr[SIZE], subStr[SIZE]; // Take the main string from the user printf("Enter a main string : "); gets(mainStr); // take the sub string printf("Enter the substring(String to search in main string): "); gets(subStr); // call the 'countSubStr' and pass two string mainStr and subStr int cnt = countSubStr(mainStr, subStr); // check the 'cnt' if(cnt == 0) printf("NOT FOUND! Substring:'%s' is NOT found in main string\n", subStr); else printf("FOUND! Substring:'%s' appeared %d times in the main string\n", subStr, cnt); return 0; } |
We have defined a function called countSubStr, This function is used to count the number of times the substring has appeared in the main string.
int countSubStr(char *mainStr, char * subStr)
As we can see from the above prototype, This function takes two character pointers( char *) as formal arguments. The first argument is mainStr and the second argument is subStr. The countSubStr() function counts all occurrences of the subStr in the mainStr.
This function also returns an integer value, It returns the total number of times subStr is found in the mainStr. If subStr not found in the mainStr, Then it will return .
To count the number of occurrences of the substring in the main string, Call the countSubStr function from the main() function.
1 2 |
// call the 'countSubStr' and pass two string mainStr and subStr int cnt = countSubStr(mainStr, subStr); |
Check the return value(i.e cnt) of the countSubStr() function and display the results.
Program Output:
Let’s compile and run the program.
1 2 3 4 5 6 |
$ gcc count-substr.c $ ./a.out Enter a main string : This is not the end. This is just the beginning Enter the substring(String to search in main string): is FOUND! Substring:'is' appeared 4 times in the main string $ |
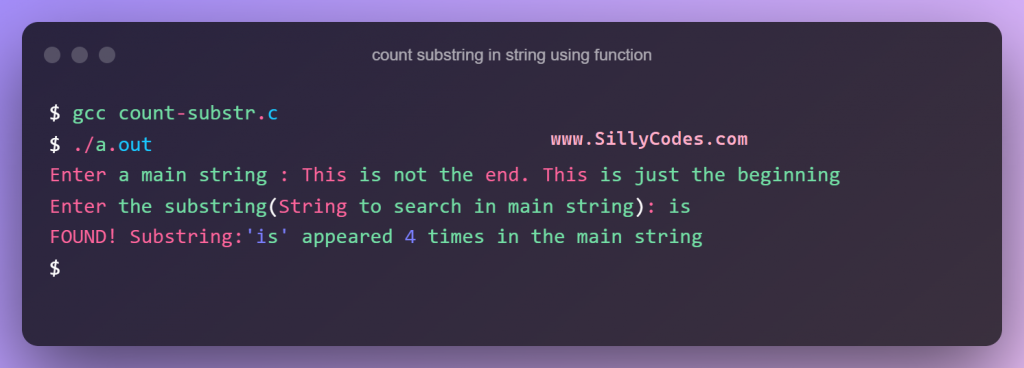
The substring is appeared four times in the main string.
1 2 3 4 5 |
$ ./a.out Enter a main string : Spaces or Tabs Enter the substring(String to search in main string): both NOT FOUND! Substring:'both' is NOT found in main string $ |
As we can see from the above examples, The countSubStr() function is properly counting the number of occurrences of the substring in a string and returning the results.
Related String Programs:
- C Tutorials Index
- C Programs Index
- C Program to Count Frequencies of each character in a string
- C Program to Find Highest Frequency character in a String
- C Program to Find Lowest Frequency Character in a String
- C Program to Remove Vowels from a String
- C Program to Remove Consonants from a String
- C Program to Sort String in Ascending order
- C Program to Sort String in Descending Order
- Sort String in Ascending and Descending order using Qsort()
- C Program to Find First Occurrences of a character in a String
- C Program to Find Last Occurrences of a character in a string