Palindrome Number using Recursion in C
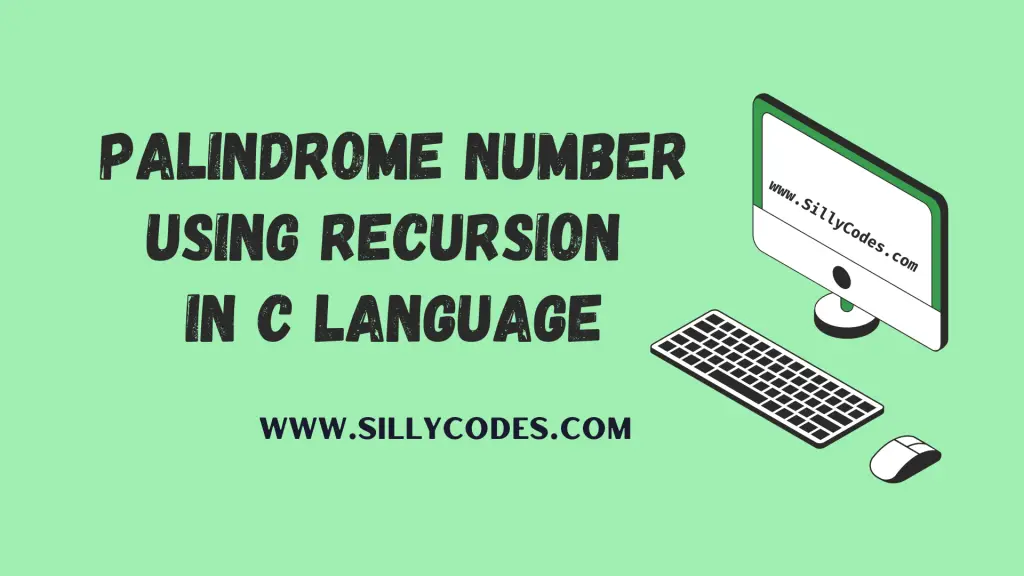
Palindrome Number using Recursion in C Program Description:
Write a Program to check whether the number is a Palindrome Number using Recursion in C programming language. The program will accept a number from the user and verifies if it is a palindrome number.
What is a Palindrome Number:
When a number is a palindrome number, It means that when it is reversed, it should still be the same as the original number.
number == reversed(number);
Example Input and Output:
Input:
Enter a Number: 1234321
Output:
Given number 1234321 is a Palindrome Number
Prerequisites:
We are going to use functions and recursion in the program. So please go through the below articles to better understand the program.
- Functions in C Langauge – Declare, Define, and Call functions
- Recursion in C language – How recursion works with step-by-step explanation
▶️ Related Program: Palindrome Number using the Iterative Method ( Using Loops).
Palindrome Number using Recursion in C Algorithm/Explanation:
- Start the program and take an integer number from the user and store it in number variable.
- We won’t allow a negative number, So check for negativity and display the error message if the number is a negative number. Use the if( number <= 0) condition.
- If the number is a palindrome, Then the original number and reversed number will be the same. So we will first calculate the reverse of the number and then compare it with the original number.
- So we are going to create two functions. One function is to reverse the number ( reverseNumber) and the other function is to check the palindrome ( checkPalindrome).
- We use the
reverseNumber function, To get the reversed value of the number. The reverseNumber function takes two arguments and returns the Reversed number. We have already discussed the Reverse Number Program using Recursion in earlier posts.
- We use the modulus operator ( number%10) to get the last digit of the number and then call the reverseNumber function recursively and pass the number by removing the last digit ( We can remove the last digit using the number/10 division operation)
- The
checkPalindrome function takes the given number(
number) as the input and check whether the
number is palindrome or not.
- The checkPalindrome function first gets the reversed number by calling the reverseNumber function. Then it will compare the reverse version with the original value. – number == <strong>reverseNumber</strong>(number, 0). If both the values are equal, Then the number is a palindrome. So the checkPalindrome function returns 1.
- The checkPalindrome function returns an integer value, We can also return the Boolean values such as true or false. But don’t forget to include the stdbool.h header file.
- Call the isPalindrome function from the main function and display the results based on the return value.
If you observe the function calls, The main() function is calling checkPalindrome function and the checkPalindrome function is calling the reverseNumber function.
📢 Function Call Flow:
main() —> checkPalindrome() –> reverseNumber()
Palindrome Number using Recursion in C Program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 |
/* Palindrome number using recursion in C Author: sillycodes.com */ #include <stdio.h> /** * @brief - Reverse Number 'num' * * @param num - Given Number * @param rev_num - Reversed Number so far. * @return int - Reversed Number */ int reverseNumber(int number, int rev_num) { // base condition if(number <= 0) { return rev_num; } // get remender and update 'rev_num' rev_num = (rev_num * 10) + (number % 10); // make a recursive call to 'reverseNumber' return reverseNumber(number/10, rev_num); } /** * @brief - Check if the 'number' is palindrome * * @param number - Input number * @return int - Returns 'True' if 'number' is palindrome, Otherwise, False */ int checkPalindrome(int number) { if(number == reverseNumber(number, 0)) { // Number is palindrome. // We can also return 'bool', use 'stdbool.h' return 1; } // Not palindrome return 0; } int main() { // Accept User Input int number; printf("Enter a Number: "); scanf("%d", &number); // Negative number check if(number <= 0) { printf("Error: Please provide positive number \n"); return 0; } // call the 'checkPalindrome' pass the 'number' // if return value '1', Then 'number' is palindrome if(checkPalindrome(number)) { // Palindrome printf("Given number %d is a Palindrome Number \n", number); } else { // Not Palindrome printf("Given number %d is Not a Palindrome Number \n", number); } return 0; } |
We used two functions(except main) in the above program, The reverseNumber and checkPalindrome functions. Here are the Prototype details of these functions.
