Passing 2d array to function in C with Example Program
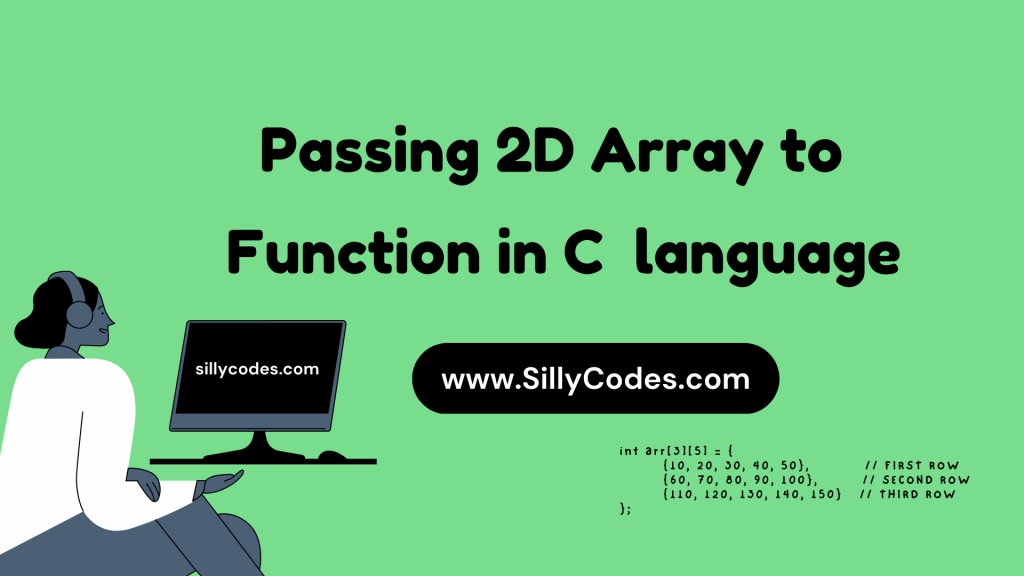
Passing 2d Array to Function in C Program Description:
In our previous article, We have looked at How to Read and Print the 2D Array elements in C language, In today’s article, We will look at Passing 2d Array to Function in C programming language with an example program. The Program should take an input matrix(2d array) from the user and Define two functions to read the 2d array elements and display the 2d array.
Example Input and Output:
Input:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
Enter the Rows size for Matrices(1-100): 4 Enter the Columns Size for Matrices(1-100): 3 Enter Elements for the Matrix(A) of Size 4X3: A[0][0]: 10 A[0][1]: 15 A[0][2]: 20 A[1][0]: 25 A[1][1]: 30 A[1][2]: 35 A[2][0]: 40 A[2][1]: 45 A[2][2]: 50 A[3][0]: 55 A[3][1]: 60 A[3][2]: 65 |
Output:
1 2 3 4 5 |
Elements of the 2D-Array or Matrix A are : 10 15 20 25 30 35 40 45 50 55 60 65 |
If we remove the array prompt ( A[i][j]) during the Input phase. Then the program no longer shows the element index like A[0][0], A[0][1], etc. Make sure to enter the correct number of elements 🐧
Input:
1 2 3 4 5 6 |
Enter the Rows size for Matrices(1-100): 3 Enter the Columns Size for Matrices(1-100): 3 Enter Elements for the Matrix(A) of Size 3X3: 11 22 33 44 55 66 77 88 99 |
Output:
1 2 3 4 |
Elements of the 2D-Array or Matrix A are : 11 22 33 44 55 66 77 88 99 |
Prerequisites:
It is recommended to go through the Arrays, Functions in C language, and 2D Arrays in C to better understand the following program.
- C Arrays
- 2D Arrays in C Language – How to Create and Use 2D Arrays or Matrices in C
- Functions in C with Examples
- Passing One-Dimensional Arrays to Functions.
Passing 2d Array to Function in C Program Explanation:
Let’s look at the step-by-step explanation of Passing a 2d Array to Function in a C Program.
- Create two Constants named MAXROWS and MAXCOLUMNS and initialize them with 100. The MAXROWS and MAXCOLUMNS constants hold the maximum size of the rows and columns of a 2D Array. If you want to use large arrays, Please change this number to the desired value.
