Calculate LCM using recursion in C Language
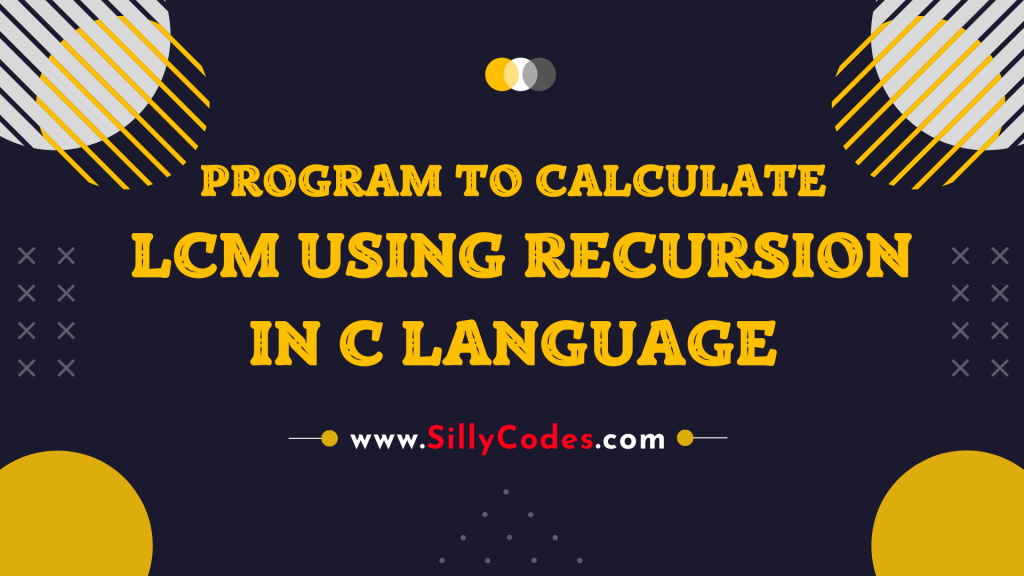
LCM using recursion in C Program Description:
Write a program to calculate the LCM using recursion in C programming language. The program will accept two integers from the user and calculates the LCM. We will use the recursion to calculate the LCM.
Example Input and Output:
Input:
Enter two numbers to calculate LCM: 4 9
Output:
The LCM of 4 and 9 is : 36
Prerequisites:
It is recommended to know the basics of the Functions in C language and Recursion in C language.
LCM using recursion in C Program Explanation:
- Take the two numbers from the user and store them in number1 and number2 variables respectively.
- Check if any of the number1 or number2 is a negative number using (number1 <= 0 || number2 <= 0) condition. Display an error message if the user provides a negative number.
- create a function called
computeLCM, The
computeLCM function takes two integer variables as input (
min,
max) and calculates the LCM using recursion. Here is the explanation of
computeLCM function.
- We should pass the minimum(smaller) value as the first parameter and the maximum(larger) value as the second parameter to the computeLCM function. ( computeLCM(int min, int max))
- Create a static variable called lcm, The lcm variable holds the calculated LCM value.
- Update the lcm variable by adding the max value. lcm += max;
- The base condition for the recursion is lcm%min == 0. So the recursion will continue until the base condition is satisfied.
- If the base condition is not true, Then make a recursive call to the computeLCM function with min and max.
- The minimum and maximum numbers must be supplied as the first and second arguments, respectively to computeLCM function. Use the (number1 > number2) condition to determine whether number1 or number2 is a large value.
- Call the computeLCM function from the main() function. – lcm = computeLCM(min, max);. Store the return value in lcm variable.
- Display the result on the console.
LCM using recursion in C Program:
Here is the program to calculate the LCM of the two numbers recursively in c programming language.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
/* Program to calculate LCM using Recursion */ #include <stdio.h> /** * @brief - Calculate the LCM of given numbers * * @param min - minimum of two numbers * @param max - maximum of two numbers * @return int - LCM of given numbers */ int computeLCM(int min, int max) { // define a static 'lcm' variable. static int lcm = 0; lcm = lcm + max; // lcm += max; // base condition if( lcm%min == 0) { return lcm; } return computeLCM(min, max); } int main() { int number1, number2, lcm; // Get User input printf("Enter two numbers to calculate LCM: "); scanf("%d%d", &number1, &number2); // Negative Number check. if(number1 <= 0 || number2 <= 0) { printf("ERROR: Invalid Input. Please try again\n"); return 0; } // call 'computeLCM' with arguments (min, max) if(number1 > number2) { // call computeLCM function with ('min', 'max') lcm = computeLCM(number2, number1); } else { // call computeLCM function with ('min', 'max') lcm = computeLCM(number1, number2); } printf("The LCM of %d and %d is : %d \n", number1, number2, lcm); return 0; } |
Program Output:
Compile and Run the program.