The reverseNumber function:
- int reverseNumber(int number, int rev_num);
- Function_name: reverseNumber
- Argument_list: (int, int) – Takes two integer arguments.
- Return_Value: int – Returns the reversed number.
The checkPalindrome function:
- int checkPalindrome(int number);
- Function_name: checkPalindrome
- Argument_list: (int) – Takes one integer as an argument.
- Return_Value: int – Returns 1 if the number is a palindrome, Otherwise, returns 0.
Program Output:
Compile and run the program using your favorite IDE.
Test 1: Positive Numbers:
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc palindrome-recursive.c $ ./a.out Enter a Number: 1234321 Given number 1234321 is a Palindrome Number $ ./a.out Enter a Number: 98789 Given number 98789 is a Palindrome Number $ ./a.out Enter a Number: 28451 Given number 28451 is Not a Palindrome Number $ |
Test 2: Positive Numbers with Zeros
1 2 3 4 |
$ ./a.out Enter a Number: 101 Given number 101 is a Palindrome Number $ |
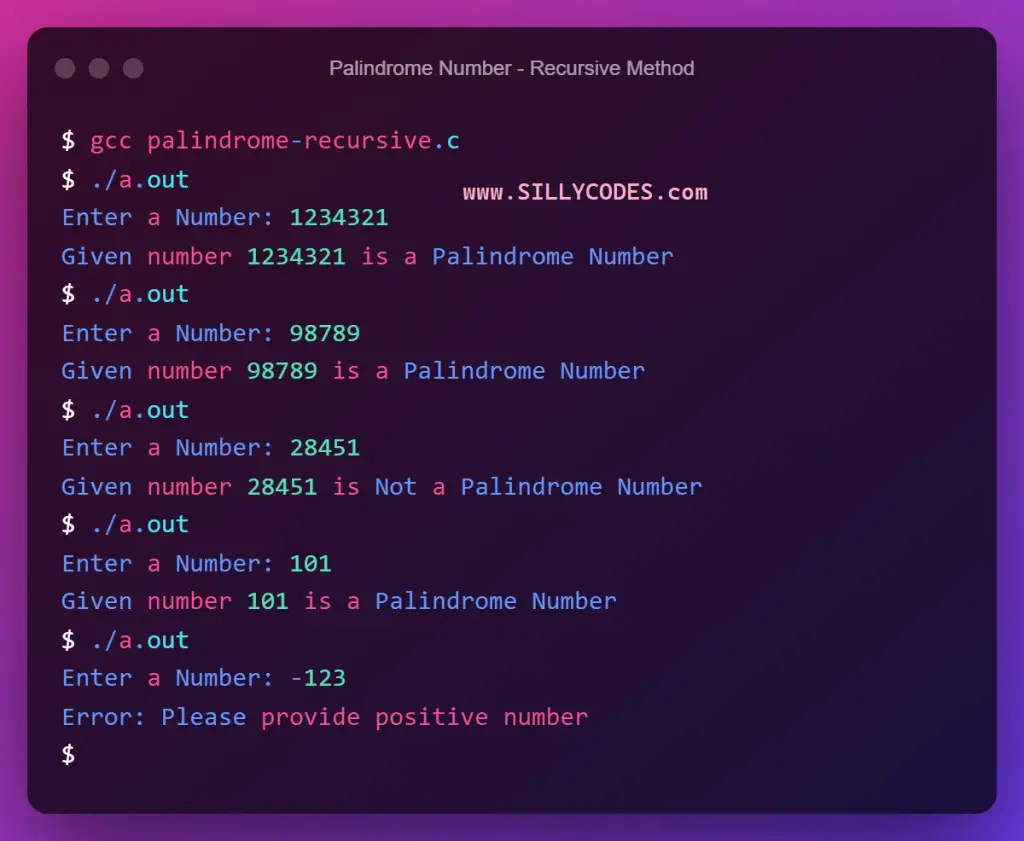
We are getting the expected results.
Test 3: Negative Numbers:
1 2 3 4 |
$ ./a.out Enter a Number: -123 Error: Please provide positive number $ |
Exercise:
Rewrite the above program and make the following changes
- The checkPalindrome function should return boolean values instead of the integer value.
- Use the static variable to calculate the reverse of the number. So change the reverseNumber program to take only one parameter and use the static variable. ( Hint: Check the Method-2 of Reverse Number using Recursion Program )
Recursion Practice Programs:
- C Program to Find Factorial of Number using Recursion
- C Program to Generate Fibonacci Number using Recursion.
- C Program to calculate the sum of Digits of Number using Recursion
- C Program to Print First N Natural Numbers using Recursion
- C Program to find sum of Fist N Natural Number using Recursion
- Product of N natural Number using Recursion C Program
- Program to Count number of Digits in a Number using Recursion
Functions Practice Programs:
- Arithmetic Operations using functions
- Fibonacci Series using function
- Factorial of a Number using functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C
- 100+ C Practice Programs
- C Tutorials Index
1 Response
[…] C Program to check whether the number is Palindrome using Recursion […]