- Declare the input array A[MAXROWS][MAXCOLUMNS] with MAXROWS and MAXCOLUMNS.
- Prompt the user to provide the desired row size and column size. and store the row size in rows variable and column size in columns variable.
- Check for array bounds using the (rows >= MAXROWS || rows <= 0 || columns >= MAXCOLUMNS || columns <= 0) condition, And display an error message if the rows or columnare beyond the present limits.
- Define Two Functions – readMatrix() function and displayMatrix() function.
- The
readMatrix() function used to read the input from the user and update the 2D Array or Matrix(
A) Elements
- void readMatrix(int rows, int columns, int A[rows][columns])
- The readMatrix() function takes three formal arguments, which are rows size, columns size, and A[][] 2D Array.
- This function uses two For loops to iterate over the given matrix A and updates its elements by using the scanf function – scanf(“%d”, &A[i][j]);
- readMatrix() function doesn’t return anything back to the caller. So return type is void.
- As the arrays are passed by the address/reference, All the changes made to the 2D Array in the readMatrix() function will be visible in the Caller Function (i.e main() function).
- The
displayMatrix() function is used to print the 2D Array or Matrix elements on the Console.
- void displayMatrix(int rows, int columns, int A[rows][columns])
- Similar to the readMatrix() function, The displayMatrix() function also takes three formal arguments. i.e rows, columns, and A[][] (2D Array)
- The displayMatrix() function uses two For loops to iterate over the A matrix and prints the elements of the matrix. – printf("%d ", A[i][j]);
- From the main() function, Call the readMatrix() function and pass the rows, columns, and 2D array( A) as the parameter – readMatrix(rows, columns, A);
- Similarly, Make another call to displayMatrix() function to display the 2D array on the console – displayMatrix(rows, columns, A);
Program to understand Passing 2d Array to Function in C:
Here is the program to demonstrate the Passing of 2D Array to Function in the C Programming language
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 |
/* Program to Add Two Matrices in C SillyCodes.com */ #include <stdio.h> // Create 'MAXROWS' and "MAXCOLUMNS" constants. // If you are using same number, then we can also use single constant. const int MAXROWS=100; const int MAXCOLUMNS=100; /** * @brief - Read 2D-Array or Matrix from the user and update 'A' * * @param rows - Rows in 'A' - Matrix(2D-Array) * @param columns - Columns in 'A' * @param A - 'A' is Matrix or 2D-Array */ void readMatrix(int rows, int columns, int A[rows][columns]) { int i, j; // Iterate through the rows for(i=0; i<rows; i++) { // Iterate through the columns for(j=0; j<columns; j++) { printf("A[%d][%d]: ", i, j); scanf("%d", &A[i][j]); } } } /** * @brief - Print the elements of the 2D-Array or Matrix * * @param rows - Rows in Matrix(2Darray) * @param columns - Columns in 'A' * @param A[][] - 'A' is a Matrix or 2D array */ void displayMatrix(int rows, int columns, int A[rows][columns]) { int i, j; // Traverse through the 'rows' for(i=0; i<rows; i++) { // Traverse through the 'columns' for(j=0; j<columns; j++) { // Print Elements of 2D Array printf("%d ", A[i][j]); } printf("\n"); } } int main() { // Declare a 2D-Array or matrix with size "MAXROWS"X"MAXCOLUMNS" int A[MAXROWS][MAXCOLUMNS]; int rows, columns, i, j; // Take the Rows size and Columns size from user printf("Enter the Rows size for Matrices(1-100): "); scanf("%d", &rows); printf("Enter the Columns Size for Matrices(1-100): "); scanf("%d", &columns); // check for array bounds if(rows >= MAXROWS || rows <= 0 || columns >= MAXCOLUMNS || columns <= 0) { printf("Invalid Row or Column, Please Try again \n"); return 0; } // Take the user input for 1st Matrix - 'A' printf("Enter Elements for the Matrix(A) of Size %dX%d: \n", rows, columns); readMatrix(rows, columns, A); // Print the Result printf("Elements of the 2D-Array or Matrix A are : \n"); displayMatrix(rows, columns, A); return 0; } |
To better understand the program, We have added a lot of comments to the program. Please go through the comments if you face any doubts.
Program Output:
Let’s compile and Run the program.
Compile the Program.
