Sum of Digits using Recursion in C Language
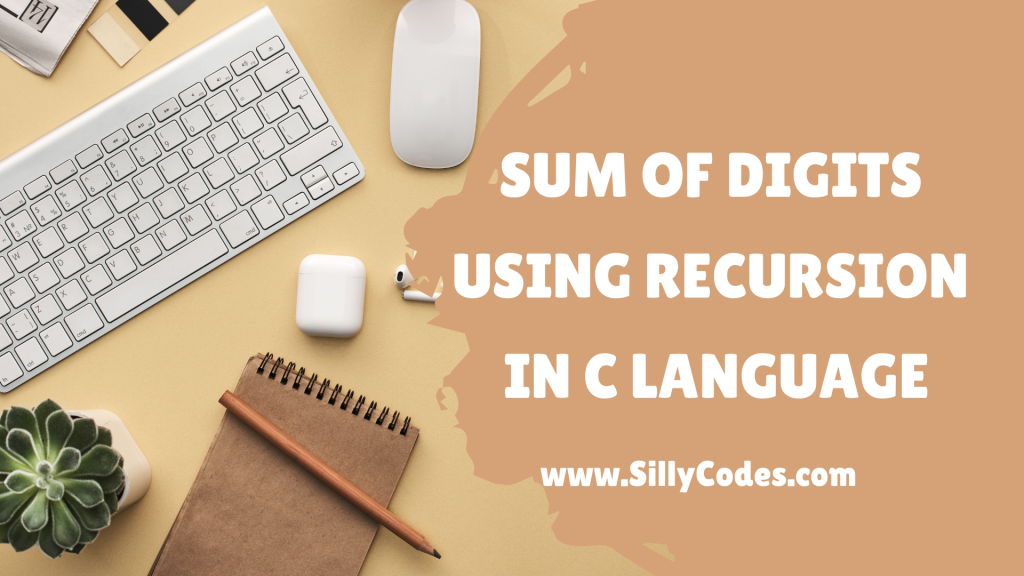
Sum of Digits using Recursion in C Program Description:
Write a Program to calculate the Sum of Digits using Recursion in C Programming language. The program should accept a number from the user and calculate the sum of each individual digit using the recursion. ( Program shouldn’t use the loops)
Example Input and Output:
Input:
Enter a Number : 8734
Output:
Sum of digits of number 8734 is : 22
📢 Related Program: Program to Calculate Sum of Digits of Number using Iterative Method
Pre-Requisites:
We are going to use functions and recursion, So please go through the following articles to learn more about functions and recursion in C.
- Functions in C Language – How to Create, declare, and Call Functions
- Recursion in C Language with Example and Call Flow
Sum of Digits using Recursion in C Algorithm/Explanation:
- Start the program and accept the input number from the user. Store the input value in the variable named n.
- Display an error message if the user enters a negative number and Prompt the user to input again using the Goto Statement
- Use goto INPUT; to jump the program control to INPUT: label. which we added at the start of the program.
- Create a function called
sumOfDigits, The
sumOfDigits function is going to take one integer number(
n) as input and calculates the sum of each individual digit of the number(
n) using recursion. Here are the details of the sumOfDigits function.
- As we are using the recursion, We need to have the base condition. The sumOfDigits function base condition is n == 0. If the number n value becomes zero, Then return the Zero(0). i.e if(n==0) return 0;
- If the number n is not zero, Then get the last digit of the number using the modulus operator ( n%10)
- Make a recursive call to sumOfDigits function, pass the number n by removing the last digit using the division operator ( n/10).
- Finally, Add the above two values – (n % 10) + sumOfDigits(n / 10)
- Call the sumOfDigits function from the main function. Pass the input number as the parameter to sumOfDigits function. – sumOfDigits(n). The sumOfDigits function returns the sum of digits of the given number n.
- Display the result on the console.
Sum of Digits using Recursion in C Program:
Here is the program to calculate the sum of digits in C using the recursion.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
/*     Program: Sum of Digits of a number using Recursion */  #include<stdio.h>  // function declaraion int sumOfDigits(int);  // main function int main() {      INPUT:    // goto label     int n;     // Take Input     printf("Enter a Number : ");     scanf("%d", &n);      // Check for negative number     if(n <= 0)     {         printf("ERROR: Invalid Input, Please provide Positive Number\n");         goto INPUT;     }      // Print Results     printf("Sum of digits of number %d is : %d \n", n, sumOfDigits(n));      return 0; }   /*     Function_name: sumOfDigits     arguments_list: int     return_type: int     Description: Calculate the sum of digits of number 'n' */ int sumOfDigits(int n) {     // Our Base Condition     if(n == 0)         return 0;         /*       Get the last number using modulus operator       Then call the sumOfDigits by removing last number by using division operator.     */     return ( (n % 10) + sumOfDigits(n / 10) ); } |
Prototype details of the sumOfDigits function.