Test Case 1: Positive Numbers:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$ gcc lcm-recursive.c $ ./a.out Enter two numbers to calculate LCM: 30 7 The LCM of 30 and 7 is : 210 $ ./a.out Enter two numbers to calculate LCM: 2 10 The LCM of 2 and 10 is : 10 $ ./a.out Enter two numbers to calculate LCM: 4 9 The LCM of 4 and 9 is : 36 $ ./a.out Enter two numbers to calculate LCM: 100 730 The LCM of 100 and 730 is : 7300 $ |
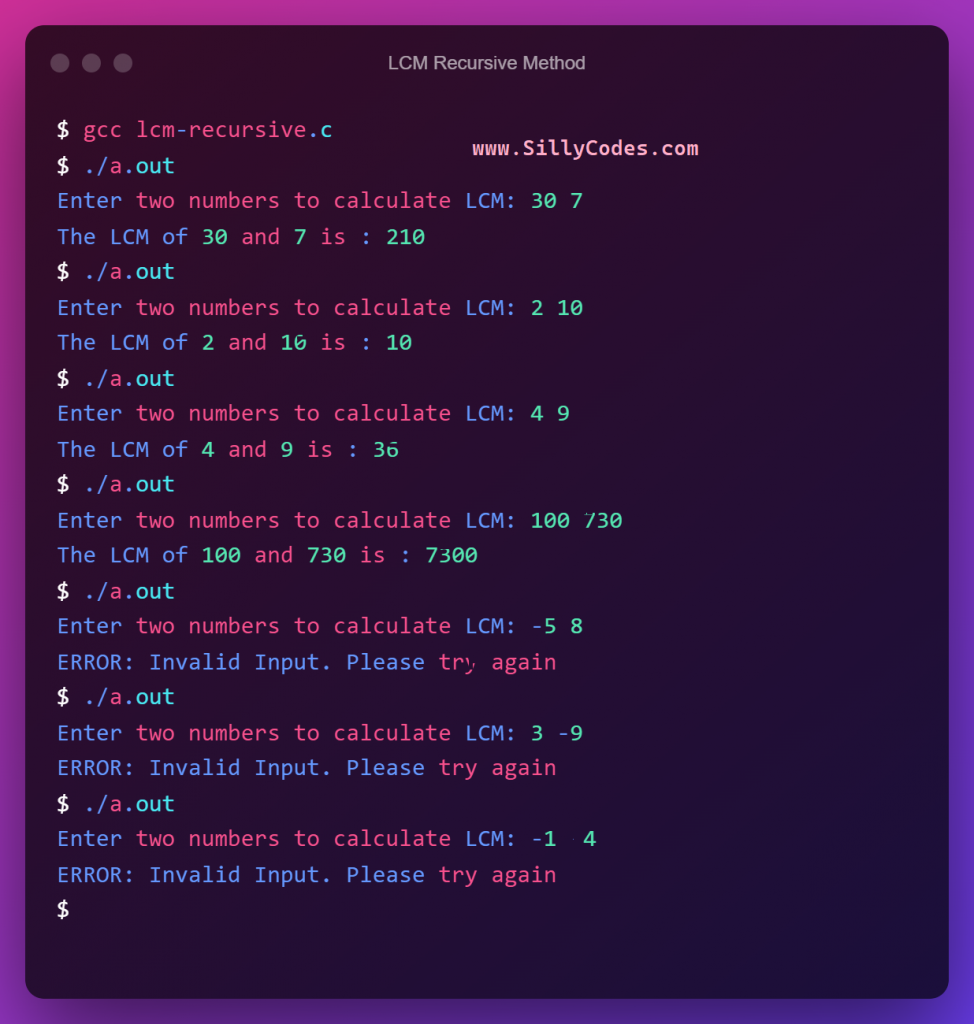
Test Case 2: Negative Numbers:
1 2 3 4 5 6 7 8 9 10 |
$ ./a.out Enter two numbers to calculate LCM: -5 8 ERROR: Invalid Input. Please try again $ ./a.out Enter two numbers to calculate LCM: 3 -9 ERROR: Invalid Input. Please try again $ ./a.out Enter two numbers to calculate LCM: -1 -4 ERROR: Invalid Input. Please try again $ |
As we can see from the above output, The program is providing the excepted output.
Recursion Practice Programs:
- C Program to Find Factorial of Number using Recursion
- C Program to Generate Fibonacci Number using Recursion.
- C Program to calculate the sum of Digits of Number using Recursion
- C Program to Print First N Natural Numbers using Recursion
- C Program to find sum of Fist N Natural Number using Recursion
- Product of N natural Number using Recursion C Program
- Program to Count number of Digits in a Number using Recursion
- Palindrome Number in C using Recursion.
Functions Practice Programs:
- Arithmetic Operations using functions
- Fibonacci Series using function
- Factorial of a Number using functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C
- 100+ C Practice Programs
- C Tutorials Index