$ gcc passing-2darray-func.c
Run the Program
Test 1: Normal cases:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
$ ./a.out Enter the Rows size for Matrices(1-100): 4 Enter the Columns Size for Matrices(1-100): 3 Enter Elements for the Matrix(A) of Size 4X3: A[0][0]: 10 A[0][1]: 15 A[0][2]: 20 A[1][0]: 25 A[1][1]: 30 A[1][2]: 35 A[2][0]: 40 A[2][1]: 45 A[2][2]: 50 A[3][0]: 55 A[3][1]: 60 A[3][2]: 65 Elements of the 2D-Array or Matrix A are : 10 15 20 25 30 35 40 45 50 55 60 65 $ |
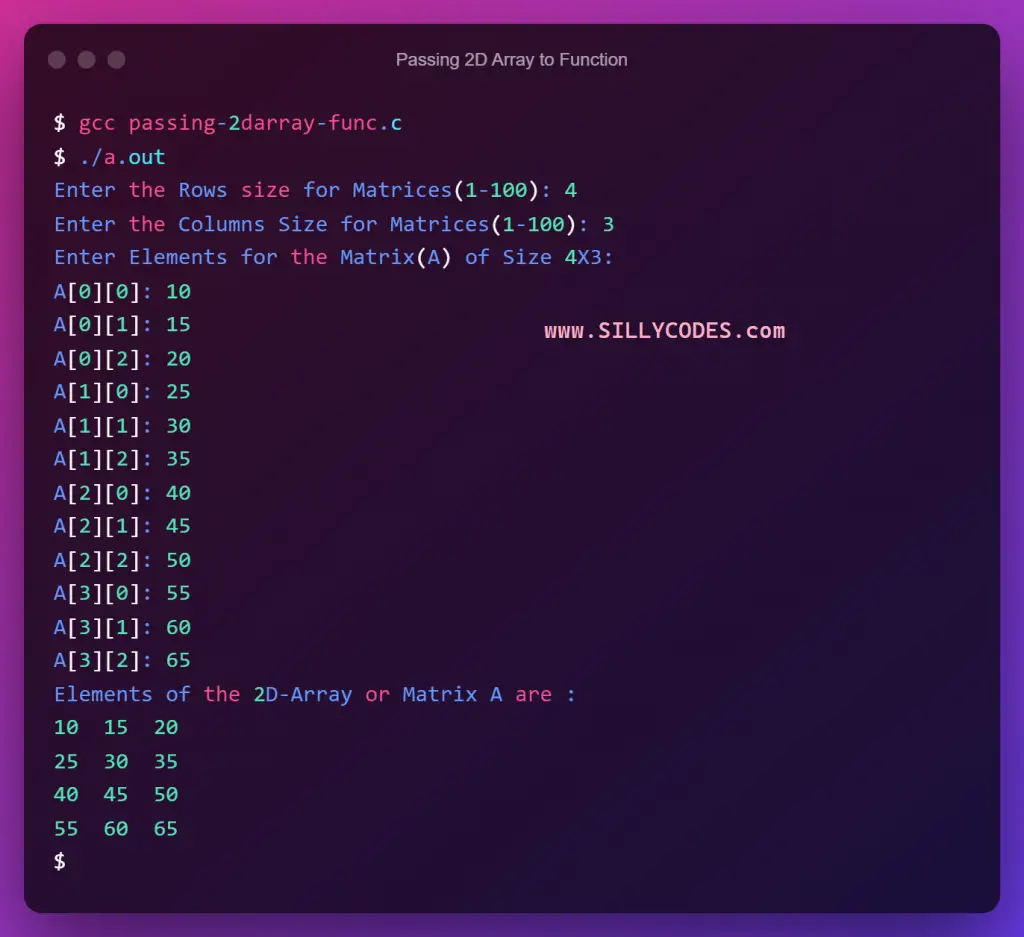
As we can see from the above output, The program is properly reading and printing the 2D Array or Matrix.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
$ ./a.out Enter the Rows size for Matrices(1-100): 2 Enter the Columns Size for Matrices(1-100): 2 Enter Elements for the Matrix(A) of Size 2X2: A[0][0]: 10 A[0][1]: 20 A[1][0]: 30 A[1][1]: 40 Elements of the 2D-Array or Matrix A are : 10 20 30 40 $ ./a.out Enter the Rows size for Matrices(1-100): 3 Enter the Columns Size for Matrices(1-100): 3 Enter Elements for the Matrix(A) of Size 3X3: A[0][0]: 1 A[0][1]: 2 A[0][2]: 3 A[1][0]: 4 A[1][1]: 5 A[1][2]: 6 A[2][0]: 7 A[2][1]: 8 A[2][2]: 9 Elements of the 2D-Array or Matrix A are : 1 2 3 4 5 6 7 8 9 $ |
Test 2: When the user enters Invalid Size:
1 2 3 4 5 6 7 8 9 10 |
// Negative Tests $ ./a.out Enter the Rows size for Matrices(1-100): 777 Enter the Columns Size for Matrices(1-100): 4 Invalid Row or Column, Please Try again $ ./a.out Enter the Rows size for Matrices(1-100): 2 Enter the Columns Size for Matrices(1-100): 2222 Invalid Row or Column, Please Try again $ |
As excepted, When the user enters an array size that is out of the present limit (1 to 100), The program is displaying the error message – Invalid Row or Column, Please Try again.