- int sumOfDigits(int);
- Function_name: sumOfDigits
- Arguments_list: int
- Return_type: int
- Program Description: Calculate the sum of digits of the number ‘n’ and return the result back to the caller.
Program Output:
Compile and Run the program using your IDE. we are using the GCC compiler on Ubuntu Linux.
Test Case 1: When the Positive Numbers are entered by the user
1 2 3 4 5 6 7 8 9 10 11 |
$ gcc sumOfDigits-recursive.c $ ./a.out Enter a Number : 562 Sum of digits of number 562 is : 13 $ ./a.out Enter a Number : 8734 Sum of digits of number 8734 is : 22 $ ./a.out Enter a Number : 1000 Sum of digits of number 1000 is : 1 $ |
As we can see from the above output, The program generated a valid output.
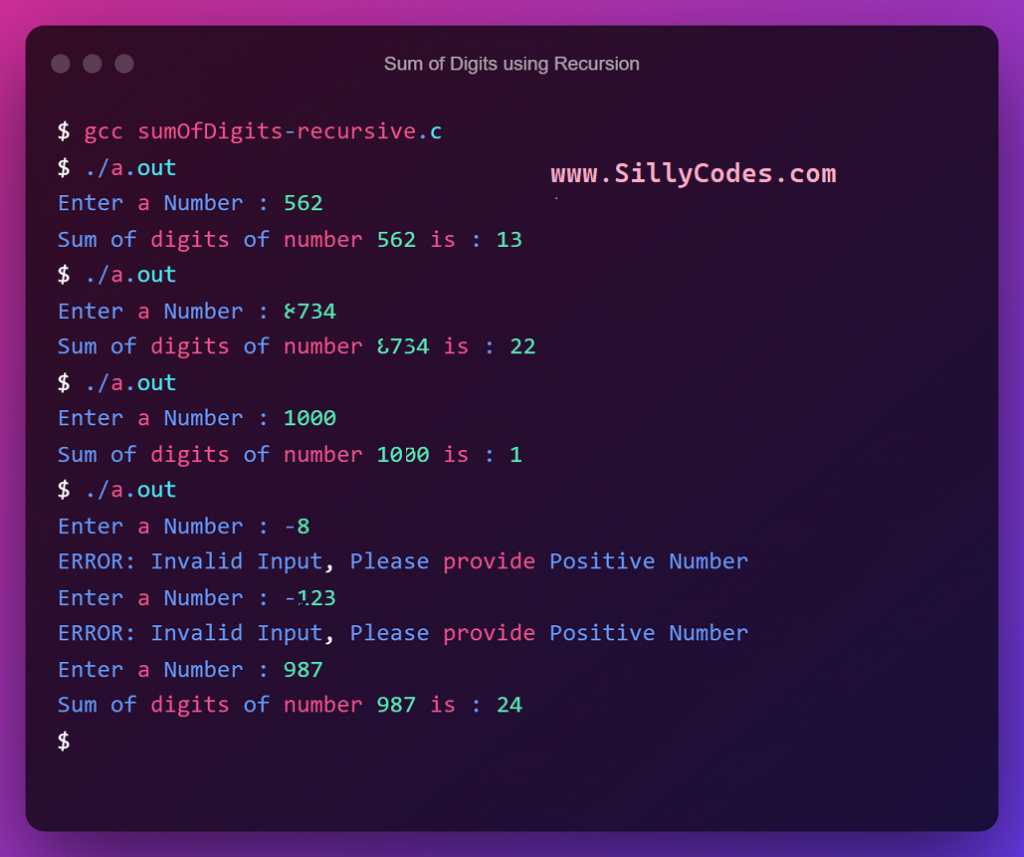
Test Case 2: When the Negative numbers are entered by the user:
1 2 3 4 5 6 7 8 |
$ ./a.out Enter a Number : -8 ERROR: Invalid Input, Please provide Positive Number Enter a Number : -123 ERROR: Invalid Input, Please provide Positive Number Enter a Number : 987 Sum of digits of number 987 is : 24 $ |
If the user enters a negative number, The program is displaying an error message ( ERROR: Invalid Input, Please provide Positive Number). And the program also allows the user to provide the input again. This process will continue until a valid number is provided by the user.
Recursion Practice Programs:
- Calculate Factorial of a number using Recursion in C
- Program to print first n natural numbers using recursion in C
- Calculate Sum of N Natural Numbers in C using Recursion
- Fibonacci Number in C using Recursion
Functions Practice Programs:
- Arithmetic Operations using functions
- Fibonacci Series using function
- Factorial of a Number using functions
- Armstrong Number using Function in C language
- Prime number program using Function
- Program to Caclulate Area of Circle using Function
- Program to Check Even or Odd number using Function
- Binary to Decimal Conversion using function in C
- 100+ C Practice Programs
- C Tutorials Index
1 Response
[…] C Program to Calculate the Sum of Digits using Recursion […]