Remove the Prompt Message while reading the 2D Array Input:
Let’s make a one-line change to the above program to remove the info message in the readMatrix() function. So that we no longer get the element index details during the array input.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 |
/* Program to Add Two Matrices in C SillyCodes.com */ #include <stdio.h> // Create 'MAXROWS' and "MAXCOLUMNS" constants. // If you are using same number, then we can also use single constant. const int MAXROWS=100; const int MAXCOLUMNS=100; /** * @brief - Read 2D-Array or Matrix from the user and update 'A' * * @param rows - Rows in 'A' - Matrix(2D-Array) * @param columns - Columns in 'A' * @param A - 'A' is Matrix or 2D-Array */ void readMatrix(int rows, int columns, int A[rows][columns]) { int i, j; // Iterate through the rows for(i=0; i<rows; i++) { // Iterate through the columns for(j=0; j<columns; j++) { // printf("A[%d][%d]: ", i, j); scanf("%d", &A[i][j]); } } } /** * @brief - Print the elements of the 2D-Array or Matrix * * @param rows - Rows in Matrix(2Darray) * @param columns - Columns in 'A' * @param A[][] - 'A' is a Matrix or 2D array */ void displayMatrix(int rows, int columns, int A[rows][columns]) { int i, j; // Traverse through the 'rows' for(i=0; i<rows; i++) { // Traverse through the 'columns' for(j=0; j<columns; j++) { // Print Elements of 2D Array printf("%d ", A[i][j]); } printf("\n"); } } int main() { // Declare a 2D-Array or matrix with size "MAXROWS"X"MAXCOLUMNS" int A[MAXROWS][MAXCOLUMNS]; int rows, columns, i, j; // Take the Rows size and Columns size from user printf("Enter the Rows size for Matrices(1-100): "); scanf("%d", &rows); printf("Enter the Columns Size for Matrices(1-100): "); scanf("%d", &columns); // check for array bounds if(rows >= MAXROWS || rows <= 0 || columns >= MAXCOLUMNS || columns <= 0) { printf("Invalid Row or Column, Please Try again \n"); return 0; } // Take the user input for 1st Matrix - 'A' printf("Enter Elements for the Matrix(A) of Size %dX%d: \n", rows, columns); readMatrix(rows, columns, A); // Print the Result printf("Elements of the 2D-Array or Matrix A are : \n"); displayMatrix(rows, columns, A); return 0; } |
We commented the printf at line 31 in the readMatrix() function.
Program Output:
Compile and Run the Program.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
$ gcc passing-2darray-func.c $ ./a.out Enter the Rows size for Matrices(1-100): 3 Enter the Columns Size for Matrices(1-100): 3 Enter Elements for the Matrix(A) of Size 3X3: 11 22 33 44 55 66 77 88 99 Elements of the 2D-Array or Matrix A are : 11 22 33 44 55 66 77 88 99 $ // Test 2: $ ./a.out Enter the Rows size for Matrices(1-100): 4 Enter the Columns Size for Matrices(1-100): 2 Enter Elements for the Matrix(A) of Size 4X2: 10 20 30 40 50 60 70 80 Elements of the 2D-Array or Matrix A are : 10 20 30 40 50 60 70 80 $ |
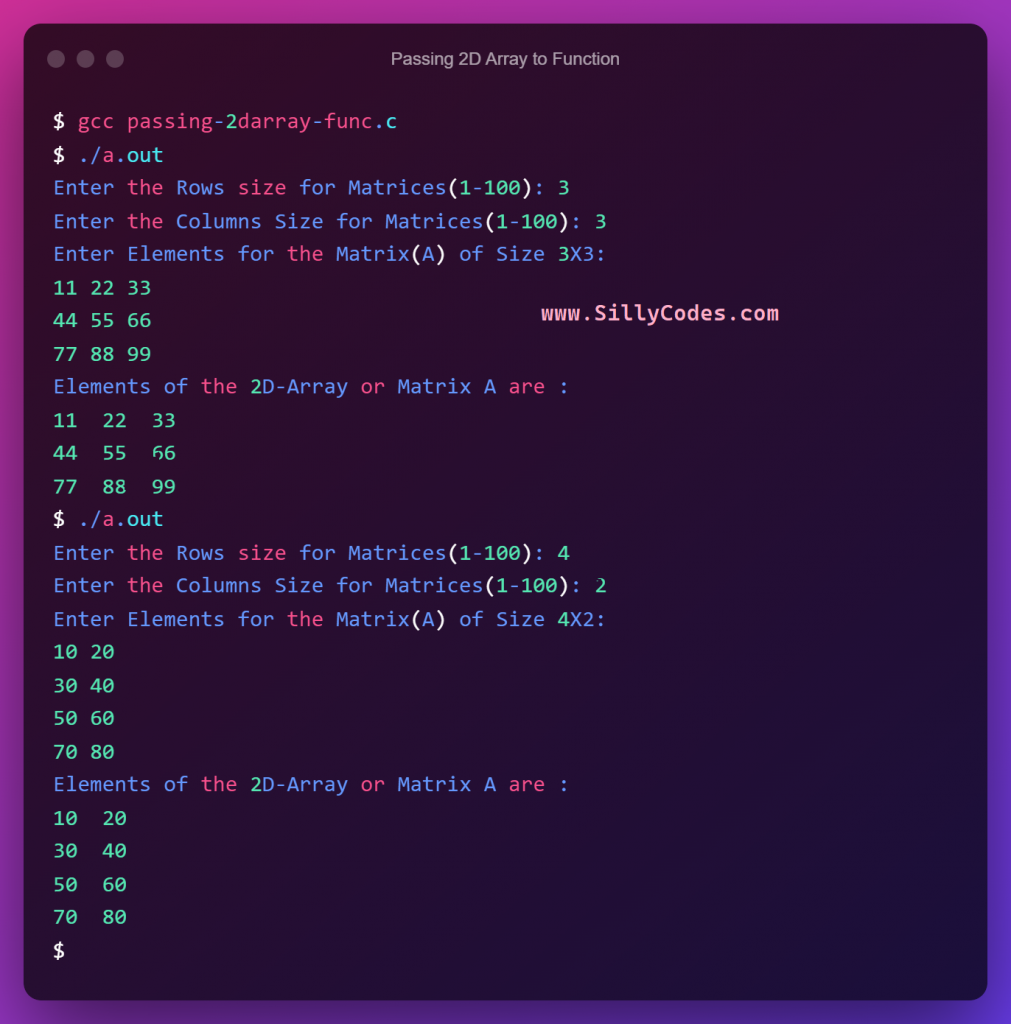
As we can see from the above output, The program is no longer showing the array index during the input phase.
Related Array Programs:
- C Program to Merge Two Sorted Array and Generate Sorted Array
- C Program to Copy array to Another Array
- C Program to Find Second Smallest Element in Array
- C Program to Delete Duplicate Elements in an Array
- C Program to Left Rotate Array by ‘N’ Times
- C Program to Right Rotate Array Elements by ‘N’ Positions
- Linear Search in C Language with Example Programs
- C Program to Count Frequencies of Each Element in the Array
3 Responses
[…] have looked at the Reading and Printing Matrices and How to Pass Matrices to functions programs in earlier articles, In today’s article, We will look at the Addition of Two Matrices in […]
[…] C Program to demonstrate How to Pass 2D Array to Functions […]
[…] C Program to demonstrate How to Pass 2D Array to Functions […]